Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CommonUI / System / Drawing / Printing / PageSettings.cs / 1 / PageSettings.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Drawing.Internal; ////// /// [Serializable] public class PageSettings : ICloneable { internal PrinterSettings printerSettings; private TriState color = TriState.Default; private PaperSize paperSize; private PaperSource paperSource; private PrinterResolution printerResolution; private TriState landscape = TriState.Default; private Margins margins = new Margins(); ////// Specifies /// settings that apply to a single page. /// ////// /// public PageSettings() : this(new PrinterSettings()) { } ////// Initializes a new instance of the ///class using /// the default printer. /// /// /// public PageSettings(PrinterSettings printerSettings) { Debug.Assert(printerSettings != null, "printerSettings == null"); this.printerSettings = printerSettings; } ///Initializes a new instance of the ///class using /// the specified printer. /// /// public Rectangle Bounds { get { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); IntPtr modeHandle = printerSettings.GetHdevmode(); Rectangle pageBounds = GetBounds(modeHandle); SafeNativeMethods.GlobalFree(new HandleRef(this, modeHandle)); return pageBounds; } } ////// Gets the bounds of the page, taking into account the Landscape property. /// ////// /// public bool Color { get { if (color.IsDefault) return printerSettings.GetModeField(ModeField.Color, SafeNativeMethods.DMCOLOR_MONOCHROME) == SafeNativeMethods.DMCOLOR_COLOR; else return(bool) color; } set { color = value;} } ////// Gets or sets a value indicating whether the page is printed in color. /// ////// /// public float HardMarginX { [SuppressMessage("Microsoft.Security", "CA2106:SecureAsserts")] get { // SECREVIEW: // Its ok to Assert the permission and Let the user know the HardMarginX. // This is consistent with the Bounds property. IntSecurity.AllPrintingAndUnmanagedCode.Assert(); float hardMarginX = 0; DeviceContext dc = printerSettings.CreateDeviceContext(this); try { int dpiX = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.LOGPIXELSX); int hardMarginX_DU = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.PHYSICALOFFSETX); hardMarginX = hardMarginX_DU * 100 / dpiX; } finally { dc.Dispose(); } return hardMarginX; } } ///Returns the x dimension of the hard margin ////// /// public float HardMarginY { get { // SECREVIEW: // Its ok to Assert the permission and Let the user know the HardMarginY. // This is consistent with the Bounds property. float hardMarginY = 0; DeviceContext dc = printerSettings.CreateDeviceContext(this); try { int dpiY = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.LOGPIXELSY); int hardMarginY_DU = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.PHYSICALOFFSETY); hardMarginY = hardMarginY_DU * 100 / dpiY; } finally { dc.Dispose(); } return hardMarginY; } } ///Returns the y dimension of the hard margin ////// /// public bool Landscape { get { if (landscape.IsDefault) return printerSettings.GetModeField(ModeField.Orientation, SafeNativeMethods.DMORIENT_PORTRAIT) == SafeNativeMethods.DMORIENT_LANDSCAPE; else return(bool) landscape; } set { landscape = value;} } ////// Gets or sets a value indicating whether the page should be printed in landscape or portrait orientation. /// ////// /// public Margins Margins { get { return margins;} set { margins = value;} } ////// Gets or sets a value indicating the margins for this page. /// /// ////// /// public PaperSize PaperSize { get { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); return GetPaperSize(IntPtr.Zero); } set { paperSize = value;} } ////// Gets or sets the paper size. /// ////// /// public PaperSource PaperSource { get { if (paperSource == null) { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); IntPtr modeHandle = printerSettings.GetHdevmode(); IntPtr modePointer = SafeNativeMethods.GlobalLock(new HandleRef(this, modeHandle)); SafeNativeMethods.DEVMODE mode = (SafeNativeMethods.DEVMODE) UnsafeNativeMethods.PtrToStructure(modePointer, typeof(SafeNativeMethods.DEVMODE)); PaperSource result = PaperSourceFromMode(mode); SafeNativeMethods.GlobalUnlock(new