Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / Advanced / TextureBrush.cs / 1 / TextureBrush.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /*************************************************************************\ * * Copyright (c) 1998-1999, Microsoft Corp. All Rights Reserved. * * Module Name: * * TextureBrush.cs * * Abstract: * * COM+ wrapper for GDI+ TextureBrush Brush objects * * Revision History: * * 12/15/1998 [....] * Created it. * \**************************************************************************/ namespace System.Drawing { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Drawing; using Microsoft.Win32; using System.ComponentModel; using System.Drawing.Drawing2D; using System.Drawing.Internal; using System.Drawing.Imaging; /** * Represent a Texture brush object */ ////// /// Encapsulates a public sealed class TextureBrush : Brush { /** * Create a new texture brush object * * @notes Should the rectangle parameter be Rectangle or RectF? * We'll use Rectangle to specify pixel unit source image * rectangle for now. Eventually, we'll need a mechanism * to specify areas of an image in a resolution-independent way. * * @notes We'll make a copy of the bitmap object passed in. */ // When creating a texture brush from a metafile image, the dstRect // is used to specify the size that the metafile image should be // rendered at in the device units of the destination graphics. // It is NOT used to crop the metafile image, so only the width // and height values matter for metafiles. ///that uses an fills the /// interior of a shape with an image. /// /// /// Initializes a new instance of the public TextureBrush(Image bitmap) : this(bitmap, System.Drawing.Drawing2D.WrapMode.Tile) { } // When creating a texture brush from a metafile image, the dstRect // is used to specify the size that the metafile image should be // rendered at in the device units of the destination graphics. // It is NOT used to crop the metafile image, so only the width // and height values matter for metafiles. ////// class with the specified image. /// /// /// public TextureBrush(Image image, WrapMode wrapMode) { if (image == null) throw new ArgumentNullException("image"); //validate the WrapMode enum //valid values are 0x0 to 0x4 if (!ClientUtils.IsEnumValid(wrapMode, (int)wrapMode, (int)WrapMode.Tile, (int)WrapMode.Clamp)){ throw new InvalidEnumArgumentException("wrapMode", (int)wrapMode, typeof(WrapMode)); } IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateTexture(new HandleRef(image, image.nativeImage), (int) wrapMode, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrush(brush); } // When creating a texture brush from a metafile image, the dstRect // is used to specify the size that the metafile image should be // rendered at in the device units of the destination graphics. // It is NOT used to crop the metafile image, so only the width // and height values matter for metafiles. // float version ////// Initializes a new instance of the ////// class with the specified image and wrap mode. /// /// /// public TextureBrush(Image image, WrapMode wrapMode, RectangleF dstRect) { if (image == null) throw new ArgumentNullException("image"); //validate the WrapMode enum //valid values are 0x0 to 0x4 if (!ClientUtils.IsEnumValid(wrapMode, (int)wrapMode, (int)WrapMode.Tile, (int)WrapMode.Clamp)) { throw new InvalidEnumArgumentException("wrapMode", (int)wrapMode, typeof(WrapMode)); } IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateTexture2(new HandleRef(image, image.nativeImage), (int) wrapMode, dstRect.X, dstRect.Y, dstRect.Width, dstRect.Height, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrush(brush); } // int version // When creating a texture brush from a metafile image, the dstRect // is used to specify the size that the metafile image should be // rendered at in the device units of the destination graphics. // It is NOT used