Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Imaging / CroppedBitmap.cs / 1305600 / CroppedBitmap.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation. All Rights Reserved. // // File: CroppedBitmap.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { #region CroppedBitmap ////// CroppedBitmap provides caching functionality for a BitmapSource. /// public sealed partial class CroppedBitmap : Imaging.BitmapSource, ISupportInitialize { ////// Constructor /// public CroppedBitmap() : base(true) { } ////// Construct a CroppedBitmap /// /// BitmapSource to apply to the crop to /// Source rect of the bitmap to use public CroppedBitmap(BitmapSource source, Int32Rect sourceRect) : base(true) // Use base class virtuals { if (source == null) { throw new ArgumentNullException("source"); } _bitmapInit.BeginInit(); Source = source; SourceRect = sourceRect; _bitmapInit.EndInit(); FinalizeCreation(); } // ISupportInitialize ////// Prepare the bitmap to accept initialize paramters. /// public void BeginInit() { WritePreamble(); _bitmapInit.BeginInit(); } ////// Prepare the bitmap to accept initialize paramters. /// ////// Critical - access critical resources /// PublicOK - All inputs verified /// [SecurityCritical ] public void EndInit() { WritePreamble(); _bitmapInit.EndInit(); IsValidForFinalizeCreation(/* throwIfInvalid = */ true); FinalizeCreation(); } private void ClonePrequel(CroppedBitmap otherCroppedBitmap) { BeginInit(); } private void ClonePostscript(CroppedBitmap otherCroppedBitmap) { EndInit(); } /// /// Create the unmanaged resources /// ////// Critical - access critical resource /// [SecurityCritical] internal override void FinalizeCreation() { _bitmapInit.EnsureInitializedComplete(); BitmapSourceSafeMILHandle wicClipper = null; Int32Rect rect = SourceRect; BitmapSource source = Source; if (rect.IsEmpty) { rect.Width = source.PixelWidth; rect.Height = source.PixelHeight; } using (FactoryMaker factoryMaker = new FactoryMaker()) { try { IntPtr wicFactory = factoryMaker.ImagingFactoryPtr; HRESULT.Check(UnsafeNativeMethods.WICImagingFactory.CreateBitmapClipper( wicFactory, out wicClipper)); lock (_syncObject) { HRESULT.Check(UnsafeNativeMethods.WICBitmapClipper.Initialize( wicClipper, source.WicSourceHandle, ref rect)); } // // This is just a link in a BitmapSource chain. The memory is being used by // the BitmapSource at the end of the chain, so no memory pressure needs // to be added here. // WicSourceHandle = wicClipper; _isSourceCached = source.IsSourceCached; wicClipper = null; } catch { _bitmapInit.Reset(); throw; } finally { if (wicClipper != null) { wicClipper.Close(); } } } CreationCompleted = true; UpdateCachedSettings(); } ////// Notification on source changing. /// private void SourcePropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { BitmapSource newSource = e.NewValue as BitmapSource; _source = newSource; RegisterDownloadEventSource(_source); _syncObject = (newSource != null) ? newSource.SyncObject : _bitmapInit; } } internal override bool IsValidForFinalizeCreation(bool throwIfInvalid) { if (Source == null) { if (throwIfInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_NoArgument, "Source")); } return false; } return