Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntityDesign / Design / System / Data / EntityModel / Emitters / SchemaTypeEmitter.cs / 1305376 / SchemaTypeEmitter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.CodeDom; using System.Data.EntityModel.SchemaObjectModel; using Som = System.Data.EntityModel.SchemaObjectModel; using System.Data.Metadata.Edm; using System.Reflection; using System.Diagnostics; using System.Collections.Generic; using System.Data.Entity.Design; using System.Data.Entity.Design.SsdlGenerator; namespace System.Data.EntityModel.Emitters { ////// /// internal abstract class SchemaTypeEmitter : MetadataItemEmitter { #region Public Methods public abstract CodeTypeDeclarationCollection EmitApiClass(); #endregion #region Protected Methods ////// /// /// /// protected SchemaTypeEmitter(ClientApiGenerator generator, MetadataItem item) : base(generator, item) { } ////// /// /// protected virtual void EmitTypeAttributes( CodeTypeDeclaration typeDecl ) { Generator.AttributeEmitter.EmitTypeAttributes( this, typeDecl ); } ////// Emitter-specific validation: for SchemaTypeEmitter-derived classes, we /// check the EdmItemCollection for other entities that have the same name /// but differ in case /// protected override void Validate() { Generator.VerifyLanguageCaseSensitiveCompatibilityForType(Item); } ////// Add attributes to a type's CustomAttributes collection /// /// The name of the type /// The type to annotate /// The additional attributes protected void EmitTypeAttributes(string itemName, CodeTypeDeclaration typeDecl, ListadditionalAttributes) { if (additionalAttributes != null && additionalAttributes.Count > 0) { try { typeDecl.CustomAttributes.AddRange(additionalAttributes.ToArray()); } catch (ArgumentNullException e) { Generator.AddError(Strings.InvalidAttributeSuppliedForType(itemName), ModelBuilderErrorCode.InvalidAttributeSuppliedForType, EdmSchemaErrorSeverity.Error, e); } } EmitTypeAttributes(typeDecl); } /// /// Add interfaces to the type's list of BaseTypes /// /// The name of the type /// The type whose list of base types needs to be extended /// The interfaces to add to the list of base types protected void AddInterfaces(string itemName, CodeTypeDeclaration typeDecl, ListadditionalInterfaces) { if (additionalInterfaces != null) { try { foreach (Type interfaceType in additionalInterfaces) { typeDecl.BaseTypes.Add(new CodeTypeReference(interfaceType)); } } catch (ArgumentNullException e) { Generator.AddError(Strings.InvalidInterfaceSuppliedForType(itemName), ModelBuilderErrorCode.InvalidInterfaceSuppliedForType, EdmSchemaErrorSeverity.Error, e); } } } /// /// Add interfaces to the type's list of BaseTypes /// /// The name of the type /// The type to which members need to be added /// The members to add protected void AddMembers(string itemName, CodeTypeDeclaration typeDecl, ListadditionalMembers) { if (additionalMembers != null && additionalMembers.Count > 0) { try { typeDecl.Members.AddRange(additionalMembers.ToArray()); } catch (ArgumentNullException e) { Generator.AddError(Strings.InvalidMemberSuppliedForType(itemName), ModelBuilderErrorCode.InvalidMemberSuppliedForType, EdmSchemaErrorSeverity.Error, e); } } } #endregion #region Protected Properties /// /// Gets the element that code is being emitted for. /// internal new GlobalItem Item { get { return base.Item as GlobalItem; } } internal void SetTypeVisibility(CodeTypeDeclaration typeDecl) { typeDecl.TypeAttributes &= ~System.Reflection.TypeAttributes.VisibilityMask; typeDecl.TypeAttributes |= GetTypeAccessibilityValue(Item); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.CodeDom; using System.Data.EntityModel.SchemaObjectModel; using Som = System.Data.EntityModel.SchemaObjectModel; using System.Data.Metadata.Edm; using System.Reflection; using System.Diagnostics; using System.Collections.Generic; using System.Data.Entity.Design; using System.Data.Entity.Design.SsdlGenerator; namespace System.Data.EntityModel.Emitters { ////// /// internal abstract class SchemaTypeEmitter : MetadataItemEmitter { #region Public Methods public abstract CodeTypeDeclarationCollection EmitApiClass(); #endregion #region Protected Methods ////// /// /// /// protected SchemaTypeEmitter(ClientApiGenerator generator, MetadataItem item) : base(generator, item) { } ////// /// /// protected virtual void EmitTypeAttributes( CodeTypeDeclaration typeDecl ) { Generator.AttributeEmitter.EmitTypeAttributes( this, typeDecl ); } ////// Emitter-specific validation: for SchemaTypeEmitter-derived classes, we /// check the EdmItemCollection for other entities that have the same name /// but differ in case /// protected override void Validate() { Generator.VerifyLanguageCaseSensitiveCompatibilityForType(Item); } ////// Add