Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / SyncMethodInvoker.cs / 1 / SyncMethodInvoker.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.Reflection; using System.ServiceModel.Diagnostics; using System.Globalization; using System.Threading; using System.Collections; using System.Diagnostics; using System.Security; class SyncMethodInvoker : IOperationInvoker { Type type; string methodName; MethodInfo method; InvokeDelegate invokeDelegate; int inputParameterCount; int outputParameterCount; public SyncMethodInvoker(MethodInfo method) { if (method == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("method")); this.method = method; } public SyncMethodInvoker(Type type, string methodName) { if (type == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("type")); if (methodName == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("methodName")); this.type = type; this.methodName = methodName; } public bool IsSynchronous { get { return true; } } public MethodInfo Method { get { if (method == null) method = type.GetMethod(methodName); return method; } } public string MethodName { get { if (methodName == null) methodName = method.Name; return methodName; } } public object[] AllocateInputs() { EnsureIsInitialized(); return EmptyArray.Allocate(this.inputParameterCount); } public object Invoke(object instance, object[] inputs, out object[] outputs) { EnsureIsInitialized(); if (instance == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.SFxNoServiceObject))); if (inputs == null) { if (this.inputParameterCount > 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.SFxInputParametersToServiceNull, this.inputParameterCount))); } else if (inputs.Length != this.inputParameterCount) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.SFxInputParametersToServiceInvalid, this.inputParameterCount, inputs.Length))); outputs = EmptyArray.Allocate(this.outputParameterCount); long startCounter = 0; long stopCounter = 0; bool callFailed = true; bool callFaulted = false; if (PerformanceCounters.PerformanceCountersEnabled) { PerformanceCounters.MethodCalled(this.MethodName); try { if (System.ServiceModel.Channels.UnsafeNativeMethods.QueryPerformanceCounter(out startCounter) == 0) { startCounter = -1; } } catch (SecurityException securityException) { if (DiagnosticUtility.ShouldTraceWarning) { DiagnosticUtility.ExceptionUtility.TraceHandledException(securityException, TraceEventType.Warning); } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SecurityException(SR.GetString( SR.PartialTrustPerformanceCountersNotEnabled), securityException)); } } object returnValue; try { ServiceModelActivity activity = null; IDisposable boundActivity = null; if (DiagnosticUtility.ShouldUseActivity) { activity = ServiceModelActivity.CreateBoundedActivity(true); boundActivity = activity; } else if (TraceUtility.ShouldPropagateActivity) { Guid activityId = ActivityIdHeader.ExtractActivityId(OperationContext.Current.IncomingMessage); if (activityId != Guid.Empty) { boundActivity = Activity.CreateActivity(activityId); } } using (boundActivity) { if (DiagnosticUtility.ShouldUseActivity) { ServiceModelActivity.Start(activity, SR.GetString(SR.ActivityExecuteMethod, this.method.DeclaringType.FullName, this.method.Name), ActivityType.ExecuteUserCode); } returnValue = this.invokeDelegate(instance, inputs, outputs); callFailed = false; } } catch (System.ServiceModel.FaultException) { callFaulted = true; callFailed = false; throw; } catch (System.Security.SecurityException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(AuthorizationBehavior.CreateAccessDeniedFaultException()); } finally { if (PerformanceCounters.PerformanceCountersEnabled) { if (callFailed) // call succeeded { PerformanceCounters.MethodReturnedError(this.MethodName); } else if (callFaulted) // call faulted { PerformanceCounters.MethodReturnedFault(this.MethodName); } else // call errored { long elapsedTime; if (startCounter >= 0 && System.ServiceModel.Channels.UnsafeNativeMethods.QueryPerformanceCounter(out stopCounter) != 0) { elapsedTime = stopCounter - startCounter; } else { elapsedTime = 0; } PerformanceCounters.MethodReturnedSuccess(this.MethodName, elapsedTime); } } } return returnValue; } public IAsyncResult InvokeBegin(object instance, object[] inputs, AsyncCallback callback, object state) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public object InvokeEnd(object instance, out object[] outputs, IAsyncResult result) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } void EnsureIsInitialized() { if (this.invokeDelegate == null) { EnsureIsInitializedCore(); } } void EnsureIsInitializedCore() { // Only pass locals byref because InvokerUtil may store temporary results in the byref. // If two threads both reference this.count, temporary results may interact. int inputParameterCount; int outputParameterCount; InvokeDelegate invokeDelegate = new InvokerUtil().GenerateInvokeDelegate(this.Method, out inputParameterCount, out outputParameterCount); this.outputParameterCount = outputParameterCount; this.inputParameterCount = inputParameterCount; this.invokeDelegate = invokeDelegate; // must set this last due to race } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
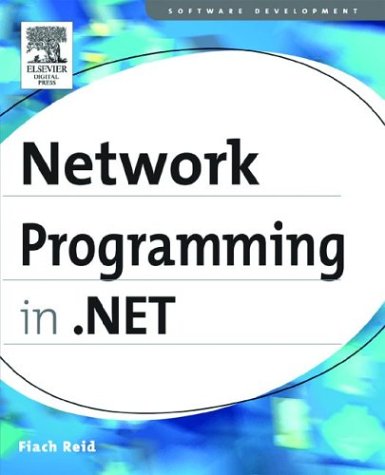
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CheckBoxRenderer.cs
- PrintController.cs
- Control.cs
- AbstractDataSvcMapFileLoader.cs
- Int32CollectionConverter.cs
- BindingExpressionUncommonField.cs
- PageContentAsyncResult.cs
- Rights.cs
- BindingSourceDesigner.cs
- RegisteredExpandoAttribute.cs
- TreeViewImageIndexConverter.cs
- Pens.cs
- WsdlBuildProvider.cs
- SourceChangedEventArgs.cs
- MSAANativeProvider.cs
- PagerSettings.cs
- TextBoxRenderer.cs
- TTSEngineProxy.cs
- SetterBase.cs
- RemoteX509AsymmetricSecurityKey.cs
- DetailsViewPageEventArgs.cs
- SourceFileBuildProvider.cs
- MethodBuilder.cs
- SQLGuid.cs
- FigureHelper.cs
- QuadTree.cs
- SecurityProtocolFactory.cs
- HttpModule.cs
- AssemblyCollection.cs
- FormViewPagerRow.cs
- SessionIDManager.cs
- SerialPort.cs
- Int32AnimationUsingKeyFrames.cs
- HttpModuleAction.cs
- WorkflowMarkupElementEventArgs.cs
- DataMisalignedException.cs
- OrderedDictionary.cs
- SoapObjectWriter.cs
- GeometryDrawing.cs
- MatrixTransform3D.cs
- Dispatcher.cs
- ResourcesChangeInfo.cs
- XDeferredAxisSource.cs
- Int64.cs
- ItemTypeToolStripMenuItem.cs
- WindowsListViewGroupSubsetLink.cs
- SoapMessage.cs
- WaitHandle.cs
- TemplateManager.cs
- UserControlDocumentDesigner.cs
- EntityDataSource.cs
- EasingKeyFrames.cs
- ForeignConstraint.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- EdmComplexTypeAttribute.cs
- NullableDecimalMinMaxAggregationOperator.cs
- BindingEntityInfo.cs
- MouseBinding.cs
- SimpleTextLine.cs
- ObjectDataSourceFilteringEventArgs.cs
- ErrorTableItemStyle.cs
- StickyNoteAnnotations.cs
- NameValueSectionHandler.cs
- NetworkInformationException.cs
- ReaderContextStackData.cs
- PlatformCulture.cs
- FileVersionInfo.cs
- SqlXmlStorage.cs
- StringDictionary.cs
- SiteMembershipCondition.cs
- MimeParameterWriter.cs
- DataGridViewElement.cs
- HttpBrowserCapabilitiesWrapper.cs
- HtmlProps.cs
- Helper.cs
- UrlPath.cs
- ExtensionSimplifierMarkupObject.cs
- XmlSortKeyAccumulator.cs
- InkCanvasSelection.cs
- SimpleTextLine.cs
- DataGridCheckBoxColumn.cs
- FileAuthorizationModule.cs
- RewritingPass.cs
- TextBoxBase.cs
- Color.cs
- CodeAttachEventStatement.cs
- ResourceAttributes.cs
- XmlCodeExporter.cs
- ServiceHostFactory.cs
- DataGridViewRowPostPaintEventArgs.cs
- DBSqlParserColumn.cs
- MethodCallTranslator.cs
- ServiceDeploymentInfo.cs
- Decorator.cs
- HostnameComparisonMode.cs
- RequestValidator.cs
- StringConverter.cs
- AssemblySettingAttributes.cs
- StsCommunicationException.cs
- PropertySourceInfo.cs