HandleRef(this, modeHandle)); SafeNativeMethods.GlobalFree(new HandleRef(this, modeHandle)); return result; } else return paperSource; } set { paperSource = value;} } ////// Gets or sets a value indicating the paper source (i.e. upper bin). /// /// ////// /// public RectangleF PrintableArea { get { RectangleF printableArea = new RectangleF(); DeviceContext dc = printerSettings.CreateInformationContext(this); HandleRef hdc = new HandleRef(dc, dc.Hdc); try { int dpiX = UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.LOGPIXELSX); int dpiY = UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.LOGPIXELSY); if (!this.Landscape) { // // Need to convert the printable area to 100th of an inch from the device units printableArea.X = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.PHYSICALOFFSETX) * 100 / dpiX; printableArea.Y = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.PHYSICALOFFSETY) * 100 / dpiY; printableArea.Width = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.HORZRES) * 100 / dpiX; printableArea.Height = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.VERTRES) * 100 / dpiY; } else { // // Need to convert the printable area to 100th of an inch from the device units printableArea.Y = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.PHYSICALOFFSETX) * 100 / dpiX; printableArea.X = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.PHYSICALOFFSETY) * 100 / dpiY; printableArea.Height = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.HORZRES) * 100 / dpiX; printableArea.Width = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.VERTRES) * 100 / dpiY; } } finally { dc.Dispose(); } return printableArea; } } ////// Gets the PrintableArea for the printer. Units = 100ths of an inch. /// ////// /// public PrinterResolution PrinterResolution { get { if (printerResolution == null) { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); IntPtr modeHandle = printerSettings.GetHdevmode(); IntPtr modePointer = SafeNativeMethods.GlobalLock(new HandleRef(this, modeHandle)); SafeNativeMethods.DEVMODE mode = (SafeNativeMethods.DEVMODE) UnsafeNativeMethods.PtrToStructure(modePointer, typeof(SafeNativeMethods.DEVMODE)); PrinterResolution result = PrinterResolutionFromMode(mode); SafeNativeMethods.GlobalUnlock(new HandleRef(this, modeHandle)); SafeNativeMethods.GlobalFree(new HandleRef(this, modeHandle)); return result; } else return printerResolution; } set { printerResolution = value; } } ////// Gets or sets the printer resolution for the page. /// ////// /// public PrinterSettings PrinterSettings { get { return printerSettings;} set { if (value == null) value = new PrinterSettings(); printerSettings = value; } } ////// Gets or sets the /// associated printer settings. /// ////// /// Copies the settings and margins. /// public object Clone() { PageSettings result = (PageSettings) MemberwiseClone(); result.margins = (Margins) margins.Clone(); return result; } ////// /// public void CopyToHdevmode(IntPtr hdevmode) { IntSecurity.AllPrinting.Demand(); IntPtr modePointer = SafeNativeMethods.GlobalLock(new HandleRef(null, hdevmode)); SafeNativeMethods.DEVMODE mode = (SafeNativeMethods.DEVMODE) UnsafeNativeMethods.PtrToStructure(modePointer, typeof(SafeNativeMethods.DEVMODE)); if (color.IsNotDefault && ((mode.dmFields & SafeNativeMethods.DM_COLOR) == SafeNativeMethods.DM_COLOR)) mode.dmColor = (short) (((bool) color) ? SafeNativeMethods.DMCOLOR_COLOR : SafeNativeMethods.DMCOLOR_MONOCHROME); if (landscape.IsNotDefault && ((mode.dmFields & SafeNativeMethods.DM_ORIENTATION) == SafeNativeMethods.DM_ORIENTATION)) mode.dmOrientation = (short) (((bool) landscape) ? SafeNativeMethods.DMORIENT_LANDSCAPE : SafeNativeMethods.DMORIENT_PORTRAIT); if (paperSize != null) { if ((mode.dmFields & SafeNativeMethods.DM_PAPERSIZE) == SafeNativeMethods.DM_PAPERSIZE) { mode.dmPaperSize = (short) paperSize.RawKind; } bool setWidth = false; bool setLength = false; if ((mode.dmFields & SafeNativeMethods.DM_PAPERLENGTH) == SafeNativeMethods.DM_PAPERLENGTH) { // dmPaperLength is always in tenths of millimeter but paperSizes are in hundredth of inch .. // so we need to convert :: use