Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / IO / UnmanagedMemoryStreamWrapper.cs / 1 / UnmanagedMemoryStreamWrapper.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: UnmanagedMemoryStreamWrapper ** ** Purpose: Create a Memorystream over an UnmanagedMemoryStream ** ===========================================================*/ using System; using System.Runtime.InteropServices; using System.Security.Permissions; namespace System.IO { // Needed for backwards compatibility with V1.x usages of the // ResourceManager, where a MemoryStream is now returned as an // UnmanagedMemoryStream from ResourceReader. internal sealed class UnmanagedMemoryStreamWrapper : MemoryStream { private UnmanagedMemoryStream _unmanagedStream; internal UnmanagedMemoryStreamWrapper(UnmanagedMemoryStream stream) { _unmanagedStream = stream; } public override bool CanRead { get { return _unmanagedStream.CanRead; } } public override bool CanSeek { get { return _unmanagedStream.CanSeek; } } public override bool CanWrite { get { return _unmanagedStream.CanWrite; } } protected override void Dispose(bool disposing) { try { if (disposing) _unmanagedStream.Close(); } finally { base.Dispose(disposing); } } public override void Flush() { _unmanagedStream.Flush(); } public override byte[] GetBuffer() { throw new UnauthorizedAccessException(Environment.GetResourceString("UnauthorizedAccess_MemStreamBuffer")); } public override int Capacity { get { return (int) _unmanagedStream.Capacity; } set { throw new IOException(Environment.GetResourceString("IO.IO_FixedCapacity")); } } public override long Length { get { return _unmanagedStream.Length; } } public override long Position { get { return _unmanagedStream.Position; } set { _unmanagedStream.Position = value; } } public override int Read([In, Out] byte[] buffer, int offset, int count) { return _unmanagedStream.Read(buffer, offset, count); } public override int ReadByte() { return _unmanagedStream.ReadByte(); } public override long Seek(long offset, SeekOrigin loc) { return _unmanagedStream.Seek(offset, loc); } public unsafe override byte[] ToArray() { if (!_unmanagedStream._isOpen) __Error.StreamIsClosed(); if (!_unmanagedStream.CanRead) __Error.ReadNotSupported(); byte[] buffer = new byte[_unmanagedStream.Length]; Buffer.memcpy(_unmanagedStream.Pointer, 0, buffer, 0, (int) _unmanagedStream.Length); return buffer; } public override void Write(byte[] buffer, int offset, int count) { _unmanagedStream.Write(buffer, offset, count); } public override void WriteByte(byte value) { _unmanagedStream.WriteByte(value); } // Writes this MemoryStream to another stream. public unsafe override void WriteTo(Stream stream) { if (!_unmanagedStream._isOpen) __Error.StreamIsClosed(); if (!_unmanagedStream.CanRead) __Error.ReadNotSupported(); if (stream==null) throw new ArgumentNullException("stream", Environment.GetResourceString("ArgumentNull_Stream")); byte[] buffer = ToArray(); stream.Write(buffer, 0, buffer.Length); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: UnmanagedMemoryStreamWrapper ** ** Purpose: Create a Memorystream over an UnmanagedMemoryStream ** ===========================================================*/ using System; using System.Runtime.InteropServices; using System.Security.Permissions; namespace System.IO { // Needed for backwards compatibility with V1.x usages of the // ResourceManager, where a MemoryStream is now returned as an // UnmanagedMemoryStream from ResourceReader. internal sealed class UnmanagedMemoryStreamWrapper : MemoryStream { private UnmanagedMemoryStream _unmanagedStream; internal UnmanagedMemoryStreamWrapper(UnmanagedMemoryStream stream) { _unmanagedStream = stream; } public override bool CanRead { get { return _unmanagedStream.CanRead; } } public override bool CanSeek { get { return _unmanagedStream.CanSeek; } } public override bool CanWrite { get { return _unmanagedStream.CanWrite; } } protected override void Dispose(bool disposing) { try { if (disposing) _unmanagedStream.Close(); } finally { base.Dispose(disposing); } } public override void Flush() { _unmanagedStream.Flush(); } public override byte[] GetBuffer() { throw new