Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / ObjectPersistData.cs / 1 / ObjectPersistData.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Collections; using System.Collections.Specialized; using System.Web.Util; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ObjectPersistData { private Type _objectType; private bool _isCollection; private ArrayList _collectionItems; private bool _localize; private string _resourceKey; private IDictionary _propertyTableByFilter; private IDictionary _propertyTableByProperty; private ArrayList _allPropertyEntries; private ArrayList _eventEntries; private IDictionary _builtObjects; public ObjectPersistData(ControlBuilder builder, IDictionary builtObjects) { _objectType = builder.ControlType; _localize = builder.Localize; _resourceKey = builder.GetResourceKey(); _builtObjects = builtObjects; if (typeof(ICollection).IsAssignableFrom(_objectType)) { _isCollection = true; } _collectionItems = new ArrayList(); _propertyTableByFilter = new HybridDictionary(true); _propertyTableByProperty = new HybridDictionary(true); _allPropertyEntries = new ArrayList(); _eventEntries = new ArrayList(); foreach (PropertyEntry entry in builder.SimplePropertyEntries) { AddPropertyEntry(entry); } foreach (PropertyEntry entry in builder.ComplexPropertyEntries) { AddPropertyEntry(entry); } foreach (PropertyEntry entry in builder.TemplatePropertyEntries) { AddPropertyEntry(entry); } foreach (PropertyEntry entry in builder.BoundPropertyEntries) { AddPropertyEntry(entry); } foreach (EventEntry entry in builder.EventEntries) { AddEventEntry(entry); } } ////// Get all property entries /// public ICollection AllPropertyEntries { get { return _allPropertyEntries; } } public IDictionary BuiltObjects { get { return _builtObjects; } } public ICollection CollectionItems { get { return _collectionItems; } } public ICollection EventEntries { get { return _eventEntries; } } ////// True if this persistence data is for a collection /// public bool IsCollection { get { return _isCollection; } } public bool Localize { get { return _localize; } } ////// The type of the object with these properties. /// public Type ObjectType { get { return _objectType; } } public string ResourceKey { get { return _resourceKey; } } ////// Adds a property to this persistence data, adding it to all necessary /// data structures. /// private void AddPropertyEntry(PropertyEntry entry) { if (_isCollection && (entry is ComplexPropertyEntry && ((ComplexPropertyEntry)entry).IsCollectionItem)) { _collectionItems.Add(entry); } else { IDictionary filteredProperties = (IDictionary)_propertyTableByFilter[entry.Filter]; if (filteredProperties == null) { filteredProperties = new HybridDictionary(true); _propertyTableByFilter[entry.Filter] = filteredProperties; } Debug.Assert((entry.Name != null) && (entry.Name.Length > 0)); filteredProperties[entry.Name] = entry; ArrayList properties = (ArrayList)_propertyTableByProperty[entry.Name]; if (properties == null) { properties = new ArrayList(); _propertyTableByProperty[entry.Name] = properties; } properties.Add(entry); } _allPropertyEntries.Add(entry); } private void AddEventEntry(EventEntry entry) { _eventEntries.Add(entry); } ////// public void AddToObjectControlBuilderTable(IDictionary table) { if (_builtObjects != null) { foreach (DictionaryEntry entry in _builtObjects) { table[entry.Key] = entry.Value; } } } /// /// Gets a PropertyEntry for the specified filter and property name /// public PropertyEntry GetFilteredProperty(string filter, string name) { IDictionary filteredProperties = GetFilteredProperties(filter); if (filteredProperties != null) { return (PropertyEntry)filteredProperties[name]; } return null; } ////// Gets all PropertyEntries for the specified filter /// public IDictionary GetFilteredProperties(string filter) { return (IDictionary)_propertyTableByFilter[filter]; } ////// Gets all filtered PropertiesEntries for a specified property (name uses dot-syntax e.g. Font.Bold) /// public ICollection GetPropertyAllFilters(string name) { ICollection properties = (ICollection)_propertyTableByProperty[name]; if (properties == null) { return new ArrayList(); } return properties; } } }
Link Menu
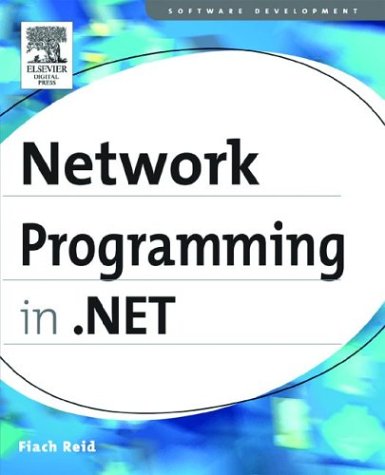
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegexCompilationInfo.cs
- ControlUtil.cs
- TraceInternal.cs
- CurrentChangingEventArgs.cs
- RectKeyFrameCollection.cs
- UTF8Encoding.cs
- IdentityValidationException.cs
- WebPartManager.cs
- WebBaseEventKeyComparer.cs
- ProxyWebPart.cs
- arc.cs
- CallSiteOps.cs
- NotImplementedException.cs
- NavigationHelper.cs
- BaseCodePageEncoding.cs
- PropertyGridView.cs
- CodeIdentifiers.cs
- ResourceWriter.cs
- ThreadPool.cs
- Misc.cs
- MessageSecurityOverTcp.cs
- XmlAttributeAttribute.cs
- ImageAttributes.cs
- StackSpiller.Generated.cs
- SaveFileDialog.cs
- CodeValidator.cs
- InfoCardUIAgent.cs
- TouchPoint.cs
- Vector3DCollection.cs
- KeysConverter.cs
- CheckedPointers.cs
- Subtree.cs
- ExtendedPropertyCollection.cs
- StylusDevice.cs
- HwndAppCommandInputProvider.cs
- Size.cs
- AppDomainManager.cs
- FrameworkTextComposition.cs
- SelectorItemAutomationPeer.cs
- RuleValidation.cs
- FontResourceCache.cs
- CustomTrackingRecord.cs
- FlowLayoutPanel.cs
- QueryOperator.cs
- ProxyFragment.cs
- WebScriptMetadataInstanceContextProvider.cs
- ProcessHostMapPath.cs
- EmbeddedMailObjectsCollection.cs
- Rectangle.cs
- PeerApplicationLaunchInfo.cs
- XmlILModule.cs
- ObjectDataSourceEventArgs.cs
- RectAnimationClockResource.cs
- WebBrowserDocumentCompletedEventHandler.cs
- SqlCrossApplyToCrossJoin.cs
- CompositeCollection.cs
- SafeNativeMethodsCLR.cs
- EventMappingSettings.cs
- TextSearch.cs
- StringUtil.cs
- TypeToken.cs
- HtmlTableRowCollection.cs
- EntityClassGenerator.cs
- MDIWindowDialog.cs
- EnumBuilder.cs
- MgmtConfigurationRecord.cs
- Model3D.cs
- TextElementEditingBehaviorAttribute.cs
- SystemInfo.cs
- SchemaExporter.cs
- BlockCollection.cs
- xmlfixedPageInfo.cs
- ProbeMatchesApril2005.cs
- WebBrowserBase.cs
- StaticTextPointer.cs
- ProfileManager.cs
- XmlEnumAttribute.cs
- recordstatescratchpad.cs
- UrlMappingsSection.cs
- MeasureItemEvent.cs
- ArgumentValueSerializer.cs
- BufferManager.cs
- JsonFormatWriterGenerator.cs
- ChannelServices.cs
- WebZone.cs
- Keyboard.cs
- CheckBoxField.cs
- ToggleButton.cs
- ConstraintCollection.cs
- XsltException.cs
- MaskedTextBoxTextEditorDropDown.cs
- EncoderFallback.cs
- EncodingNLS.cs
- IdentitySection.cs
- GifBitmapEncoder.cs
- CriticalFinalizerObject.cs
- GPStream.cs
- WebPartEditorCancelVerb.cs
- SystemColors.cs
- StylusPointProperty.cs