Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / WebControls / BoundColumn.cs / 1 / BoundColumn.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Web; using System.Web.UI; using System.Security.Permissions; using System.Web.Util; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class BoundColumn : DataGridColumn { ///Creates a column bounded to a data field in a ///. /// public static readonly string thisExpr = "!"; private PropertyDescriptor boundFieldDesc; private bool boundFieldDescValid; private string boundField; private string formatting; ///Specifies a string that represents "this". This field is read-only. ////// public BoundColumn() { } ///Initializes a new instance of a ///class. /// [ WebCategory("Data"), DefaultValue(""), WebSysDescription(SR.BoundColumn_DataField) ] public virtual string DataField { get { object o = ViewState["DataField"]; if (o != null) return (string)o; return String.Empty; } set { ViewState["DataField"] = value; OnColumnChanged(); } } ///Gets or sets the field name from the data model bound to this column. ////// [ WebCategory("Behavior"), DefaultValue(""), WebSysDescription(SR.BoundColumn_DataFormatString) ] public virtual string DataFormatString { get { object o = ViewState["DataFormatString"]; if (o != null) return (string)o; return String.Empty; } set { ViewState["DataFormatString"] = value; OnColumnChanged(); } } ///Gets or sets the display format of data in this /// column. ////// [ WebCategory("Behavior"), DefaultValue(false), WebSysDescription(SR.BoundColumn_ReadOnly) ] public virtual bool ReadOnly { get { object o = ViewState["ReadOnly"]; if (o != null) return (bool)o; return false; } set { ViewState["ReadOnly"] = value; OnColumnChanged(); } } ///Gets or sets the property that prevents modification to data /// in this column. ////// protected virtual string FormatDataValue(object dataValue) { string formattedValue = String.Empty; if (!DataBinder.IsNull(dataValue)) { if (formatting.Length == 0) { formattedValue = dataValue.ToString(); } else { formattedValue = String.Format(CultureInfo.CurrentCulture, formatting, dataValue); } } return formattedValue; } ////// public override void Initialize() { base.Initialize(); boundFieldDesc = null; boundFieldDescValid = false; boundField = DataField; formatting = DataFormatString; } ////// public override void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { base.InitializeCell(cell, columnIndex, itemType); Control childControl = null; Control boundControl = null; switch (itemType) { case ListItemType.Header: case ListItemType.Footer: break; case ListItemType.Item: case ListItemType.AlternatingItem: case ListItemType.SelectedItem: if (DataField.Length != 0) { boundControl = cell; } break; case ListItemType.EditItem: if (ReadOnly == true) { goto case ListItemType.Item; } else { // TextBox editor = new TextBox(); childControl = editor; if (boundField.Length != 0) { boundControl = editor; } } break; } if (childControl != null) { cell.Controls.Add(childControl); } if (boundControl != null) { boundControl.DataBinding += new EventHandler(this.OnDataBindColumn); } } ///Initializes a cell in the DataGridColumn. ////// private void OnDataBindColumn(object sender, EventArgs e) { Debug.Assert(DataField.Length != 0, "Shouldn't be DataBinding without a DataField"); Control boundControl = (Control)sender; DataGridItem item = (DataGridItem)boundControl.NamingContainer; object dataItem = item.DataItem; if (boundFieldDescValid == false) { if (!boundField.Equals(thisExpr)) { boundFieldDesc = TypeDescriptor.GetProperties(dataItem).Find(boundField, true); if ((boundFieldDesc == null) && !DesignMode) { throw