Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / Clipboard.cs / 1 / Clipboard.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.IO; using System.Runtime.InteropServices; using System.Runtime.InteropServices.ComTypes; using System.Security; using IComDataObject = System.Runtime.InteropServices.ComTypes.IDataObject; using System.Globalization; using System.Security.Permissions; using System.Collections; ////// /// public sealed class Clipboard { // not creatable... // private Clipboard() { } // // Checks the validity of format while setting data into ClipBoard // private static bool IsFormatValid(DataObject data) { string[] formats = data.GetFormats(); Debug.Assert(formats != null, "Null returned from GetFormats"); if (formats != null) { if (formats.Length <= 4) { for (int i=0; iProvides methods to place data on and retrieve data from the system clipboard. This class cannot be inherited. ////// /// public static void SetDataObject(object data) { SetDataObject(data, false); } ///Places nonpersistent data on the system ///. /// /// public static void SetDataObject(object data, bool copy) { SetDataObject(data, copy, 10 /*retryTimes*/, 100 /*retryDelay*/); } ///Overload that uses default values for retryTimes and retryDelay. ////// /// [UIPermission(SecurityAction.Demand, Clipboard=UIPermissionClipboard.OwnClipboard)] public static void SetDataObject(object data, bool copy, int retryTimes, int retryDelay) { if (Application.OleRequired() != System.Threading.ApartmentState.STA) { throw new System.Threading.ThreadStateException(SR.GetString(SR.ThreadMustBeSTA)); } if (data == null) { throw new ArgumentNullException("data"); } if (retryTimes < 0) { throw new ArgumentOutOfRangeException("retryTimes", SR.GetString(SR.InvalidLowBoundArgumentEx, "retryTimes", retryTimes.ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } if (retryDelay < 0) { throw new ArgumentOutOfRangeException("retryDelay", SR.GetString(SR.InvalidLowBoundArgumentEx, "retryDelay", retryDelay.ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } DataObject dataObject = null; if (!(data is IComDataObject)) { dataObject = new DataObject(data); } bool restrictedFormats = false; // If the caller doesnt have AllClipBoard permission then we allow only restricted formats. // These formats are TEXT, UNICODETEXT,String and CSV try { IntSecurity.ClipboardRead.Demand(); } catch (SecurityException) { // We dont have allClipBoard so we can set only data in the following formats // TEXT, UNICODETEXT, and CSV. restrictedFormats = true; } // Compute the format of the "data" passed in iff setText == true; if (restrictedFormats) { if (dataObject == null) { dataObject = data as DataObject; } if (!IsFormatValid(dataObject)) { throw new SecurityException(SR.GetString(SR.ClipboardSecurityException)); } } int hr, retry = retryTimes; IntSecurity.UnmanagedCode.Assert(); try { do { if (data is IComDataObject) { hr = UnsafeNativeMethods.OleSetClipboard((IComDataObject)data); } else { hr = UnsafeNativeMethods.OleSetClipboard(dataObject); } if (hr != 0) { if (retry == 0) { ThrowIfFailed(hr); } retry--; System.Threading.Thread.Sleep(retryDelay /*ms*/); } } while (hr != 0); if (copy) { retry = retryTimes; do { hr = UnsafeNativeMethods.OleFlushClipboard(); if (hr != 0) { if (retry == 0) { ThrowIfFailed(hr); } retry--; System.Threading.Thread.Sleep(retryDelay /*ms*/); } } while (hr != 0); } } finally { CodeAccessPermission.RevertAssert(); } } ///Places data on the system ///and uses copy to specify whether the data /// should remain on the /// after the application exits. /// /// public static IDataObject GetDataObject() { Debug.WriteLineIf(IntSecurity.SecurityDemand.TraceVerbose, "ClipboardRead Demanded"); IntSecurity.ClipboardRead.Demand(); if (Application.OleRequired() != System.Threading.ApartmentState.STA) { // only