Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Controls / Primitives / ToolBarOverflowPanel.cs / 1 / ToolBarOverflowPanel.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Collections.Generic; using System.Threading; using System.Windows; using System.Windows.Controls; using System.Windows.Media; using System.Windows.Shapes; using System.Windows.Documents; using System; namespace System.Windows.Controls.Primitives { ////// ToolBarOverflowPanel /// public class ToolBarOverflowPanel : Panel { #region Properties ////// WrapWidth Property /// public static readonly DependencyProperty WrapWidthProperty = DependencyProperty.Register( "WrapWidth", typeof(double), typeof(ToolBarOverflowPanel), new FrameworkPropertyMetadata(Double.NaN, FrameworkPropertyMetadataOptions.AffectsMeasure), new ValidateValueCallback(IsWrapWidthValid)); ////// WrapWidth Property /// public double WrapWidth { get { return (double)GetValue(WrapWidthProperty); } set { SetValue(WrapWidthProperty, value); } } private static bool IsWrapWidthValid(object value) { double v = (double)value; return (MS.Internal.DoubleUtil.IsNaN(v)) || (v >= 0.0d && !Double.IsPositiveInfinity(v)); } #endregion Properties #region Override methods ////// Measure the content and store the desired size of the content /// /// ///protected override Size MeasureOverride(Size constraint) { int i; Size curLineSize = new Size(); Size panelSize = new Size(); _wrapWidth = double.IsNaN(WrapWidth) ? constraint.Width : WrapWidth; UIElementCollection children = this.Children; // Add ToolBar items which has IsOverflowItem = true ToolBar toolBar = ToolBar; if (toolBar != null) { ToolBarPanel toolBarPanel = toolBar.ToolBarPanel; if (toolBarPanel != null) { children.Clear(); List generatedItemsCollection = toolBarPanel.GeneratedItemsCollection; for (i = 0; i < generatedItemsCollection.Count; i++) { UIElement uiElement = generatedItemsCollection[i]; if (uiElement != null && ToolBar.GetIsOverflowItem(uiElement) && !(uiElement is Separator)) { children.Add(uiElement); } } } } // Measure all children to determine if we need to increase desired wrapWidth for (i = 0; i < children.Count; i++) { UIElement child = children[i] as UIElement; child.Measure(constraint); Size sz = child.DesiredSize; if (sz.Width > _wrapWidth) _wrapWidth = sz.Width; } // wrapWidth should not be bigger than constraint.Width _wrapWidth = Math.Min(_wrapWidth, constraint.Width); for (i = 0; i < children.Count; i++) { UIElement child = children[i] as UIElement; Size sz = child.DesiredSize; if (curLineSize.Width + sz.Width > _wrapWidth) //need to switch to another line { panelSize.Width = Math.Max(curLineSize.Width, panelSize.Width); panelSize.Height += curLineSize.Height; curLineSize = sz; if (sz.Width > _wrapWidth) //the element is wider then the constraint - give it a separate line { panelSize.Width = Math.Max(sz.Width, panelSize.Width); panelSize.Height += sz.Height; curLineSize = new Size(); } } else //continue to accumulate a line { curLineSize.Width += sz.Width; curLineSize.Height = Math.Max(sz.Height, curLineSize.Height); } } //the last line size, if any should be added panelSize.Width = Math.Max(curLineSize.Width, panelSize.Width); panelSize.Height += curLineSize.Height; return panelSize; } /// /// Content arrangement. /// /// ///protected override Size ArrangeOverride(Size arrangeBounds) { int firstInLine = 0; Size curLineSize = new Size(); double accumulatedHeight = 0d; _wrapWidth = Math.Min(_wrapWidth, arrangeBounds.Width); UIElementCollection children = this.Children; for (int i = 0; i < children.Count; i++) { Size sz = children[i].DesiredSize; if (curLineSize.Width + sz.Width > _wrapWidth) //need to switch to another line { // Arrange the items in the current line not including the current arrangeLine(accumulatedHeight, curLineSize.Height, firstInLine, i); accumulatedHeight += curLineSize.Height; // Current item will be first on the next line firstInLine = i; curLineSize = sz; } else //continue to accumulate a line { curLineSize.Width += sz.Width; curLineSize.Height = Math.Max(sz.Height, curLineSize.Height); } } arrangeLine(accumulatedHeight, curLineSize.Height, firstInLine, children.Count); return DesiredSize; } /// /// Creates a new UIElementCollection. Panel-derived class can create its own version of /// UIElementCollection -derived class to add cached information to every child or to /// intercept any Add/Remove actions (for example, for incremental layout update) /// protected override UIElementCollection CreateUIElementCollection(FrameworkElement logicalParent) { // we ignore the Logical Parent (this) if we have ToolBar as our TemplatedParent return new UIElementCollection(this, TemplatedParent == null ? logicalParent : null); } private void arrangeLine(double y, double lineHeight, int start, int end) { double x = 0; UIElementCollection children = this.Children; for (int i = start; i < end; i++) { UIElement child = children[i]; child.Arrange(new Rect(x, y, child.DesiredSize.Width, lineHeight)); x += child.DesiredSize.Width; } } #endregion Override methods #region private implementation private ToolBar ToolBar { get { return TemplatedParent as ToolBar; } } private ToolBarPanel ToolBarPanel { get { ToolBar tb = ToolBar; return tb == null ? null : tb.ToolBarPanel; } } #endregion private implementation #region private data private double _wrapWidth; // calculated in MeasureOverride and used in ArrangeOverride #endregion private data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
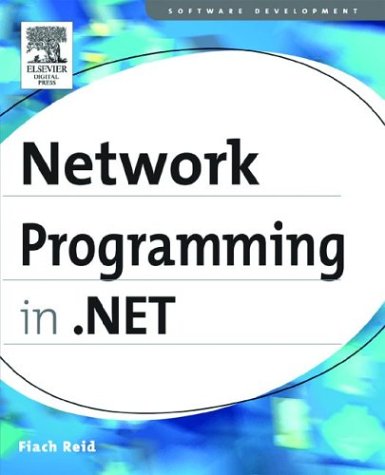
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnsafeNativeMethods.cs
- SizeConverter.cs
- KnownTypes.cs
- GridViewCommandEventArgs.cs
- ConnectionsZone.cs
- QilChoice.cs
- MarkupCompilePass1.cs
- PathGeometry.cs
- EdmValidator.cs
- CommandHelpers.cs
- ThreadExceptionDialog.cs
- ColorConverter.cs
- BindValidator.cs
- TransactionInformation.cs
- _DomainName.cs
- WSTransactionSection.cs
- PlacementWorkspace.cs
- WindowsListViewScroll.cs
- ToolStripLocationCancelEventArgs.cs
- RTLAwareMessageBox.cs
- ParseChildrenAsPropertiesAttribute.cs
- NullableBoolConverter.cs
- CalendarDay.cs
- BoundingRectTracker.cs
- UrlPath.cs
- AvTraceFormat.cs
- IndexedEnumerable.cs
- HandlerFactoryCache.cs
- SectionInformation.cs
- CodeSubDirectoriesCollection.cs
- IxmlLineInfo.cs
- DesignerActionVerbItem.cs
- ParameterToken.cs
- AnalyzedTree.cs
- TimeSpanStorage.cs
- TextBreakpoint.cs
- StandardToolWindows.cs
- NavigationWindowAutomationPeer.cs
- GPPOINTF.cs
- FrameworkPropertyMetadata.cs
- FactoryRecord.cs
- DbBuffer.cs
- HttpListenerPrefixCollection.cs
- TrackBarRenderer.cs
- InvalidDocumentContentsException.cs
- DelegatingConfigHost.cs
- TemplateXamlParser.cs
- MetadataProperty.cs
- ExtenderProvidedPropertyAttribute.cs
- ReferenceSchema.cs
- RunWorkerCompletedEventArgs.cs
- PassportPrincipal.cs
- ObjectItemLoadingSessionData.cs
- GlyphRunDrawing.cs
- DataColumnPropertyDescriptor.cs
- X509CertificateChain.cs
- WrapperSecurityCommunicationObject.cs
- DataTable.cs
- WindowsSpinner.cs
- StyleBamlTreeBuilder.cs
- TextStore.cs
- TextLineBreak.cs
- MSAAEventDispatcher.cs
- TextRangeProviderWrapper.cs
- IndentedTextWriter.cs
- EventSinkHelperWriter.cs
- StatusBarDrawItemEvent.cs
- ToolStripContextMenu.cs
- Transform3DGroup.cs
- _NestedSingleAsyncResult.cs
- SqlRecordBuffer.cs
- AlgoModule.cs
- ExpressionBuilderContext.cs
- HttpListenerContext.cs
- translator.cs
- DropShadowEffect.cs
- WebReferencesBuildProvider.cs
- PostBackTrigger.cs
- AuthorizationRule.cs
- X509CertificateRecipientServiceCredential.cs
- XmlIlVisitor.cs
- DrawingCollection.cs
- SegmentInfo.cs
- ToolStripPanelRenderEventArgs.cs
- BitmapImage.cs
- ReadOnlyDataSource.cs
- XmlSchemaImporter.cs
- EventSource.cs
- TextServicesPropertyRanges.cs
- DeclarationUpdate.cs
- DataSourceControl.cs
- UiaCoreTypesApi.cs
- PoisonMessageException.cs
- TimersDescriptionAttribute.cs
- WebScriptMetadataMessageEncoderFactory.cs
- BindingManagerDataErrorEventArgs.cs
- AudioException.cs
- ResolveCriteria11.cs
- UnauthorizedWebPart.cs
- GridErrorDlg.cs