new HttpException(SR.GetString(SR.Field_Not_Found, boundField)); } } boundFieldDescValid = true; } object data = dataItem; string dataValue; if ((boundFieldDesc == null) && DesignMode) { dataValue = SR.GetString(SR.Sample_Databound_Text); } else { if (boundFieldDesc != null) { data = boundFieldDesc.GetValue(dataItem); } dataValue = FormatDataValue(data); } if (boundControl is TableCell) { if (dataValue.Length == 0) { dataValue = " "; } ((TableCell)boundControl).Text = dataValue; } else { Debug.Assert(boundControl is TextBox, "Expected the bound control to be a TextBox"); ((TextBox)boundControl).Text = dataValue; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Web; using System.Web.UI; using System.Security.Permissions; using System.Web.Util; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class BoundColumn : DataGridColumn { ///Creates a column bounded to a data field in a ///. /// public static readonly string thisExpr = "!"; private PropertyDescriptor boundFieldDesc; private bool boundFieldDescValid; private string boundField; private string formatting; ///Specifies a string that represents "this". This field is read-only. ////// public BoundColumn() { } ///Initializes a new instance of a ///class. /// [ WebCategory("Data"), DefaultValue(""), WebSysDescription(SR.BoundColumn_DataField) ] public virtual string DataField { get { object o = ViewState["DataField"]; if (o != null) return (string)o; return String.Empty; } set { ViewState["DataField"] = value; OnColumnChanged(); } } ///Gets or sets the field name from the data model bound to this column. ////// [ WebCategory("Behavior"), DefaultValue(""), WebSysDescription(SR.BoundColumn_DataFormatString) ] public virtual string DataFormatString { get { object o = ViewState["DataFormatString"]; if (o != null) return (string)o; return String.Empty; } set { ViewState["DataFormatString"] = value; OnColumnChanged(); } } ///Gets or sets the display format of data in this /// column. ////// [ WebCategory("Behavior"), DefaultValue(false), WebSysDescription(SR.BoundColumn_ReadOnly) ] public virtual bool ReadOnly { get { object o = ViewState["ReadOnly"]; if (o != null) return (bool)o; return false; } set { ViewState["ReadOnly"] = value; OnColumnChanged(); } } ///Gets or sets the property that prevents modification to data /// in this column. ////// protected virtual string FormatDataValue(object dataValue) { string formattedValue = String.Empty; if (!DataBinder.IsNull(dataValue)) { if (formatting.Length == 0) { formattedValue = dataValue.ToString(); } else { formattedValue = String.Format(CultureInfo.CurrentCulture, formatting, dataValue); } } return formattedValue; } ////// public override void Initialize() { base.Initialize(); boundFieldDesc = null; boundFieldDescValid = false; boundField = DataField; formatting = DataFormatString; } ////// public override void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { base.InitializeCell(cell, columnIndex, itemType); Control childControl = null; Control boundControl = null; switch (itemType) { case ListItemType.Header: case ListItemType.Footer: break; case ListItemType.Item: case ListItemType.AlternatingItem: case ListItemType.SelectedItem: if (DataField.Length != 0) { boundControl = cell; } break; case ListItemType.EditItem: if (ReadOnly == true) { goto case ListItemType.Item; } else { // TextBox editor = new TextBox(); childControl = editor; if (boundField.Length != 0) { boundControl = editor; } } break; } if (childControl != null) { cell.Controls.Add(childControl); } if (boundControl != null) { boundControl.DataBinding += new EventHandler(this.OnDataBindColumn); } } ///Initializes