Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / ComponentModel / NullableConverter.cs / 1305376 / NullableConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System.Collections; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Runtime.Serialization.Formatters; using System.Security.Permissions; ////// /// [HostProtection(SharedState = true)] public class NullableConverter : TypeConverter { Type nullableType; Type simpleType; TypeConverter simpleTypeConverter; ////// /// public NullableConverter(Type type) { this.nullableType = type; this.simpleType = Nullable.GetUnderlyingType(type); if (this.simpleType == null) { throw new ArgumentException(SR.GetString(SR.NullableConverterBadCtorArg), "type"); } this.simpleTypeConverter = TypeDescriptor.GetConverter(this.simpleType); } ////// /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == this.simpleType) { return true; } else if (this.simpleTypeConverter != null) { return this.simpleTypeConverter.CanConvertFrom(context, sourceType); } else { return base.CanConvertFrom(context, sourceType); } } ////// /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null || value.GetType() == this.simpleType) { return value; } else if (value is String && String.IsNullOrEmpty(value as String)) { return null; } else if (this.simpleTypeConverter != null) { object convertedValue = this.simpleTypeConverter.ConvertFrom(context, culture, value); return convertedValue; } else { return base.ConvertFrom(context, culture, value); } } ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == this.simpleType) { return true; } else if (destinationType == typeof(InstanceDescriptor)) { return true; } else if (this.simpleTypeConverter != null) { return this.simpleTypeConverter.CanConvertTo(context, destinationType); } else { return base.CanConvertTo(context, destinationType); } } ////// /// public override object ConvertTo(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == this.simpleType && this.nullableType.IsInstanceOfType(value)) { return value; } else if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = nullableType.GetConstructor(new Type[] {simpleType}); Debug.Assert(ci != null, "Couldn't find constructor"); return new InstanceDescriptor(ci, new object[] {value}, true); } else if (value == null) { // Handle our own nulls here if (destinationType == typeof(string)) { return string.Empty; } } else if (this.simpleTypeConverter != null) { return this.simpleTypeConverter.ConvertTo(context, culture, value, destinationType); } return base.ConvertTo(context, culture, value, destinationType); } ////// /// public override object CreateInstance(ITypeDescriptorContext context, IDictionary propertyValues) { if (simpleTypeConverter != null) { object instance = simpleTypeConverter.CreateInstance(context, propertyValues); return instance; } return base.CreateInstance(context, propertyValues); } ////// public override bool GetCreateInstanceSupported(ITypeDescriptorContext context) { if (simpleTypeConverter != null) { return simpleTypeConverter.GetCreateInstanceSupported(context); } return base.GetCreateInstanceSupported(context); } ///Gets a value indicating whether changing a value on this object requires a /// call to ///to create a new value, /// using the specified context. /// public override PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object value, Attribute[] attributes) { if (simpleTypeConverter != null) { object unwrappedValue = value; return simpleTypeConverter.GetProperties(context, unwrappedValue, attributes); } return base.GetProperties(context, value, attributes); } ///Gets a collection of properties for /// the type of array specified by the value parameter using the specified context and /// attributes. ////// public override bool GetPropertiesSupported(ITypeDescriptorContext context) { if (simpleTypeConverter != null) { return simpleTypeConverter.GetPropertiesSupported(context); } return base.GetPropertiesSupported(context); } ///Gets a value indicating /// whether this object supports properties using the /// specified context. ////// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (simpleTypeConverter != null) { StandardValuesCollection values = simpleTypeConverter.GetStandardValues(context); if (GetStandardValuesSupported(context) && values != null) { // Create a set of