Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Base / System / Security / RightsManagement / UnsignedPublishLicense.cs / 1 / UnsignedPublishLicense.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class implements the UnsignedPublishLicense class // this class is the first step in the RightsManagement publishing process // // History: // 06/01/2005: IgorBel : Initial Implementation // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Globalization; using System.Windows; using MS.Internal.Security.RightsManagement; using MS.Internal; using SecurityHelper=MS.Internal.WindowsBase.SecurityHelper; // Disable message about unknown message numbers so as to allow the suppression // of PreSharp warnings (whose numbers are unknown to the compiler). #pragma warning disable 1634, 1691 namespace System.Security.RightsManagement { ////// UnsignedPublishLicense class is used to represent publish license information before it was signed. /// It can be used to build and sign Publish License, and it also can be used to build and serialize Publish License Template. /// ////// Critical: This class expose access to methods that eventually do one or more of the the following /// 1. call into unmanaged code /// 2. affects state/data that will eventually cross over unmanaged code boundary /// 3. Return some RM related information which is considered private /// /// TreatAsSafe: This attribute is automatically applied to all public entry points. All the public entry points have /// Demands for RightsManagementPermission at entry to counter the possible attacks that do /// not lead to the unamanged code directly(which is protected by another Demand there) but rather leave /// some status/data behind which eventually might cross the unamanaged boundary. /// [SecurityCritical(SecurityCriticalScope.Everything)] public class UnsignedPublishLicense { ////// This constructor builds an empty Publish License. /// public UnsignedPublishLicense() { SecurityHelper.DemandRightsManagementPermission(); _grantCollection = new Collection(); _contentId = Guid.NewGuid(); } /// /// This constructor accepts XrML Publish License template as a parameter. It parses the XrRML document /// and initializes class based on that. /// public UnsignedPublishLicense(string publishLicenseTemplate) :this () { SecurityHelper.DemandRightsManagementPermission(); if (publishLicenseTemplate == null) { throw new ArgumentNullException("publishLicenseTemplate"); } using(IssuanceLicense issuanceLicense = new IssuanceLicense( DateTime.MinValue, // validFrom, - default DateTime.MaxValue, // validUntil, - default null, // referralInfoName, null, // referralInfoUrl, null, // owner, publishLicenseTemplate, SafeRightsManagementHandle.InvalidHandle, // boundLicenseHandle, _contentId, // contentId, null, // grantCollection null, // Localized Name Description pairs collection null, // Application Specific Data Dictionary 0, // validity interval days null)) // revocation point info { // update our instance data based on the parsed information issuanceLicense.UpdateUnsignedPublishLicense(this); } } ////// This functions signs the Publish License offline, and as a result produces 2 objects. It makes an instance of the PublishLicense /// and it also builds an instance of the UseLicense, which represeents the authors UseLicense /// public PublishLicense Sign(SecureEnvironment secureEnvironment, out UseLicense authorUseLicense) { SecurityHelper.DemandRightsManagementPermission(); if (secureEnvironment == null) { throw new ArgumentNullException("secureEnvironment"); } // in case owner wasn't specified we can just assume default owner // based on the user identity that was used to build the secure environment ContentUser contentOwner; if (_owner != null) { contentOwner = _owner; } else { contentOwner = secureEnvironment.User; } using(IssuanceLicense issuanceLicense = new IssuanceLicense( DateTime.MinValue, // validFrom, - default DateTime.MaxValue, // validUntil, - default _referralInfoName, _referralInfoUri, contentOwner, null, SafeRightsManagementHandle.InvalidHandle, // boundLicenseHandle, _contentId, Grants, LocalizedNameDescriptionDictionary, ApplicationSpecificDataDictionary, _rightValidityIntervalDays, _revocationPoint)) { // The SecureEnvironment constructor makes sure ClientSession cannot be null. // Accordingly suppressing preSharp warning about having to validate ClientSession. #pragma warning suppress 6506 return secureEnvironment.ClientSession.SignIssuanceLicense(issuanceLicense, out authorUseLicense); } } ////// This property represent the user that will be the owner of the Pubish lciense. /// This owner is also associated to the Owner node in the