Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / SystemBrushes.cs / 1 / SystemBrushes.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing { using System.Diagnostics; using System; ////// /// Brushes for select Windows system-wide colors. Whenever possible, try to use /// SystemPens and SystemBrushes rather than SystemColors. /// public sealed class SystemBrushes { static readonly object SystemBrushesKey = new object(); private SystemBrushes() { } ////// /// Brush is the color of the active window border. /// public static Brush ActiveBorder { get { return FromSystemColor(SystemColors.ActiveBorder); } } ////// /// Brush is the color of the active caption bar. /// public static Brush ActiveCaption { get { return FromSystemColor(SystemColors.ActiveCaption); } } ////// /// Brush is the color of the active caption bar. /// public static Brush ActiveCaptionText { get { return FromSystemColor(SystemColors.ActiveCaptionText); } } ////// /// Brush is the color of the app workspace window. /// public static Brush AppWorkspace { get { return FromSystemColor(SystemColors.AppWorkspace); } } ////// /// Brush for the ButtonFace system color. /// public static Brush ButtonFace { get { return FromSystemColor(SystemColors.ButtonFace); } } ////// /// Brush for the ButtonHighlight system color. /// public static Brush ButtonHighlight { get { return FromSystemColor(SystemColors.ButtonHighlight); } } ////// /// Brush for the ButtonShadow system color. /// public static Brush ButtonShadow { get { return FromSystemColor(SystemColors.ButtonShadow); } } ////// /// Brush is the control color, which is the surface color for 3D elements. /// public static Brush Control { get { return FromSystemColor(SystemColors.Control); } } ////// /// Brush is the lighest part of a 3D element. /// public static Brush ControlLightLight { get { return FromSystemColor(SystemColors.ControlLightLight); } } ////// /// Brush is the highlight part of a 3D element. /// public static Brush ControlLight { get { return FromSystemColor(SystemColors.ControlLight); } } ////// /// Brush is the shadow part of a 3D element. /// public static Brush ControlDark { get { return FromSystemColor(SystemColors.ControlDark); } } ////// /// Brush is the darkest part of a 3D element. /// public static Brush ControlDarkDark { get { return FromSystemColor(SystemColors.ControlDarkDark); } } ////// /// Brush is the color of text on controls. /// public static Brush ControlText { get { return FromSystemColor(SystemColors.ControlText); } } ////// /// Brush is the color of the desktop. /// public static Brush Desktop { get { return FromSystemColor(SystemColors.Desktop); } } ////// /// Brush for the GradientActiveCaption system color. /// public static Brush GradientActiveCaption { get { return FromSystemColor(SystemColors.GradientActiveCaption); } } ////// /// Brush for the GradientInactiveCaption system color. /// public static Brush GradientInactiveCaption { get { return FromSystemColor(SystemColors.GradientInactiveCaption); } } ////// /// Brush for the GrayText system color. /// public static Brush GrayText { get { return FromSystemColor(SystemColors.GrayText); } } ////// /// Brush is the color of the background of highlighted elements. /// public static Brush Highlight { get { return FromSystemColor(SystemColors.Highlight); } } ////// /// Brush is the color of the foreground of highlighted elements. /// public static Brush HighlightText { get { return FromSystemColor(SystemColors.HighlightText); } } ////// /// Brush is the color used to represent hot tracking. /// public static Brush HotTrack { get { return FromSystemColor(SystemColors.HotTrack); } } ////// /// Brush is the color of an inactive caption bar. /// public static Brush InactiveCaption { get { return FromSystemColor(SystemColors.InactiveCaption); } } ////// /// Brush is the color if an inactive window border. /// public static Brush InactiveBorder { get { return