Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Cryptography / RijndaelManaged.cs / 1305376 / RijndaelManaged.cs
using System.Diagnostics.Contracts; // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // RijndaelManaged.cs // namespace System.Security.Cryptography { [System.Runtime.InteropServices.ComVisible(true)] public sealed class RijndaelManaged : Rijndael { public RijndaelManaged () { #if FEATURE_CRYPTO if (CryptoConfig.AllowOnlyFipsAlgorithms) throw new InvalidOperationException(Environment.GetResourceString("Cryptography_NonCompliantFIPSAlgorithm")); Contract.EndContractBlock(); #endif // FEATURE_CRYPTO } // #CoreCLRRijndaelModes // // On CoreCLR we limit the supported cipher modes and padding modes for the AES algorithm to a // single hard coded value. This allows us to remove a lot of code by removing support for the // uncommon cases and forcing everyone to use the same common padding and ciper modes: // // - CipherMode: CipherMode.CBC // - PaddingMode: PaddingMode.PKCS7 public override ICryptoTransform CreateEncryptor (byte[] rgbKey, byte[] rgbIV) { return NewEncryptor(rgbKey, ModeValue, rgbIV, FeedbackSizeValue, RijndaelManagedTransformMode.Encrypt); } public override ICryptoTransform CreateDecryptor (byte[] rgbKey, byte[] rgbIV) { return NewEncryptor(rgbKey, ModeValue, rgbIV, FeedbackSizeValue, RijndaelManagedTransformMode.Decrypt); } public override void GenerateKey () { KeyValue = Utils.GenerateRandom(KeySizeValue / 8); } public override void GenerateIV () { IVValue = Utils.GenerateRandom(BlockSizeValue / 8); } private ICryptoTransform NewEncryptor (byte[] rgbKey, CipherMode mode, byte[] rgbIV, int feedbackSize, RijndaelManagedTransformMode encryptMode) { // Build the key if one does not already exist if (rgbKey == null) { rgbKey = Utils.GenerateRandom(KeySizeValue / 8); } // If not ECB mode, make sure we have an IV. In CoreCLR we do not support ECB, so we must have // an IV in all cases. #if !FEATURE_CRYPTO if (mode != CipherMode.ECB) { #endif // !FEATURE_CRYPTO if (rgbIV == null) { rgbIV = Utils.GenerateRandom(BlockSizeValue / 8); } #if !FEATURE_CRYPTO } #endif // !FEATURE_CRYPTO // Create the encryptor/decryptor object return new RijndaelManagedTransform (rgbKey, mode, rgbIV, BlockSizeValue, feedbackSize, PaddingValue, encryptMode); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Diagnostics.Contracts; // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // RijndaelManaged.cs // namespace System.Security.Cryptography { [System.Runtime.InteropServices.ComVisible(true)] public sealed class RijndaelManaged : Rijndael { public RijndaelManaged () { #if FEATURE_CRYPTO if (CryptoConfig.AllowOnlyFipsAlgorithms) throw new InvalidOperationException(Environment.GetResourceString("Cryptography_NonCompliantFIPSAlgorithm")); Contract.EndContractBlock(); #endif // FEATURE_CRYPTO } // #CoreCLRRijndaelModes // // On CoreCLR we limit the supported cipher modes and padding modes for the AES algorithm to a // single hard coded value. This allows us to remove a lot of code by removing support for the // uncommon cases and forcing everyone to use the same common padding and ciper modes: // // - CipherMode: CipherMode.CBC // - PaddingMode: PaddingMode.PKCS7 public override ICryptoTransform CreateEncryptor (byte[] rgbKey, byte[] rgbIV) { return NewEncryptor(rgbKey, ModeValue, rgbIV, FeedbackSizeValue, RijndaelManagedTransformMode.Encrypt); } public override ICryptoTransform CreateDecryptor (byte[] rgbKey, byte[] rgbIV) { return