Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / RequestCachePolicyConverter.cs / 1 / RequestCachePolicyConverter.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2007 // // File: RequesetCachePolicyConverter.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using System.Net.Cache; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// RequestCachePolicyConverter Parses a RequestCachePolicy. /// public sealed class RequestCachePolicyConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// CanConvertTo - Returns whether or not this class can convert to a given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } ////// ConvertFrom - attempt to convert to a RequestCachePolicy from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a RequestCachePolicy. /// public override object ConvertFrom(ITypeDescriptorContext td, System.Globalization.CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } string s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } HttpRequestCacheLevel level = (HttpRequestCacheLevel)Enum.Parse(typeof(HttpRequestCacheLevel), s, true); return new HttpRequestCachePolicy(level); } ////// ConvertTo - Attempt to convert to the given type /// ////// The object which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the object is not null, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The policy to convert. /// The type to which to convert the policy. ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for RequestCachePolicy/HttpRequestCachePolicy, not an arbitrary class /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } HttpRequestCachePolicy httpPolicy = value as HttpRequestCachePolicy; if(httpPolicy != null) { if (destinationType == typeof(string)) { return httpPolicy.Level.ToString(); } else if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(HttpRequestCachePolicy).GetConstructor(new Type[] { typeof(HttpRequestCachePolicy) }); return new InstanceDescriptor(ci, new object[] { httpPolicy.Level }); } } //if it's not an HttpRequestCachePolicy, try a regular RequestCachePolicy RequestCachePolicy policy = value as RequestCachePolicy; if (policy != null) { if (destinationType == typeof(string)) { return policy.Level.ToString(); } else if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(RequestCachePolicy).GetConstructor(new Type[] { typeof(RequestCachePolicy) }); return new InstanceDescriptor(ci, new object[] { policy.Level }); } } throw GetConvertToException(value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2007 // // File: RequesetCachePolicyConverter.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using System.Net.Cache; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// RequestCachePolicyConverter Parses a RequestCachePolicy. /// public sealed class RequestCachePolicyConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// CanConvertTo - Returns whether or not this class can convert to a given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } ////// ConvertFrom - attempt to convert to a RequestCachePolicy from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a RequestCachePolicy. /// public override object ConvertFrom(ITypeDescriptorContext td, System.Globalization.CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } string s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } HttpRequestCacheLevel level = (HttpRequestCacheLevel)Enum.Parse(typeof(HttpRequestCacheLevel), s, true); return new HttpRequestCachePolicy(level); } ////// ConvertTo - Attempt to convert to the given type /// ////// The object which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the object is not null, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The policy to convert. /// The type to which to convert the policy. ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for RequestCachePolicy/HttpRequestCachePolicy, not an arbitrary class /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } HttpRequestCachePolicy httpPolicy = value as HttpRequestCachePolicy; if(httpPolicy != null) { if (destinationType == typeof(string)) { return httpPolicy.Level.ToString(); } else if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(HttpRequestCachePolicy).GetConstructor(new Type[] { typeof(HttpRequestCachePolicy) }); return new InstanceDescriptor(ci, new object[] { httpPolicy.Level }); } } //if it's not an HttpRequestCachePolicy, try a regular RequestCachePolicy RequestCachePolicy policy = value as RequestCachePolicy; if (policy != null) { if (destinationType == typeof(string)) { return policy.Level.ToString(); } else if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(RequestCachePolicy).GetConstructor(new Type[] { typeof(RequestCachePolicy) }); return new InstanceDescriptor(ci, new object[] { policy.Level }); } } throw GetConvertToException(value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
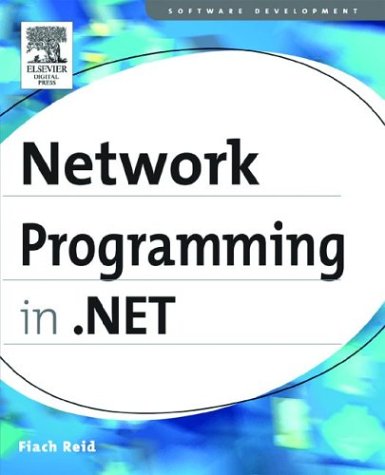
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- xmlfixedPageInfo.cs
- RuleSettingsCollection.cs
- DataGridViewCellLinkedList.cs
- BmpBitmapDecoder.cs
- MatchNoneMessageFilter.cs
- DesignerEditorPartChrome.cs
- InkPresenter.cs
- Set.cs
- ResolveMatchesMessage11.cs
- TableItemProviderWrapper.cs
- TagPrefixInfo.cs
- XmlDataDocument.cs
- HebrewCalendar.cs
- DataTableNameHandler.cs
- UnsafeNativeMethods.cs
- isolationinterop.cs
- MdiWindowListStrip.cs
- Resources.Designer.cs
- FileDetails.cs
- LogSwitch.cs
- DataRelationPropertyDescriptor.cs
- MediaCommands.cs
- __ConsoleStream.cs
- IconHelper.cs
- SqlFormatter.cs
- Geometry.cs
- ListViewItem.cs
- FieldNameLookup.cs
- ImageBrush.cs
- SqlDataSourceSelectingEventArgs.cs
- ViewKeyConstraint.cs
- XmlSchemaValidationException.cs
- XamlNamespaceHelper.cs
- DefaultBindingPropertyAttribute.cs
- TextModifierScope.cs
- AppDomain.cs
- XPathMessageFilterTable.cs
- SecurityUtils.cs
- StorageMappingItemLoader.cs
- EntityDescriptor.cs
- Triangle.cs
- NamespaceInfo.cs
- safelinkcollection.cs
- ContentPlaceHolder.cs
- Mouse.cs
- PointLightBase.cs
- PrintDialogException.cs
- ResourceReader.cs
- RegistrationServices.cs
- CodeComment.cs
- ExpressionConverter.cs
- LineMetrics.cs
- COM2EnumConverter.cs
- controlskin.cs
- NavigationHelper.cs
- MetadataSource.cs
- PlanCompiler.cs
- Cloud.cs
- TransportElement.cs
- Attributes.cs
- HttpWebRequestElement.cs
- DataColumnPropertyDescriptor.cs
- DataGridViewCell.cs
- ParseNumbers.cs
- SqlParameterizer.cs
- ThreadStaticAttribute.cs
- Suspend.cs
- MimeBasePart.cs
- WorkflowTraceTransfer.cs
- SoapAttributeAttribute.cs
- WSFederationHttpBinding.cs
- EdmToObjectNamespaceMap.cs
- DataGridViewAutoSizeModeEventArgs.cs
- HashCryptoHandle.cs
- ErrorProvider.cs
- DaylightTime.cs
- ListViewItemMouseHoverEvent.cs
- AttributeCollection.cs
- QueryOutputWriterV1.cs
- SchemaDeclBase.cs
- COAUTHINFO.cs
- Sql8ConformanceChecker.cs
- SecurityRequiresReviewAttribute.cs
- CircleHotSpot.cs
- SortDescriptionCollection.cs
- HttpWebRequest.cs
- ToolboxService.cs
- cookieexception.cs
- EdmMember.cs
- PerformanceCounterNameAttribute.cs
- RowToParametersTransformer.cs
- EmptyElement.cs
- TripleDESCryptoServiceProvider.cs
- SolidColorBrush.cs
- MatchingStyle.cs
- XmlRawWriter.cs
- LinearGradientBrush.cs
- CompletedAsyncResult.cs
- GeneralTransform.cs
- InputMethodStateTypeInfo.cs