FromSystemColor(SystemColors.InactiveBorder); } } ////// /// Brush is the color of an inactive caption text. /// public static Brush InactiveCaptionText { get { return FromSystemColor(SystemColors.InactiveCaptionText); } } ////// /// Brush is the color of the background of the info tooltip. /// public static Brush Info { get { return FromSystemColor(SystemColors.Info); } } ////// /// Brush is the color of the info tooltip's text. /// public static Brush InfoText { get { return FromSystemColor(SystemColors.InfoText); } } ////// /// Brush is the color of the menu background. /// public static Brush Menu { get { return FromSystemColor(SystemColors.Menu); } } ////// /// Brush is the color of the menu background. /// public static Brush MenuBar { get { return FromSystemColor(SystemColors.MenuBar); } } ////// /// Brush for the MenuHighlight system color. /// public static Brush MenuHighlight { get { return FromSystemColor(SystemColors.MenuHighlight); } } ////// /// Brush is the color of the menu text. /// public static Brush MenuText { get { return FromSystemColor(SystemColors.MenuText); } } ////// /// Brush is the color of the scroll bar area that is not being used by the /// thumb button. /// public static Brush ScrollBar { get { return FromSystemColor(SystemColors.ScrollBar); } } ////// /// Brush is the color of the window background. /// public static Brush Window { get { return FromSystemColor(SystemColors.Window); } } ////// /// Brush is the color of the thin frame drawn around a window. /// public static Brush WindowFrame { get { return FromSystemColor(SystemColors.WindowFrame); } } ////// /// Brush is the color of text on controls. /// public static Brush WindowText { get { return FromSystemColor(SystemColors.WindowText); } } ////// /// Retrieves a brush given a system color. An error will be raised /// if the color provide is not a system color. /// public static Brush FromSystemColor(Color c) { if (!c.IsSystemColor) { throw new ArgumentException(SR.GetString(SR.ColorNotSystemColor, c.ToString())); } Brush[] systemBrushes = (Brush[])SafeNativeMethods.Gdip.ThreadData[SystemBrushesKey]; if (systemBrushes == null) { systemBrushes = new Brush[(int)KnownColor.WindowText + (int)KnownColor.MenuHighlight - (int)KnownColor.YellowGreen]; SafeNativeMethods.Gdip.ThreadData[SystemBrushesKey] = systemBrushes; } int idx = (int)c.ToKnownColor(); if (idx > (int)KnownColor.YellowGreen) { idx -= (int)KnownColor.YellowGreen - (int)KnownColor.WindowText; } idx--; Debug.Assert(idx >= 0 && idx < systemBrushes.Length, "System colors have been added but our system color array has not been expanded."); if (systemBrushes[idx] == null) { systemBrushes[idx] = new SolidBrush(c, true); } return systemBrushes[idx]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing { using System.Diagnostics; using System; ////// /// Brushes for select Windows system-wide colors. Whenever possible, try to use /// SystemPens and SystemBrushes rather than SystemColors. /// public sealed class SystemBrushes { static readonly object SystemBrushesKey = new object(); private SystemBrushes() { } ////// /// Brush is the color of the active window border. /// public static Brush ActiveBorder { get { return FromSystemColor(SystemColors.ActiveBorder); } } ////// /// Brush is the color of the active caption bar. /// public static Brush ActiveCaption { get { return FromSystemColor(SystemColors.ActiveCaption); } } ////// /// Brush is the color of the active caption bar. /// public static Brush ActiveCaptionText { get { return FromSystemColor(SystemColors.ActiveCaptionText); } } ////// /// Brush is the color of the app workspace window. /// public static Brush AppWorkspace { get { return FromSystemColor(SystemColors.AppWorkspace); } } ////// /// Brush for the ButtonFace system color. /// public static Brush ButtonFace { get { return FromSystemColor(SystemColors.ButtonFace); } } ////// /// Brush for the ButtonHighlight system color. /// public static Brush