Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewElement.cs / 1 / DataGridViewElement.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; ////// /// public class DataGridViewElement { private DataGridViewElementStates state; // enabled frozen readOnly resizable selected visible private DataGridView dataGridView; ///Identifies an element in the dataGridView (base class for TCell, TBand, TRow, TColumn. ////// /// public DataGridViewElement() { this.state = DataGridViewElementStates.Visible; } internal DataGridViewElement(DataGridViewElement dgveTemplate) { // Selected and Displayed states are not inherited this.state = dgveTemplate.State & (DataGridViewElementStates.Frozen | DataGridViewElementStates.ReadOnly | DataGridViewElementStates.Resizable | DataGridViewElementStates.ResizableSet | DataGridViewElementStates.Visible); } ////// Initializes a new instance of the ///class. /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Advanced) ] public virtual DataGridViewElementStates State { get { return this.state; } } internal DataGridViewElementStates StateInternal { set { this.state = value; } } internal bool StateIncludes(DataGridViewElementStates elementState) { return (this.State & elementState) == elementState; } internal bool StateExcludes(DataGridViewElementStates elementState) { return (this.State & elementState) == 0; } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public DataGridView DataGridView { get { return this.dataGridView; } } internal DataGridView DataGridViewInternal { set { if (this.DataGridView != value) { this.dataGridView = value; OnDataGridViewChanged(); } } } /// protected virtual void OnDataGridViewChanged() { } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellClick(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellClickInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellContentClick(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellContentClickInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellContentDoubleClick(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellContentDoubleClickInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellValueChanged(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellValueChangedInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseDataError(DataGridViewDataErrorEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnDataErrorInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseMouseWheel(MouseEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnMouseWheelInternal(e); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; ////// /// public class DataGridViewElement { private DataGridViewElementStates state; // enabled frozen readOnly resizable selected visible private DataGridView dataGridView; ///Identifies an element in the dataGridView (base class for TCell, TBand, TRow, TColumn. ////// /// public DataGridViewElement() { this.state = DataGridViewElementStates.Visible; } internal DataGridViewElement(DataGridViewElement dgveTemplate) { // Selected and Displayed states are not inherited this.state = dgveTemplate.State & (DataGridViewElementStates.Frozen | DataGridViewElementStates.ReadOnly | DataGridViewElementStates.Resizable | DataGridViewElementStates.ResizableSet | DataGridViewElementStates.Visible); } ////// Initializes a new instance of the ///class. /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Advanced) ] public virtual DataGridViewElementStates State { get { return this.state; } } internal DataGridViewElementStates StateInternal { set { this.state = value; } } internal bool StateIncludes(DataGridViewElementStates elementState) { return (this.State & elementState) == elementState; } internal bool StateExcludes(DataGridViewElementStates elementState) { return (this.State & elementState) == 0; } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public DataGridView DataGridView { get { return this.dataGridView; } } internal DataGridView DataGridViewInternal { set { if (this.DataGridView != value) { this.dataGridView = value; OnDataGridViewChanged(); } } } /// protected virtual void OnDataGridViewChanged() { } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellClick(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellClickInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellContentClick(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellContentClickInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellContentDoubleClick(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellContentDoubleClickInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellValueChanged(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellValueChangedInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseDataError(DataGridViewDataErrorEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnDataErrorInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseMouseWheel(MouseEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnMouseWheelInternal(e); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
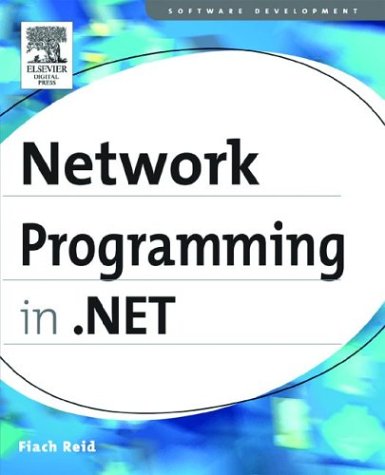
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ElapsedEventArgs.cs
- ListDesigner.cs
- CodeBlockBuilder.cs
- EntityClientCacheEntry.cs
- PrintPageEvent.cs
- NamespaceMapping.cs
- RawTextInputReport.cs
- SqlAggregateChecker.cs
- SimpleWorkerRequest.cs
- UnsafeCollabNativeMethods.cs
- ApplicationServicesHostFactory.cs
- CodeMemberMethod.cs
- ItemsControl.cs
- SettingsBindableAttribute.cs
- AspNetHostingPermission.cs
- StrokeRenderer.cs
- UniqueIdentifierService.cs
- ParentControlDesigner.cs
- AdjustableArrowCap.cs
- MetafileEditor.cs
- EncodingTable.cs
- OrthographicCamera.cs
- ObjectDataSourceMethodEventArgs.cs
- FocusWithinProperty.cs
- ImageIndexConverter.cs
- Context.cs
- SqlTopReducer.cs
- ToolStripComboBox.cs
- DefaultProfileManager.cs
- ListBoxAutomationPeer.cs
- Point.cs
- NativeObjectSecurity.cs
- ElementsClipboardData.cs
- XmlConvert.cs
- GeneralTransform3D.cs
- GridViewColumnHeader.cs
- XpsS0ValidatingLoader.cs
- RandomNumberGenerator.cs
- InvalidCastException.cs
- PropertyGroupDescription.cs
- IntPtr.cs
- BitmapEffectState.cs
- DataServiceRequestException.cs
- ServiceProviders.cs
- VariableQuery.cs
- SerializationInfoEnumerator.cs
- RawKeyboardInputReport.cs
- LiteralControl.cs
- FixUpCollection.cs
- SourceChangedEventArgs.cs
- MetaTableHelper.cs
- SchemaTypeEmitter.cs
- FreeFormDragDropManager.cs
- BindingValueChangedEventArgs.cs
- RawUIStateInputReport.cs
- XmlCharCheckingWriter.cs
- FormatSettings.cs
- SqlResolver.cs
- ResourceDictionary.cs
- XmlDocumentSerializer.cs
- WebPartDisplayModeCancelEventArgs.cs
- Span.cs
- COM2PictureConverter.cs
- ToolStripButton.cs
- SettingsPropertyValueCollection.cs
- ExternalException.cs
- ResourcePermissionBase.cs
- DBNull.cs
- TraversalRequest.cs
- XmlSecureResolver.cs
- InvalidateEvent.cs
- DomainConstraint.cs
- TextEndOfLine.cs
- UnionCqlBlock.cs
- InputReportEventArgs.cs
- ObjectQueryProvider.cs
- IDispatchConstantAttribute.cs
- QuinticEase.cs
- GenericAuthenticationEventArgs.cs
- Separator.cs
- DecimalKeyFrameCollection.cs
- DataBindEngine.cs
- LocalBuilder.cs
- DataTemplateSelector.cs
- TableProviderWrapper.cs
- BatchServiceHost.cs
- IgnoreFlushAndCloseStream.cs
- TerminatorSinks.cs
- WorkflowRuntimeServiceElement.cs
- WebServiceFault.cs
- ServiceOperationHelpers.cs
- AnonymousIdentificationSection.cs
- Context.cs
- TableRowCollection.cs
- Win32.cs
- Connector.cs
- BreakSafeBase.cs
- LinkLabelLinkClickedEvent.cs
- RawStylusInputCustomData.cs
- ParameterElement.cs