Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Messaging / System / Messaging / MessageQueueTransaction.cs / 1305376 / MessageQueueTransaction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Messaging { using System.Threading; using System.Diagnostics; using System.Messaging.Interop; ////// /// public class MessageQueueTransaction : IDisposable { private ITransaction internalTransaction; private MessageQueueTransactionStatus transactionStatus; private bool disposed; ///[To be supplied.] ////// /// public MessageQueueTransaction() { this.transactionStatus = MessageQueueTransactionStatus.Initialized; } internal ITransaction InnerTransaction { get { return this.internalTransaction; } } ////// Creates a new Message Queuing internal transaction context. /// ////// /// public MessageQueueTransactionStatus Status { get { return this.transactionStatus; } } ////// The status of the transaction that this object represents. /// ////// /// public void Abort() { lock (this) { if (this.internalTransaction == null) throw new InvalidOperationException(Res.GetString(Res.TransactionNotStarted)); else { this.AbortInternalTransaction(); } } } ////// Rolls back the pending internal transaction. /// ////// private void AbortInternalTransaction() { int status = this.internalTransaction.Abort(0, 0, 0); if (MessageQueue.IsFatalError(status)) throw new MessageQueueException(status); this.internalTransaction = null; this.transactionStatus = MessageQueueTransactionStatus.Aborted; } /// /// /// public void Begin() { //Won't allow begining a new transaction after the object has been disposed. if (this.disposed) throw new ObjectDisposedException(GetType().Name); lock (this) { if (internalTransaction != null) throw new InvalidOperationException(Res.GetString(Res.TransactionStarted)); else { int status = SafeNativeMethods.MQBeginTransaction(out this.internalTransaction); if (MessageQueue.IsFatalError(status)) { this.internalTransaction = null; throw new MessageQueueException(status); } this.transactionStatus = MessageQueueTransactionStatus.Pending; } } } ////// Begins a new Message Queuing internal transaction context. /// ////// internal ITransaction BeginQueueOperation() { #pragma warning disable 0618 //@ Monitor.Enter(this); #pragma warning restore 0618 return this.internalTransaction; } /// /// /// public void Commit() { lock (this) { if (this.internalTransaction == null) throw new InvalidOperationException(Res.GetString(Res.TransactionNotStarted)); else { int status = this.internalTransaction.Commit(0, 0, 0); if (MessageQueue.IsFatalError(status)) throw new MessageQueueException(status); this.internalTransaction = null; this.transactionStatus = MessageQueueTransactionStatus.Committed; } } } ////// Commits a pending internal transaction. /// ////// /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Disposes this transaction instance, if it is in a /// pending status, the transaction will be aborted. /// ////// /// protected virtual void Dispose(bool disposing) { if (disposing) { lock (this) { if (internalTransaction != null) this.AbortInternalTransaction(); } } this.disposed = true; } ////// ////// ~MessageQueueTransaction() { Dispose(false); } /// /// internal void EndQueueOperation() { Monitor.Exit(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
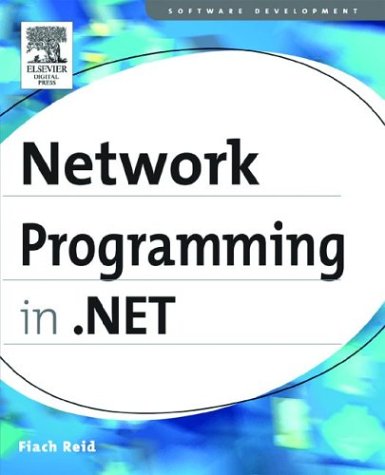
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AccessControlList.cs
- TrackingLocationCollection.cs
- MergeFailedEvent.cs
- TableItemPatternIdentifiers.cs
- SelectingProviderEventArgs.cs
- SqlException.cs
- _SSPISessionCache.cs
- XPathAxisIterator.cs
- SqlWorkflowPersistenceService.cs
- XPathDocumentIterator.cs
- ObjectItemAttributeAssemblyLoader.cs
- CellParagraph.cs
- ApplicationSecurityInfo.cs
- DataGridViewCellMouseEventArgs.cs
- ListViewGroupItemCollection.cs
- RichTextBoxAutomationPeer.cs
- ImageField.cs
- InternalConfigEventArgs.cs
- SqlBulkCopy.cs
- CqlParser.cs
- WindowsIdentity.cs
- BooleanProjectedSlot.cs
- BuildProvider.cs
- CloseCollectionAsyncResult.cs
- SpecularMaterial.cs
- Style.cs
- TableDetailsRow.cs
- PeerName.cs
- SqlError.cs
- DataControlPagerLinkButton.cs
- IsolatedStoragePermission.cs
- XmlWriterTraceListener.cs
- JournalEntryStack.cs
- ACE.cs
- Registry.cs
- Margins.cs
- EncryptedReference.cs
- LowerCaseStringConverter.cs
- AnnotationHighlightLayer.cs
- InternalUserCancelledException.cs
- BuildManagerHost.cs
- SchemaImporter.cs
- DirectoryInfo.cs
- Helper.cs
- SqlRecordBuffer.cs
- NetworkInterface.cs
- StaticResourceExtension.cs
- LongValidatorAttribute.cs
- CodeDomConfigurationHandler.cs
- GlyphCache.cs
- OracleTransaction.cs
- CredentialCache.cs
- RepeatBehavior.cs
- TraceHandlerErrorFormatter.cs
- NeedSkipTokenVisitor.cs
- ConnectionManagementElementCollection.cs
- _CacheStreams.cs
- EncryptedKey.cs
- DbXmlEnabledProviderManifest.cs
- PaperSource.cs
- StylusDownEventArgs.cs
- BooleanAnimationUsingKeyFrames.cs
- SessionEndingCancelEventArgs.cs
- TransformerInfo.cs
- CalendarItem.cs
- BuildProvidersCompiler.cs
- RuleRef.cs
- ParagraphResult.cs
- SiteMapNodeItemEventArgs.cs
- XamlParser.cs
- BindingEntityInfo.cs
- ButtonFlatAdapter.cs
- COM2TypeInfoProcessor.cs
- ToolBarOverflowPanel.cs
- SelectionService.cs
- UInt64Storage.cs
- SqlUserDefinedAggregateAttribute.cs
- SqlCacheDependencyDatabaseCollection.cs
- SymbolMethod.cs
- ImageCodecInfo.cs
- AddInContractAttribute.cs
- FormsAuthenticationUserCollection.cs
- ProcessManager.cs
- ConditionalAttribute.cs
- FontEmbeddingManager.cs
- ExtenderControl.cs
- CorrelationTokenInvalidatedHandler.cs
- odbcmetadatacolumnnames.cs
- LifetimeServices.cs
- Timer.cs
- BaseParser.cs
- FreezableCollection.cs
- PermissionSet.cs
- DesignerHierarchicalDataSourceView.cs
- TrackingLocationCollection.cs
- Assert.cs
- TypedTableHandler.cs
- ToolZone.cs
- SystemUdpStatistics.cs
- ExpressionReplacer.cs