ButtonHighlight { get { return FromSystemColor(SystemColors.ButtonHighlight); } } ////// /// Brush for the ButtonShadow system color. /// public static Brush ButtonShadow { get { return FromSystemColor(SystemColors.ButtonShadow); } } ////// /// Brush is the control color, which is the surface color for 3D elements. /// public static Brush Control { get { return FromSystemColor(SystemColors.Control); } } ////// /// Brush is the lighest part of a 3D element. /// public static Brush ControlLightLight { get { return FromSystemColor(SystemColors.ControlLightLight); } } ////// /// Brush is the highlight part of a 3D element. /// public static Brush ControlLight { get { return FromSystemColor(SystemColors.ControlLight); } } ////// /// Brush is the shadow part of a 3D element. /// public static Brush ControlDark { get { return FromSystemColor(SystemColors.ControlDark); } } ////// /// Brush is the darkest part of a 3D element. /// public static Brush ControlDarkDark { get { return FromSystemColor(SystemColors.ControlDarkDark); } } ////// /// Brush is the color of text on controls. /// public static Brush ControlText { get { return FromSystemColor(SystemColors.ControlText); } } ////// /// Brush is the color of the desktop. /// public static Brush Desktop { get { return FromSystemColor(SystemColors.Desktop); } } ////// /// Brush for the GradientActiveCaption system color. /// public static Brush GradientActiveCaption { get { return FromSystemColor(SystemColors.GradientActiveCaption); } } ////// /// Brush for the GradientInactiveCaption system color. /// public static Brush GradientInactiveCaption { get { return FromSystemColor(SystemColors.GradientInactiveCaption); } } ////// /// Brush for the GrayText system color. /// public static Brush GrayText { get { return FromSystemColor(SystemColors.GrayText); } } ////// /// Brush is the color of the background of highlighted elements. /// public static Brush Highlight { get { return FromSystemColor(SystemColors.Highlight); } } ////// /// Brush is the color of the foreground of highlighted elements. /// public static Brush HighlightText { get { return FromSystemColor(SystemColors.HighlightText); } } ////// /// Brush is the color used to represent hot tracking. /// public static Brush HotTrack { get { return FromSystemColor(SystemColors.HotTrack); } } ////// /// Brush is the color of an inactive caption bar. /// public static Brush InactiveCaption { get { return FromSystemColor(SystemColors.InactiveCaption); } } ////// /// Brush is the color if an inactive window border. /// public static Brush InactiveBorder { get { return FromSystemColor(SystemColors.InactiveBorder); } } ////// /// Brush is the color of an inactive caption text. /// public static Brush InactiveCaptionText { get { return FromSystemColor(SystemColors.InactiveCaptionText); } } ////// /// Brush is the color of the background of the info tooltip. /// public static Brush Info { get { return FromSystemColor(SystemColors.Info); } } ////// /// Brush is the color of the info tooltip's text. /// public static Brush InfoText { get { return FromSystemColor(SystemColors.InfoText); } } ////// /// Brush is the color of the menu background. /// public static Brush Menu { get { return FromSystemColor(SystemColors.Menu); } } ////// /// Brush is the color of the menu background. /// public static Brush MenuBar { get { return FromSystemColor(SystemColors.MenuBar); } } ////// /// Brush for the MenuHighlight system color. /// public static Brush MenuHighlight { get { return FromSystemColor(SystemColors.MenuHighlight); } } ////// /// Brush is the color of the menu text. /// public static Brush MenuText { get { return FromSystemColor(SystemColors.MenuText); } } ////// /// Brush is the color of the scroll bar area that is not being used by the /// thumb button. /// public static Brush ScrollBar { get { return FromSystemColor(SystemColors.ScrollBar); } } ////// /// Brush is the color of the window background. /// public