throw if a message loop was started. This makes the case of trying // to query the clipboard from your finalizer or non-ui MTA thread // silently fail, instead of making your app die. // // however, if you are trying to write a normal windows forms app and // forget to set the STAThread attribute, we will correctly report // an error to aid in debugging. // if (Application.MessageLoop) { throw new System.Threading.ThreadStateException(SR.GetString(SR.ThreadMustBeSTA)); } else { return null; } } // Vswhidbey :: 476911. We need to retry the GetDataObject() since the clipBaord is busy sometimes and hence the GetDataObject would fail with ClipBoardException. return GetDataObject(10 /*retryTimes*/, 100 /*retryDelay*/); } // Private method to help accessing clipBoard for know retries before failing. private static IDataObject GetDataObject(int retryTimes, int retryDelay) { IComDataObject dataObject = null; int hr, retry = retryTimes; do { hr = UnsafeNativeMethods.OleGetClipboard(ref dataObject); if (hr != 0) { if (retry == 0) { ThrowIfFailed(hr); } retry--; System.Threading.Thread.Sleep(retryDelay /*ms*/); } } while (hr != 0); if (dataObject != null) { if (dataObject is IDataObject && !Marshal.IsComObject(dataObject)) { return (IDataObject)dataObject; } else { return new DataObject(dataObject); } } return null; } // <-- WHIDBEY ADDITIONS ///Retrieves the data that is currently on the system /// ///. /// /// public static void Clear() { Clipboard.SetDataObject(new DataObject()); } ///[To be supplied.] ////// /// public static bool ContainsAudio() { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetDataPresent(DataFormats.WaveAudio, false); } return false; } ///[To be supplied.] ////// /// public static bool ContainsData(string format) { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetDataPresent(format, false); } return false; } ///[To be supplied.] ////// /// public static bool ContainsFileDropList() { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetDataPresent(DataFormats.FileDrop, true); } return false; } ///[To be supplied.] ////// /// public static bool ContainsImage() { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetDataPresent(DataFormats.Bitmap, true); } return false; } ///[To be supplied.] ////// /// public static bool ContainsText() { if (Environment.OSVersion.Platform != System.PlatformID.Win32NT || Environment.OSVersion.Version.Major < 5) { return ContainsText(TextDataFormat.Text); } else { return ContainsText(TextDataFormat.UnicodeText); } } ///[To be supplied.] ////// /// public static bool ContainsText(TextDataFormat format) { // valid values are 0x0-0x4 inclusive if (!ClientUtils.IsEnumValid(format, (int)format, (int)TextDataFormat.Text, (int)TextDataFormat.CommaSeparatedValue)){ throw new InvalidEnumArgumentException("format", (int)format, typeof(TextDataFormat)); } IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetDataPresent(ConvertToDataFormats(format), false); } return false; } ///[To be supplied.] ////// /// public static Stream GetAudioStream() { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetData(DataFormats.WaveAudio, false) as Stream; } return null; } ///[To be supplied.] ////// /// public static object GetData(string format) { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetData(format); } return null; } ///[To be supplied.] ////// /// public static StringCollection GetFileDropList() { IDataObject dataObject = Clipboard.GetDataObject(); StringCollection retVal = new StringCollection(); if (dataObject != null) { string[] strings = dataObject.GetData(DataFormats.FileDrop, true) as string[]; if (strings != null) { retVal.AddRange(strings); } } return retVal; } ///[To be supplied.] ////// /// public static Image GetImage() { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetData(DataFormats.Bitmap, true) as