PrinterUnitConvert.Convert(value, PrinterUnit.TenthsOfAMillimeter /*fromUnit*/, PrinterUnit.Display /*ToUnit*/) mode.dmPaperLength = (short)PrinterUnitConvert.Convert(paperSize.Height, PrinterUnit.Display, PrinterUnit.TenthsOfAMillimeter); setLength = true; } if ((mode.dmFields & SafeNativeMethods.DM_PAPERWIDTH) == SafeNativeMethods.DM_PAPERWIDTH) { mode.dmPaperWidth = (short)PrinterUnitConvert.Convert(paperSize.Width, PrinterUnit.Display, PrinterUnit.TenthsOfAMillimeter); setWidth = true; } if (paperSize.Kind == PaperKind.Custom) { if (!setLength) { mode.dmFields |= SafeNativeMethods.DM_PAPERLENGTH; mode.dmPaperLength = (short)PrinterUnitConvert.Convert(paperSize.Height, PrinterUnit.Display, PrinterUnit.TenthsOfAMillimeter); } if (!setWidth) { mode.dmFields |= SafeNativeMethods.DM_PAPERWIDTH; mode.dmPaperWidth = (short)PrinterUnitConvert.Convert(paperSize.Width, PrinterUnit.Display, PrinterUnit.TenthsOfAMillimeter); } } } if (paperSource != null && ((mode.dmFields & SafeNativeMethods.DM_DEFAULTSOURCE) == SafeNativeMethods.DM_DEFAULTSOURCE)) { mode.dmDefaultSource = (short) paperSource.RawKind; } if (printerResolution != null) { if (printerResolution.Kind == PrinterResolutionKind.Custom) { if ((mode.dmFields & SafeNativeMethods.DM_PRINTQUALITY) == SafeNativeMethods.DM_PRINTQUALITY) { mode.dmPrintQuality = (short) printerResolution.X; } if ((mode.dmFields & SafeNativeMethods.DM_YRESOLUTION) == SafeNativeMethods.DM_YRESOLUTION) { mode.dmYResolution = (short) printerResolution.Y; } } else { if ((mode.dmFields & SafeNativeMethods.DM_PRINTQUALITY) == SafeNativeMethods.DM_PRINTQUALITY) { mode.dmPrintQuality = (short) printerResolution.Kind; } } } Marshal.StructureToPtr(mode, modePointer, false); int retCode = SafeNativeMethods.DocumentProperties(NativeMethods.NullHandleRef, NativeMethods.NullHandleRef, printerSettings.PrinterName, modePointer, modePointer, SafeNativeMethods.DM_IN_BUFFER | SafeNativeMethods.DM_OUT_BUFFER); if (retCode < 0) { SafeNativeMethods.GlobalFree(new HandleRef(null, modePointer)); } SafeNativeMethods.GlobalUnlock(new HandleRef(null, hdevmode)); } // This function shows up big on profiles, so we need to make it fast internal Rectangle GetBounds(IntPtr modeHandle) { Rectangle pageBounds; PaperSize size = GetPaperSize(modeHandle); if (GetLandscape(modeHandle)) pageBounds = new Rectangle(0, 0, size.Height, size.Width); else pageBounds = new Rectangle(0, 0, size.Width, size.Height); return pageBounds; } private bool GetLandscape(IntPtr modeHandle) { if (landscape.IsDefault) return printerSettings.GetModeField(ModeField.Orientation, SafeNativeMethods.DMORIENT_PORTRAIT, modeHandle) == SafeNativeMethods.DMORIENT_LANDSCAPE; else return(bool) landscape; } private PaperSize GetPaperSize(IntPtr modeHandle) { if (paperSize == null) { bool ownHandle = false; if (modeHandle == IntPtr.Zero) { modeHandle = printerSettings.GetHdevmode(); ownHandle = true; } IntPtr modePointer = SafeNativeMethods.GlobalLock(new HandleRef(null, modeHandle)); SafeNativeMethods.DEVMODE mode = (SafeNativeMethods.DEVMODE) UnsafeNativeMethods.PtrToStructure(modePointer, typeof(SafeNativeMethods.DEVMODE)); PaperSize result = PaperSizeFromMode(mode); SafeNativeMethods.GlobalUnlock(new HandleRef(null, modeHandle)); if (ownHandle) SafeNativeMethods.GlobalFree(new HandleRef(null, modeHandle)); return result; } else return paperSize; } private PaperSize PaperSizeFromMode(SafeNativeMethods.DEVMODE mode) { PaperSize[] sizes = printerSettings.Get_PaperSizes(); if ((mode.dmFields & SafeNativeMethods.DM_PAPERSIZE) == SafeNativeMethods.DM_PAPERSIZE) { for (int i = 0; i < sizes.Length; i++) { if ((int)sizes[i].RawKind == mode.dmPaperSize) return sizes[i]; } } return new PaperSize(PaperKind.Custom, "custom", //mode.dmPaperWidth, mode.dmPaperLength); PrinterUnitConvert.Convert(mode.dmPaperWidth, PrinterUnit.TenthsOfAMillimeter, PrinterUnit.Display), PrinterUnitConvert.Convert(mode.dmPaperLength, PrinterUnit.TenthsOfAMillimeter, PrinterUnit.Display)); } private PaperSource PaperSourceFromMode(SafeNativeMethods.DEVMODE mode) { PaperSource[] sources = printerSettings.Get_PaperSources(); if ((mode.dmFields & SafeNativeMethods.DM_DEFAULTSOURCE) == SafeNativeMethods.DM_DEFAULTSOURCE) { for (int i = 0; i < sources.Length; i++) { // the dmDefaultSource == to the RawKind in the Papersource.. and Not the Kind... // if the PaperSource is populated with CUSTOM values... if ((short)sources[i].RawKind == mode.dmDefaultSource) { return sources[i]; } } } return new PaperSource((PaperSourceKind) mode.dmDefaultSource, "unknown"); } private PrinterResolution PrinterResolutionFromMode(SafeNativeMethods.DEVMODE mode) { PrinterResolution[] resolutions = printerSettings.Get_PrinterResolutions(); for (int i = 0; i < resolutions.Length; i++) { if (mode.dmPrintQuality >= 0 && ((mode.dmFields & SafeNativeMethods.DM_PRINTQUALITY) == SafeNativeMethods.DM_PRINTQUALITY) && ((mode.dmFields & SafeNativeMethods.DM_YRESOLUTION) == SafeNativeMethods.DM_YRESOLUTION)) { if (resolutions[i].X == (int)(PrinterResolutionKind) mode.dmPrintQuality && resolutions[i].Y == (int)(PrinterResolutionKind) mode.dmYResolution) return resolutions[i]; } else { if ((mode.dmFields & SafeNativeMethods.DM_PRINTQUALITY) == SafeNativeMethods.DM_PRINTQUALITY) { if (resolutions[i].Kind == (PrinterResolutionKind) mode.dmPrintQuality) return resolutions[i]; } } } return new PrinterResolution(PrinterResolutionKind.Custom, mode.dmPrintQuality, mode.dmYResolution); } ////// Copies the relevant information out of the PageSettings and into the handle. /// ////// /// public void SetHdevmode(IntPtr hdevmode) { // SECREVIEW: // PrinterSettings.SetHdevmode demand AllPrintingANDUMC so lets be consistent here. IntSecurity.AllPrintingAndUnmanagedCode.Demand(); if (hdevmode == IntPtr.Zero) throw new ArgumentException(SR.GetString(SR.InvalidPrinterHandle, hdevmode)); IntPtr pointer = SafeNativeMethods.GlobalLock(new HandleRef(null, hdevmode)); SafeNativeMethods.DEVMODE mode = (SafeNativeMethods.DEVMODE) UnsafeNativeMethods.PtrToStructure(pointer, typeof(SafeNativeMethods.DEVMODE)); if ((mode.dmFields & SafeNativeMethods.DM_COLOR) == SafeNativeMethods.DM_COLOR) { color = (mode.dmColor == SafeNativeMethods.DMCOLOR_COLOR); } if ((mode.dmFields & SafeNativeMethods.DM_ORIENTATION) == SafeNativeMethods.DM_ORIENTATION) { landscape = (mode.dmOrientation == SafeNativeMethods.DMORIENT_LANDSCAPE); } paperSize = PaperSizeFromMode(mode); paperSource = PaperSourceFromMode(mode); printerResolution = PrinterResolutionFromMode(mode); SafeNativeMethods.GlobalUnlock(new HandleRef(null, hdevmode)); } ////// Copies the relevant information out of the handle and into the PageSettings. /// ////// /// /// public override string ToString() { return "[PageSettings:" + " Color=" + Color.ToString() + ", Landscape=" + Landscape.ToString() + ", Margins=" + Margins.ToString() + ", PaperSize=" + PaperSize.ToString() + ", PaperSource=" + PaperSource.ToString() + ", PrinterResolution=" + PrinterResolution.ToString() + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Provides some interesting information about the PageSettings in /// String form. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Drawing.Internal; ////// /// [Serializable] public class PageSettings : ICloneable { internal PrinterSettings printerSettings; private TriState color = TriState.Default; private PaperSize paperSize; private PaperSource paperSource; private PrinterResolution printerResolution; private TriState landscape = TriState.Default; private Margins margins = new Margins(); ////// Specifies /// settings that apply to a single page. /// ////// /// public PageSettings() : this(new PrinterSettings()) { } ////// Initializes a new instance of the ///class using /// the default printer. /// /// /// public PageSettings(PrinterSettings printerSettings) { Debug.Assert(printerSettings != null, "printerSettings == null"); this.printerSettings = printerSettings; } ///Initializes a new instance of the ///class using /// the specified printer. /// /// public Rectangle Bounds { get { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); IntPtr modeHandle = printerSettings.GetHdevmode(); Rectangle pageBounds = GetBounds(modeHandle); SafeNativeMethods.GlobalFree(new HandleRef(this, modeHandle)); return pageBounds; } } ////// Gets the bounds of the page, taking into account the Landscape