static Brush Window { get { return FromSystemColor(SystemColors.Window); } } ////// /// Brush is the color of the thin frame drawn around a window. /// public static Brush WindowFrame { get { return FromSystemColor(SystemColors.WindowFrame); } } ////// /// Brush is the color of text on controls. /// public static Brush WindowText { get { return FromSystemColor(SystemColors.WindowText); } } ////// /// Retrieves a brush given a system color. An error will be raised /// if the color provide is not a system color. /// public static Brush FromSystemColor(Color c) { if (!c.IsSystemColor) { throw new ArgumentException(SR.GetString(SR.ColorNotSystemColor, c.ToString())); } Brush[] systemBrushes = (Brush[])SafeNativeMethods.Gdip.ThreadData[SystemBrushesKey]; if (systemBrushes == null) { systemBrushes = new Brush[(int)KnownColor.WindowText + (int)KnownColor.MenuHighlight - (int)KnownColor.YellowGreen]; SafeNativeMethods.Gdip.ThreadData[SystemBrushesKey] = systemBrushes; } int idx = (int)c.ToKnownColor(); if (idx > (int)KnownColor.YellowGreen) { idx -= (int)KnownColor.YellowGreen - (int)KnownColor.WindowText; } idx--; Debug.Assert(idx >= 0 && idx < systemBrushes.Length, "System colors have been added but our system color array has not been expanded."); if (systemBrushes[idx] == null) { systemBrushes[idx] = new SolidBrush(c, true); } return systemBrushes[idx]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
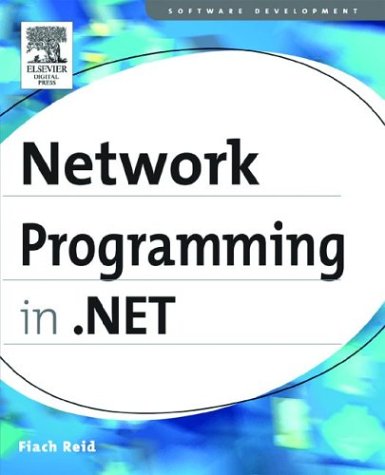
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EdmToObjectNamespaceMap.cs
- WsdlEndpointConversionContext.cs
- XmlException.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- OdbcConnectionFactory.cs
- webeventbuffer.cs
- DispatcherHookEventArgs.cs
- Avt.cs
- ImagingCache.cs
- StoryFragments.cs
- StickyNote.cs
- ContentTextAutomationPeer.cs
- WindowsGraphicsCacheManager.cs
- XmlTextAttribute.cs
- PopupRootAutomationPeer.cs
- TargetException.cs
- ProviderSettingsCollection.cs
- TargetPerspective.cs
- FunctionUpdateCommand.cs
- KeyValueSerializer.cs
- XmlStreamNodeWriter.cs
- CriticalHandle.cs
- RemotingConfigParser.cs
- Menu.cs
- GlobalizationSection.cs
- filewebresponse.cs
- NativeRightsManagementAPIsStructures.cs
- Bold.cs
- SqlDataSourceStatusEventArgs.cs
- BindableTemplateBuilder.cs
- AppearanceEditorPart.cs
- IdentifierElement.cs
- TextElementEnumerator.cs
- TypedReference.cs
- sortedlist.cs
- OrderingQueryOperator.cs
- ConnectionManager.cs
- SystemIPGlobalProperties.cs
- StateChangeEvent.cs
- IDQuery.cs
- SqlDataSourceView.cs
- ConfigurationErrorsException.cs
- DropSource.cs
- FontSource.cs
- QuaternionConverter.cs
- Random.cs
- RuntimeConfigLKG.cs
- FlowDocumentReaderAutomationPeer.cs
- SafeMemoryMappedFileHandle.cs
- DataSet.cs
- GroupStyle.cs
- CollectionConverter.cs
- OlePropertyStructs.cs
- HttpRawResponse.cs
- ZipIOLocalFileBlock.cs
- XmlDataSourceView.cs
- BrushValueSerializer.cs
- WebPartsPersonalizationAuthorization.cs
- DataGridViewColumn.cs
- Point4D.cs
- XamlFilter.cs
- SmtpNtlmAuthenticationModule.cs
- VariableQuery.cs
- NotificationContext.cs
- ZeroOpNode.cs
- EntityDataSourceDataSelection.cs
- UrlAuthorizationModule.cs
- LayoutUtils.cs
- TraceSection.cs
- TextTreeUndoUnit.cs
- FileEnumerator.cs
- securitymgrsite.cs
- SelfIssuedTokenFactoryCredential.cs
- HostnameComparisonMode.cs
- FloaterParaClient.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- OracleDataReader.cs
- ConsoleTraceListener.cs
- TableLayoutPanelCodeDomSerializer.cs
- MediaEntryAttribute.cs
- DataGridViewCellParsingEventArgs.cs
- PropertyRef.cs
- Comparer.cs
- ObsoleteAttribute.cs
- SemaphoreFullException.cs
- NameNode.cs
- StructuredTypeEmitter.cs
- CustomAttribute.cs
- DataGridAutoFormatDialog.cs
- DivideByZeroException.cs
- BasicCommandTreeVisitor.cs
- CalendarDateChangedEventArgs.cs
- FtpWebRequest.cs
- CodeIdentifiers.cs
- ModuleElement.cs
- DataStreams.cs
- GroupBox.cs
- TypeDelegator.cs
- XmlSchemaFacet.cs
- SyncMethodInvoker.cs