UnauthorizedAccessException(Environment.GetResourceString("UnauthorizedAccess_MemStreamBuffer")); } public override int Capacity { get { return (int) _unmanagedStream.Capacity; } set { throw new IOException(Environment.GetResourceString("IO.IO_FixedCapacity")); } } public override long Length { get { return _unmanagedStream.Length; } } public override long Position { get { return _unmanagedStream.Position; } set { _unmanagedStream.Position = value; } } public override int Read([In, Out] byte[] buffer, int offset, int count) { return _unmanagedStream.Read(buffer, offset, count); } public override int ReadByte() { return _unmanagedStream.ReadByte(); } public override long Seek(long offset, SeekOrigin loc) { return _unmanagedStream.Seek(offset, loc); } public unsafe override byte[] ToArray() { if (!_unmanagedStream._isOpen) __Error.StreamIsClosed(); if (!_unmanagedStream.CanRead) __Error.ReadNotSupported(); byte[] buffer = new byte[_unmanagedStream.Length]; Buffer.memcpy(_unmanagedStream.Pointer, 0, buffer, 0, (int) _unmanagedStream.Length); return buffer; } public override void Write(byte[] buffer, int offset, int count) { _unmanagedStream.Write(buffer, offset, count); } public override void WriteByte(byte value) { _unmanagedStream.WriteByte(value); } // Writes this MemoryStream to another stream. public unsafe override void WriteTo(Stream stream) { if (!_unmanagedStream._isOpen) __Error.StreamIsClosed(); if (!_unmanagedStream.CanRead) __Error.ReadNotSupported(); if (stream==null) throw new ArgumentNullException("stream", Environment.GetResourceString("ArgumentNull_Stream")); byte[] buffer = ToArray(); stream.Write(buffer, 0, buffer.Length); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
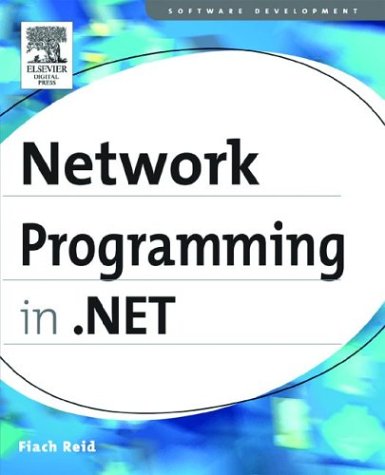
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RuntimeIdentifierPropertyAttribute.cs
- LinkLabelLinkClickedEvent.cs
- SecurityTokenValidationException.cs
- CaseExpr.cs
- Adorner.cs
- ColorConvertedBitmapExtension.cs
- Int32EqualityComparer.cs
- TimelineClockCollection.cs
- NullRuntimeConfig.cs
- XmlDataSource.cs
- RectangleGeometry.cs
- TextBoxDesigner.cs
- FontSource.cs
- UriExt.cs
- OletxResourceManager.cs
- WindowsPrincipal.cs
- Timer.cs
- StylusTouchDevice.cs
- ViewStateAttachedPropertyFeature.cs
- StringConcat.cs
- BuilderPropertyEntry.cs
- ObjectDataSourceChooseMethodsPanel.cs
- LabelDesigner.cs
- SqlSelectStatement.cs
- RelatedImageListAttribute.cs
- BinaryUtilClasses.cs
- SharedPersonalizationStateInfo.cs
- CommandDesigner.cs
- CustomSignedXml.cs
- QuaternionAnimationUsingKeyFrames.cs
- AssemblyBuilder.cs
- UserControlFileEditor.cs
- XmlEventCache.cs
- COSERVERINFO.cs
- JsonEncodingStreamWrapper.cs
- CompositeActivityTypeDescriptor.cs
- MulticastOption.cs
- PropertyInformationCollection.cs
- COM2ExtendedBrowsingHandler.cs
- ToolStripMenuItemCodeDomSerializer.cs
- TextDecoration.cs
- CustomValidator.cs
- DependencyObject.cs
- BrowserCapabilitiesFactory.cs
- CommonXSendMessage.cs
- StringKeyFrameCollection.cs
- RealizationContext.cs
- Transform3DGroup.cs
- SystemColorTracker.cs
- WorkflowMarkupSerializationManager.cs
- UserPreferenceChangedEventArgs.cs
- ObjectSecurityT.cs
- WebPartEditorOkVerb.cs
- ObjectStateEntry.cs
- HtmlHistory.cs
- filewebresponse.cs
- ExtensionsSection.cs
- sqlser.cs
- InstalledVoice.cs
- BaseCodePageEncoding.cs
- SoapReflectionImporter.cs
- EditCommandColumn.cs
- BufferedMessageWriter.cs
- DataPagerFieldCollection.cs
- TemplateKey.cs
- TextTreeText.cs
- CharUnicodeInfo.cs
- MaterialCollection.cs
- ClientCultureInfo.cs
- SafeEventLogWriteHandle.cs
- XmlNotation.cs
- UnknownBitmapEncoder.cs
- SR.cs
- IpcChannelHelper.cs
- RequestCacheEntry.cs
- StubHelpers.cs
- NullableIntMinMaxAggregationOperator.cs
- SmtpClient.cs
- ProfileBuildProvider.cs
- DynamicRendererThreadManager.cs
- DataGridColumnHeadersPresenterAutomationPeer.cs
- CodeGen.cs
- ReadOnlyKeyedCollection.cs
- RoutedCommand.cs
- SqlConnectionFactory.cs
- ScrollChrome.cs
- RequestCacheValidator.cs
- LinqDataSourceView.cs
- ControllableStoryboardAction.cs
- _HeaderInfoTable.cs
- ByeMessageCD1.cs
- Set.cs
- Enum.cs
- ParserContext.cs
- BrushConverter.cs
- OuterGlowBitmapEffect.cs
- QilTargetType.cs
- DodSequenceMerge.cs
- LingerOption.cs
- ChildDocumentBlock.cs