true; } ////// Notification on source rect changing. /// private void SourceRectPropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { _sourceRect = (Int32Rect)e.NewValue; } } ////// Coerce Source /// private static object CoerceSource(DependencyObject d, object value) { CroppedBitmap bitmap = (CroppedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._source; } else { return value; } } ////// Coerce SourceRect /// private static object CoerceSourceRect(DependencyObject d, object value) { CroppedBitmap bitmap = (CroppedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._sourceRect; } else { return value; } } #region Data members private BitmapSource _source; private Int32Rect _sourceRect; #endregion } #endregion // CroppedBitmap } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
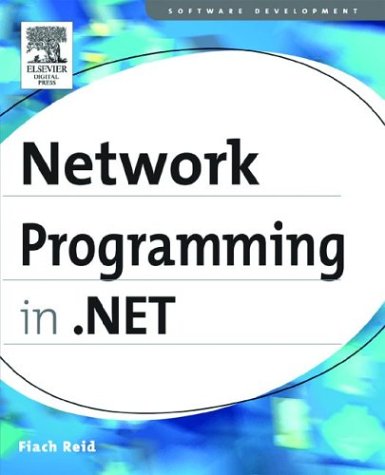
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XPathAxisIterator.cs
- ContentTypeSettingDispatchMessageFormatter.cs
- NamespaceQuery.cs
- SoapRpcServiceAttribute.cs
- CommandBinding.cs
- EDesignUtil.cs
- MobileControlBuilder.cs
- GeometryConverter.cs
- PaintEvent.cs
- TextDecoration.cs
- Perspective.cs
- ProtocolsConfiguration.cs
- OdbcErrorCollection.cs
- WhiteSpaceTrimStringConverter.cs
- FigureParagraph.cs
- NativeMethods.cs
- StylusPointPropertyUnit.cs
- ToolBarButtonClickEvent.cs
- MessageHeader.cs
- OnOperation.cs
- DrawingImage.cs
- LineProperties.cs
- GPRECTF.cs
- WebBodyFormatMessageProperty.cs
- ScriptRef.cs
- PersonalizableAttribute.cs
- ApplicationFileParser.cs
- DelegateTypeInfo.cs
- XmlAnyAttributeAttribute.cs
- NetTcpSecurity.cs
- shaperfactory.cs
- ArrayWithOffset.cs
- XmlNodeWriter.cs
- IgnoreDataMemberAttribute.cs
- WebPartExportVerb.cs
- QilXmlReader.cs
- QueryBranchOp.cs
- SelectionRangeConverter.cs
- NotifyInputEventArgs.cs
- HMAC.cs
- Int64AnimationBase.cs
- TabletCollection.cs
- ContractHandle.cs
- Matrix.cs
- DbConnectionPoolCounters.cs
- ExceptQueryOperator.cs
- ImageField.cs
- EventManager.cs
- SqlSelectStatement.cs
- AccessKeyManager.cs
- BuildProvider.cs
- elementinformation.cs
- SpStreamWrapper.cs
- WebPartDescription.cs
- ClientRolePrincipal.cs
- DragStartedEventArgs.cs
- XmlCDATASection.cs
- XmlQualifiedName.cs
- InkPresenter.cs
- TraceListeners.cs
- ADMembershipProvider.cs
- ObjectQuery_EntitySqlExtensions.cs
- EdgeModeValidation.cs
- OleDbEnumerator.cs
- AutomationPatternInfo.cs
- ObjectStateFormatter.cs
- CodePrimitiveExpression.cs
- DataMember.cs
- StorageMappingItemLoader.cs
- XmlSerializerNamespaces.cs
- DPCustomTypeDescriptor.cs
- Properties.cs
- LocalizationCodeDomSerializer.cs
- TraceHandlerErrorFormatter.cs
- PasswordTextContainer.cs
- ScriptBehaviorDescriptor.cs
- InternalCache.cs
- TableCell.cs
- RegexCaptureCollection.cs
- PasswordBox.cs
- configsystem.cs
- StringValueConverter.cs
- ToolStripTextBox.cs
- TakeOrSkipQueryOperator.cs
- TreeWalkHelper.cs
- MemberHolder.cs
- ItemDragEvent.cs
- SourceSwitch.cs
- CommandField.cs
- ListArgumentProvider.cs
- XmlNodeChangedEventArgs.cs
- AuthenticationService.cs
- StrongTypingException.cs
- ProgressChangedEventArgs.cs
- ApplyImportsAction.cs
- DecimalAnimation.cs
- VisualStyleRenderer.cs
- DateTimeUtil.cs
- SemanticKeyElement.cs
- sqlmetadatafactory.cs