issuance license XrML. /// By default if Owner isn't specified it will be assigned to the identity of the user /// signing the UnsignedPublishLicense /// public ContentUser Owner { get { SecurityHelper.DemandRightsManagementPermission(); return _owner; } set { SecurityHelper.DemandRightsManagementPermission(); _owner = value; } } ////// This property in conbimation with ReferralInfoUri is commonly used to enable /// consumers of the protected content to contact the author/publisher of the content. /// public string ReferralInfoName { get { SecurityHelper.DemandRightsManagementPermission(); return _referralInfoName; } set { SecurityHelper.DemandRightsManagementPermission(); _referralInfoName = value; } } ////// This property in conbimation with ReferralInfoName is commonly used to enable /// consumers of the protected content to contact the author/publisher of the content. /// public Uri ReferralInfoUri { get { SecurityHelper.DemandRightsManagementPermission(); return _referralInfoUri; } set { SecurityHelper.DemandRightsManagementPermission(); _referralInfoUri = value; } } ////// The ContentId is created by the publisher and can be used to match content to UseLicense and PublishLicenses. /// public Guid ContentId { get { SecurityHelper.DemandRightsManagementPermission(); return _contentId; } set { SecurityHelper.DemandRightsManagementPermission(); // Guid is a value type, so it can never be null; therefore, there is no nreed to check this _contentId = value; } } ////// This collection is used to assign rights to users in an Unsigned Publish License. /// public ICollectionGrants { get { SecurityHelper.DemandRightsManagementPermission(); return _grantCollection; } } /// /// This collection is used to assign Name Description pairs of strings to the /// unsigned publish License templates based on the Local Id as a Key of the dictionary. /// public IDictionaryLocalizedNameDescriptionDictionary { get { SecurityHelper.DemandRightsManagementPermission(); if (_localizedNameDescriptionDictionary == null) { _localizedNameDescriptionDictionary = new Dictionary (10); } return _localizedNameDescriptionDictionary; } } /// /// This method produces serialized Publish License XRML template. /// override public string ToString() { SecurityHelper.DemandRightsManagementPermission(); using(IssuanceLicense issuanceLicense = new IssuanceLicense( DateTime.MinValue, DateTime.MaxValue, _referralInfoName, _referralInfoUri, _owner, null, SafeRightsManagementHandle.InvalidHandle, // boundLicenseHandle, _contentId, Grants, LocalizedNameDescriptionDictionary, ApplicationSpecificDataDictionary, _rightValidityIntervalDays, _revocationPoint)) { return issuanceLicense.ToString(); } } ////// This constructor accepts Signed XrML Publish License as a parameter. /// It decrypts and parses parses the XrRML document and initializes class based on that. /// internal UnsignedPublishLicense(SafeRightsManagementHandle boundLicenseHandle, string publishLicenseTemplate) :this () { Invariant.Assert(!boundLicenseHandle.IsInvalid); Invariant.Assert(publishLicenseTemplate != null); using(IssuanceLicense issuanceLicense = new IssuanceLicense( DateTime.MinValue, // validFrom, - default DateTime.MaxValue, // validUntil, - default null, // referralInfoName, null, // referralInfoUrl, null, // owner, publishLicenseTemplate, boundLicenseHandle, // boundLicenseHandle, _contentId, // contentId, null, // grantCollection null, // Localized Name Description pairs collection null, // Application Specific Data Dictionary 0, // validity interval days null)) // revocation point info { // update our instance data based on the parsed information issuanceLicense.UpdateUnsignedPublishLicense(this); } } ////// This property sets/gets the number of days for a time condition of an issuance license. /// Unmanged SDK treats 0 as a missing(not set) value /// internal int RightValidityIntervalDays { get { return _rightValidityIntervalDays; } set { // Invariant.Assert(value>=0); _rightValidityIntervalDays = value; } } ////// This collection is used to assign Name Description pairs of strings to the /// unsigned publish License templates based on the Local Id as a Key of the dictionary. /// internal IDictionaryApplicationSpecificDataDictionary { get { SecurityHelper.DemandRightsManagementPermission(); if (_applicationSpecificDataDictionary == null) { _applicationSpecificDataDictionary = new Dictionary (5); } return _applicationSpecificDataDictionary; } } /// /// This property enables us to implemen a revocation list pass through for template based publishing /// takes from DRM SDK: /// Revocation list can revoke end-user licenses, server licensor certificates, or /// almost