Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Generated / DrawingContextDrawingContextWalker.cs / 1 / DrawingContextDrawingContextWalker.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Effects; using System.Windows.Media.Imaging; using System.Windows.Media.Media3D; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Security; using System.Security.Permissions; namespace System.Windows.Media { ////// DrawingContextDrawingContextWalker is a DrawingContextWalker /// that forwards all of it's calls to a DrawingContext. /// internal partial class DrawingContextDrawingContextWalker: DrawingContextWalker { ////// DrawLine - /// Draws a line with the specified pen. /// Note that this API does not accept a Brush, as there is no area to fill. /// /// The Pen with which to stroke the line. /// The start Point for the line. /// The end Point for the line. public override void DrawLine( Pen pen, Point point0, Point point1) { _drawingContext.DrawLine( pen, point0, point1 ); } ////// DrawLine - /// Draws a line with the specified pen. /// Note that this API does not accept a Brush, as there is no area to fill. /// /// The Pen with which to stroke the line. /// The start Point for the line. /// Optional AnimationClock for point0. /// The end Point for the line. /// Optional AnimationClock for point1. public override void DrawLine( Pen pen, Point point0, AnimationClock point0Animations, Point point1, AnimationClock point1Animations) { _drawingContext.DrawLine( pen, point0, point0Animations, point1, point1Animations ); } ////// DrawRectangle - /// Draw a rectangle with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the rectangle. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the rectangle. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Rect to fill and/or stroke. public override void DrawRectangle( Brush brush, Pen pen, Rect rectangle) { _drawingContext.DrawRectangle( brush, pen, rectangle ); } ////// DrawRectangle - /// Draw a rectangle with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the rectangle. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the rectangle. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Rect to fill and/or stroke. /// Optional AnimationClock for rectangle. public override void DrawRectangle( Brush brush, Pen pen, Rect rectangle, AnimationClock rectangleAnimations) { _drawingContext.DrawRectangle( brush, pen, rectangle, rectangleAnimations ); } ////// DrawRoundedRectangle - /// Draw a rounded rectangle with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the rectangle. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the rectangle. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Rect to fill and/or stroke. /// /// The radius in the X dimension of the rounded corners of this /// rounded Rect. This value will be clamped to the range [0..rectangle.Width/2] /// /// /// The radius in the Y dimension of the rounded corners of this /// rounded Rect. This value will be clamped to the range [0..rectangle.Height/2]. /// public override void DrawRoundedRectangle( Brush brush, Pen pen, Rect rectangle, Double radiusX, Double radiusY) { _drawingContext.DrawRoundedRectangle( brush, pen, rectangle, radiusX, radiusY ); } ////// DrawRoundedRectangle - /// Draw a rounded rectangle with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the rectangle. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the rectangle. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Rect to fill and/or stroke. /// Optional AnimationClock for rectangle. /// /// The radius in the X dimension of the rounded corners of this /// rounded Rect. This value will be clamped to the range [0..rectangle.Width/2] /// /// Optional AnimationClock for radiusX. /// /// The radius in the Y dimension of the rounded corners of this /// rounded Rect. This value will be clamped to the range [0..rectangle.Height/2]. /// /// Optional AnimationClock for radiusY. public override void DrawRoundedRectangle( Brush brush, Pen pen, Rect rectangle, AnimationClock rectangleAnimations, Double radiusX, AnimationClock radiusXAnimations, Double radiusY, AnimationClock radiusYAnimations) { _drawingContext.DrawRoundedRectangle( brush, pen, rectangle, rectangleAnimations, radiusX, radiusXAnimations, radiusY, radiusYAnimations ); } ////// DrawEllipse - /// Draw an ellipse with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the ellipse. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the ellipse. /// This is optional, and can be null, in which case no stroke is performed. /// /// /// The center of the ellipse to fill and/or stroke. /// /// /// The radius in the X dimension of the ellipse. /// The absolute value of the radius provided will be used. /// /// /// The radius in the Y dimension of the ellipse. /// The absolute value of the radius provided will be used. /// public override void DrawEllipse( Brush brush, Pen pen, Point center, Double radiusX, Double radiusY) { _drawingContext.DrawEllipse( brush, pen, center, radiusX, radiusY ); } ////// DrawEllipse - /// Draw an ellipse with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the ellipse. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the ellipse. /// This is optional, and can be null, in which case no stroke is performed. /// /// /// The center of the ellipse to fill and/or stroke. /// /// Optional AnimationClock for center. /// /// The radius in the X dimension of the ellipse. /// The absolute value of the radius provided will be used. /// /// Optional AnimationClock for radiusX. /// /// The radius in the Y dimension of the ellipse. /// The absolute value of the radius provided will be used. /// /// Optional AnimationClock for radiusY. public override void DrawEllipse( Brush brush, Pen pen, Point center, AnimationClock centerAnimations, Double radiusX, AnimationClock radiusXAnimations, Double radiusY, AnimationClock radiusYAnimations) { _drawingContext.DrawEllipse( brush, pen, center, centerAnimations, radiusX, radiusXAnimations, radiusY, radiusYAnimations ); } ////// DrawGeometry - /// Draw a Geometry with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the Geometry. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the Geometry. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Geometry to fill and/or stroke. public override void DrawGeometry( Brush brush, Pen pen, Geometry geometry) { _drawingContext.DrawGeometry( brush, pen, geometry ); } ////// DrawImage - /// Draw an Image into the region specified by the Rect. /// The Image will potentially be stretched and distorted to fit the Rect. /// For more fine grained control, consider filling a Rect with an ImageBrush via /// DrawRectangle. /// /// The ImageSource to draw. /// /// The Rect into which the ImageSource will be fit. /// public override void DrawImage( ImageSource imageSource, Rect rectangle) { _drawingContext.DrawImage( imageSource, rectangle ); } ////// DrawImage - /// Draw an Image into the region specified by the Rect. /// The Image will potentially be stretched and distorted to fit the Rect. /// For more fine grained control, consider filling a Rect with an ImageBrush via /// DrawRectangle. /// /// The ImageSource to draw. /// /// The Rect into which the ImageSource will be fit. /// /// Optional AnimationClock for rectangle. public override void DrawImage( ImageSource imageSource, Rect rectangle, AnimationClock rectangleAnimations) { _drawingContext.DrawImage( imageSource, rectangle, rectangleAnimations ); } ////// DrawGlyphRun - /// Draw a GlyphRun /// /// /// Foreground brush to draw the GlyphRun with. /// /// The GlyphRun to draw. public override void DrawGlyphRun( Brush foregroundBrush, GlyphRun glyphRun) { _drawingContext.DrawGlyphRun( foregroundBrush, glyphRun ); } ////// DrawDrawing - /// Draw a Drawing by appending a sub-Drawing to the current Drawing. /// /// The drawing to draw. public override void DrawDrawing( Drawing drawing) { _drawingContext.DrawDrawing( drawing ); } ////// DrawVideo - /// Draw a Video into the region specified by the Rect. /// The Video will potentially be stretched and distorted to fit the Rect. /// For more fine grained control, consider filling a Rect with an VideoBrush via /// DrawRectangle. /// /// The MediaPlayer to draw. /// The Rect into which the media will be fit. public override void DrawVideo( MediaPlayer player, Rect rectangle) { _drawingContext.DrawVideo( player, rectangle ); } ////// DrawVideo - /// Draw a Video into the region specified by the Rect. /// The Video will potentially be stretched and distorted to fit the Rect. /// For more fine grained control, consider filling a Rect with an VideoBrush via /// DrawRectangle. /// /// The MediaPlayer to draw. /// The Rect into which the media will be fit. /// Optional AnimationClock for rectangle. public