Image; } return null; } ///[To be supplied.] ////// /// public static string GetText() { // Pass in Text format for Win98... if (Environment.OSVersion.Platform != System.PlatformID.Win32NT || Environment.OSVersion.Version.Major < 5) { return GetText(TextDataFormat.Text); } else { return GetText(TextDataFormat.UnicodeText); } } ///[To be supplied.] ////// /// public static string GetText(TextDataFormat format) { // valid values are 0x0 to 0x4 inclusive if (!ClientUtils.IsEnumValid(format, (int)format, (int)TextDataFormat.Text, (int)TextDataFormat.CommaSeparatedValue)) { throw new InvalidEnumArgumentException("format", (int)format, typeof(TextDataFormat)); } IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { string text = dataObject.GetData(ConvertToDataFormats(format), false) as string; if (text != null) { return text; } } return String.Empty; } ///[To be supplied.] ////// /// public static void SetAudio(byte[] audioBytes) { if (audioBytes == null) { throw new ArgumentNullException("audioBytes"); } SetAudio(new MemoryStream(audioBytes)); } ///[To be supplied.] ////// /// public static void SetAudio(Stream audioStream) { if (audioStream == null) { throw new ArgumentNullException("audioStream"); } IDataObject dataObject = new DataObject(); dataObject.SetData(DataFormats.WaveAudio, false, audioStream); Clipboard.SetDataObject(dataObject, true); } ///[To be supplied.] ////// /// public static void SetData(string format, object data) { //Note: We delegate argument checking to IDataObject.SetData, if it wants to do so. IDataObject dataObject = new DataObject(); dataObject.SetData(format, data); Clipboard.SetDataObject(dataObject, true); } ///[To be supplied.] ////// /// public static void SetFileDropList(StringCollection filePaths) { if (filePaths == null) { throw new ArgumentNullException("filePaths"); } // VsWhidbey:432618 throw Argument exception for zero-length filepath collection. if (filePaths.Count == 0) { throw new ArgumentException(SR.GetString(SR.CollectionEmptyException)); } //VSWhidbey #163538 - Validate the paths to make sure they don't contain invalid characters foreach (string path in filePaths) { try { string temp = Path.GetFullPath(path); } catch (Exception e) { if (ClientUtils.IsSecurityOrCriticalException(e)) { throw; } throw new ArgumentException(SR.GetString(SR.Clipboard_InvalidPath, path, "filePaths"), e); } } if (filePaths.Count > 0) { IDataObject dataObject = new DataObject(); string[] strings = new string[filePaths.Count]; filePaths.CopyTo(strings, 0); dataObject.SetData(DataFormats.FileDrop, true, strings); Clipboard.SetDataObject(dataObject, true); } } ///[To be supplied.] ////// /// public static void SetImage(Image image) { if (image == null) { throw new ArgumentNullException("image"); } IDataObject dataObject = new DataObject(); dataObject.SetData(DataFormats.Bitmap, true, image); Clipboard.SetDataObject(dataObject, true); } ///[To be supplied.] ////// /// public static void SetText(string text) { // Pass in Text format for Win98... if (Environment.OSVersion.Platform != System.PlatformID.Win32NT || Environment.OSVersion.Version.Major < 5) { SetText(text, TextDataFormat.Text); } else { SetText(text, TextDataFormat.UnicodeText); } } ///[To be supplied.] ////// /// public static void SetText(string text, TextDataFormat format) { if (String.IsNullOrEmpty(text)) { throw new ArgumentNullException("text"); } //valid values are 0x0 to 0x4 if (!ClientUtils.IsEnumValid(format, (int)format, (int)TextDataFormat.Text, (int)TextDataFormat.CommaSeparatedValue)) { throw new InvalidEnumArgumentException("format", (int)format, typeof(TextDataFormat)); } IDataObject dataObject = new DataObject(); dataObject.SetData(ConvertToDataFormats(format), false, text); Clipboard.SetDataObject(dataObject, true); } private