anything else with an identifying GUID. See Revocation for a list of the /// items that can be revoked. The URL provided should refer to the list file itself. /// The rights management system handles checking for a valid revocation list. /// This function should only be called once, since subsequent calls will overwrite /// the previous revocation point in the issuance license. /// The public key must be a base-64 encoded string. /// Note that if there is no revocation point set in the license, the license can /// still be revoked by a revocation list signed by the issuer of the license. /// internal RevocationPoint RevocationPoint { get { return _revocationPoint; } set { _revocationPoint = value; } } private Guid _contentId; private ContentUser _owner; private ICollection_grantCollection; private string _referralInfoName; private Uri _referralInfoUri; private IDictionary _localizedNameDescriptionDictionary = null; private IDictionary _applicationSpecificDataDictionary = null; private int _rightValidityIntervalDays; // default 0 value is treated by the RM SDK as a non-defined missing value private RevocationPoint _revocationPoint; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class implements the UnsignedPublishLicense class // this class is the first step in the RightsManagement publishing process // // History: // 06/01/2005: IgorBel : Initial Implementation // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Globalization; using System.Windows; using MS.Internal.Security.RightsManagement; using MS.Internal; using SecurityHelper=MS.Internal.WindowsBase.SecurityHelper; // Disable message about unknown message numbers so as to allow the suppression // of PreSharp warnings (whose numbers are unknown to the compiler). #pragma warning disable 1634, 1691 namespace System.Security.RightsManagement { ////// UnsignedPublishLicense class is used to represent publish license information before it was signed. /// It can be used to build and sign Publish License, and it also can be used to build and serialize Publish License Template. /// ////// Critical: This class expose access to methods that eventually do one or more of the the following /// 1. call into unmanaged code /// 2. affects state/data that will eventually cross over unmanaged code boundary /// 3. Return some RM related information which is considered private /// /// TreatAsSafe: This attribute is automatically applied to all public entry points. All the public entry points have /// Demands for RightsManagementPermission at entry to counter the possible attacks that do /// not lead to the unamanged code directly(which is protected by another Demand there) but rather leave /// some status/data behind which eventually might cross the unamanaged boundary. /// [SecurityCritical(SecurityCriticalScope.Everything)] public class UnsignedPublishLicense { ////// This constructor builds an empty Publish License. /// public UnsignedPublishLicense() { SecurityHelper.DemandRightsManagementPermission(); _grantCollection = new Collection(); _contentId = Guid.NewGuid(); } /// /// This constructor accepts XrML Publish License template as a parameter. It parses the XrRML document /// and initializes class based on that. /// public UnsignedPublishLicense(string publishLicenseTemplate) :this () { SecurityHelper.DemandRightsManagementPermission(); if (publishLicenseTemplate == null) { throw new ArgumentNullException("publishLicenseTemplate"); } using(IssuanceLicense issuanceLicense = new IssuanceLicense( DateTime.MinValue, // validFrom, - default DateTime.MaxValue, // validUntil, - default null, // referralInfoName, null, // referralInfoUrl, null, // owner, publishLicenseTemplate, SafeRightsManagementHandle.InvalidHandle, // boundLicenseHandle, _contentId, // contentId, null, // grantCollection null, // Localized Name Description pairs collection null, // Application Specific Data Dictionary 0, // validity interval days null)) // revocation point info { // update our instance data based on the parsed information issuanceLicense.UpdateUnsignedPublishLicense(this); } } ////// This functions signs the Publish License offline, and as a result produces 2 objects. It makes an instance of the PublishLicense /// and it also builds an instance of the UseLicense, which represeents the authors UseLicense /// public PublishLicense Sign(SecureEnvironment secureEnvironment, out UseLicense authorUseLicense) { SecurityHelper.DemandRightsManagementPermission(); if (secureEnvironment == null) { throw new ArgumentNullException("secureEnvironment"); } // in case owner wasn't specified we can just assume default owner // based on the user identity that was used to