Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Automation / Peers / FlowDocumentReaderAutomationPeer.cs / 1305600 / FlowDocumentReaderAutomationPeer.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: FlowDocumentReaderAutomationPeer.cs // // Description: AutomationPeer associated with FlowDocumentReader. // //--------------------------------------------------------------------------- using System.Collections.Generic; // Listusing System.Windows.Automation.Provider; // IMultipleViewProvider using System.Windows.Controls; // FlowDocumentReader using System.Windows.Documents; // FlowDocument using MS.Internal; // Invariant namespace System.Windows.Automation.Peers { /// /// AutomationPeer associated with FlowDocumentScrollViewer. /// public class FlowDocumentReaderAutomationPeer : FrameworkElementAutomationPeer, IMultipleViewProvider { ////// Constructor. /// /// Owner of the AutomationPeer. public FlowDocumentReaderAutomationPeer(FlowDocumentReader owner) : base(owner) { } ////// public override object GetPattern(PatternInterface patternInterface) { object returnValue = null; if (patternInterface == PatternInterface.MultipleView) { returnValue = this; } else { returnValue = base.GetPattern(patternInterface); } return returnValue; } ////// /// ////// /// AutomationPeer associated with FlowDocumentScrollViewer returns an AutomationPeer /// for hosted Document and for elements in the style. /// protected override ListGetChildrenCore() { // Get children for all elements in the style. List children = base.GetChildrenCore(); // Add AutomationPeer associated with the document. // Make it the first child of the collection. FlowDocument document = ((FlowDocumentReader)Owner).Document; if (document != null) { AutomationPeer documentPeer = ContentElementAutomationPeer.CreatePeerForElement(document); if (_documentPeer != documentPeer) { if (_documentPeer != null) { _documentPeer.OnDisconnected(); } _documentPeer = documentPeer as DocumentAutomationPeer; } if (documentPeer != null) { if (children == null) { children = new List (); } children.Add(documentPeer); } } return children; } /// /// protected override string GetClassNameCore() { return "FlowDocumentReader"; } ////// /// This helper synchronously fires automation PropertyChange event /// in responce to current view mode change. /// // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseCurrentViewChangedEvent(FlowDocumentReaderViewingMode newMode, FlowDocumentReaderViewingMode oldMode) { if (newMode != oldMode) { RaisePropertyChangedEvent(MultipleViewPatternIdentifiers.CurrentViewProperty, ConvertModeToViewId(newMode), ConvertModeToViewId(oldMode)); } } ////// This helper synchronously fires automation PropertyChange event /// in responce to supported views change. /// // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseSupportedViewsChangedEvent(DependencyPropertyChangedEventArgs e) { bool newSingle, oldSingle, newFacing, oldFacing, newScroll, oldScroll; if (e.Property == FlowDocumentReader.IsPageViewEnabledProperty) { newSingle = (bool)e.NewValue; oldSingle = (bool)e.OldValue; newFacing = oldFacing = FlowDocumentReader.IsTwoPageViewEnabled; newScroll = oldScroll = FlowDocumentReader.IsScrollViewEnabled; } else if (e.Property == FlowDocumentReader.IsTwoPageViewEnabledProperty) { newSingle = oldSingle = FlowDocumentReader.IsPageViewEnabled; newFacing = (bool)e.NewValue; oldFacing = (bool)e.OldValue; newScroll = oldScroll = FlowDocumentReader.IsScrollViewEnabled; } else// if (e.Property == FlowDocumentReader.IsScrollViewEnabledProperty) { newSingle = oldSingle = FlowDocumentReader.IsPageViewEnabled; newFacing = oldFacing = FlowDocumentReader.IsTwoPageViewEnabled; newScroll = (bool)e.NewValue; oldScroll = (bool)e.OldValue; } if (newSingle != oldSingle || newFacing != oldFacing || newScroll != oldScroll) { int[] newViews = GetSupportedViews(newSingle, newFacing, newScroll); int[] oldViews = GetSupportedViews(oldSingle, oldFacing, oldScroll); RaisePropertyChangedEvent(MultipleViewPatternIdentifiers.SupportedViewsProperty, newViews, oldViews); } } //------------------------------------------------------------------- // // Private Members // //------------------------------------------------------------------- #region Private Members private int[] GetSupportedViews(bool single, bool facing, bool scroll) { int count = 0; if (single) { count++; } if (facing) { count++; } if (scroll) { count++; } int[] views = count > 0 ? new int[count] : null; count = 0; if (single) { views[count++] = ConvertModeToViewId(FlowDocumentReaderViewingMode.Page); } if (facing) { views[count++] = ConvertModeToViewId(FlowDocumentReaderViewingMode.TwoPage); } if (scroll) { views[count++] = ConvertModeToViewId(FlowDocumentReaderViewingMode.Scroll); } return views; } ////// Converts viewing mode to view id. /// private int ConvertModeToViewId(FlowDocumentReaderViewingMode mode) { return (int)mode; } ////// Converts view id to viewing mode. /// private FlowDocumentReaderViewingMode ConvertViewIdToMode(int viewId) { Invariant.Assert(viewId >= 0 && viewId <= 2); return (FlowDocumentReaderViewingMode)viewId; } ////// FlowDocumentReader associated with the peer. /// private FlowDocumentReader FlowDocumentReader { get { return (FlowDocumentReader)Owner; } } private DocumentAutomationPeer _documentPeer; #endregion Private Members //-------------------------------------------------------------------- // // IMultipleViewProvider Members // //------------------------------------------------------------------- #region IMultipleViewProvider Members ////// string IMultipleViewProvider.GetViewName(int viewId) { string name = string.Empty; if (viewId >= 0 && viewId <= 2) { FlowDocumentReaderViewingMode mode = ConvertViewIdToMode(viewId); if (mode == FlowDocumentReaderViewingMode.Page) { name = SR.Get(SRID.FlowDocumentReader_MultipleViewProvider_PageViewName); } else if (mode == FlowDocumentReaderViewingMode.TwoPage) { name = SR.Get(SRID.FlowDocumentReader_MultipleViewProvider_TwoPageViewName); } else if (mode == FlowDocumentReaderViewingMode.Scroll) { name = SR.Get(SRID.FlowDocumentReader_MultipleViewProvider_ScrollViewName); } } return name; } ////// /// void IMultipleViewProvider.SetCurrentView(int viewId) { if (viewId >= 0 && viewId <= 2) { FlowDocumentReader.ViewingMode = ConvertViewIdToMode(viewId); } } ////// /// int IMultipleViewProvider.CurrentView { get { return ConvertModeToViewId(FlowDocumentReader.ViewingMode); } } ////// /// int[] IMultipleViewProvider.GetSupportedViews() { return GetSupportedViews( FlowDocumentReader.IsPageViewEnabled, FlowDocumentReader.IsTwoPageViewEnabled, FlowDocumentReader.IsScrollViewEnabled); } #endregion IMultipleViewProvider Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
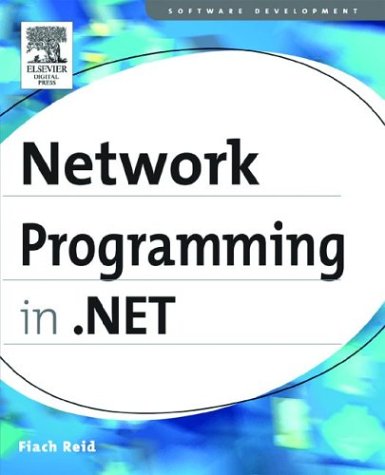
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SchemaImporterExtensionElement.cs
- ProcessRequestArgs.cs
- EncodingInfo.cs
- ZoneIdentityPermission.cs
- TypeLoadException.cs
- ToolboxDataAttribute.cs
- ListViewInsertionMark.cs
- HierarchicalDataSourceControl.cs
- PrimitiveList.cs
- FileAccessException.cs
- Utility.cs
- HelpPage.cs
- XmlLinkedNode.cs
- SizeChangedEventArgs.cs
- GlyphRun.cs
- RuleInfoComparer.cs
- RegisteredHiddenField.cs
- ActionMessageFilter.cs
- Span.cs
- EventSourceCreationData.cs
- JpegBitmapEncoder.cs
- MonthCalendar.cs
- TextRangeProviderWrapper.cs
- DataIdProcessor.cs
- DataControlFieldCollection.cs
- StandardOleMarshalObject.cs
- IntSecurity.cs
- SqlCrossApplyToCrossJoin.cs
- ThreadStaticAttribute.cs
- CFStream.cs
- PropagatorResult.cs
- smtpconnection.cs
- sqlinternaltransaction.cs
- HMACMD5.cs
- XPathDescendantIterator.cs
- ToolBarTray.cs
- Site.cs
- ToolStripRendererSwitcher.cs
- ChangePassword.cs
- XamlUtilities.cs
- WebPartDisplayModeCollection.cs
- GeometryGroup.cs
- MenuAutomationPeer.cs
- PathParser.cs
- RenamedEventArgs.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- LayoutEditorPart.cs
- SettingsBindableAttribute.cs
- DbDataAdapter.cs
- Repeater.cs
- Int32Storage.cs
- LocatorPart.cs
- AffineTransform3D.cs
- FixedSOMPageElement.cs
- Conditional.cs
- ParseHttpDate.cs
- GenericRootAutomationPeer.cs
- SynchronizedReadOnlyCollection.cs
- GZipStream.cs
- BatchParser.cs
- UnionQueryOperator.cs
- EnvelopedPkcs7.cs
- FormClosingEvent.cs
- DataControlField.cs
- NaturalLanguageHyphenator.cs
- ImageBrush.cs
- SerializerProvider.cs
- Assembly.cs
- LocatorGroup.cs
- TextFormatter.cs
- MailAddress.cs
- DataGridViewAccessibleObject.cs
- EncodingDataItem.cs
- ResourceReferenceExpression.cs
- AlphabetConverter.cs
- SettingsAttributes.cs
- ListItemCollection.cs
- ReachSerializer.cs
- Style.cs
- SurrogateDataContract.cs
- CallSiteOps.cs
- FormViewPagerRow.cs
- CookielessHelper.cs
- XPathDocument.cs
- SectionInformation.cs
- GenericUriParser.cs
- XamlGridLengthSerializer.cs
- SecurityTokenProvider.cs
- MessageBox.cs
- OutOfProcStateClientManager.cs
- bindurihelper.cs
- Matrix3DStack.cs
- KeySpline.cs
- RectValueSerializer.cs
- System.Data_BID.cs
- HttpResponseWrapper.cs
- WindowShowOrOpenTracker.cs
- BoundPropertyEntry.cs
- TrackingStringDictionary.cs
- SqlReorderer.cs