static string ConvertToDataFormats(TextDataFormat format) { switch (format) { case TextDataFormat.Text: return DataFormats.Text; case TextDataFormat.UnicodeText: return DataFormats.UnicodeText; case TextDataFormat.Rtf: return DataFormats.Rtf; case TextDataFormat.Html: return DataFormats.Html; case TextDataFormat.CommaSeparatedValue: return DataFormats.CommaSeparatedValue; } return DataFormats.UnicodeText; } // END - WHIDBEY ADDITIONS --> private static void ThrowIfFailed(int hr) { // if (hr != 0) { ExternalException e = new ExternalException(SR.GetString(SR.ClipboardOperationFailed), hr); throw e; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.IO; using System.Runtime.InteropServices; using System.Runtime.InteropServices.ComTypes; using System.Security; using IComDataObject = System.Runtime.InteropServices.ComTypes.IDataObject; using System.Globalization; using System.Security.Permissions; using System.Collections; ////// /// public sealed class Clipboard { // not creatable... // private Clipboard() { } // // Checks the validity of format while setting data into ClipBoard // private static bool IsFormatValid(DataObject data) { string[] formats = data.GetFormats(); Debug.Assert(formats != null, "Null returned from GetFormats"); if (formats != null) { if (formats.Length <= 4) { for (int i=0; iProvides methods to place data on and retrieve data from the system clipboard. This class cannot be inherited. ////// /// public static void SetDataObject(object data) { SetDataObject(data, false); } ///Places nonpersistent data on the system ///. /// /// public static void SetDataObject(object data, bool copy) { SetDataObject(data, copy, 10 /*retryTimes*/, 100 /*retryDelay*/); } ///Overload that uses default values for retryTimes and retryDelay. ////// /// [UIPermission(SecurityAction.Demand, Clipboard=UIPermissionClipboard.OwnClipboard)] public static void SetDataObject(object data, bool copy, int retryTimes, int retryDelay) { if (Application.OleRequired() != System.Threading.ApartmentState.STA) { throw new System.Threading.ThreadStateException(SR.GetString(SR.ThreadMustBeSTA)); } if (data == null) { throw new ArgumentNullException("data"); } if (retryTimes < 0) { throw new ArgumentOutOfRangeException("retryTimes", SR.GetString(SR.InvalidLowBoundArgumentEx, "retryTimes", retryTimes.ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } if (retryDelay < 0) { throw new ArgumentOutOfRangeException("retryDelay", SR.GetString(SR.InvalidLowBoundArgumentEx, "retryDelay", retryDelay.ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } DataObject dataObject = null; if (!(data is IComDataObject)) { dataObject = new DataObject(data); } bool restrictedFormats = false; // If the caller doesnt have AllClipBoard permission then we allow only restricted formats. // These formats are TEXT, UNICODETEXT,String and CSV try { IntSecurity.ClipboardRead.Demand(); } catch (SecurityException) { // We dont have allClipBoard so we can set only data in the following formats // TEXT, UNICODETEXT, and CSV. restrictedFormats = true; } // Compute the format of the "data" passed in iff setText == true; if (restrictedFormats) { if (dataObject == null) { dataObject = data as DataObject; } if (!IsFormatValid(dataObject)) { throw new SecurityException(SR.GetString(SR.ClipboardSecurityException)); } } int hr, retry = retryTimes; IntSecurity.UnmanagedCode.Assert(); try { do { if (data is IComDataObject) { hr = UnsafeNativeMethods.OleSetClipboard((IComDataObject)data); } else { hr = UnsafeNativeMethods.OleSetClipboard(dataObject); } if (hr != 0) { if (retry == 0) { ThrowIfFailed(hr); } retry--; System.Threading.Thread.Sleep(retryDelay /*ms*/); } } while (hr != 0); if (copy) { retry = retryTimes; do { hr = UnsafeNativeMethods.OleFlushClipboard(); if (hr != 