Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntityDesign / Design / System / Data / Entity / Design / SSDLGenerator / TableDetailsRow.cs / 1305376 / TableDetailsRow.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Xml; using System.Data.Entity.Design.Common; using System.Globalization; using System.Data; namespace System.Data.Entity.Design.SsdlGenerator { ////// Strongly typed DataTable for TableDetails /// internal sealed class TableDetailsRow : System.Data.DataRow { private TableDetailsCollection _tableTableDetails; [System.Diagnostics.DebuggerNonUserCodeAttribute()] internal TableDetailsRow(System.Data.DataRowBuilder rb) : base(rb) { this._tableTableDetails = ((TableDetailsCollection)(base.Table)); } ////// Gets a strongly typed table /// public new TableDetailsCollection Table { get { return _tableTableDetails; } } ////// Gets the Catalog column value /// public string Catalog { get { try { return ((string)(this[this._tableTableDetails.CatalogColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.CatalogColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.CatalogColumn] = value; } } ////// Gets the Schema column value /// public string Schema { get { try { return ((string)(this[this._tableTableDetails.SchemaColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.SchemaColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.SchemaColumn] = value; } } ////// Gets the TableName column value /// public string TableName { get { try { return ((string)(this[this._tableTableDetails.TableNameColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.TableNameColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.TableNameColumn] = value; } } ////// Gets the ColumnName column value /// public string ColumnName { get { try { return ((string)(this[this._tableTableDetails.ColumnNameColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.ColumnNameColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.ColumnNameColumn] = value; } } ////// Gets the IsNullable column value /// public bool IsNullable { get { try { return ((bool)(this[this._tableTableDetails.IsNullableColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsNullableColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsNullableColumn] = value; } } ////// Gets the DataType column value /// public string DataType { get { try { return ((string)(this[this._tableTableDetails.DataTypeColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.DataTypeColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.DataTypeColumn] = value; } } ////// Gets the MaximumLength column value /// public int MaximumLength { get { try { return ((int)(this[this._tableTableDetails.MaximumLengthColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.MaximumLengthColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.MaximumLengthColumn] = value; } } ////// Gets the DateTime Precision column value /// public int DateTimePrecision { get { try { return ((int)(this[this._tableTableDetails.DateTimePrecisionColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.DateTimePrecisionColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.DateTimePrecisionColumn] = value; } } ////// Gets the Precision column value /// public int Precision { get { try { return ((int)(this[this._tableTableDetails.PrecisionColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.PrecisionColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.PrecisionColumn] = value; } } ////// Gets the Scale column value /// public int Scale { get { try { return ((int)(this[this._tableTableDetails.ScaleColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.ScaleColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.ScaleColumn] = value; } } ////// Gets the IsServerGenerated column value /// public bool IsIdentity { get { try { return ((bool)(this[this._tableTableDetails.IsIdentityColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsIdentityColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsIdentityColumn] = value; } } ////// Gets the IsServerGenerated column value /// public bool IsServerGenerated { get { try { return ((bool)(this[this._tableTableDetails.IsServerGeneratedColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsServerGeneratedColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsServerGeneratedColumn] = value; } } ////// Gets the IsPrimaryKey column value /// public bool IsPrimaryKey { get { try { return ((bool)(this[this._tableTableDetails.IsPrimaryKeyColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsPrimaryKeyColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsPrimaryKeyColumn] = value; } } ////// Determines if the Catalog column value is null /// ///true if the value is null, otherwise false. public bool IsCatalogNull() { return this.IsNull(this._tableTableDetails.CatalogColumn); } ////// Determines if the Schema column value is null /// ///true if the value is null, otherwise false. public bool IsSchemaNull() { return this.IsNull(this._tableTableDetails.SchemaColumn); } ////// Determines if the DataType column value is null /// ///true if the value is null, otherwise false. public bool IsDataTypeNull() { return this.IsNull(this._tableTableDetails.DataTypeColumn); } ////// Determines if the MaximumLength column value is null /// ///true if the value is null, otherwise