Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / Facet.cs / 1305376 / Facet.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Text; using System.Diagnostics; using System.Data.Entity; using System.Globalization; namespace System.Data.Metadata.Edm { ////// Class for representing a Facet object /// This object is Immutable (not just set to readonly) and /// some parts of the system are depending on that behavior /// [DebuggerDisplay("{Name,nq}={Value}")] public sealed class Facet : MetadataItem { #region Constructors ////// The constructor for constructing a Facet object with the facet description and a value /// /// The object describing this facet /// The value of the facet ///Thrown if facetDescription argument is null private Facet(FacetDescription facetDescription, object value) :base(MetadataFlags.Readonly) { EntityUtil.GenericCheckArgumentNull(facetDescription, "facetDescription"); _facetDescription = facetDescription; _value = value; } ////// Creates a Facet instance with the specified value for the given /// facet description. /// /// The object describing this facet /// The value of the facet ///Thrown if facetDescription argument is null internal static Facet Create(FacetDescription facetDescription, object value) { return Create(facetDescription, value, false); } ////// Creates a Facet instance with the specified value for the given /// facet description. /// /// The object describing this facet /// The value of the facet /// true to bypass caching and known values; false otherwise. ///Thrown if facetDescription argument is null internal static Facet Create(FacetDescription facetDescription, object value, bool bypassKnownValues) { EntityUtil.CheckArgumentNull(facetDescription, "facetDescription"); if (!bypassKnownValues) { // Reuse facets with a null value. if (object.ReferenceEquals(value, null)) { return facetDescription.NullValueFacet; } // Reuse facets with a default value. if (object.Equals(facetDescription.DefaultValue, value)) { return facetDescription.DefaultValueFacet; } // Special case boolean facets. if (facetDescription.FacetType.Identity == "Edm.Boolean") { bool boolValue = (bool)value; return facetDescription.GetBooleanFacet(boolValue); } } Facet result = new Facet(facetDescription, value); // Check the type of the value only if we know what the correct CLR type is if (value != null && !Helper.IsUnboundedFacetValue(result) && result.FacetType.ClrType != null) { Type valueType = value.GetType(); Debug.Assert( valueType == result.FacetType.ClrType || result.FacetType.ClrType.IsAssignableFrom(valueType), string.Format(CultureInfo.CurrentCulture, "The facet {0} has type {1}, but a value of type {2} was supplied.", result.Name, result.FacetType.ClrType, valueType) ); } return result; } #endregion #region Fields ///The object describing this facet. private readonly FacetDescription _facetDescription; ///The value assigned to this facet. private readonly object _value; #endregion #region Properties ////// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.Facet; } } ////// Gets the description object for describing the facet /// public FacetDescription Description { get { return _facetDescription; } } ////// Gets/Sets the name of the facet /// [MetadataProperty(PrimitiveTypeKind.String, false)] public String Name { get { return _facetDescription.FacetName; } } ////// Gets/Sets the type of the facet /// [MetadataProperty(BuiltInTypeKind.EdmType, false)] public EdmType FacetType { get { return _facetDescription.FacetType; } } ////// Gets/Sets the value of the facet /// ///Thrown if the Facet instance is in ReadOnly state [MetadataProperty(typeof(Object), false)] public Object Value { get { return _value; } } ////// Gets the identity for this item as a string /// internal override string Identity { get { return _facetDescription.FacetName; } } ////// Indicates whether the value of the facet is unbounded /// public bool IsUnbounded { get { return object.ReferenceEquals(Value, EdmConstants.UnboundedValue); } } #endregion #region Methods ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return this.Name; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
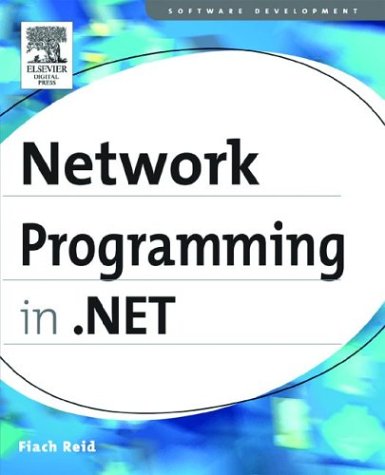
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResourceWriter.cs
- ObjectSet.cs
- RemotingService.cs
- NodeInfo.cs
- WebPartCancelEventArgs.cs
- WebPartConnectionsCloseVerb.cs
- LocalizedNameDescriptionPair.cs
- CacheAxisQuery.cs
- ToolStripItemEventArgs.cs
- MasterPageParser.cs
- HostingEnvironment.cs
- SettingsPropertyCollection.cs
- DebugTrace.cs
- ControlValuePropertyAttribute.cs
- ValidationErrorCollection.cs
- PageAdapter.cs
- ControllableStoryboardAction.cs
- DesignBindingEditor.cs
- ResourceReferenceExpression.cs
- dbdatarecord.cs
- WebPartTracker.cs
- FileDialog_Vista_Interop.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- GrammarBuilderWildcard.cs
- MatrixAnimationBase.cs
- CodeLabeledStatement.cs
- ArrayEditor.cs
- FactoryId.cs
- AstTree.cs
- ConfigurationValidatorBase.cs
- DataGridCellEditEndingEventArgs.cs
- SimpleModelProvider.cs
- GZipDecoder.cs
- FormViewDesigner.cs
- CommandValueSerializer.cs
- _ShellExpression.cs
- DataContractSerializerOperationGenerator.cs
- PropertyChangedEventArgs.cs
- InstrumentationTracker.cs
- RepeatInfo.cs
- CanonicalizationDriver.cs
- ModelVisual3D.cs
- UICuesEvent.cs
- PageAsyncTask.cs
- querybuilder.cs
- ThreadSafeList.cs
- XmlIgnoreAttribute.cs
- Compensation.cs
- DSASignatureDeformatter.cs
- Version.cs
- ComAdminInterfaces.cs
- ListenerAdapter.cs
- PseudoWebRequest.cs
- InstanceCreationEditor.cs
- SessionStateUtil.cs
- CellIdBoolean.cs
- EncodingTable.cs
- SystemColors.cs
- XmlSchema.cs
- BufferedGraphics.cs
- TextTreeNode.cs
- PageParser.cs
- XhtmlTextWriter.cs
- IndentedWriter.cs
- DataGrid.cs
- HtmlWindow.cs
- PropertyGrid.cs
- MiniCustomAttributeInfo.cs
- XmlEncoding.cs
- ServiceObjectContainer.cs
- XmlElementList.cs
- Base64Decoder.cs
- RandomDelayQueuedSendsAsyncResult.cs
- CardSpaceShim.cs
- BeginStoryboard.cs
- FrugalList.cs
- DebugHandleTracker.cs
- TemplateLookupAction.cs
- IntSecurity.cs
- WorkItem.cs
- AuthorizationRule.cs
- documentsequencetextview.cs
- XmlSchemaAnyAttribute.cs
- altserialization.cs
- Visual.cs
- Table.cs
- GridToolTip.cs
- ServicePoint.cs
- _NtlmClient.cs
- ExpandSegment.cs
- SizeAnimation.cs
- StrokeDescriptor.cs
- HttpBrowserCapabilitiesWrapper.cs
- EdmFunction.cs
- DbConnectionClosed.cs
- CollectionConverter.cs
- WebSysDisplayNameAttribute.cs
- InvalidPropValue.cs
- Base64Decoder.cs
- XmlSchemaCollection.cs