0) { if (retry == 0) { ThrowIfFailed(hr); } retry--; System.Threading.Thread.Sleep(retryDelay /*ms*/); } } while (hr != 0); } } finally { CodeAccessPermission.RevertAssert(); } } ///Places data on the system ///and uses copy to specify whether the data /// should remain on the /// after the application exits. /// /// public static IDataObject GetDataObject() { Debug.WriteLineIf(IntSecurity.SecurityDemand.TraceVerbose, "ClipboardRead Demanded"); IntSecurity.ClipboardRead.Demand(); if (Application.OleRequired() != System.Threading.ApartmentState.STA) { // only throw if a message loop was started. This makes the case of trying // to query the clipboard from your finalizer or non-ui MTA thread // silently fail, instead of making your app die. // // however, if you are trying to write a normal windows forms app and // forget to set the STAThread attribute, we will correctly report // an error to aid in debugging. // if (Application.MessageLoop) { throw new System.Threading.ThreadStateException(SR.GetString(SR.ThreadMustBeSTA)); } else { return null; } } // Vswhidbey :: 476911. We need to retry the GetDataObject() since the clipBaord is busy sometimes and hence the GetDataObject would fail with ClipBoardException. return GetDataObject(10 /*retryTimes*/, 100 /*retryDelay*/); } // Private method to help accessing clipBoard for know retries before failing. private static IDataObject GetDataObject(int retryTimes, int retryDelay) { IComDataObject dataObject = null; int hr, retry = retryTimes; do { hr = UnsafeNativeMethods.OleGetClipboard(ref dataObject); if (hr != 0) { if (retry == 0) { ThrowIfFailed(hr); } retry--; System.Threading.Thread.Sleep(retryDelay /*ms*/); } } while (hr != 0); if (dataObject != null) { if (dataObject is IDataObject && !Marshal.IsComObject(dataObject)) { return (IDataObject)dataObject; } else { return new DataObject(dataObject); } } return null; } // <-- WHIDBEY ADDITIONS ///Retrieves the data that is currently on the system /// ///. /// /// public static void Clear() { Clipboard.SetDataObject(new DataObject()); } ///[To be supplied.] ////// /// public static bool ContainsAudio() { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetDataPresent(DataFormats.WaveAudio, false); } return false; } ///[To be supplied.] ////// /// public static bool ContainsData(string format) { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetDataPresent(format, false); } return false; } ///[To be supplied.] ////// /// public static bool ContainsFileDropList() { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetDataPresent(DataFormats.FileDrop, true); } return false; } ///[To be supplied.] ////// /// public static bool ContainsImage() { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetDataPresent(DataFormats.Bitmap, true); } return false; } ///[To be supplied.] ////// /// public static bool ContainsText() { if (Environment.OSVersion.Platform != System.PlatformID.Win32NT || Environment.OSVersion.Version.Major < 5) { return ContainsText(TextDataFormat.Text); } else { return ContainsText(TextDataFormat.UnicodeText); } } ///[To be supplied.] ////// /// public static bool ContainsText(TextDataFormat format) { // valid values are 0x0-0x4 inclusive if (!ClientUtils.IsEnumValid(format, (int)format, (int)TextDataFormat.Text, (int)TextDataFormat.CommaSeparatedValue)){ throw new InvalidEnumArgumentException("format", (int)format, typeof(TextDataFormat)); } IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetDataPresent(ConvertToDataFormats(format), false); } return false; } ///[To be supplied.] ////// /// public static Stream GetAudioStream() { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetData(DataFormats.WaveAudio, false) as Stream; } return null; } ///[To be supplied.] ////// /// public static object GetData(string format) { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetData(format); } return null; } ///[To be supplied.] ////// /// public static StringCollection GetFileDropList() { IDataObject dataObject = Clipboard.GetDataObject(); StringCollection retVal = new StringCollection(); if (dataObject != null) { string[] strings = dataObject.GetData(DataFormats.FileDrop, true) as string[]; if (strings != null) { retVal.AddRange(strings); } } return retVal; } ///[To be supplied.] ////// /// public static Image GetImage() { IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { return dataObject.GetData(DataFormats.Bitmap, true) as Image; } return null; } ///[To be supplied.] ////// /// public static string GetText() { // Pass in Text format for Win98... if (Environment.OSVersion.Platform != System.PlatformID.Win32NT || Environment.OSVersion.Version.Major < 5) { return GetText(TextDataFormat.Text); } else { return GetText(TextDataFormat.UnicodeText); } } ///[To be supplied.] ////// /// public static string GetText(TextDataFormat format) { // valid values are 0x0 to 0x4 inclusive if (!ClientUtils.IsEnumValid(format, (int)format, (int)TextDataFormat.Text, (int)TextDataFormat.CommaSeparatedValue)) { throw new InvalidEnumArgumentException("format", (int)format, typeof(TextDataFormat)); } IDataObject dataObject = Clipboard.GetDataObject(); if (dataObject != null) { string text = dataObject.GetData(ConvertToDataFormats(format), false) as string; if (text != null) { return text; } } return String.Empty; } ///[To be supplied.] ////// /// public static void SetAudio(byte[] audioBytes) { if (audioBytes == null) { throw new ArgumentNullException("audioBytes"); } SetAudio(new MemoryStream(audioBytes)); } ///[To be supplied.] ////// /// public static void SetAudio(Stream audioStream) { if (audioStream == null) { throw new ArgumentNullException("audioStream"); } IDataObject dataObject = new DataObject(); dataObject.SetData(DataFormats.WaveAudio, false, audioStream); Clipboard.SetDataObject(dataObject, true); } ///[To be supplied.] ////// /// public static void SetData(string format, object data) { //Note: We delegate argument checking to IDataObject.SetData, if it wants to do so. IDataObject dataObject = new DataObject(); dataObject.SetData(format, data); Clipboard.SetDataObject(dataObject, true); } ///[To be supplied.] ////// /// public static void SetFileDropList(StringCollection filePaths) { if (filePaths == null) { throw new ArgumentNullException("filePaths"); } // VsWhidbey:432618 throw Argument exception for zero-length filepath collection. if (filePaths.Count == 0) { throw new ArgumentException(SR.GetString(SR.CollectionEmptyException)); } //VSWhidbey #163538 - Validate the paths to make sure they don't contain invalid characters foreach (string path in filePaths) { try { string temp = Path.GetFullPath(path); } catch (Exception e) { if (ClientUtils.IsSecurityOrCriticalException(e)) { throw; } throw new ArgumentException(SR.GetString(SR.Clipboard_InvalidPath, path, "filePaths"), e); } } if (filePaths.Count > 0) { IDataObject dataObject = new DataObject(); string[] strings = new string[filePaths.Count]; filePaths.CopyTo(strings, 0); dataObject.SetData(DataFormats.FileDrop, true, strings); Clipboard.SetDataObject(dataObject, true); } } ///[To be supplied.] ////// /// public static void SetImage(Image image) { if (image == null) { throw new ArgumentNullException("image"); } IDataObject dataObject = new DataObject(); dataObject.SetData(DataFormats.Bitmap, true, image); Clipboard.SetDataObject(dataObject, true); } ///[To be supplied.] ////// /// public static void SetText(string text) { // Pass in Text format for Win98... if (Environment.OSVersion.Platform != System.PlatformID.Win32NT || Environment.OSVersion.Version.Major < 5) { SetText(text, TextDataFormat.Text); } else { SetText(text, TextDataFormat.UnicodeText); } } ///[To be supplied.] ////// /// public static void SetText(string text, TextDataFormat format) { if (String.IsNullOrEmpty(text)) { throw new ArgumentNullException("text"); } //valid values are 0x0 to 0x4 if (!ClientUtils.IsEnumValid(format, (int)format, (int)TextDataFormat.Text, (int)TextDataFormat.CommaSeparatedValue)) { throw new InvalidEnumArgumentException("format", (int)format, typeof(TextDataFormat)); } IDataObject dataObject = new DataObject(); dataObject.SetData(ConvertToDataFormats(format), false, text); Clipboard.SetDataObject(dataObject, true); } private static string ConvertToDataFormats(TextDataFormat format) { switch (format) { case TextDataFormat.Text: return DataFormats.Text; case TextDataFormat.UnicodeText: return DataFormats.UnicodeText; case TextDataFormat.Rtf: return DataFormats.Rtf; case TextDataFormat.Html: return DataFormats.Html; case TextDataFormat.CommaSeparatedValue: return DataFormats.CommaSeparatedValue; } return DataFormats.UnicodeText; } // END - WHIDBEY ADDITIONS --> private static void ThrowIfFailed(int hr) { // if (hr != 0) { ExternalException e = new ExternalException(SR.GetString(SR.ClipboardOperationFailed), hr); throw e; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
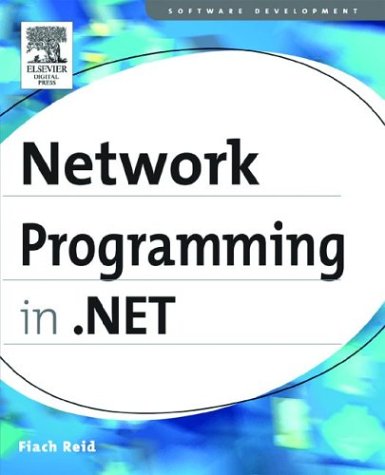
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProcessInfo.cs
- InvalidFilterCriteriaException.cs
- cookiecontainer.cs
- HttpCookieCollection.cs
- CodeThrowExceptionStatement.cs
- ObjectQuery_EntitySqlExtensions.cs
- JsonEncodingStreamWrapper.cs
- EntityWithChangeTrackerStrategy.cs
- GroupQuery.cs
- HighlightOverlayGlyph.cs
- WebBrowser.cs
- ConnectionPointConverter.cs
- SimpleBitVector32.cs
- BaseHashHelper.cs
- ConfigPathUtility.cs
- TrackingStringDictionary.cs
- FixedSOMTable.cs
- RuleProcessor.cs
- XmlSchemaSimpleContentExtension.cs
- Label.cs
- PageRanges.cs
- TextControl.cs
- LiteralControl.cs
- WCFBuildProvider.cs
- SuppressMergeCheckAttribute.cs
- DeferredElementTreeState.cs
- TrackBarRenderer.cs
- XsltArgumentList.cs
- MessageSecurityException.cs
- ApplicationFileParser.cs
- TreeSet.cs
- Rectangle.cs
- BamlBinaryReader.cs
- SystemInformation.cs
- streamingZipPartStream.cs
- ToolStripScrollButton.cs
- XmlComplianceUtil.cs
- ChannelRequirements.cs
- StylusPointProperties.cs
- OdbcHandle.cs
- ObjectViewEntityCollectionData.cs
- MenuItemCollectionEditor.cs
- CommandEventArgs.cs
- RequestQueryProcessor.cs
- NativeMethods.cs
- List.cs
- OciLobLocator.cs
- DefaultBinder.cs
- XsltCompileContext.cs
- KeyGestureValueSerializer.cs
- ErrorWrapper.cs
- ClrProviderManifest.cs
- PerformanceCounterManager.cs
- GlyphInfoList.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- SrgsRulesCollection.cs
- PieceNameHelper.cs
- SchemaTypeEmitter.cs
- CodeSnippetTypeMember.cs
- BitmapEffectGroup.cs
- HitTestWithPointDrawingContextWalker.cs
- RenderDataDrawingContext.cs
- Region.cs
- ObjectListSelectEventArgs.cs
- Visitor.cs
- HttpStreamXmlDictionaryReader.cs
- ServiceModelEnumValidatorAttribute.cs
- FontStretchConverter.cs
- SystemBrushes.cs
- GlobalizationSection.cs
- httpstaticobjectscollection.cs
- PointLight.cs
- WsatServiceAddress.cs
- GeneralTransformCollection.cs
- AlternateView.cs
- SqlMethodTransformer.cs
- DataSysAttribute.cs
- Evidence.cs
- Win32.cs
- ImageClickEventArgs.cs
- VisualBasicSettings.cs
- StateValidator.cs
- FilteredAttributeCollection.cs
- SynchronizingStream.cs
- ModelFunctionTypeElement.cs
- Size3D.cs
- TypedLocationWrapper.cs
- MessageQueueTransaction.cs
- SqlBulkCopy.cs
- DataColumnMappingCollection.cs
- StringStorage.cs
- ProviderConnectionPoint.cs
- XmlTextWriter.cs
- WebControl.cs
- EditCommandColumn.cs
- XmlAttributeCollection.cs
- GeneralTransformGroup.cs
- HttpException.cs
- VectorAnimationUsingKeyFrames.cs
- ScrollBar.cs