false. public bool IsMaximumLengthNull() { return this.IsNull(this._tableTableDetails.MaximumLengthColumn); } ////// Determines if the Precision column value is null /// ///true if the value is null, otherwise false. public bool IsPrecisionNull() { return this.IsNull(this._tableTableDetails.PrecisionColumn); } ////// Determines if the DateTime Precision column value is null /// ///true if the value is null, otherwise false. public bool IsDateTimePrecisionNull() { return this.IsNull(this._tableTableDetails.DateTimePrecisionColumn); } ////// Determines if the Scale column value is null /// ///true if the value is null, otherwise false. public bool IsScaleNull() { return this.IsNull(this._tableTableDetails.ScaleColumn); } ////// Determines if the IsIdentity column value is null /// ///true if the value is null, otherwise false. public bool IsIsIdentityNull() { return this.IsNull(this._tableTableDetails.IsIdentityColumn); } ////// Determines if the IsIdentity column value is null /// ///true if the value is null, otherwise false. public bool IsIsServerGeneratedNull() { return this.IsNull(this._tableTableDetails.IsServerGeneratedColumn); } public string GetMostQualifiedTableName() { string name = string.Empty; if (!IsCatalogNull()) { name = Catalog; } if (!IsSchemaNull()) { if (name != string.Empty) { name += "."; } name += Schema; } if (name != string.Empty) { name += "."; } // TableName is not allowed to be null name += TableName; return name; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Xml; using System.Data.Entity.Design.Common; using System.Globalization; using System.Data; namespace System.Data.Entity.Design.SsdlGenerator { ////// Strongly typed DataTable for TableDetails /// internal sealed class TableDetailsRow : System.Data.DataRow { private TableDetailsCollection _tableTableDetails; [System.Diagnostics.DebuggerNonUserCodeAttribute()] internal TableDetailsRow(System.Data.DataRowBuilder rb) : base(rb) { this._tableTableDetails = ((TableDetailsCollection)(base.Table)); } ////// Gets a strongly typed table /// public new TableDetailsCollection Table { get { return _tableTableDetails; } } ////// Gets the Catalog column value /// public string Catalog { get { try { return ((string)(this[this._tableTableDetails.CatalogColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.CatalogColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.CatalogColumn] = value; } } ////// Gets the Schema column value /// public string Schema { get { try { return ((string)(this[this._tableTableDetails.SchemaColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.SchemaColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.SchemaColumn] = value; } } ////// Gets the TableName column value /// public string TableName { get { try { return ((string)(this[this._tableTableDetails.TableNameColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.TableNameColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.TableNameColumn] = value; } } ////// Gets the ColumnName column value /// public string ColumnName { get { try { return ((string)(this[this._tableTableDetails.ColumnNameColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.ColumnNameColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.ColumnNameColumn] = value; } } ////// Gets the IsNullable column value /// public bool IsNullable { get { try { return ((bool)(this[this._tableTableDetails.IsNullableColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsNullableColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsNullableColumn] = value; } } ////// Gets the DataType column value /// public string DataType { get { try { return ((string)(this[this._tableTableDetails.DataTypeColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.DataTypeColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.DataTypeColumn] = value; } } ////// Gets the MaximumLength column value /// public int MaximumLength { get { try { return ((int)(this[this._tableTableDetails.MaximumLengthColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.MaximumLengthColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.MaximumLengthColumn] = value; } } ////// Gets the DateTime Precision column value /// public int DateTimePrecision { get { try { return ((int)(this[this._tableTableDetails.DateTimePrecisionColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.DateTimePrecisionColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.DateTimePrecisionColumn] = value; } } ////// Gets the Precision column value /// public int Precision { get { try { return ((int)(this[this._tableTableDetails.PrecisionColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.PrecisionColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.PrecisionColumn] = value; } } ////// Gets the Scale column value /// public int Scale { get { try { return ((int)(this[this._tableTableDetails.ScaleColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.ScaleColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.ScaleColumn] = value; } } ////// Gets the IsServerGenerated column value /// public bool IsIdentity { get { try { return ((bool)(this[this._tableTableDetails.IsIdentityColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsIdentityColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsIdentityColumn] = value; } } ////// Gets