standard values around nullable instances. object[] wrappedValues = new object[values.Count + 1]; int idx = 0; wrappedValues[idx++] = null; foreach(object value in values) { wrappedValues[idx++] = value; } return new StandardValuesCollection(wrappedValues); } } return base.GetStandardValues(context); } ///Gets a collection of standard values for the data type this type converter is /// designed for. ////// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { if (simpleTypeConverter != null) { return simpleTypeConverter.GetStandardValuesExclusive(context); } return base.GetStandardValuesExclusive(context); } ///Gets a value indicating whether the collection of standard values returned from /// ///is an exclusive /// list of possible values, using the specified context. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { if (simpleTypeConverter != null) { return simpleTypeConverter.GetStandardValuesSupported(context); } return base.GetStandardValuesSupported(context); } ///Gets a value indicating /// whether this object /// supports a standard set of values that can be picked /// from a list using the specified context. ////// public override bool IsValid(ITypeDescriptorContext context, object value) { if (simpleTypeConverter != null) { object unwrappedValue = value; if (unwrappedValue == null) { return true; // null is valid for nullable. } else { return simpleTypeConverter.IsValid(context, unwrappedValue); } } return base.IsValid(context, value); } ///Gets /// a value indicating whether the given value object is valid for this type. ////// /// public Type NullableType { get { return nullableType; } } ////// /// public Type UnderlyingType { get { return simpleType; } } ////// /// public TypeConverter UnderlyingTypeConverter { get { return simpleTypeConverter; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
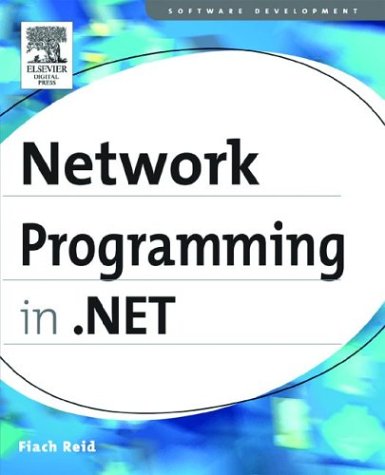
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- designeractionlistschangedeventargs.cs
- XmlObjectSerializerReadContextComplexJson.cs
- LingerOption.cs
- RadioButtonStandardAdapter.cs
- RectangleConverter.cs
- printdlgexmarshaler.cs
- GuidConverter.cs
- DES.cs
- OutputCacheSection.cs
- SoapProcessingBehavior.cs
- NumberFunctions.cs
- Literal.cs
- PropertyTabChangedEvent.cs
- MediaScriptCommandRoutedEventArgs.cs
- Pair.cs
- ConnectionManagementElement.cs
- CodeStatement.cs
- RenderDataDrawingContext.cs
- CheckBoxDesigner.cs
- ValidationErrorEventArgs.cs
- CollectionView.cs
- TokenBasedSetEnumerator.cs
- TextElementEnumerator.cs
- ConvertEvent.cs
- DataChangedEventManager.cs
- Journal.cs
- CngKeyBlobFormat.cs
- UnsafeNativeMethodsCLR.cs
- DataGridColumnFloatingHeader.cs
- SequenceRange.cs
- UrlPath.cs
- XmlDataProvider.cs
- TableCell.cs
- RestHandler.cs
- Brush.cs
- EdmItemError.cs
- ResponseBodyWriter.cs
- QuaternionRotation3D.cs
- TripleDES.cs
- FlowNode.cs
- SchemaTableColumn.cs
- ResourceBinder.cs
- CombinedGeometry.cs
- DataGridViewCellValidatingEventArgs.cs
- IisTraceWebEventProvider.cs
- CollectionEditor.cs
- WindowsButton.cs
- MergeFilterQuery.cs
- ContractMapping.cs
- SqlGatherProducedAliases.cs
- TableLayoutCellPaintEventArgs.cs
- XPathException.cs
- SqlStatistics.cs
- UrlMappingCollection.cs
- BackgroundWorker.cs
- WindowsFormsHostPropertyMap.cs
- TextTreeNode.cs
- SchemaObjectWriter.cs
- TabletDevice.cs
- SettingsAttributes.cs
- IgnorePropertiesAttribute.cs
- RoutedEventConverter.cs
- CodeDirectiveCollection.cs
- MemberInfoSerializationHolder.cs
- EventRecord.cs
- GridItemCollection.cs
- MemberDescriptor.cs
- HttpListenerResponse.cs
- TextCompositionEventArgs.cs
- QuaternionAnimationUsingKeyFrames.cs
- NumberAction.cs
- SqlFacetAttribute.cs
- NumberFormatInfo.cs
- _ListenerResponseStream.cs
- ImageListUtils.cs
- XmlElementList.cs
- SystemColorTracker.cs
- ObjectStorage.cs
- DocumentGridContextMenu.cs
- ExtenderProviderService.cs
- LoginName.cs
- EpmSourceTree.cs
- CapabilitiesUse.cs
- TextServicesCompartmentContext.cs
- RegexStringValidatorAttribute.cs
- Menu.cs
- TranslateTransform3D.cs
- Identity.cs
- XmlWrappingReader.cs
- TextBoxAutoCompleteSourceConverter.cs
- LabelDesigner.cs
- DataGridRelationshipRow.cs
- ParameterElement.cs
- SecurityTokenRequirement.cs
- NegationPusher.cs
- LinqToSqlWrapper.cs
- SEHException.cs
- ObjRef.cs
- CheckBoxList.cs
- DocumentReference.cs