property. /// ////// /// public bool Color { get { if (color.IsDefault) return printerSettings.GetModeField(ModeField.Color, SafeNativeMethods.DMCOLOR_MONOCHROME) == SafeNativeMethods.DMCOLOR_COLOR; else return(bool) color; } set { color = value;} } ////// Gets or sets a value indicating whether the page is printed in color. /// ////// /// public float HardMarginX { [SuppressMessage("Microsoft.Security", "CA2106:SecureAsserts")] get { // SECREVIEW: // Its ok to Assert the permission and Let the user know the HardMarginX. // This is consistent with the Bounds property. IntSecurity.AllPrintingAndUnmanagedCode.Assert(); float hardMarginX = 0; DeviceContext dc = printerSettings.CreateDeviceContext(this); try { int dpiX = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.LOGPIXELSX); int hardMarginX_DU = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.PHYSICALOFFSETX); hardMarginX = hardMarginX_DU * 100 / dpiX; } finally { dc.Dispose(); } return hardMarginX; } } ///Returns the x dimension of the hard margin ////// /// public float HardMarginY { get { // SECREVIEW: // Its ok to Assert the permission and Let the user know the HardMarginY. // This is consistent with the Bounds property. float hardMarginY = 0; DeviceContext dc = printerSettings.CreateDeviceContext(this); try { int dpiY = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.LOGPIXELSY); int hardMarginY_DU = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.PHYSICALOFFSETY); hardMarginY = hardMarginY_DU * 100 / dpiY; } finally { dc.Dispose(); } return hardMarginY; } } ///Returns the y dimension of the hard margin ////// /// public bool Landscape { get { if (landscape.IsDefault) return printerSettings.GetModeField(ModeField.Orientation, SafeNativeMethods.DMORIENT_PORTRAIT) == SafeNativeMethods.DMORIENT_LANDSCAPE; else return(bool) landscape; } set { landscape = value;} } ////// Gets or sets a value indicating whether the page should be printed in landscape or portrait orientation. /// ////// /// public Margins Margins { get { return margins;} set { margins = value;} } ////// Gets or sets a value indicating the margins for this page. /// /// ////// /// public PaperSize PaperSize { get { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); return GetPaperSize(IntPtr.Zero); } set { paperSize = value;} } ////// Gets or sets the paper size. /// ////// /// public PaperSource PaperSource { get { if (paperSource == null) { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); IntPtr modeHandle = printerSettings.GetHdevmode(); IntPtr modePointer = SafeNativeMethods.GlobalLock(new HandleRef(this, modeHandle)); SafeNativeMethods.DEVMODE mode = (SafeNativeMethods.DEVMODE) UnsafeNativeMethods.PtrToStructure(modePointer, typeof(SafeNativeMethods.DEVMODE)); PaperSource result = PaperSourceFromMode(mode); SafeNativeMethods.GlobalUnlock(new HandleRef(this, modeHandle)); SafeNativeMethods.GlobalFree(new HandleRef(this, modeHandle)); return result; } else return paperSource; } set { paperSource = value;} } ////// Gets or sets a value indicating the paper source (i.e. upper bin). /// /// ////// /// public RectangleF PrintableArea { get { RectangleF printableArea = new RectangleF(); DeviceContext dc = printerSettings.CreateInformationContext(this); HandleRef hdc = new HandleRef(dc, dc.Hdc); try { int dpiX = UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.LOGPIXELSX); int dpiY = UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.LOGPIXELSY); if (!this.Landscape) { // // Need to convert the printable area to 100th of an inch from the device units printableArea.X = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.PHYSICALOFFSETX) * 100 / dpiX; printableArea.Y = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.PHYSICALOFFSETY) * 100 / dpiY; printableArea.Width = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.HORZRES) * 100 / dpiX; printableArea.Height = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.VERTRES) * 100 / dpiY; } else { // // Need to convert the printable area to 100th of an inch from the device units printableArea.Y = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.PHYSICALOFFSETX) * 100 / dpiX; printableArea.X = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.PHYSICALOFFSETY) * 100 / dpiY; printableArea.Height = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.HORZRES) * 100 / dpiX; printableArea.Width = (float)UnsafeNativeMethods.GetDeviceCaps(hdc, SafeNativeMethods.VERTRES) * 100 / dpiY; } } finally { dc.Dispose(); } return printableArea; } } ////// Gets the PrintableArea for the printer. Units = 100ths of an inch. /// ////// /// public PrinterResolution PrinterResolution { get { if (printerResolution == null) { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); IntPtr modeHandle = printerSettings.GetHdevmode(); IntPtr modePointer = SafeNativeMethods.GlobalLock(new HandleRef(this, modeHandle)); SafeNativeMethods.DEVMODE mode = (SafeNativeMethods.DEVMODE) UnsafeNativeMethods.PtrToStructure(modePointer, typeof(SafeNativeMethods.DEVMODE)); PrinterResolution result = PrinterResolutionFromMode(mode); SafeNativeMethods.GlobalUnlock(new HandleRef(this, modeHandle)); SafeNativeMethods.GlobalFree(new HandleRef(this, modeHandle)); return result; } else return printerResolution; } set { printerResolution = value; } } ////// Gets or sets the printer resolution for the page. /// ////// /// public PrinterSettings PrinterSettings { get { return printerSettings;} set { if (value == null) value = new PrinterSettings(); printerSettings = value; } } ////// Gets or sets the /// associated printer settings. /// ////// /// Copies the settings and margins. /// public object Clone() { PageSettings result = (PageSettings) MemberwiseClone(); result.margins = (Margins) margins.Clone(); return result; } ////// /// public void CopyToHdevmode(IntPtr hdevmode) { IntSecurity.AllPrinting.Demand(); IntPtr modePointer = SafeNativeMethods.GlobalLock(new HandleRef(null, hdevmode)); SafeNativeMethods.DEVMODE mode = (SafeNativeMethods.DEVMODE) UnsafeNativeMethods.PtrToStructure(modePointer, typeof(SafeNativeMethods.DEVMODE)); if (color.IsNotDefault && ((mode.dmFields & SafeNativeMethods.DM_COLOR) == SafeNativeMethods.DM_COLOR)) mode.dmColor = (short) (((bool) color) ? SafeNativeMethods.DMCOLOR_COLOR : SafeNativeMethods.DMCOLOR_MONOCHROME); if (landscape.IsNotDefault && ((mode.dmFields & SafeNativeMethods.DM_ORIENTATION) == SafeNativeMethods.DM_ORIENTATION)) mode.dmOrientation = (short) (((bool) landscape) ? SafeNativeMethods.DMORIENT_LANDSCAPE : SafeNativeMethods.DMORIENT_PORTRAIT); if (paperSize != null) { if ((mode.dmFields & SafeNativeMethods.DM_PAPERSIZE) == SafeNativeMethods.DM_PAPERSIZE) { mode.dmPaperSize = (short) paperSize.RawKind; } bool setWidth = false; bool setLength = false; if ((mode.dmFields & SafeNativeMethods.DM_PAPERLENGTH) == SafeNativeMethods.DM_PAPERLENGTH) { // dmPaperLength is always in tenths of millimeter but paperSizes are in hundredth of inch .. // so we need to convert :: use PrinterUnitConvert.Convert(value, PrinterUnit.TenthsOfAMillimeter /*fromUnit*/, PrinterUnit.Display /*ToUnit*/) mode.dmPaperLength = (short)PrinterUnitConvert.Convert(paperSize.Height, PrinterUnit.Display, PrinterUnit.TenthsOfAMillimeter); setLength = true; } if ((mode.dmFields & SafeNativeMethods.DM_PAPERWIDTH) == SafeNativeMethods.DM_PAPERWIDTH) { mode.dmPaperWidth = (short)PrinterUnitConvert.Convert(paperSize.Width, PrinterUnit.Display, PrinterUnit.TenthsOfAMillimeter); setWidth = true; } if (paperSize.Kind == PaperKind.Custom) { if (!setLength) { mode.dmFields |= SafeNativeMethods.DM_PAPERLENGTH; mode.dmPaperLength = (short)PrinterUnitConvert.Convert(paperSize.Height, PrinterUnit.Display, PrinterUnit.TenthsOfAMillimeter); } if (!setWidth) { mode.dmFields |= SafeNativeMethods.DM_PAPERWIDTH; mode.dmPaperWidth = (short)PrinterUnitConvert.Convert(paperSize.Width, PrinterUnit.Display, PrinterUnit.TenthsOfAMillimeter); } } } if (paperSource != null && ((mode.dmFields & SafeNativeMethods.DM_DEFAULTSOURCE) == SafeNativeMethods.DM_DEFAULTSOURCE)) { mode.dmDefaultSource = (short) paperSource.RawKind; } if (printerResolution != null) { if (printerResolution.Kind == PrinterResolutionKind.Custom) { if ((mode.dmFields & SafeNativeMethods.DM_PRINTQUALITY) == SafeNativeMethods.DM_PRINTQUALITY) { mode.dmPrintQuality = (short) printerResolution.X; } if ((mode.dmFields & SafeNativeMethods.DM_YRESOLUTION) == SafeNativeMethods.DM_YRESOLUTION) { mode.dmYResolution = (short) printerResolution.Y; } } else { if ((mode.dmFields & SafeNativeMethods.DM_PRINTQUALITY) == SafeNativeMethods.DM_PRINTQUALITY) { mode.dmPrintQuality = (short) printerResolution.Kind; } } } Marshal.StructureToPtr(mode, modePointer, false); int retCode = SafeNativeMethods.DocumentProperties(NativeMethods.NullHandleRef, NativeMethods.NullHandleRef, printerSettings.PrinterName, modePointer, modePointer, SafeNativeMethods.DM_IN_BUFFER | SafeNativeMethods.DM_OUT_BUFFER); if (retCode < 0) { SafeNativeMethods.GlobalFree(new HandleRef(null, modePointer)); } SafeNativeMethods.GlobalUnlock(new HandleRef(null, hdevmode)); } // This function shows up big on profiles, so we need to make it fast internal Rectangle GetBounds(IntPtr modeHandle) { Rectangle pageBounds; PaperSize size = GetPaperSize(modeHandle); if (GetLandscape(modeHandle)) pageBounds = new Rectangle(0, 0, size.Height, size.Width); else pageBounds = new Rectangle(0, 0, size.Width, size.Height); return pageBounds; } private bool GetLandscape(IntPtr modeHandle) { if (landscape.IsDefault) return printerSettings.GetModeField(ModeField.Orientation, SafeNativeMethods.DMORIENT_PORTRAIT, modeHandle) == SafeNativeMethods.DMORIENT_LANDSCAPE; else return(bool) landscape; } private PaperSize GetPaperSize(IntPtr modeHandle) { if (paperSize == null) { bool ownHandle = false; if (modeHandle == IntPtr.Zero) { modeHandle = printerSettings.GetHdevmode(); ownHandle = true; } IntPtr modePointer = SafeNativeMethods.GlobalLock(new HandleRef(null, modeHandle)); SafeNativeMethods.DEVMODE mode = (SafeNativeMethods.DEVMODE) UnsafeNativeMethods.PtrToStructure(modePointer, typeof(SafeNativeMethods.DEVMODE)); PaperSize result = PaperSizeFromMode(mode); SafeNativeMethods.GlobalUnlock(new HandleRef(null, modeHandle)); if (ownHandle) SafeNativeMethods.GlobalFree(new HandleRef(null, modeHandle)); return result; } else return paperSize; } private PaperSize PaperSizeFromMode(SafeNativeMethods.DEVMODE mode) { PaperSize[] sizes = printerSettings.Get_PaperSizes(); if ((mode.dmFields & SafeNativeMethods.DM_PAPERSIZE) == SafeNativeMethods.DM_PAPERSIZE) { for (int i = 0; i < sizes.Length; i++) { if ((int)sizes[i].RawKind == mode.dmPaperSize) return sizes[i]; } } return new PaperSize(PaperKind.Custom, "custom", //mode.dmPaperWidth, mode.dmPaperLength); PrinterUnitConvert.Convert(mode.dmPaperWidth, PrinterUnit.TenthsOfAMillimeter, PrinterUnit.Display), PrinterUnitConvert.Convert(mode.dmPaperLength, PrinterUnit.TenthsOfAMillimeter, PrinterUnit.Display)); } private PaperSource PaperSourceFromMode(SafeNativeMethods.DEVMODE mode) { PaperSource[] sources = printerSettings.Get_PaperSources(); if ((mode.dmFields & SafeNativeMethods.DM_DEFAULTSOURCE) == SafeNativeMethods.DM_DEFAULTSOURCE) { for (int i = 0; i < sources.Length; i++) { // the dmDefaultSource == to the RawKind in the Papersource.. and Not the Kind... // if the PaperSource is populated with CUSTOM values... if ((short)sources[i].RawKind == mode.dmDefaultSource) { return sources[i]; } } } return new PaperSource((PaperSourceKind) mode.dmDefaultSource, "unknown"); } private PrinterResolution PrinterResolutionFromMode(SafeNativeMethods.DEVMODE mode) { PrinterResolution[] resolutions = printerSettings.Get_PrinterResolutions(); for (int i = 0; i < resolutions.Length; i++) { if (mode.dmPrintQuality >= 0 && ((mode.dmFields & SafeNativeMethods.DM_PRINTQUALITY) == SafeNativeMethods.DM_PRINTQUALITY) && ((mode.dmFields & SafeNativeMethods.DM_YRESOLUTION) == SafeNativeMethods.DM_YRESOLUTION)) { if (resolutions[i].X == (int)(PrinterResolutionKind) mode.dmPrintQuality && resolutions[i].Y == (int)(PrinterResolutionKind) mode.dmYResolution) return resolutions[i]; } else { if ((mode.dmFields & SafeNativeMethods.DM_PRINTQUALITY) == SafeNativeMethods.DM_PRINTQUALITY) { if (resolutions[i].Kind == (PrinterResolutionKind) mode.dmPrintQuality) return resolutions[i]; } } } return new PrinterResolution(PrinterResolutionKind.Custom, mode.dmPrintQuality, mode.dmYResolution); } ////// Copies the relevant information out of the PageSettings and