attributes to a type's CustomAttributes collection /// /// The name of the type /// The type to annotate /// The additional attributes protected void EmitTypeAttributes(string itemName, CodeTypeDeclaration typeDecl, ListadditionalAttributes) { if (additionalAttributes != null && additionalAttributes.Count > 0) { try { typeDecl.CustomAttributes.AddRange(additionalAttributes.ToArray()); } catch (ArgumentNullException e) { Generator.AddError(Strings.InvalidAttributeSuppliedForType(itemName), ModelBuilderErrorCode.InvalidAttributeSuppliedForType, EdmSchemaErrorSeverity.Error, e); } } EmitTypeAttributes(typeDecl); } /// /// Add interfaces to the type's list of BaseTypes /// /// The name of the type /// The type whose list of base types needs to be extended /// The interfaces to add to the list of base types protected void AddInterfaces(string itemName, CodeTypeDeclaration typeDecl, ListadditionalInterfaces) { if (additionalInterfaces != null) { try { foreach (Type interfaceType in additionalInterfaces) { typeDecl.BaseTypes.Add(new CodeTypeReference(interfaceType)); } } catch (ArgumentNullException e) { Generator.AddError(Strings.InvalidInterfaceSuppliedForType(itemName), ModelBuilderErrorCode.InvalidInterfaceSuppliedForType, EdmSchemaErrorSeverity.Error, e); } } } /// /// Add interfaces to the type's list of BaseTypes /// /// The name of the type /// The type to which members need to be added /// The members to add protected void AddMembers(string itemName, CodeTypeDeclaration typeDecl, ListadditionalMembers) { if (additionalMembers != null && additionalMembers.Count > 0) { try { typeDecl.Members.AddRange(additionalMembers.ToArray()); } catch (ArgumentNullException e) { Generator.AddError(Strings.InvalidMemberSuppliedForType(itemName), ModelBuilderErrorCode.InvalidMemberSuppliedForType, EdmSchemaErrorSeverity.Error, e); } } } #endregion #region Protected Properties /// /// Gets the element that code is being emitted for. /// internal new GlobalItem Item { get { return base.Item as GlobalItem; } } internal void SetTypeVisibility(CodeTypeDeclaration typeDecl) { typeDecl.TypeAttributes &= ~System.Reflection.TypeAttributes.VisibilityMask; typeDecl.TypeAttributes |= GetTypeAccessibilityValue(Item); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
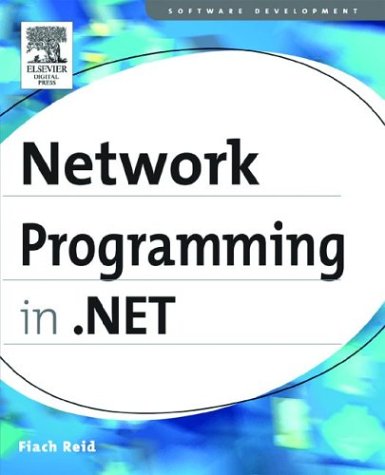
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PointLight.cs
- DataGridAddNewRow.cs
- Symbol.cs
- XamlWriter.cs
- NativeMethods.cs
- MethodToken.cs
- DuplexChannelBinder.cs
- HandleValueEditor.cs
- FormViewPagerRow.cs
- UnknownBitmapEncoder.cs
- SerializationStore.cs
- PropertyExpression.cs
- Baml2006KeyRecord.cs
- XamlRtfConverter.cs
- Shape.cs
- StylusSystemGestureEventArgs.cs
- ThumbButtonInfo.cs
- NotFiniteNumberException.cs
- Axis.cs
- MaskedTextProvider.cs
- ComponentCollection.cs
- TreeNode.cs
- Stackframe.cs
- Odbc32.cs
- UpdatePanelTriggerCollection.cs
- Descriptor.cs
- PaintEvent.cs
- XmlDictionaryString.cs
- exports.cs
- TemplateColumn.cs
- ACE.cs
- DataGridViewComboBoxColumnDesigner.cs
- DataObject.cs
- AtomicFile.cs
- State.cs
- CodeGen.cs
- MessageHeaderInfoTraceRecord.cs
- SimpleLine.cs
- ElementProxy.cs
- BindingNavigatorDesigner.cs
- DistributedTransactionPermission.cs
- IERequestCache.cs
- ToolStripOverflow.cs
- HTTPNotFoundHandler.cs
- ExtensionWindowResizeGrip.cs
- UnionExpr.cs
- TimelineCollection.cs
- XmlCharacterData.cs
- FormsAuthenticationConfiguration.cs
- ImpersonationContext.cs
- GraphicsPathIterator.cs
- ProjectedSlot.cs
- ToolStripSeparator.cs
- QilValidationVisitor.cs
- PenContext.cs
- WorkflowMarkupSerializer.cs
- DependencyObjectProvider.cs
- Memoizer.cs
- PageHandlerFactory.cs
- HelpInfo.cs
- RadioButton.cs
- XmlSchemaSimpleTypeRestriction.cs
- TextEndOfParagraph.cs
- RangeValueProviderWrapper.cs
- AttributedMetaModel.cs
- SafeRightsManagementHandle.cs
- RuleRef.cs
- TraversalRequest.cs
- CacheRequest.cs
- DataRowCollection.cs
- ContextBase.cs
- XmlBoundElement.cs
- EventHandlerList.cs
- XmlSchemaCompilationSettings.cs
- ProgressChangedEventArgs.cs
- Transform3DCollection.cs
- BoolExpression.cs
- InitiatorServiceModelSecurityTokenRequirement.cs
- MembershipSection.cs
- PaintValueEventArgs.cs
- SQLBinary.cs
- ListControl.cs
- FlagPanel.cs
- PrimitiveDataContract.cs
- MenuItemCollection.cs
- CompiledQuery.cs
- IdentityHolder.cs
- Queue.cs
- CultureTableRecord.cs
- XPathQilFactory.cs
- EntityStoreSchemaFilterEntry.cs
- PersonalizableAttribute.cs
- InheritablePropertyChangeInfo.cs
- DocumentViewerConstants.cs
- NullableIntMinMaxAggregationOperator.cs
- ScriptResourceAttribute.cs
- PropertyStore.cs
- XmlHierarchicalDataSourceView.cs
- SoapRpcServiceAttribute.cs
- DbParameterHelper.cs