the IsServerGenerated column value /// public bool IsServerGenerated { get { try { return ((bool)(this[this._tableTableDetails.IsServerGeneratedColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsServerGeneratedColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsServerGeneratedColumn] = value; } } ////// Gets the IsPrimaryKey column value /// public bool IsPrimaryKey { get { try { return ((bool)(this[this._tableTableDetails.IsPrimaryKeyColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsPrimaryKeyColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsPrimaryKeyColumn] = value; } } ////// Determines if the Catalog column value is null /// ///true if the value is null, otherwise false. public bool IsCatalogNull() { return this.IsNull(this._tableTableDetails.CatalogColumn); } ////// Determines if the Schema column value is null /// ///true if the value is null, otherwise false. public bool IsSchemaNull() { return this.IsNull(this._tableTableDetails.SchemaColumn); } ////// Determines if the DataType column value is null /// ///true if the value is null, otherwise false. public bool IsDataTypeNull() { return this.IsNull(this._tableTableDetails.DataTypeColumn); } ////// Determines if the MaximumLength column value is null /// ///true if the value is null, otherwise false. public bool IsMaximumLengthNull() { return this.IsNull(this._tableTableDetails.MaximumLengthColumn); } ////// Determines if the Precision column value is null /// ///true if the value is null, otherwise false. public bool IsPrecisionNull() { return this.IsNull(this._tableTableDetails.PrecisionColumn); } ////// Determines if the DateTime Precision column value is null /// ///true if the value is null, otherwise false. public bool IsDateTimePrecisionNull() { return this.IsNull(this._tableTableDetails.DateTimePrecisionColumn); } ////// Determines if the Scale column value is null /// ///true if the value is null, otherwise false. public bool IsScaleNull() { return this.IsNull(this._tableTableDetails.ScaleColumn); } ////// Determines if the IsIdentity column value is null /// ///true if the value is null, otherwise false. public bool IsIsIdentityNull() { return this.IsNull(this._tableTableDetails.IsIdentityColumn); } ////// Determines if the IsIdentity column value is null /// ///true if the value is null, otherwise false. public bool IsIsServerGeneratedNull() { return this.IsNull(this._tableTableDetails.IsServerGeneratedColumn); } public string GetMostQualifiedTableName() { string name = string.Empty; if (!IsCatalogNull()) { name = Catalog; } if (!IsSchemaNull()) { if (name != string.Empty) { name += "."; } name += Schema; } if (name != string.Empty) { name += "."; } // TableName is not allowed to be null name += TableName; return name; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
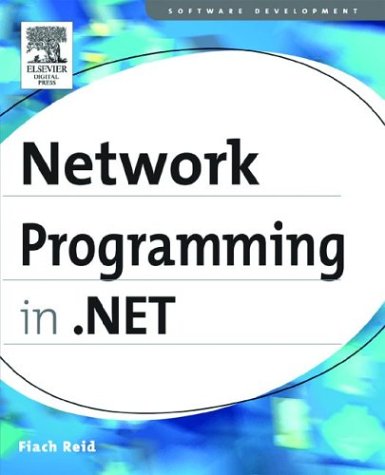
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewItemSelectionChangedEvent.cs
- BindingMemberInfo.cs
- Pair.cs
- SqlParameterCollection.cs
- FormsAuthenticationTicket.cs
- ToolStripRendererSwitcher.cs
- Material.cs
- LinqToSqlWrapper.cs
- CompositeDataBoundControl.cs
- DATA_BLOB.cs
- ProvidersHelper.cs
- LoginName.cs
- basevalidator.cs
- StreamingContext.cs
- ResizeGrip.cs
- AuthenticationSection.cs
- ArrayWithOffset.cs
- Stack.cs
- CrossContextChannel.cs
- SessionPageStatePersister.cs
- DeploymentSectionCache.cs
- BlurEffect.cs
- XPathPatternParser.cs
- RectAnimation.cs
- COM2FontConverter.cs
- ToolBar.cs
- Signature.cs
- RoleManagerEventArgs.cs
- DrawingGroupDrawingContext.cs
- DataGridViewCellValidatingEventArgs.cs
- DocumentEventArgs.cs
- DataGridViewRowStateChangedEventArgs.cs
- Geometry.cs
- PrincipalPermission.cs
- MetadataExchangeClient.cs
- TreeViewAutomationPeer.cs
- ViewManager.cs
- NaturalLanguageHyphenator.cs
- ipaddressinformationcollection.cs
- ExpressionNode.cs
- NetStream.cs
- CloseSequence.cs
- BamlRecordWriter.cs
- DescendantQuery.cs
- MessageQueuePermission.cs
- XmlSchemaExporter.cs
- ParseHttpDate.cs
- ExtensionQuery.cs
- ToolStripPanelRow.cs
- MaterializeFromAtom.cs
- XamlTreeBuilderBamlRecordWriter.cs
- SqlReferenceCollection.cs
- DefaultValueAttribute.cs
- AppDomainAttributes.cs
- DecoderNLS.cs
- SerializerWriterEventHandlers.cs
- DataGridBoundColumn.cs
- ReadOnlyAttribute.cs
- figurelengthconverter.cs
- XPathScanner.cs
- AVElementHelper.cs
- _LoggingObject.cs
- ProvidersHelper.cs
- DocumentOrderQuery.cs
- SecurityTokenSerializer.cs
- RootBrowserWindow.cs
- _HeaderInfoTable.cs
- returneventsaver.cs
- BindingExpressionBase.cs
- LocalizableAttribute.cs
- UnsafeNetInfoNativeMethods.cs
- LiteralControl.cs
- IPPacketInformation.cs
- WmpBitmapEncoder.cs
- ToolStripSplitStackLayout.cs
- WebBrowserContainer.cs
- ColumnClickEvent.cs
- FontStretch.cs
- CLRBindingWorker.cs
- DetailsViewPageEventArgs.cs
- VirtualizingStackPanel.cs
- DependencyObjectProvider.cs
- PeerApplicationLaunchInfo.cs
- GenericWebPart.cs
- ResourceReferenceExpressionConverter.cs
- DataSourceView.cs
- WriteableBitmap.cs
- ToolStripDropTargetManager.cs
- UnlockInstanceCommand.cs
- HwndSource.cs
- SoapProtocolReflector.cs
- SQLByteStorage.cs
- ExpressionVisitorHelpers.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- EndpointDiscoveryMetadataCD1.cs
- XmlLinkedNode.cs
- EntityKey.cs
- __Error.cs
- BaseProcessor.cs
- DateTimeOffset.cs