Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / ComplexType.cs / 1305376 / ComplexType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Threading; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// Represent the Edm Complex Type /// public class ComplexType : StructuralType { #region Constructors ////// Initializes a new instance of Complex Type with the given properties /// /// The name of the complex type /// The namespace name of the type /// The version of this type /// dataSpace in which this ComplexType belongs to ///If either name, namespace or version arguments are null internal ComplexType(string name, string namespaceName, DataSpace dataSpace) : base(name, namespaceName, dataSpace) { } ////// Initializes a new instance of Complex Type - required for bootstraping code /// internal ComplexType() { // No initialization of item attributes in here, it's used as a pass thru in the case for delay population // of item attributes } #endregion #region Fields private ReadOnlyMetadataCollection_properties; #endregion #region Properties /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.ComplexType; } } ////// Returns just the properties from the collection /// of members on this type /// public ReadOnlyMetadataCollectionProperties { get { Debug.Assert(IsReadOnly, "this is a wrapper around this.Members, don't call it during metadata loading, only call it after the metadata is set to readonly"); if (null == _properties) { Interlocked.CompareExchange(ref _properties, new FilteredReadOnlyMetadataCollection ( this.Members, Helper.IsEdmProperty), null); } return _properties; } } #endregion #region Methods /// /// Validates a EdmMember object to determine if it can be added to this type's /// Members collection. If this method returns without throwing, it is assumed /// the member is valid. /// /// The member to validate ///Thrown if the member is not a EdmProperty internal override void ValidateMemberForAdd(EdmMember member) { Debug.Assert(Helper.IsEdmProperty(member) || Helper.IsNavigationProperty(member), "Only members of type Property may be added to ComplexType."); } #endregion } internal sealed class ClrComplexType : ComplexType { ///cached CLR type handle, allowing the Type reference to be GC'd private readonly System.RuntimeTypeHandle _type; ///cached dynamic method to construct a CLR instance private Delegate _constructor; private readonly string _cspaceTypeName; ////// Initializes a new instance of Complex Type with properties from the type. /// /// The CLR type to construct from internal ClrComplexType(Type clrType, string cspaceNamespaceName, string cspaceTypeName) : base(EntityUtil.GenericCheckArgumentNull(clrType, "clrType").Name, clrType.Namespace ?? string.Empty, DataSpace.OSpace) { System.Diagnostics.Debug.Assert(!String.IsNullOrEmpty(cspaceNamespaceName) && !String.IsNullOrEmpty(cspaceTypeName), "Mapping information must never be null"); _type = clrType.TypeHandle; _cspaceTypeName = cspaceNamespaceName + "." + cspaceTypeName; this.Abstract = clrType.IsAbstract; } internal static ClrComplexType CreateReadonlyClrComplexType(Type clrType, string cspaceNamespaceName, string cspaceTypeName) { ClrComplexType type = new ClrComplexType(clrType, cspaceNamespaceName, cspaceTypeName); type.SetReadOnly(); return type; } ///cached dynamic method to construct a CLR instance internal Delegate Constructor { get { return _constructor; } set { // It doesn't matter which delegate wins, but only one should be jitted Interlocked.CompareExchange(ref _constructor, value, null); } } ////// internal override System.Type ClrType { get { return Type.GetTypeFromHandle(_type); } } internal string CSpaceTypeName { get { return _cspaceTypeName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Threading; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// Represent the Edm Complex Type /// public class ComplexType : StructuralType { #region Constructors ////// Initializes a new instance of Complex Type with the given properties /// /// The name of the complex type /// The namespace name of the type /// The version of this type /// dataSpace in which this ComplexType belongs to ///If either name, namespace or version arguments are null internal ComplexType(string name, string namespaceName, DataSpace dataSpace) : base(name, namespaceName, dataSpace) { } ////// Initializes a new instance of Complex Type - required for bootstraping code /// internal ComplexType() { // No initialization of item attributes in here, it's used as a pass thru in the case for delay population // of item attributes } #endregion #region Fields private ReadOnlyMetadataCollection_properties; #endregion #region Properties /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.ComplexType; } } ////// Returns just the properties from the collection /// of members on this type /// public ReadOnlyMetadataCollectionProperties { get { Debug.Assert(IsReadOnly, "this is a wrapper around this.Members, don't call it during metadata loading, only call it after the metadata is set to readonly"); if (null == _properties) { Interlocked.CompareExchange(ref _properties, new FilteredReadOnlyMetadataCollection ( this.Members, Helper.IsEdmProperty), null); } return _properties; } } #endregion #region Methods /// /// Validates a EdmMember object to determine if it can be added to this type's /// Members collection. If this method returns without throwing, it is assumed /// the member is valid. /// /// The member to validate ///Thrown if the member is not a EdmProperty internal override void ValidateMemberForAdd(EdmMember member) { Debug.Assert(Helper.IsEdmProperty(member) || Helper.IsNavigationProperty(member), "Only members of type Property may be added to ComplexType."); } #endregion } internal sealed class ClrComplexType : ComplexType { ///cached CLR type handle, allowing the Type reference to be GC'd private readonly System.RuntimeTypeHandle _type; ///cached dynamic method to construct a CLR instance private Delegate _constructor; private readonly string _cspaceTypeName; ////// Initializes a new instance of Complex Type with properties from the type. /// /// The CLR type to construct from internal ClrComplexType(Type clrType, string cspaceNamespaceName, string cspaceTypeName) : base(EntityUtil.GenericCheckArgumentNull(clrType, "clrType").Name, clrType.Namespace ?? string.Empty, DataSpace.OSpace) { System.Diagnostics.Debug.Assert(!String.IsNullOrEmpty(cspaceNamespaceName) && !String.IsNullOrEmpty(cspaceTypeName), "Mapping information must never be null"); _type = clrType.TypeHandle; _cspaceTypeName = cspaceNamespaceName + "." + cspaceTypeName; this.Abstract = clrType.IsAbstract; } internal static ClrComplexType CreateReadonlyClrComplexType(Type clrType, string cspaceNamespaceName, string cspaceTypeName) { ClrComplexType type = new ClrComplexType(clrType, cspaceNamespaceName, cspaceTypeName); type.SetReadOnly(); return type; } ///cached dynamic method to construct a CLR instance internal Delegate Constructor { get { return _constructor; } set { // It doesn't matter which delegate wins, but only one should be jitted Interlocked.CompareExchange(ref _constructor, value, null); } } ////// internal override System.Type ClrType { get { return Type.GetTypeFromHandle(_type); } } internal string CSpaceTypeName { get { return _cspaceTypeName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
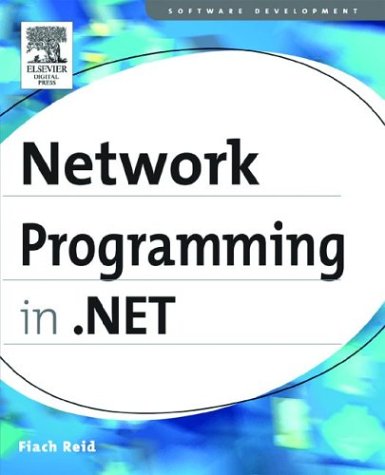
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SessionStateUtil.cs
- WebPartZoneBase.cs
- FileAccessException.cs
- LabelAutomationPeer.cs
- HostedNamedPipeTransportManager.cs
- MarginsConverter.cs
- SizeKeyFrameCollection.cs
- ContentElement.cs
- DataSpaceManager.cs
- DocumentationServerProtocol.cs
- ChannelTraceRecord.cs
- TraceSource.cs
- SystemDiagnosticsSection.cs
- GeometryCollection.cs
- ObjectViewQueryResultData.cs
- ColorMap.cs
- WindowsFormsHostPropertyMap.cs
- SchemaMerger.cs
- BaseAsyncResult.cs
- SafeNativeMethods.cs
- IndexingContentUnit.cs
- ObjectViewListener.cs
- AsyncPostBackErrorEventArgs.cs
- DynamicUpdateCommand.cs
- Button.cs
- StringSource.cs
- TextRangeProviderWrapper.cs
- ShaperBuffers.cs
- EntityDataSourceView.cs
- WpfPayload.cs
- OdbcCommand.cs
- XmlTypeAttribute.cs
- DocumentCollection.cs
- DataGridViewCheckBoxCell.cs
- CodeAttributeArgument.cs
- CoTaskMemHandle.cs
- HealthMonitoringSectionHelper.cs
- CommandEventArgs.cs
- GenericXmlSecurityTokenAuthenticator.cs
- SiteMapNodeItem.cs
- HttpValueCollection.cs
- ResourceType.cs
- MaskedTextBox.cs
- InkCanvasInnerCanvas.cs
- InfoCardHelper.cs
- MediaPlayerState.cs
- VerbConverter.cs
- CodeExpressionCollection.cs
- SortableBindingList.cs
- StylesEditorDialog.cs
- MonthCalendar.cs
- CommandDevice.cs
- Path.cs
- HttpInputStream.cs
- DeviceContext.cs
- PersonalizationAdministration.cs
- CommonDialog.cs
- SapiGrammar.cs
- AsnEncodedData.cs
- VarInfo.cs
- ExceptionHandler.cs
- ConfigViewGenerator.cs
- NetworkInformationException.cs
- ColumnHeader.cs
- SchemaMapping.cs
- DesignerUtility.cs
- UriTemplateMatchException.cs
- StatusStrip.cs
- SymbolDocumentInfo.cs
- PackageDocument.cs
- COMException.cs
- DbConnectionStringCommon.cs
- MembershipSection.cs
- UrlEncodedParameterWriter.cs
- Function.cs
- ScrollChrome.cs
- FileDialogCustomPlace.cs
- ActivityTypeDesigner.xaml.cs
- GeneralTransform3DGroup.cs
- StrongNameKeyPair.cs
- ExpressionBinding.cs
- ImageCodecInfo.cs
- RegexRunnerFactory.cs
- SqlCacheDependencyDatabaseCollection.cs
- DbConnectionPoolGroup.cs
- MinMaxParagraphWidth.cs
- HighlightVisual.cs
- DeviceContext.cs
- Attributes.cs
- Stroke2.cs
- ContentOperations.cs
- RuleInfoComparer.cs
- GregorianCalendar.cs
- RangeValuePattern.cs
- ObjectListDesigner.cs
- PowerEase.cs
- AdapterDictionary.cs
- PersonalizableAttribute.cs
- PropertyTabAttribute.cs
- BuildManagerHost.cs