Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Base / System / Security / RightsManagement / Grant.cs / 1 / Grant.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: This class represents a (ContentUser , ContentRight) pair. // // History: // 06/01/2005: IgorBel : Initial Implementation // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Windows; using MS.Internal.Security.RightsManagement; using SecurityHelper=MS.Internal.WindowsBase.SecurityHelper; namespace System.Security.RightsManagement { ////// ContentGrant class represent a (ContentUser , ContentRight) pair this is /// a basic building block for structures that need to express information about rights granted to a document. /// ////// Critical: This class expose access to methods that eventually do one or more of the the following /// 1. call into unmanaged code /// 2. affects state/data that will eventually cross over unmanaged code boundary /// 3. Return some RM related information which is considered private /// /// TreatAsSafe: This attribute automatically applied to all public entry points. All the public entry points have /// Demands for RightsManagementPermission at entry to counter the possible attacks that do /// not lead to the unmanaged code directly(which is protected by another Demand there) but rather leave /// some status/data behind which eventually might cross the unamanaged boundary. /// [SecurityCritical(SecurityCriticalScope.Everything)] public class ContentGrant { ////// Constructor for the read only ContentGrant class. It takes values for user and right as parameters. /// public ContentGrant(ContentUser user, ContentRight right) : this(user, right, DateTime.MinValue, DateTime.MaxValue) { } ////// Constructor for the read only ContentGrant class. It takes values for /// user, right, validFrom, and validUntil as parameters. /// public ContentGrant(ContentUser user, ContentRight right, DateTime validFrom, DateTime validUntil) { SecurityHelper.DemandRightsManagementPermission(); // Add validation here if (user == null) { throw new ArgumentNullException("user"); } if ((right != ContentRight.View) && (right != ContentRight.Edit) && (right != ContentRight.Print) && (right != ContentRight.Extract) && (right != ContentRight.ObjectModel) && (right != ContentRight.Owner) && (right != ContentRight.ViewRightsData) && (right != ContentRight.Forward) && (right != ContentRight.Reply) && (right != ContentRight.ReplyAll) && (right != ContentRight.Sign) && (right != ContentRight.DocumentEdit) && (right != ContentRight.Export)) { throw new ArgumentOutOfRangeException("right"); } if (validFrom > validUntil) { throw new ArgumentOutOfRangeException("validFrom"); } _user = user; _right = right; _validFrom = validFrom; _validUntil = validUntil; } ////// Read only User propery. /// public ContentUser User { get { SecurityHelper.DemandRightsManagementPermission(); return _user; } } ////// Read only Right propery. /// public ContentRight Right { get { SecurityHelper.DemandRightsManagementPermission(); return _right; } } ////// The starting validity time, in UTC time, for the grant. /// public DateTime ValidFrom { get { SecurityHelper.DemandRightsManagementPermission(); return _validFrom; } } ////// The ending validity time, in UTC time, for the grant. /// public DateTime ValidUntil { get { SecurityHelper.DemandRightsManagementPermission(); return _validUntil; } } private ContentUser _user; private ContentRight _right; private DateTime _validFrom; private DateTime _validUntil; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: This class represents a (ContentUser , ContentRight) pair. // // History: // 06/01/2005: IgorBel : Initial Implementation // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Windows; using MS.Internal.Security.RightsManagement; using SecurityHelper=MS.Internal.WindowsBase.SecurityHelper; namespace System.Security.RightsManagement { ////// ContentGrant class represent a (ContentUser , ContentRight) pair this is /// a basic building block for structures that need to express information about rights granted to a document. /// ////// Critical: This class expose access to methods that eventually do one or more of the the following /// 1. call into unmanaged code /// 2. affects state/data that will eventually cross over unmanaged code boundary /// 3. Return some RM related information which is considered private /// /// TreatAsSafe: This attribute automatically applied to all public entry points. All the public entry points have /// Demands for RightsManagementPermission at entry to counter the possible attacks that do /// not lead to the unmanaged code directly(which is protected by another Demand there) but rather leave /// some status/data behind which eventually