a cell in the DataGridColumn. ////// private void OnDataBindColumn(object sender, EventArgs e) { Debug.Assert(DataField.Length != 0, "Shouldn't be DataBinding without a DataField"); Control boundControl = (Control)sender; DataGridItem item = (DataGridItem)boundControl.NamingContainer; object dataItem = item.DataItem; if (boundFieldDescValid == false) { if (!boundField.Equals(thisExpr)) { boundFieldDesc = TypeDescriptor.GetProperties(dataItem).Find(boundField, true); if ((boundFieldDesc == null) && !DesignMode) { throw new HttpException(SR.GetString(SR.Field_Not_Found, boundField)); } } boundFieldDescValid = true; } object data = dataItem; string dataValue; if ((boundFieldDesc == null) && DesignMode) { dataValue = SR.GetString(SR.Sample_Databound_Text); } else { if (boundFieldDesc != null) { data = boundFieldDesc.GetValue(dataItem); } dataValue = FormatDataValue(data); } if (boundControl is TableCell) { if (dataValue.Length == 0) { dataValue = " "; } ((TableCell)boundControl).Text = dataValue; } else { Debug.Assert(boundControl is TextBox, "Expected the bound control to be a TextBox"); ((TextBox)boundControl).Text = dataValue; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
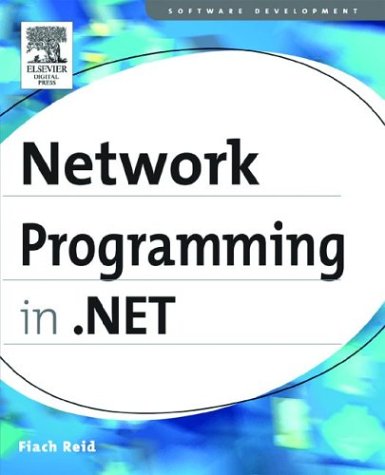
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Triangle.cs
- ListViewCommandEventArgs.cs
- SQLRoleProvider.cs
- Math.cs
- DataViewSetting.cs
- DbConnectionPoolGroupProviderInfo.cs
- DependencyObjectValidator.cs
- ZipFileInfoCollection.cs
- BaseTemplateCodeDomTreeGenerator.cs
- NopReturnReader.cs
- FormsAuthenticationModule.cs
- ObjectPersistData.cs
- _IPv4Address.cs
- XmlObjectSerializerReadContext.cs
- CriticalExceptions.cs
- DataShape.cs
- _SafeNetHandles.cs
- TextMessageEncoder.cs
- CompleteWizardStep.cs
- StoryFragments.cs
- UriSection.cs
- Activity.cs
- InvalidateEvent.cs
- DocumentGrid.cs
- ManualResetEvent.cs
- Region.cs
- GeneralTransformGroup.cs
- _IPv6Address.cs
- DataGridViewColumnCollectionEditor.cs
- GeometryConverter.cs
- MostlySingletonList.cs
- ModelUIElement3D.cs
- WorkflowClientDeliverMessageWrapper.cs
- CroppedBitmap.cs
- EntityAdapter.cs
- WebServiceHandlerFactory.cs
- SocketAddress.cs
- ApplicationManager.cs
- RealizationDrawingContextWalker.cs
- ServerValidateEventArgs.cs
- DropAnimation.xaml.cs
- WorkflowMarkupSerializationException.cs
- MetadataSource.cs
- StructuredTypeEmitter.cs
- sqlser.cs
- PropertyValue.cs
- TypeBrowser.xaml.cs
- PageSettings.cs
- CustomSignedXml.cs
- EntityStoreSchemaFilterEntry.cs
- ChtmlTextWriter.cs
- SamlConditions.cs
- ComboBoxHelper.cs
- XslTransform.cs
- StylusButtonEventArgs.cs
- UiaCoreTypesApi.cs
- SplitterEvent.cs
- XmlSchemaAppInfo.cs
- ContentPlaceHolder.cs
- XPathSingletonIterator.cs
- Geometry3D.cs
- StringInfo.cs
- TextEditorTables.cs
- GifBitmapEncoder.cs
- MessageQueuePermissionAttribute.cs
- HttpServerUtilityWrapper.cs
- HttpClientCertificate.cs
- InnerItemCollectionView.cs
- CodeIdentifiers.cs
- CodeCompiler.cs
- EventTrigger.cs
- RelatedEnd.cs
- DirectionalLight.cs
- _Events.cs
- SiteMapDataSource.cs
- ToolBarPanel.cs
- DataGridViewRowCollection.cs
- CapabilitiesRule.cs
- DocumentPageTextView.cs
- InteropBitmapSource.cs
- TransformValueSerializer.cs
- UnsafeNativeMethods.cs
- ResolveNameEventArgs.cs
- DynamicValidator.cs
- WindowsSlider.cs
- DatasetMethodGenerator.cs
- ObjectTag.cs
- XmlAttributeHolder.cs
- PropertyChangedEventManager.cs
- LinkTarget.cs
- UpdatePanel.cs
- UIServiceHelper.cs
- InputDevice.cs
- CodeTypeDeclarationCollection.cs
- UnknownBitmapDecoder.cs
- DataServiceRequest.cs
- ScrollProperties.cs
- TableAdapterManagerGenerator.cs
- ElementUtil.cs
- TypedReference.cs