into the handle. /// ////// /// public void SetHdevmode(IntPtr hdevmode) { // SECREVIEW: // PrinterSettings.SetHdevmode demand AllPrintingANDUMC so lets be consistent here. IntSecurity.AllPrintingAndUnmanagedCode.Demand(); if (hdevmode == IntPtr.Zero) throw new ArgumentException(SR.GetString(SR.InvalidPrinterHandle, hdevmode)); IntPtr pointer = SafeNativeMethods.GlobalLock(new HandleRef(null, hdevmode)); SafeNativeMethods.DEVMODE mode = (SafeNativeMethods.DEVMODE) UnsafeNativeMethods.PtrToStructure(pointer, typeof(SafeNativeMethods.DEVMODE)); if ((mode.dmFields & SafeNativeMethods.DM_COLOR) == SafeNativeMethods.DM_COLOR) { color = (mode.dmColor == SafeNativeMethods.DMCOLOR_COLOR); } if ((mode.dmFields & SafeNativeMethods.DM_ORIENTATION) == SafeNativeMethods.DM_ORIENTATION) { landscape = (mode.dmOrientation == SafeNativeMethods.DMORIENT_LANDSCAPE); } paperSize = PaperSizeFromMode(mode); paperSource = PaperSourceFromMode(mode); printerResolution = PrinterResolutionFromMode(mode); SafeNativeMethods.GlobalUnlock(new HandleRef(null, hdevmode)); } ////// Copies the relevant information out of the handle and into the PageSettings. /// ////// /// /// public override string ToString() { return "[PageSettings:" + " Color=" + Color.ToString() + ", Landscape=" + Landscape.ToString() + ", Margins=" + Margins.ToString() + ", PaperSize=" + PaperSize.ToString() + ", PaperSource=" + PaperSource.ToString() + ", PrinterResolution=" + PrinterResolution.ToString() + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Provides some interesting information about the PageSettings in /// String form. /// ///
Link Menu
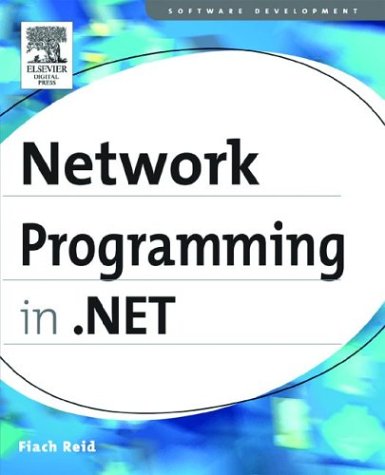
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsSolidBrush.cs
- XmlSchemaSimpleContent.cs
- ContentDefinition.cs
- GridProviderWrapper.cs
- FlowLayout.cs
- XmlQualifiedName.cs
- ScrollBar.cs
- CodeArrayIndexerExpression.cs
- XmlSchemaAnnotated.cs
- RawStylusInputCustomData.cs
- ColorMap.cs
- XmlNamespaceDeclarationsAttribute.cs
- DataGridColumnHeaderAutomationPeer.cs
- Encoder.cs
- HyperLinkStyle.cs
- TraceUtility.cs
- GeneralTransform.cs
- SortDescription.cs
- ValidationError.cs
- Literal.cs
- XPathDocumentIterator.cs
- ArgumentException.cs
- CacheMemory.cs
- NetNamedPipeBindingCollectionElement.cs
- PKCS1MaskGenerationMethod.cs
- Listbox.cs
- DefaultParameterValueAttribute.cs
- WebPartUserCapability.cs
- PointHitTestResult.cs
- BuildResult.cs
- ListBindingHelper.cs
- Utils.cs
- NonSerializedAttribute.cs
- Flowchart.cs
- PreProcessor.cs
- ItemAutomationPeer.cs
- TreeNodeCollection.cs
- DSASignatureDeformatter.cs
- LinkedList.cs
- DataContractSet.cs
- ProfileServiceManager.cs
- CodeDomDesignerLoader.cs
- SelectionEditingBehavior.cs
- CommandID.cs
- FocusChangedEventArgs.cs
- CompiledQuery.cs
- CreateUserErrorEventArgs.cs
- UserNameSecurityTokenProvider.cs
- WindowClosedEventArgs.cs
- basecomparevalidator.cs
- ImageBrush.cs
- SqlUtil.cs
- ObjectHandle.cs
- TemplateControlCodeDomTreeGenerator.cs
- DataConnectionHelper.cs
- CodeConditionStatement.cs
- Attributes.cs
- FrameSecurityDescriptor.cs
- XmlAttributes.cs
- XmlStreamStore.cs
- DataGridTable.cs
- Enum.cs
- SqlClientMetaDataCollectionNames.cs
- InternalConfigSettingsFactory.cs
- PackUriHelper.cs
- httpserverutility.cs
- ValidateNames.cs
- ConstraintStruct.cs
- SoapServerProtocol.cs
- RawKeyboardInputReport.cs
- ContextDataSourceContextData.cs
- DesignTimeVisibleAttribute.cs
- RealProxy.cs
- ContentControl.cs
- DataObjectMethodAttribute.cs
- HostnameComparisonMode.cs
- DoubleKeyFrameCollection.cs
- WebConfigurationHost.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- InternalsVisibleToAttribute.cs
- Workspace.cs
- _ListenerResponseStream.cs
- PasswordTextContainer.cs
- MouseOverProperty.cs
- RightsManagementResourceHelper.cs
- DesignTimeDataBinding.cs
- ToolStripSplitButton.cs
- CharConverter.cs
- CheckBoxFlatAdapter.cs
- DefaultSection.cs
- EventLogTraceListener.cs
- StringValidatorAttribute.cs
- wmiprovider.cs
- ContainerParaClient.cs
- SqlWebEventProvider.cs
- PersonalizationEntry.cs
- KerberosSecurityTokenAuthenticator.cs
- DataKeyCollection.cs
- BitmapVisualManager.cs
- TransportConfigurationTypeElement.cs