might cross the unamanaged boundary. /// [SecurityCritical(SecurityCriticalScope.Everything)] public class ContentGrant { ////// Constructor for the read only ContentGrant class. It takes values for user and right as parameters. /// public ContentGrant(ContentUser user, ContentRight right) : this(user, right, DateTime.MinValue, DateTime.MaxValue) { } ////// Constructor for the read only ContentGrant class. It takes values for /// user, right, validFrom, and validUntil as parameters. /// public ContentGrant(ContentUser user, ContentRight right, DateTime validFrom, DateTime validUntil) { SecurityHelper.DemandRightsManagementPermission(); // Add validation here if (user == null) { throw new ArgumentNullException("user"); } if ((right != ContentRight.View) && (right != ContentRight.Edit) && (right != ContentRight.Print) && (right != ContentRight.Extract) && (right != ContentRight.ObjectModel) && (right != ContentRight.Owner) && (right != ContentRight.ViewRightsData) && (right != ContentRight.Forward) && (right != ContentRight.Reply) && (right != ContentRight.ReplyAll) && (right != ContentRight.Sign) && (right != ContentRight.DocumentEdit) && (right != ContentRight.Export)) { throw new ArgumentOutOfRangeException("right"); } if (validFrom > validUntil) { throw new ArgumentOutOfRangeException("validFrom"); } _user = user; _right = right; _validFrom = validFrom; _validUntil = validUntil; } ////// Read only User propery. /// public ContentUser User { get { SecurityHelper.DemandRightsManagementPermission(); return _user; } } ////// Read only Right propery. /// public ContentRight Right { get { SecurityHelper.DemandRightsManagementPermission(); return _right; } } ////// The starting validity time, in UTC time, for the grant. /// public DateTime ValidFrom { get { SecurityHelper.DemandRightsManagementPermission(); return _validFrom; } } ////// The ending validity time, in UTC time, for the grant. /// public DateTime ValidUntil { get { SecurityHelper.DemandRightsManagementPermission(); return _validUntil; } } private ContentUser _user; private ContentRight _right; private DateTime _validFrom; private DateTime _validUntil; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
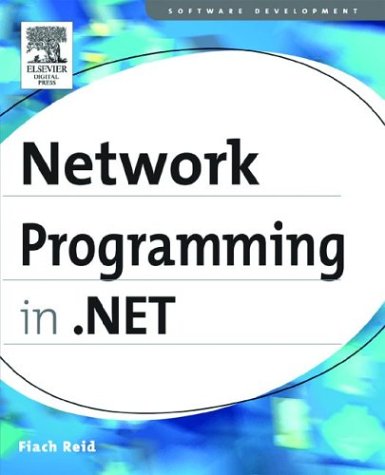
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextFormatter.cs
- AppDomainAttributes.cs
- StandardOleMarshalObject.cs
- PipelineComponent.cs
- EventTrigger.cs
- WorkflowCompensationBehavior.cs
- ExpressionVisitor.cs
- HttpsHostedTransportConfiguration.cs
- CodeParameterDeclarationExpressionCollection.cs
- WmlTextViewAdapter.cs
- SymmetricKeyWrap.cs
- DataGridAddNewRow.cs
- Icon.cs
- ResourcePermissionBaseEntry.cs
- DirectionalLight.cs
- NonParentingControl.cs
- TextContainerChangedEventArgs.cs
- UnmanagedMemoryStreamWrapper.cs
- ScalarOps.cs
- SocketElement.cs
- HtmlInputText.cs
- NumericExpr.cs
- ByteAnimationBase.cs
- DataBindingExpressionBuilder.cs
- StrongName.cs
- PropertyTab.cs
- InputMethodStateChangeEventArgs.cs
- CaseInsensitiveHashCodeProvider.cs
- Emitter.cs
- TriggerAction.cs
- RegexNode.cs
- StylesEditorDialog.cs
- HostingEnvironmentException.cs
- MDIControlStrip.cs
- WindowsListViewItemCheckBox.cs
- CheckBoxPopupAdapter.cs
- CryptoStream.cs
- TreeViewCancelEvent.cs
- CatalogZone.cs
- XmlJsonWriter.cs
- InstanceData.cs
- SurrogateEncoder.cs
- TextView.cs
- XPathNavigator.cs
- BulletChrome.cs
- WorkflowMarkupSerializerMapping.cs
- MetaDataInfo.cs
- SQLStringStorage.cs
- TypeConverters.cs
- NavigationFailedEventArgs.cs
- AccessedThroughPropertyAttribute.cs
- BamlRecords.cs
- DefaultHttpHandler.cs
- AttributeAction.cs
- IteratorFilter.cs
- NGCSerializer.cs
- SiteMap.cs
- ConfigXmlElement.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- BooleanProjectedSlot.cs
- RemoteWebConfigurationHostServer.cs
- CodePropertyReferenceExpression.cs
- __Filters.cs
- ILGenerator.cs
- EntityDataSourceValidationException.cs
- PreProcessInputEventArgs.cs
- safelinkcollection.cs
- Latin1Encoding.cs
- TcpTransportElement.cs
- RepeatButton.cs
- SafePEFileHandle.cs
- ConfigurationSettings.cs
- ServiceAuthorizationBehavior.cs
- RelationshipWrapper.cs
- loginstatus.cs
- XpsSerializerWriter.cs
- InvalidFilterCriteriaException.cs
- UITypeEditor.cs
- InternalResources.cs
- CloseSequenceResponse.cs
- Line.cs
- IndentedTextWriter.cs
- SamlAssertionKeyIdentifierClause.cs
- SizeKeyFrameCollection.cs
- XpsDigitalSignature.cs
- SystemException.cs
- HtmlFormWrapper.cs
- SchemaManager.cs
- ReachUIElementCollectionSerializer.cs
- ThumbAutomationPeer.cs
- WaitHandle.cs
- XmlCompatibilityReader.cs
- FileDialog_Vista_Interop.cs
- ImageAnimator.cs
- EventLogException.cs
- ExpressionEditorAttribute.cs
- HandleTable.cs
- ProtocolsConfiguration.cs
- Listbox.cs
- StylusDownEventArgs.cs