Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media3D / RayMeshGeometry3DHitTestResult.cs / 1 / RayMeshGeometry3DHitTestResult.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // History: // 06/22/2005 : [....] - Integrated from RayHitTestResult. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; namespace System.Windows.Media.Media3D { ////// The HitTestResult of a Visual3D.HitTest(...) where the parameter /// was a RayHitTestParameter and the ray intersected a MeshGeometry3D. /// /// NOTE: This might have originated as a PointHitTest on a 2D Visual /// which was extended into 3D. /// public sealed class RayMeshGeometry3DHitTestResult : RayHitTestResult { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal RayMeshGeometry3DHitTestResult( Visual3D visualHit, Model3D modelHit, MeshGeometry3D meshHit, Point3D pointHit, double distanceToRayOrigin, int vertexIndex1, int vertexIndex2, int vertexIndex3, Point barycentricCoordinate) : base (visualHit, modelHit) { _meshHit = meshHit; _pointHit = pointHit; _distanceToRayOrigin = distanceToRayOrigin; _vertexIndex1 = vertexIndex1; _vertexIndex2 = vertexIndex2; _vertexIndex3 = vertexIndex3; _barycentricCoordinate = barycentricCoordinate; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// This is a point in 3-space at which the ray intersected /// the geometry of the hit Model3D. This point is in the /// coordinate system of the Visual3D. /// public override Point3D PointHit { get { return _pointHit; } } ////// This is the distance between the ray's origin and the /// point the PointHit. /// public override double DistanceToRayOrigin { get { return _distanceToRayOrigin; } } ////// Index of the 1st vertex of the triangle which was intersected. /// Use this to retrieve the position, texturecoordinate, etc. from /// the MeshHit. /// public int VertexIndex1 { get { return _vertexIndex1; } } ////// Index of the 2nd vertex of the triangle which was intersected. /// Use this to retrieve the position, texturecoordinate, etc. from /// the MeshHit. /// public int VertexIndex2 { get { return _vertexIndex2; } } ////// Index of the 3rd vertex of the triangle which was intersected. /// Use this to retrieve the position, texturecoordinate, etc. from /// the MeshHit. /// public int VertexIndex3 { get { return _vertexIndex3; } } ///public double VertexWeight1 { get { return 1 - VertexWeight2 - VertexWeight3; } } /// public double VertexWeight2 { get { return _barycentricCoordinate.X; } } /// public double VertexWeight3 { get { return _barycentricCoordinate.Y; } } /// /// The MeshGeometry3D which was intersected by the ray. /// public MeshGeometry3D MeshHit { get { return _meshHit; } } //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- internal override void SetDistanceToRayOrigin(double distance) { _distanceToRayOrigin = distance; } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private double _distanceToRayOrigin; // Not readonly because it can be adjusted after construction. private readonly int _vertexIndex1; private readonly int _vertexIndex2; private readonly int _vertexIndex3; private readonly Point _barycentricCoordinate; private readonly MeshGeometry3D _meshHit; private readonly Point3D _pointHit; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // History: // 06/22/2005 : [....] - Integrated from RayHitTestResult. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; namespace System.Windows.Media.Media3D { ////// The HitTestResult of a Visual3D.HitTest(...) where the parameter /// was a RayHitTestParameter and the ray intersected a MeshGeometry3D. /// /// NOTE: This might have originated as a PointHitTest on a 2D Visual /// which was extended into 3D. /// public sealed class RayMeshGeometry3DHitTestResult : RayHitTestResult { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal RayMeshGeometry3DHitTestResult( Visual3D visualHit, Model3D modelHit, MeshGeometry3D meshHit, Point3D pointHit, double distanceToRayOrigin, int vertexIndex1, int vertexIndex2, int vertexIndex3, Point barycentricCoordinate) : base (visualHit, modelHit) { _meshHit = meshHit; _pointHit = pointHit; _distanceToRayOrigin = distanceToRayOrigin; _vertexIndex1 = vertexIndex1; _vertexIndex2 = vertexIndex2; _vertexIndex3 = vertexIndex3; _barycentricCoordinate = barycentricCoordinate; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// This is a point in 3-space at which the ray intersected /// the geometry of the hit Model3D. This point is in the /// coordinate system of the Visual3D. /// public override Point3D PointHit { get { return _pointHit; } } ////// This is the distance between the ray's origin and the /// point the PointHit. /// public override double DistanceToRayOrigin { get { return _distanceToRayOrigin; } } ////// Index of the 1st vertex of the triangle which was intersected. /// Use this to retrieve the position, texturecoordinate, etc. from /// the MeshHit. /// public int VertexIndex1 { get { return _vertexIndex1; } } ////// Index of the 2nd vertex of the triangle which was intersected. /// Use this to retrieve the position, texturecoordinate, etc. from /// the MeshHit. /// public int VertexIndex2 { get { return _vertexIndex2; } } ////// Index of the 3rd vertex of the triangle which was intersected. /// Use this to retrieve the position, texturecoordinate, etc. from /// the MeshHit. /// public int VertexIndex3 { get { return _vertexIndex3; } } ///public double VertexWeight1 { get { return 1 - VertexWeight2 - VertexWeight3; } } /// public double VertexWeight2 { get { return _barycentricCoordinate.X; } } /// public double VertexWeight3 { get { return _barycentricCoordinate.Y; } } /// /// The MeshGeometry3D which was intersected by the ray. /// public MeshGeometry3D MeshHit { get { return _meshHit; } } //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- internal override void SetDistanceToRayOrigin(double distance) { _distanceToRayOrigin = distance; } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private double _distanceToRayOrigin; // Not readonly because it can be adjusted after construction. private readonly int _vertexIndex1; private readonly int _vertexIndex2; private readonly int _vertexIndex3; private readonly Point _barycentricCoordinate; private readonly MeshGeometry3D _meshHit; private readonly Point3D _pointHit; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
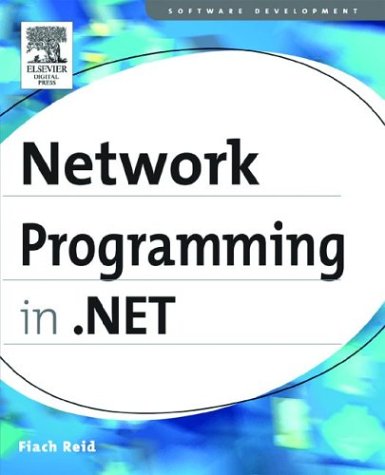
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeValidator.cs
- ReflectionPermission.cs
- TextDecoration.cs
- ProjectionQueryOptionExpression.cs
- XpsSerializationException.cs
- PassportAuthenticationModule.cs
- FixUp.cs
- DocumentViewer.cs
- CacheEntry.cs
- TypeConverterValueSerializer.cs
- AsyncStreamReader.cs
- CommonObjectSecurity.cs
- MobileControlsSectionHelper.cs
- TTSEngineTypes.cs
- MatrixKeyFrameCollection.cs
- SafeNativeMethods.cs
- RegexMatchCollection.cs
- RepeaterItemEventArgs.cs
- EndpointNotFoundException.cs
- EntityObject.cs
- MenuAdapter.cs
- SqlWebEventProvider.cs
- SmiContextFactory.cs
- IDataContractSurrogate.cs
- Matrix3DConverter.cs
- MessageSecurityException.cs
- LineBreakRecord.cs
- DivideByZeroException.cs
- SurrogateEncoder.cs
- WorkflowRuntimeService.cs
- KnownTypes.cs
- basevalidator.cs
- StringWriter.cs
- DispatcherHookEventArgs.cs
- DirectionalLight.cs
- ExpandSegmentCollection.cs
- NaturalLanguageHyphenator.cs
- ImageEditor.cs
- SearchForVirtualItemEventArgs.cs
- RemotingAttributes.cs
- MethodToken.cs
- XmlLanguageConverter.cs
- DataBoundControl.cs
- SHA512Managed.cs
- DataReaderContainer.cs
- Vector3DCollectionConverter.cs
- Random.cs
- BitmapImage.cs
- HttpModulesSection.cs
- WebBrowserUriTypeConverter.cs
- DataBoundControlHelper.cs
- NullRuntimeConfig.cs
- TextPointerBase.cs
- SafeSystemMetrics.cs
- TabControlToolboxItem.cs
- TdsParameterSetter.cs
- XmlValidatingReader.cs
- UIElementParagraph.cs
- FormViewInsertedEventArgs.cs
- InputManager.cs
- PassportAuthentication.cs
- ListSortDescription.cs
- DesignColumnCollection.cs
- Main.cs
- StorageTypeMapping.cs
- DataServiceClientException.cs
- PageCodeDomTreeGenerator.cs
- ImageAttributes.cs
- _NTAuthentication.cs
- ClaimTypeElementCollection.cs
- DateTimeSerializationSection.cs
- MarshalDirectiveException.cs
- XmlSchemaDocumentation.cs
- TypeGenericEnumerableViewSchema.cs
- Content.cs
- FlowDecisionLabelFeature.cs
- DataGridHyperlinkColumn.cs
- X509ServiceCertificateAuthenticationElement.cs
- OdbcCommand.cs
- Misc.cs
- SqlProviderServices.cs
- DispatcherHooks.cs
- XPathSingletonIterator.cs
- SqlCacheDependencyDatabaseCollection.cs
- ImageKeyConverter.cs
- PrivilegeNotHeldException.cs
- RemoveStoryboard.cs
- ScrollBar.cs
- BulletChrome.cs
- AspNetSynchronizationContext.cs
- Input.cs
- ClientRoleProvider.cs
- UrlMappingsModule.cs
- RegexCaptureCollection.cs
- FacetDescription.cs
- OutputCacheProfile.cs
- TableSectionStyle.cs
- AsymmetricKeyExchangeFormatter.cs
- CacheVirtualItemsEvent.cs
- CodeConstructor.cs