override void DrawVideo( MediaPlayer player, Rect rectangle, AnimationClock rectangleAnimations) { _drawingContext.DrawVideo( player, rectangle, rectangleAnimations ); } ////// DrawScene3D - /// Draw a Scene3D (internal object encapsulating a 3D scene) /// /// The Scene3D to draw. internal override void DrawScene3D( Scene3D scene3D) { _drawingContext.DrawScene3D( scene3D ); } ////// PushClip - /// Push a clip region, which will apply to all drawing primitives until the /// corresponding Pop call. /// /// The Geometry to which we will clip. public override void PushClip( Geometry clipGeometry) { _drawingContext.PushClip( clipGeometry ); } ////// PushOpacityMask - /// Push an opacity mask which will blend the composite of all drawing primitives added /// until the corresponding Pop call. /// /// The opacity mask public override void PushOpacityMask( Brush opacityMask) { _drawingContext.PushOpacityMask( opacityMask ); } ////// PushOpacity - /// Push an opacity which will blend the composite of all drawing primitives added /// until the corresponding Pop call. /// /// /// The opacity with which to blend - 0 is transparent, 1 is opaque. /// public override void PushOpacity( Double opacity) { _drawingContext.PushOpacity( opacity ); } ////// PushOpacity - /// Push an opacity which will blend the composite of all drawing primitives added /// until the corresponding Pop call. /// /// /// The opacity with which to blend - 0 is transparent, 1 is opaque. /// /// Optional AnimationClock for opacity. public override void PushOpacity( Double opacity, AnimationClock opacityAnimations) { _drawingContext.PushOpacity( opacity, opacityAnimations ); } ////// PushTransform - /// Push a Transform which will apply to all drawing operations until the corresponding /// Pop. /// /// The Transform to push. public override void PushTransform( Transform transform) { _drawingContext.PushTransform( transform ); } ////// PushGuidelineSet - /// Push a set of guidelines which will apply to all drawing operations until the /// corresponding Pop. /// /// The GuidelineSet to push. public override void PushGuidelineSet( GuidelineSet guidelines) { _drawingContext.PushGuidelineSet( guidelines ); } ////// PushGuidelineY1 - /// Explicitly push one horizontal guideline. /// /// The coordinate of leading guideline. internal override void PushGuidelineY1( Double coordinate) { _drawingContext.PushGuidelineY1( coordinate ); } ////// PushGuidelineY2 - /// Explicitly push a pair of horizontal guidelines. /// /// /// The coordinate of leading guideline. /// /// /// The offset from leading guideline to driven guideline. /// internal override void PushGuidelineY2( Double leadingCoordinate, Double offsetToDrivenCoordinate) { _drawingContext.PushGuidelineY2( leadingCoordinate, offsetToDrivenCoordinate ); } ////// PushEffect - /// Push a BitmapEffect which will apply to all drawing operations until the /// corresponding Pop. /// /// The BitmapEffect to push. /// The BitmapEffectInput. public override void PushEffect( BitmapEffect effect, BitmapEffectInput effectInput) { _drawingContext.PushEffect( effect, effectInput ); } ////// Pop /// public override void Pop( ) { _drawingContext.Pop( ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Effects; using System.Windows.Media.Imaging; using System.Windows.Media.Media3D; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Security; using System.Security.Permissions; namespace System.Windows.Media { ////// DrawingContextDrawingContextWalker is a DrawingContextWalker /// that forwards all of it's calls to a DrawingContext. /// internal partial class DrawingContextDrawingContextWalker: DrawingContextWalker { ////// DrawLine - /// Draws a line with the specified pen. /// Note that this API does not accept a Brush, as there is no area to fill. /// /// The Pen with which to stroke the line. /// The start Point for the line. /// The end Point for the line. public override void DrawLine( Pen pen, Point point0, Point point1) { _drawingContext.DrawLine( pen, point0, point1 ); } ////// DrawLine - /// Draws a line with the specified pen. /// Note that this API does not accept a Brush, as there is no area to fill. /// /// The Pen with which to stroke the line. /// The start Point for the line. /// Optional AnimationClock for point0. /// The end Point for the line. /// Optional AnimationClock for point1. public override void DrawLine( Pen pen, Point point0, AnimationClock point0Animations, Point point1, AnimationClock point1Animations) { _drawingContext.DrawLine( pen, point0, point0Animations, point1, point1Animations ); } ////// DrawRectangle - /// Draw a rectangle with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the rectangle. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the rectangle. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Rect to fill and/or stroke. public override void DrawRectangle( Brush brush, Pen pen, Rect rectangle) { _drawingContext.DrawRectangle( brush, pen, rectangle ); } ////// DrawRectangle - /// Draw a rectangle with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the rectangle. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the rectangle. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Rect to fill and/or stroke. /// Optional AnimationClock for rectangle. public override void DrawRectangle( Brush brush, Pen pen, Rect rectangle, AnimationClock rectangleAnimations) { _drawingContext.DrawRectangle( brush, pen, rectangle, rectangleAnimations ); } ////// DrawRoundedRectangle - /// Draw a rounded rectangle with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the rectangle. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the rectangle. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Rect to fill and/or stroke. /// /// The radius in the X dimension of the rounded corners of this /// rounded Rect. This value will be clamped to the range [0..rectangle.Width/2] /// /// /// The radius in the Y dimension of the rounded corners of this /// rounded Rect. This value will be clamped to the range [0..rectangle.Height/2]. /// public override void DrawRoundedRectangle( Brush brush, Pen pen, Rect rectangle, Double radiusX, Double radiusY) { _drawingContext.DrawRoundedRectangle( brush, pen, rectangle, radiusX, radiusY ); } ////// DrawRoundedRectangle - /// Draw a rounded rectangle with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the rectangle. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the rectangle. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Rect to fill and/or stroke. /// Optional AnimationClock for rectangle. /// /// The radius in the X dimension of the rounded corners of this /// rounded Rect. This value will be clamped to the range [0..rectangle.Width/2] /// /// Optional AnimationClock for radiusX. /// /// The radius in the Y dimension of the rounded corners of this /// rounded Rect. This value will be clamped to the range [0..rectangle.Height/2]. /// /// Optional AnimationClock for radiusY. public override void DrawRoundedRectangle( Brush brush, Pen pen, Rect rectangle, AnimationClock rectangleAnimations, Double radiusX, AnimationClock radiusXAnimations, Double radiusY, AnimationClock radiusYAnimations) { _drawingContext.DrawRoundedRectangle( brush, pen, rectangle, rectangleAnimations, radiusX, radiusXAnimations, radiusY, radiusYAnimations ); } ////// DrawEllipse - /// Draw an ellipse with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the ellipse. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the ellipse. /// This is optional, and can be null, in which case no stroke is performed. /// /// /// The center of the ellipse to fill and/or stroke. /// /// /// The radius in the X dimension of the ellipse. /// The absolute value of the radius provided will be used. /// /// /// The radius in the Y dimension of the ellipse. /// The absolute value of the radius provided will be used. /// public override void DrawEllipse( Brush brush, Pen pen, Point center, Double radiusX, Double radiusY) { _drawingContext.DrawEllipse( brush, pen, center, radiusX, radiusY ); } ////// DrawEllipse - /// Draw an ellipse with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the ellipse. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the ellipse. /// This is optional, and can be null, in which case no stroke is performed. /// /// /// The center of the ellipse to fill and/or stroke. /// /// Optional AnimationClock for center. /// /// The radius in the X dimension of the ellipse. /// The absolute value of the radius provided will be used. /// /// Optional AnimationClock for radiusX. /// /// The radius in the Y dimension of the ellipse. /// The absolute value of the radius provided will be used. /// /// Optional AnimationClock for radiusY. public override void DrawEllipse( Brush brush, Pen pen, Point center, AnimationClock centerAnimations, Double radiusX, AnimationClock radiusXAnimations, Double radiusY, AnimationClock radiusYAnimations) { _drawingContext.DrawEllipse( brush, pen, center, centerAnimations, radiusX, radiusXAnimations, radiusY, radiusYAnimations ); } ////// DrawGeometry - /// Draw a Geometry with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the Geometry. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the Geometry. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Geometry to fill and/or stroke. public override void DrawGeometry( Brush brush, Pen pen, Geometry geometry) { _drawingContext.DrawGeometry( brush, pen, geometry ); } ////// DrawImage - /// Draw an Image into the region specified by the Rect. /// The Image will potentially be stretched and distorted to fit the Rect. /// For more fine grained control, consider filling a Rect with an ImageBrush via /// DrawRectangle. /// /// The ImageSource to draw. /// /// The Rect into which the ImageSource will be fit. /// public override void DrawImage( ImageSource imageSource, Rect rectangle) { _drawingContext.DrawImage( imageSource, rectangle ); } ////// DrawImage - /// Draw an Image into the region specified by the Rect. /// The Image will potentially be stretched and distorted to fit the Rect. /// For more fine grained control, consider filling a Rect with an ImageBrush via /// DrawRectangle. /// /// The ImageSource to draw. /// /// The Rect into which the ImageSource will be fit. /// /// Optional AnimationClock for rectangle. public override void DrawImage( ImageSource imageSource, Rect rectangle, AnimationClock rectangleAnimations) { _drawingContext.DrawImage( imageSource, rectangle, rectangleAnimations ); } ////// DrawGlyphRun - /// Draw a GlyphRun /// /// /// Foreground brush to draw the GlyphRun with. /// /// The GlyphRun to draw. public override void DrawGlyphRun( Brush foregroundBrush, GlyphRun glyphRun) { _drawingContext.DrawGlyphRun( foregroundBrush, glyphRun ); } ////// DrawDrawing - /// Draw a Drawing by appending a sub-Drawing to the current Drawing. /// /// The drawing to draw. public override void DrawDrawing( Drawing drawing) { _drawingContext.DrawDrawing( drawing ); } ////// DrawVideo - /// Draw a Video into the region specified by the Rect. /// The Video will potentially be stretched and distorted to fit the Rect. /// For more fine grained control, consider filling a Rect with an VideoBrush via /// DrawRectangle. /// /// The MediaPlayer to draw. /// The Rect into which the media will be fit. public override void DrawVideo( MediaPlayer player, Rect rectangle) { _drawingContext.DrawVideo( player, rectangle ); } ////// DrawVideo - /// Draw a Video into the region specified by the Rect. /// The Video will potentially be stretched and distorted to fit the Rect. /// For more fine grained control, consider filling a Rect with an VideoBrush via /// DrawRectangle. /// /// The MediaPlayer to draw. /// The Rect into which the media will be fit. /// Optional AnimationClock for rectangle. public override void DrawVideo( MediaPlayer player, Rect rectangle, AnimationClock rectangleAnimations) { _drawingContext.DrawVideo( player, rectangle, rectangleAnimations ); } ////// DrawScene3D - /// Draw a Scene3D (internal object encapsulating a 3D scene) /// /// The Scene3D to draw. internal override void DrawScene3D( Scene3D scene3D) { _drawingContext.DrawScene3D( scene3D ); } ////// PushClip - /// Push a clip region, which will apply to all drawing primitives until the /// corresponding Pop call. /// /// The Geometry to which we will clip. public override void PushClip( Geometry clipGeometry) { _drawingContext.PushClip( clipGeometry ); } ////// PushOpacityMask - /// Push an opacity mask which will blend the composite of all drawing primitives added /// until the corresponding Pop call. /// /// The opacity mask public override void PushOpacityMask( Brush opacityMask) { _drawingContext.PushOpacityMask( opacityMask ); } ////// PushOpacity - /// Push an opacity which will blend the composite of all drawing primitives added /// until the corresponding Pop call. /// /// /// The opacity with which to blend - 0 is transparent, 1 is opaque. /// public override void PushOpacity( Double opacity) { _drawingContext.PushOpacity( opacity ); } ////// PushOpacity - /// Push an opacity which will blend the composite of all drawing primitives added /// until