build the secure environment ContentUser contentOwner; if (_owner != null) { contentOwner = _owner; } else { contentOwner = secureEnvironment.User; } using(IssuanceLicense issuanceLicense = new IssuanceLicense( DateTime.MinValue, // validFrom, - default DateTime.MaxValue, // validUntil, - default _referralInfoName, _referralInfoUri, contentOwner, null, SafeRightsManagementHandle.InvalidHandle, // boundLicenseHandle, _contentId, Grants, LocalizedNameDescriptionDictionary, ApplicationSpecificDataDictionary, _rightValidityIntervalDays, _revocationPoint)) { // The SecureEnvironment constructor makes sure ClientSession cannot be null. // Accordingly suppressing preSharp warning about having to validate ClientSession. #pragma warning suppress 6506 return secureEnvironment.ClientSession.SignIssuanceLicense(issuanceLicense, out authorUseLicense); } } ////// This property represent the user that will be the owner of the Pubish lciense. /// This owner is also associated to the Owner node in the issuance license XrML. /// By default if Owner isn't specified it will be assigned to the identity of the user /// signing the UnsignedPublishLicense /// public ContentUser Owner { get { SecurityHelper.DemandRightsManagementPermission(); return _owner; } set { SecurityHelper.DemandRightsManagementPermission(); _owner = value; } } ////// This property in conbimation with ReferralInfoUri is commonly used to enable /// consumers of the protected content to contact the author/publisher of the content. /// public string ReferralInfoName { get { SecurityHelper.DemandRightsManagementPermission(); return _referralInfoName; } set { SecurityHelper.DemandRightsManagementPermission(); _referralInfoName = value; } } ////// This property in conbimation with ReferralInfoName is commonly used to enable /// consumers of the protected content to contact the author/publisher of the content. /// public Uri ReferralInfoUri { get { SecurityHelper.DemandRightsManagementPermission(); return _referralInfoUri; } set { SecurityHelper.DemandRightsManagementPermission(); _referralInfoUri = value; } } ////// The ContentId is created by the publisher and can be used to match content to UseLicense and PublishLicenses. /// public Guid ContentId { get { SecurityHelper.DemandRightsManagementPermission(); return _contentId; } set { SecurityHelper.DemandRightsManagementPermission(); // Guid is a value type, so it can never be null; therefore, there is no nreed to check this _contentId = value; } } ////// This collection is used to assign rights to users in an Unsigned Publish License. /// public ICollectionGrants { get { SecurityHelper.DemandRightsManagementPermission(); return _grantCollection; } } /// /// This collection is used to assign Name Description pairs of strings to the /// unsigned publish License templates based on the Local Id as a Key of the dictionary. /// public IDictionaryLocalizedNameDescriptionDictionary { get { SecurityHelper.DemandRightsManagementPermission(); if (_localizedNameDescriptionDictionary == null) { _localizedNameDescriptionDictionary = new Dictionary (10); } return _localizedNameDescriptionDictionary; } } /// /// This method produces serialized Publish License XRML template. /// override public string ToString() { SecurityHelper.DemandRightsManagementPermission(); using(IssuanceLicense issuanceLicense = new IssuanceLicense( DateTime.MinValue, DateTime.MaxValue, _referralInfoName, _referralInfoUri, _owner, null, SafeRightsManagementHandle.InvalidHandle, // boundLicenseHandle, _contentId, Grants, LocalizedNameDescriptionDictionary, ApplicationSpecificDataDictionary, _rightValidityIntervalDays, _revocationPoint)) { return issuanceLicense.ToString(); } } ////// This constructor accepts Signed XrML Publish License as a parameter. /// It decrypts and parses parses the XrRML document and initializes class based on that. /// internal UnsignedPublishLicense(SafeRightsManagementHandle boundLicenseHandle, string publishLicenseTemplate) :this () { Invariant.Assert(!boundLicenseHandle.IsInvalid); Invariant.Assert(publishLicenseTemplate != null); using(IssuanceLicense issuanceLicense = new IssuanceLicense( DateTime.MinValue, // validFrom, - default DateTime.MaxValue, // validUntil, - default null, // referralInfoName, null, // referralInfoUrl, null, // owner, publishLicenseTemplate, boundLicenseHandle, // boundLicenseHandle, _contentId, // contentId, null, // grantCollection null, // Localized Name Description pairs collection null, // Application Specific Data Dictionary 0, // validity interval days null)) // revocation point info { // update our instance data based on the parsed information issuanceLicense.UpdateUnsignedPublishLicense(this); } } ////// This