NewEncryptor(rgbKey, ModeValue, rgbIV, FeedbackSizeValue, RijndaelManagedTransformMode.Decrypt); } public override void GenerateKey () { KeyValue = Utils.GenerateRandom(KeySizeValue / 8); } public override void GenerateIV () { IVValue = Utils.GenerateRandom(BlockSizeValue / 8); } private ICryptoTransform NewEncryptor (byte[] rgbKey, CipherMode mode, byte[] rgbIV, int feedbackSize, RijndaelManagedTransformMode encryptMode) { // Build the key if one does not already exist if (rgbKey == null) { rgbKey = Utils.GenerateRandom(KeySizeValue / 8); } // If not ECB mode, make sure we have an IV. In CoreCLR we do not support ECB, so we must have // an IV in all cases. #if !FEATURE_CRYPTO if (mode != CipherMode.ECB) { #endif // !FEATURE_CRYPTO if (rgbIV == null) { rgbIV = Utils.GenerateRandom(BlockSizeValue / 8); } #if !FEATURE_CRYPTO } #endif // !FEATURE_CRYPTO // Create the encryptor/decryptor object return new RijndaelManagedTransform (rgbKey, mode, rgbIV, BlockSizeValue, feedbackSize, PaddingValue, encryptMode); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
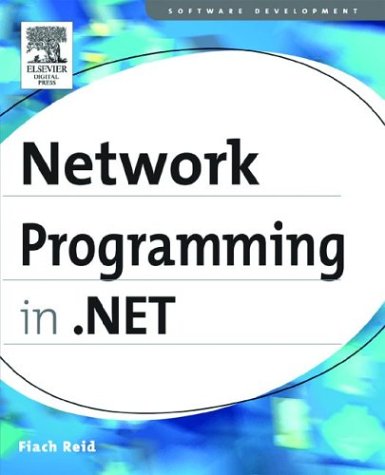
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- selecteditemcollection.cs
- ToolStripCustomTypeDescriptor.cs
- DSACryptoServiceProvider.cs
- BaseAppDomainProtocolHandler.cs
- IsolationInterop.cs
- UIElementParagraph.cs
- TimelineGroup.cs
- loginstatus.cs
- GlyphRunDrawing.cs
- SemanticBasicElement.cs
- TextEditorParagraphs.cs
- TypeUsageBuilder.cs
- FigureHelper.cs
- Metadata.cs
- DateTimeOffsetStorage.cs
- HandlerFactoryCache.cs
- regiisutil.cs
- IDReferencePropertyAttribute.cs
- ToolBarButton.cs
- WebScriptMetadataMessageEncoderFactory.cs
- SortQuery.cs
- GroupItem.cs
- ToolboxItemFilterAttribute.cs
- DropTarget.cs
- Animatable.cs
- DataMisalignedException.cs
- BindingContext.cs
- HyperLinkField.cs
- WindowsImpersonationContext.cs
- SByte.cs
- Margins.cs
- SuppressMessageAttribute.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- SimpleWorkerRequest.cs
- ConfigurationLoader.cs
- EnumConverter.cs
- ValueTypeFixupInfo.cs
- BodyWriter.cs
- ReflectionTypeLoadException.cs
- WsatAdminException.cs
- ExceptionUtil.cs
- XmlLoader.cs
- StatusBarAutomationPeer.cs
- BoundConstants.cs
- TreeNodeClickEventArgs.cs
- RichTextBox.cs
- SuppressMessageAttribute.cs
- ContextQuery.cs
- MultiDataTrigger.cs
- Compiler.cs
- RenderCapability.cs
- InternalConfigSettingsFactory.cs
- SqlNotificationRequest.cs
- HttpModuleCollection.cs
- PathParser.cs
- ExpandableObjectConverter.cs
- FormattedText.cs
- BufferCache.cs
- PasswordDeriveBytes.cs
- SourceElementsCollection.cs
- ProxyGenerator.cs
- TypeResolvingOptions.cs
- ExtractorMetadata.cs
- WizardForm.cs
- StatusBarPanelClickEvent.cs
- ContextConfiguration.cs
- ContractCodeDomInfo.cs
- ItemList.cs
- ThreadInterruptedException.cs
- FunctionParameter.cs
- PrincipalPermission.cs
- ToolStripDropDownButton.cs
- SafeCryptContextHandle.cs
- ReadOnlyDictionary.cs
- FloaterParagraph.cs
- WebBrowserBase.cs
- Parser.cs
- WriteableBitmap.cs
- CompoundFileStreamReference.cs
- XmlSerializableWriter.cs
- MenuAutomationPeer.cs
- objectquery_tresulttype.cs
- MDIWindowDialog.cs
- MachineKeySection.cs
- LoginUtil.cs
- ProcessHost.cs
- PageCodeDomTreeGenerator.cs
- SqlTriggerContext.cs
- CodeMemberField.cs
- ApplicationHost.cs
- RoutedEventArgs.cs
- AutomationElementIdentifiers.cs
- XsltConvert.cs
- BaseDataBoundControl.cs
- Timeline.cs
- PropertyGridDesigner.cs
- AuthorizationContext.cs
- FilterQuery.cs
- SqlAggregateChecker.cs
- DataReceivedEventArgs.cs