to crop the metafile image, so only the width // and height values matter for metafiles. ////// Initializes a new instance of the ////// class with the specified image, wrap mode, and bounding rectangle. /// /// /// public TextureBrush(Image image, WrapMode wrapMode, Rectangle dstRect) { if (image == null) throw new ArgumentNullException("image"); //validate the WrapMode enum //valid values are 0x0 to 0x4 if (!ClientUtils.IsEnumValid(wrapMode, (int)wrapMode, (int)WrapMode.Tile, (int)WrapMode.Clamp)) { throw new InvalidEnumArgumentException("wrapMode", (int)wrapMode, typeof(WrapMode)); } IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateTexture2I(new HandleRef(image, image.nativeImage), (int) wrapMode, dstRect.X, dstRect.Y, dstRect.Width, dstRect.Height, out brush); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } SetNativeBrush(brush); } // When creating a texture brush from a metafile image, the dstRect // is used to specify the size that the metafile image should be // rendered at in the device units of the destination graphics. // It is NOT used to crop the metafile image, so only the width // and height values matter for metafiles. ////// Initializes a new instance of the ////// class with the specified image, wrap mode, and bounding rectangle. /// /// /// public TextureBrush(Image image, RectangleF dstRect) : this(image, dstRect, (ImageAttributes)null) {} // When creating a texture brush from a metafile image, the dstRect // is used to specify the size that the metafile image should be // rendered at in the device units of the destination graphics. // It is NOT used to crop the metafile image, so only the width // and height values matter for metafiles. ////// Initializes a new instance of the ///class with the specified image /// and bounding rectangle. /// /// /// public TextureBrush(Image image, RectangleF dstRect, ImageAttributes imageAttr) { if (image == null) throw new ArgumentNullException("image"); IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateTextureIA(new HandleRef(image, image.nativeImage), new HandleRef(imageAttr, (imageAttr == null) ? IntPtr.Zero : imageAttr.nativeImageAttributes), dstRect.X, dstRect.Y, dstRect.Width, dstRect.Height, out brush); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } SetNativeBrush(brush); } // When creating a texture brush from a metafile image, the dstRect // is used to specify the size that the metafile image should be // rendered at in the device units of the destination graphics. // It is NOT used to crop the metafile image, so only the width // and height values matter for metafiles. ////// Initializes a new instance of the ///class with the specified /// image, bounding rectangle, and image attributes. /// /// /// public TextureBrush(Image image, Rectangle dstRect) : this(image, dstRect, (ImageAttributes)null) {} // When creating a texture brush from a metafile image, the dstRect // is used to specify the size that the metafile image should be // rendered at in the device units of the destination graphics. // It is NOT used to crop the metafile image, so only the width // and height values matter for metafiles. ////// Initializes a new instance of the ///class with the specified image /// and bounding rectangle. /// /// /// public TextureBrush(Image image, Rectangle dstRect, ImageAttributes imageAttr) { if (image == null) throw new ArgumentNullException("image"); IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateTextureIAI(new HandleRef(image, image.nativeImage), new HandleRef(imageAttr, (imageAttr == null) ? IntPtr.Zero : imageAttr.nativeImageAttributes), dstRect.X, dstRect.Y, dstRect.Width, dstRect.Height, out brush); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } SetNativeBrush(brush); } ////// Initializes a new instance of the ///class with the specified /// image, bounding rectangle, and image attributes. /// /// Constructor to initialized this