property sets/gets the number of days for a time condition of an issuance license. /// Unmanged SDK treats 0 as a missing(not set) value /// internal int RightValidityIntervalDays { get { return _rightValidityIntervalDays; } set { // Invariant.Assert(value>=0); _rightValidityIntervalDays = value; } } ////// This collection is used to assign Name Description pairs of strings to the /// unsigned publish License templates based on the Local Id as a Key of the dictionary. /// internal IDictionaryApplicationSpecificDataDictionary { get { SecurityHelper.DemandRightsManagementPermission(); if (_applicationSpecificDataDictionary == null) { _applicationSpecificDataDictionary = new Dictionary (5); } return _applicationSpecificDataDictionary; } } /// /// This property enables us to implemen a revocation list pass through for template based publishing /// takes from DRM SDK: /// Revocation list can revoke end-user licenses, server licensor certificates, or /// almost anything else with an identifying GUID. See Revocation for a list of the /// items that can be revoked. The URL provided should refer to the list file itself. /// The rights management system handles checking for a valid revocation list. /// This function should only be called once, since subsequent calls will overwrite /// the previous revocation point in the issuance license. /// The public key must be a base-64 encoded string. /// Note that if there is no revocation point set in the license, the license can /// still be revoked by a revocation list signed by the issuer of the license. /// internal RevocationPoint RevocationPoint { get { return _revocationPoint; } set { _revocationPoint = value; } } private Guid _contentId; private ContentUser _owner; private ICollection_grantCollection; private string _referralInfoName; private Uri _referralInfoUri; private IDictionary _localizedNameDescriptionDictionary = null; private IDictionary _applicationSpecificDataDictionary = null; private int _rightValidityIntervalDays; // default 0 value is treated by the RM SDK as a non-defined missing value private RevocationPoint _revocationPoint; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
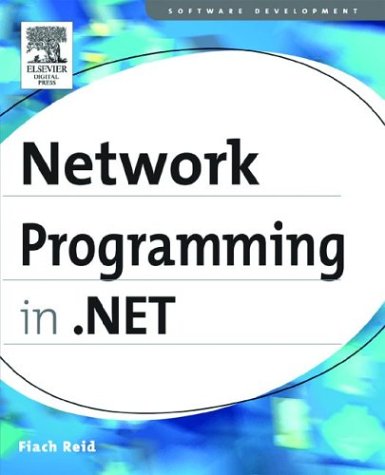
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IPAddressCollection.cs
- OleDbPropertySetGuid.cs
- TreeViewAutomationPeer.cs
- HandleCollector.cs
- OneOfConst.cs
- ClientTargetCollection.cs
- DbDataAdapter.cs
- StaticResourceExtension.cs
- BCLDebug.cs
- ArgumentValidation.cs
- ColumnWidthChangingEvent.cs
- Utils.cs
- PositiveTimeSpanValidator.cs
- TextStore.cs
- HMACMD5.cs
- MouseActionConverter.cs
- Serializer.cs
- StaticExtensionConverter.cs
- TextUtf8RawTextWriter.cs
- SoapTypeAttribute.cs
- CommandSet.cs
- BoundColumn.cs
- CatalogPartChrome.cs
- FakeModelItemImpl.cs
- ConfigurationStrings.cs
- InvalidOperationException.cs
- LinearKeyFrames.cs
- CellParagraph.cs
- VisualBrush.cs
- ParameterBuilder.cs
- DataFormats.cs
- SqlMetaData.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- Misc.cs
- ProgressBarBrushConverter.cs
- TraceXPathNavigator.cs
- TimeSpan.cs
- ControlType.cs
- PersianCalendar.cs
- ChannelSinkStacks.cs
- BamlResourceSerializer.cs
- CancellableEnumerable.cs
- QueryHandler.cs
- KeyEventArgs.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- SystemIPGlobalStatistics.cs
- RuntimeWrappedException.cs
- RemoteWebConfigurationHostStream.cs
- StrokeFIndices.cs
- Monitor.cs
- KerberosReceiverSecurityToken.cs
- DesignerEditorPartChrome.cs
- SystemResourceKey.cs
- ClientWindowsAuthenticationMembershipProvider.cs
- CompilerGlobalScopeAttribute.cs
- DataGridViewElement.cs
- InternalMappingException.cs
- PhysicalOps.cs
- CheckBoxBaseAdapter.cs
- DocumentCollection.cs
- SchemaCollectionPreprocessor.cs
- QueryContinueDragEventArgs.cs
- ClientTargetCollection.cs
- PromptStyle.cs
- ProcessStartInfo.cs
- ExpressionConverter.cs
- DayRenderEvent.cs
- CqlGenerator.cs
- RegexMatch.cs
- EndpointIdentityConverter.cs
- ChannelToken.cs
- Merger.cs
- XmlProcessingInstruction.cs
- Verify.cs
- WindowsPrincipal.cs
- DPAPIProtectedConfigurationProvider.cs
- ProviderSettings.cs
- SqlDeflator.cs
- Contracts.cs
- SecurityHelper.cs
- XmlHierarchicalEnumerable.cs
- Pair.cs
- MemberRelationshipService.cs
- UriExt.cs
- Currency.cs
- Listbox.cs
- LinearGradientBrush.cs
- ObfuscationAttribute.cs
- BroadcastEventHelper.cs
- BitmapEffectOutputConnector.cs
- SafeProcessHandle.cs
- FormatterConverter.cs
- VoiceChangeEventArgs.cs
- QuaternionConverter.cs
- DummyDataSource.cs
- KeyValuePair.cs
- CustomAttributeFormatException.cs
- SqlDataSourceAdvancedOptionsForm.cs
- ScriptingSectionGroup.cs
- TreeView.cs