the corresponding Pop call. /// /// /// The opacity with which to blend - 0 is transparent, 1 is opaque. /// /// Optional AnimationClock for opacity. public override void PushOpacity( Double opacity, AnimationClock opacityAnimations) { _drawingContext.PushOpacity( opacity, opacityAnimations ); } ////// PushTransform - /// Push a Transform which will apply to all drawing operations until the corresponding /// Pop. /// /// The Transform to push. public override void PushTransform( Transform transform) { _drawingContext.PushTransform( transform ); } ////// PushGuidelineSet - /// Push a set of guidelines which will apply to all drawing operations until the /// corresponding Pop. /// /// The GuidelineSet to push. public override void PushGuidelineSet( GuidelineSet guidelines) { _drawingContext.PushGuidelineSet( guidelines ); } ////// PushGuidelineY1 - /// Explicitly push one horizontal guideline. /// /// The coordinate of leading guideline. internal override void PushGuidelineY1( Double coordinate) { _drawingContext.PushGuidelineY1( coordinate ); } ////// PushGuidelineY2 - /// Explicitly push a pair of horizontal guidelines. /// /// /// The coordinate of leading guideline. /// /// /// The offset from leading guideline to driven guideline. /// internal override void PushGuidelineY2( Double leadingCoordinate, Double offsetToDrivenCoordinate) { _drawingContext.PushGuidelineY2( leadingCoordinate, offsetToDrivenCoordinate ); } ////// PushEffect - /// Push a BitmapEffect which will apply to all drawing operations until the /// corresponding Pop. /// /// The BitmapEffect to push. /// The BitmapEffectInput. public override void PushEffect( BitmapEffect effect, BitmapEffectInput effectInput) { _drawingContext.PushEffect( effect, effectInput ); } ////// Pop /// public override void Pop( ) { _drawingContext.Pop( ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
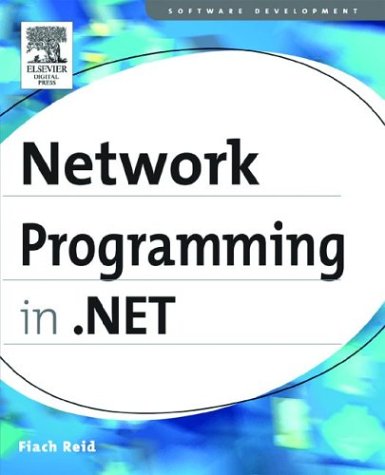
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TokenizerHelper.cs
- FixedSOMPage.cs
- TextureBrush.cs
- ProfileGroupSettings.cs
- MouseCaptureWithinProperty.cs
- IPGlobalProperties.cs
- ReadonlyMessageFilter.cs
- ResourceSet.cs
- BinHexDecoder.cs
- PropertyEmitterBase.cs
- XmlIgnoreAttribute.cs
- ApplicationDirectory.cs
- MarkerProperties.cs
- WaitHandle.cs
- SiteMapSection.cs
- ConfigurationElementProperty.cs
- RegexGroup.cs
- TextRunCacheImp.cs
- XmlConvert.cs
- EditingMode.cs
- Cursors.cs
- Fonts.cs
- AnnotationService.cs
- BitmapEffectState.cs
- SystemGatewayIPAddressInformation.cs
- InputManager.cs
- Brushes.cs
- RoutedPropertyChangedEventArgs.cs
- SByteStorage.cs
- PreviewControlDesigner.cs
- SinglePageViewer.cs
- PackUriHelper.cs
- OutputCacheProviderCollection.cs
- NamedPermissionSet.cs
- OleDbErrorCollection.cs
- SafeArrayTypeMismatchException.cs
- ToolStripItemEventArgs.cs
- MetadataPropertyvalue.cs
- TypeSemantics.cs
- WebBrowserEvent.cs
- FixedDocumentPaginator.cs
- AnimationException.cs
- ListArgumentProvider.cs
- DataBindingList.cs
- UserControl.cs
- CallbackValidatorAttribute.cs
- CompositeScriptReference.cs
- WaitForChangedResult.cs
- ScriptHandlerFactory.cs
- TreeViewImageKeyConverter.cs
- UnSafeCharBuffer.cs
- SchemaNotation.cs
- MultiTargetingUtil.cs
- SafeFileHandle.cs
- TextMarkerSource.cs
- SevenBitStream.cs
- DependencyPropertyHelper.cs
- CodeMethodMap.cs
- EmbeddedMailObjectsCollection.cs
- DbTransaction.cs
- TableRowCollection.cs
- TextServicesHost.cs
- XmlUrlResolver.cs
- Size.cs
- ConfigXmlSignificantWhitespace.cs
- DataRecordObjectView.cs
- UserPreferenceChangedEventArgs.cs
- GetPageCompletedEventArgs.cs
- GatewayDefinition.cs
- ConvertEvent.cs
- Cursor.cs
- SqlDataSourceCommandEventArgs.cs
- Composition.cs
- RootNamespaceAttribute.cs
- DataObjectEventArgs.cs
- ExpressionBuilder.cs
- AttachmentService.cs
- HyperlinkAutomationPeer.cs
- RecognizeCompletedEventArgs.cs
- SqlParameterCollection.cs
- SQLMembershipProvider.cs
- Context.cs
- WindowsFont.cs
- AdapterSwitches.cs
- ContourSegment.cs
- TileBrush.cs
- IssuedTokenClientBehaviorsElementCollection.cs
- DbParameterCollectionHelper.cs
- EntityPropertyMappingAttribute.cs
- MeshGeometry3D.cs
- LocalizabilityAttribute.cs
- AsyncResult.cs
- PersonalizationAdministration.cs
- ResourceDictionaryCollection.cs
- HijriCalendar.cs
- CollectionBuilder.cs
- AttributeCollection.cs
- FixedDocumentPaginator.cs
- SqlBulkCopyColumnMapping.cs
- StateManagedCollection.cs