Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / ComponentModel / MarshalByValueComponent.cs / 1 / MarshalByValueComponent.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.ComponentModel.Design; using System.Diagnostics.CodeAnalysis; using System.Security.Permissions; using System.Runtime.InteropServices; ////// [ ComVisible(true), Designer("System.Windows.Forms.Design.ComponentDocumentDesigner, " + AssemblyRef.SystemDesign, typeof(IRootDesigner)), DesignerCategory("Component"), TypeConverter(typeof(ComponentConverter)) ] public class MarshalByValueComponent : IComponent, IServiceProvider { ///Provides the base implementation for ///, /// which is the base class for all components in Win Forms. /// private static readonly object EventDisposed = new object(); private ISite site; private EventHandlerList events; ///Static hask key for the Disposed event. This field is read-only. ////// public MarshalByValueComponent() { } ~MarshalByValueComponent() { Dispose(false); } ///Initializes a new instance of the ///class. /// public event EventHandler Disposed { add { Events.AddHandler(EventDisposed, value); } remove { Events.RemoveHandler(EventDisposed, value); } } ///Adds a event handler to listen to the Disposed event on the component. ////// protected EventHandlerList Events { get { if (events == null) { events = new EventHandlerList(); } return events; } } ///Gets the list of event handlers that are attached to this component. ////// [Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public virtual ISite Site { get { return site;} set { site = value;} } ///Gets or sets the site of the component. ////// [SuppressMessage("Microsoft.Usage", "CA2213:DisposableFieldsShouldBeDisposed")] public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ///Disposes of the resources (other than memory) used by the component. ////// protected virtual void Dispose(bool disposing) { if (disposing) { lock(this) { if (site != null && site.Container != null) { site.Container.Remove(this); } if (events != null) { EventHandler handler = (EventHandler)events[EventDisposed]; if (handler != null) handler(this, EventArgs.Empty); } } } } ////// Disposes all the resources associated with this component. /// If disposing is false then you must never touch any other /// managed objects, as they may already be finalized. When /// in this state you should dispose any native resources /// that you have a reference to. /// ////// When disposing is true then you should dispose all data /// and objects you have references to. The normal implementation /// of this method would look something like: /// ////// public void Dispose() { /// Dispose(true); /// GC.SuppressFinalize(this); /// } /// /// protected virtual void Dispose(bool disposing) { /// if (disposing) { /// if (myobject != null) { /// myobject.Dispose(); /// myobject = null; /// } /// } /// if (myhandle != IntPtr.Zero) { /// NativeMethods.Release(myhandle); /// myhandle = IntPtr.Zero; /// } /// } /// /// ~MyClass() { /// Dispose(false); /// } ///
////// For base classes, you should never override the Finalier (~Class in C#) /// or the Dispose method that takes no arguments, rather you should /// always override the Dispose method that takes a bool. /// ////// protected override void Dispose(bool disposing) { /// if (disposing) { /// if (myobject != null) { /// myobject.Dispose(); /// myobject = null; /// } /// } /// if (myhandle != IntPtr.Zero) { /// NativeMethods.Release(myhandle); /// myhandle = IntPtr.Zero; /// } /// base.Dispose(disposing); /// } ///