object to be owned by GDI+. /// internal TextureBrush(IntPtr nativeBrush ) { Debug.Assert( nativeBrush != IntPtr.Zero, "Initializing native brush with null." ); SetNativeBrush( nativeBrush ); } ////// /// Creates an exact copy of this public override Object Clone() { IntPtr cloneBrush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneBrush(new HandleRef(this, this.NativeBrush), out cloneBrush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new TextureBrush(cloneBrush); } /** * Set/get brush transform */ private void _SetTransform(Matrix matrix) { int status = SafeNativeMethods.Gdip.GdipSetTextureTransform(new HandleRef(this, this.NativeBrush), new HandleRef(matrix, matrix.nativeMatrix)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private Matrix _GetTransform() { Matrix matrix = new Matrix(); int status = SafeNativeMethods.Gdip.GdipGetTextureTransform(new HandleRef(this, this.NativeBrush), new HandleRef(matrix, matrix.nativeMatrix)); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } return matrix; } ///. /// /// /// public Matrix Transform { get { return _GetTransform();} set { if (value == null) { throw new ArgumentNullException("value"); } _SetTransform(value); } } /** * Set/get brush wrapping mode */ private void _SetWrapMode(WrapMode wrapMode) { int status = SafeNativeMethods.Gdip.GdipSetTextureWrapMode(new HandleRef(this, this.NativeBrush), (int) wrapMode); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } } private WrapMode _GetWrapMode() { int mode = 0; int status = SafeNativeMethods.Gdip.GdipGetTextureWrapMode(new HandleRef(this, this.NativeBrush), out mode); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } return(WrapMode) mode; } ////// Gets or sets a ///that defines a local geometrical /// transform for this . /// /// /// public WrapMode WrapMode { get { return _GetWrapMode(); } set { //validate the WrapMode enum //valid values are 0x0 to 0x4 if (!ClientUtils.IsEnumValid(value, (int)value, (int)WrapMode.Tile, (int)WrapMode.Clamp)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(WrapMode)); } _SetWrapMode(value); } } ////// Gets or sets a ///that indicates the wrap mode for this /// . /// /// /// public Image Image { get { IntPtr image; int status = SafeNativeMethods.Gdip.GdipGetTextureImage(new HandleRef(this, this.NativeBrush), out image); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } return Image.CreateImageObject(image); } } ////// Gets the ///associated with this . /// /// /// public void ResetTransform() { int status = SafeNativeMethods.Gdip.GdipResetTextureTransform(new HandleRef(this, this.NativeBrush)); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } } ////// Resets the ///property to /// identity. /// /// /// public void MultiplyTransform(Matrix matrix) { MultiplyTransform(matrix, MatrixOrder.Prepend); } ////// Multiplies the ///that represents the local geometrical /// transform of this by the specified by prepending the specified . /// /// /// public void MultiplyTransform(Matrix matrix, MatrixOrder order) { if (matrix == null) { throw new ArgumentNullException("matrix"); } int status = SafeNativeMethods.Gdip.GdipMultiplyTextureTransform(new HandleRef(this, this.NativeBrush), new HandleRef(matrix, matrix.nativeMatrix), order); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } } ////// Multiplies the ///that represents the local geometrical /// transform of this by the specified in the specified order. /// /// /// public void TranslateTransform(float dx, float dy) { TranslateTransform(dx, dy, MatrixOrder.Prepend); } ////// Translates the local geometrical transform by the specified dimmensions. This /// method prepends the translation to the transform. /// ////// /// public void TranslateTransform(float dx, float dy, MatrixOrder order) { int status = SafeNativeMethods.Gdip.GdipTranslateTextureTransform(new HandleRef(this, this.NativeBrush), dx, dy, order); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } } ////// Translates