////// [Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public virtual IContainer Container { get { ISite s = site; return s == null ? null : s.Container; } } ///Gets the container for the component. ////// public virtual object GetService(Type service) { return((site==null)? null : site.GetService(service)); } ///Gets the implementer of the ///. /// [Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public virtual bool DesignMode { get { ISite s = site; return(s == null) ? false : s.DesignMode; } } ///Gets a value indicating whether the component is currently in design mode. ////// /// public override String ToString() { ISite s = site; if (s != null) return s.Name + " [" + GetType().FullName + "]"; else return GetType().FullName; } } }/// Returns a ///containing the name of the , if any. This method should not be /// overridden. For /// internal use only. ///
Link Menu
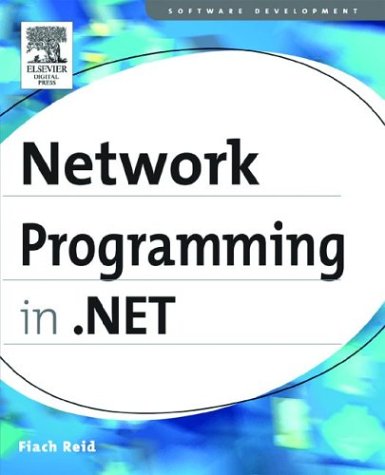
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextInfo.cs
- EdmSchemaAttribute.cs
- SiteMap.cs
- IsolatedStorageException.cs
- QuotaExceededException.cs
- BooleanConverter.cs
- PreservationFileWriter.cs
- MenuItemBindingCollection.cs
- JavascriptCallbackMessageInspector.cs
- AvTrace.cs
- DataGridTextBoxColumn.cs
- PrincipalPermission.cs
- ConstructorArgumentAttribute.cs
- PlatformCulture.cs
- LocalizableAttribute.cs
- XmlSchemaAttributeGroupRef.cs
- SafeProcessHandle.cs
- WebPartMenuStyle.cs
- RTTypeWrapper.cs
- SoapFault.cs
- DecoderExceptionFallback.cs
- WpfXamlType.cs
- LineProperties.cs
- CodeGeneratorOptions.cs
- BuildDependencySet.cs
- MetricEntry.cs
- SqlDataSourceSummaryPanel.cs
- CodeIdentifier.cs
- MatrixTransform.cs
- PersonalizationProviderCollection.cs
- OracleTransaction.cs
- Compiler.cs
- HtmlMeta.cs
- ValidationError.cs
- Random.cs
- Assembly.cs
- TimeZone.cs
- ImportCatalogPart.cs
- SmiXetterAccessMap.cs
- ToolStripOverflowButton.cs
- IndependentlyAnimatedPropertyMetadata.cs
- CharKeyFrameCollection.cs
- SqlFileStream.cs
- _SecureChannel.cs
- TextFormatter.cs
- UnsignedPublishLicense.cs
- Int32EqualityComparer.cs
- AsyncSerializedWorker.cs
- ClientSection.cs
- InputEventArgs.cs
- ClientBuildManager.cs
- Handle.cs
- HtmlLinkAdapter.cs
- RelationshipConstraintValidator.cs
- TimeEnumHelper.cs
- TextShapeableCharacters.cs
- TimeoutTimer.cs
- ActiveXHelper.cs
- XmlSchemaType.cs
- Byte.cs
- EntityDataSourceView.cs
- XmlSchemaElement.cs
- PathFigureCollectionConverter.cs
- GridViewEditEventArgs.cs
- VisualCollection.cs
- XslVisitor.cs
- PolicyException.cs
- DbgUtil.cs
- DynamicRendererThreadManager.cs
- BinaryConverter.cs
- KeyInfo.cs
- AppSettingsReader.cs
- SettingsPropertyValue.cs
- AsnEncodedData.cs
- XmlSerializer.cs
- MailAddress.cs
- DesignerAdapterUtil.cs
- ByteConverter.cs
- ZipIOCentralDirectoryBlock.cs
- XPathScanner.cs
- UrlPath.cs
- Vector3DCollection.cs
- MessageQueuePermissionAttribute.cs
- ConfigurationStrings.cs
- ScriptReference.cs
- SlotInfo.cs
- KnownColorTable.cs
- _LocalDataStore.cs
- HtmlProps.cs
- ParenthesizePropertyNameAttribute.cs
- _NetRes.cs
- EdmToObjectNamespaceMap.cs
- JavaScriptString.cs
- Math.cs
- ListViewItem.cs
- WebMethodAttribute.cs
- TextDecorationCollection.cs
- CodeBlockBuilder.cs
- DesignerWebPartChrome.cs
- Baml2006ReaderFrame.cs