the local geometrical transform by the specified dimmensions in /// the specified order. /// ////// /// public void ScaleTransform(float sx, float sy) { ScaleTransform(sx, sy, MatrixOrder.Prepend); } ////// Scales the local geometric transform by the specified amounts. This method /// prepends the scaling matrix to the transform. /// ////// /// public void ScaleTransform(float sx, float sy, MatrixOrder order) { int status = SafeNativeMethods.Gdip.GdipScaleTextureTransform(new HandleRef(this, this.NativeBrush), sx, sy, order); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } } ////// Scales the local geometric transform by the specified amounts in the /// specified order. /// ////// /// public void RotateTransform(float angle) { RotateTransform(angle, MatrixOrder.Prepend); } ////// Rotates the local geometric transform by the specified amount. This method /// prepends the rotation to the transform. /// ////// /// public void RotateTransform(float angle, MatrixOrder order) { int status = SafeNativeMethods.Gdip.GdipRotateTextureTransform(new HandleRef(this, this.NativeBrush), angle, order); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Rotates the local geometric transform by the specified amount in the /// specified order. /// ///
Link Menu
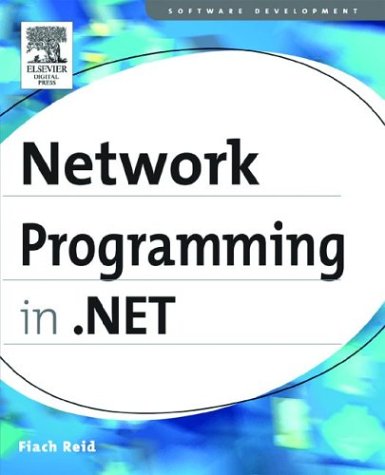
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Condition.cs
- TextServicesManager.cs
- Bits.cs
- ErrorStyle.cs
- ListControlConvertEventArgs.cs
- HandlerMappingMemo.cs
- WorkerRequest.cs
- CheckedListBox.cs
- TextParagraphCache.cs
- QueryTreeBuilder.cs
- StatusBar.cs
- SystemUnicastIPAddressInformation.cs
- NavigatorInvalidBodyAccessException.cs
- Application.cs
- KeyboardEventArgs.cs
- DataBindingList.cs
- CacheDependency.cs
- WrappedReader.cs
- FrameworkPropertyMetadata.cs
- MouseButton.cs
- RegularExpressionValidator.cs
- ObjectSet.cs
- ExpandSegmentCollection.cs
- GeometryGroup.cs
- ConfigurationSectionGroupCollection.cs
- UnconditionalPolicy.cs
- SingleAnimationBase.cs
- QueryCursorEventArgs.cs
- Attributes.cs
- SingleKeyFrameCollection.cs
- StorageModelBuildProvider.cs
- IntSumAggregationOperator.cs
- QilStrConcatenator.cs
- XmlSchemaInferenceException.cs
- ListView.cs
- DoubleKeyFrameCollection.cs
- GeometryGroup.cs
- TemplateXamlTreeBuilder.cs
- TrustManagerPromptUI.cs
- ContractType.cs
- SupportsPreviewControlAttribute.cs
- TargetException.cs
- ArcSegment.cs
- InnerItemCollectionView.cs
- SystemIPv6InterfaceProperties.cs
- SchemaImporterExtension.cs
- OleDbWrapper.cs
- NamespaceMapping.cs
- BufferedReceiveManager.cs
- TogglePatternIdentifiers.cs
- AsyncCompletedEventArgs.cs
- RectConverter.cs
- ZipIOModeEnforcingStream.cs
- HttpCachePolicyWrapper.cs
- ListSortDescription.cs
- EventMap.cs
- AsyncOperationManager.cs
- ElementHostAutomationPeer.cs
- ProfileBuildProvider.cs
- RuntimeArgumentHandle.cs
- View.cs
- NullEntityWrapper.cs
- TreeNodeMouseHoverEvent.cs
- InvalidFilterCriteriaException.cs
- DataServiceRequestOfT.cs
- StringAnimationUsingKeyFrames.cs
- UpdatePanelTriggerCollection.cs
- OleDbInfoMessageEvent.cs
- _ListenerAsyncResult.cs
- PublisherIdentityPermission.cs
- ExpressionBuilder.cs
- PaperSize.cs
- Geometry3D.cs
- DoubleAnimationClockResource.cs
- DataControlFieldCell.cs
- TabRenderer.cs
- Viewport3DAutomationPeer.cs
- ItemChangedEventArgs.cs
- WindowsIdentity.cs
- FilteredAttributeCollection.cs
- InvalidCommandTreeException.cs
- PeerApplication.cs
- NumberFormatter.cs
- ScrollChrome.cs
- XmlAttributeOverrides.cs
- SessionStateContainer.cs
- AssemblyInfo.cs
- DataKeyCollection.cs
- TargetParameterCountException.cs
- BrowserCapabilitiesFactoryBase.cs
- EntityDataSourceColumn.cs
- MarkupObject.cs
- DataGridRow.cs
- DescendentsWalker.cs
- Wizard.cs
- CaseInsensitiveComparer.cs
- FilteredDataSetHelper.cs
- DataGrid.cs
- CodeTypeReferenceCollection.cs
- CustomAttributeFormatException.cs