Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / Advanced / EncoderParameters.cs / 1 / EncoderParameters.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Text; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing.Internal; using Marshal = System.Runtime.InteropServices.Marshal; using System.Drawing; //[StructLayout(LayoutKind.Sequential)] ////// /// public sealed class EncoderParameters : IDisposable { EncoderParameter[] param; ///[To be supplied.] ////// /// public EncoderParameters(int count) { param = new EncoderParameter[count]; } ///[To be supplied.] ////// /// public EncoderParameters() { param = new EncoderParameter[1]; } ///[To be supplied.] ////// /// public EncoderParameter[] Param { // get { return param; } set { param = value; } } ///[To be supplied.] ////// Copy the EncoderParameters data into a chunk of memory to be consumed by native GDI+ code. /// /// We need to marshal the EncoderParameters info from/to native GDI+ ourselve since the definition of the managed/unmanaged classes /// are different and the native class is a bit weird. The native EncoderParameters class is defined in GDI+ as follows: /// /// class EncoderParameters { /// UINT Count; // Number of parameters in this structure /// EncoderParameter Parameter[1]; // Parameter values /// }; /// /// We don't have the 'Count' field since the managed array contains it. In order for this structure to work with more than one /// EncoderParameter we need to preallocate memory for the extra n-1 elements, something like this: /// /// EncoderParameters* pEncoderParameters = (EncoderParameters*) malloc(sizeof(EncoderParameters) + (n-1) * sizeof(EncoderParameter)); /// /// Also, in 64-bit platforms, 'Count' is aligned in 8 bytes (4 extra padding bytes) so we use IntPtr instead of Int32 to account for /// that (See VSW#451333). /// internal IntPtr ConvertToMemory() { int size = Marshal.SizeOf(typeof(EncoderParameter)); IntPtr memory = Marshal.AllocHGlobal(param.Length * size + Marshal.SizeOf(typeof(IntPtr))); if (memory == IntPtr.Zero){ throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); } Marshal.WriteIntPtr(memory, (IntPtr) param.Length); long arrayOffset = (long) memory + Marshal.SizeOf(typeof(IntPtr)); for (int i=0; i/// Copy the native GDI+ EncoderParameters data from a chunk of memory into a managed EncoderParameters object. /// See ConvertToMemory for more info. /// [SuppressMessage("Microsoft.Performance", "CA1808:AvoidCallsThatBoxValueTypes")] internal static EncoderParameters ConvertFromMemory(IntPtr memory) { if (memory == IntPtr.Zero) { throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); } int count = Marshal.ReadIntPtr(memory).ToInt32(); EncoderParameters p = new EncoderParameters(count); int size = Marshal.SizeOf(typeof(EncoderParameter)); long arrayOffset = (long)memory + Marshal.SizeOf(typeof(IntPtr)); for (int i=0; i public void Dispose() { foreach (EncoderParameter p in param) { if( p != null ){ p.Dispose(); } } param = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Text; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing.Internal; using Marshal = System.Runtime.InteropServices.Marshal; using System.Drawing; //[StructLayout(LayoutKind.Sequential)] ////// /// public sealed class EncoderParameters : IDisposable { EncoderParameter[] param; ///[To be supplied.] ////// /// public EncoderParameters(int count) { param = new EncoderParameter[count]; } ///[To be supplied.] ////// /// public EncoderParameters() { param = new EncoderParameter[1]; } ///[To be supplied.] ////// /// public EncoderParameter[] Param { // get { return param; } set { param = value; } } ///[To be supplied.] ////// Copy the EncoderParameters data into a chunk of memory to be consumed by native GDI+ code. /// /// We need to marshal the EncoderParameters info from/to native GDI+ ourselve since the definition of the managed/unmanaged classes /// are different and the native class is a bit weird. The native EncoderParameters class is defined in GDI+ as follows: /// /// class EncoderParameters { /// UINT Count; // Number of parameters in this structure /// EncoderParameter Parameter[1]; // Parameter values /// }; /// /// We don't have the 'Count' field since the managed array contains it. In order for this structure to work with more than one /// EncoderParameter we need to preallocate memory for the extra n-1 elements, something like this: /// /// EncoderParameters* pEncoderParameters = (EncoderParameters*) malloc(sizeof(EncoderParameters) + (n-1) * sizeof(EncoderParameter)); /// /// Also, in 64-bit platforms, 'Count' is aligned in 8 bytes (4 extra padding bytes) so we use IntPtr instead of Int32 to account for /// that (See VSW#451333). /// internal IntPtr ConvertToMemory() { int size = Marshal.SizeOf(typeof(EncoderParameter)); IntPtr memory = Marshal.AllocHGlobal(param.Length * size + Marshal.SizeOf(typeof(IntPtr))); if (memory == IntPtr.Zero){ throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); } Marshal.WriteIntPtr(memory, (IntPtr) param.Length); long arrayOffset = (long) memory + Marshal.SizeOf(typeof(IntPtr)); for (int i=0; i/// Copy the native GDI+ EncoderParameters data from a chunk of memory into a managed EncoderParameters object. /// See ConvertToMemory for more info. /// [SuppressMessage("Microsoft.Performance", "CA1808:AvoidCallsThatBoxValueTypes")] internal static EncoderParameters ConvertFromMemory(IntPtr memory) { if (memory == IntPtr.Zero) { throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); } int count = Marshal.ReadIntPtr(memory).ToInt32(); EncoderParameters p = new EncoderParameters(count); int size = Marshal.SizeOf(typeof(EncoderParameter)); long arrayOffset = (long)memory + Marshal.SizeOf(typeof(IntPtr)); for (int i=0; i public void Dispose() { foreach (EncoderParameter p in param) { if( p != null ){ p.Dispose(); } } param = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
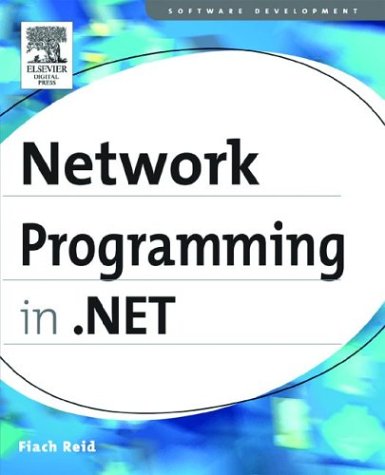
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SecurityRuntime.cs
- ContextToken.cs
- WindowsTitleBar.cs
- IteratorDescriptor.cs
- XPathNodeIterator.cs
- StringComparer.cs
- ServiceObjectContainer.cs
- KeyValuePairs.cs
- StringArrayConverter.cs
- ListParaClient.cs
- PowerEase.cs
- HttpModuleActionCollection.cs
- _ListenerResponseStream.cs
- SafeCertificateStore.cs
- AspProxy.cs
- ItemsChangedEventArgs.cs
- InternalConfigEventArgs.cs
- DateTimeStorage.cs
- GenericTextProperties.cs
- WrappedDispatcherException.cs
- OutputScopeManager.cs
- RegexCharClass.cs
- CompositeKey.cs
- XsltQilFactory.cs
- HttpListenerRequest.cs
- GridSplitter.cs
- DbDataAdapter.cs
- WebSysDisplayNameAttribute.cs
- HttpUnhandledOperationInvoker.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- NonClientArea.cs
- BitmapMetadataBlob.cs
- ValidationErrorEventArgs.cs
- MenuItemCollection.cs
- HtmlValidatorAdapter.cs
- SerializationException.cs
- ElementAction.cs
- SystemUnicastIPAddressInformation.cs
- SQLByteStorage.cs
- SafeCloseHandleCritical.cs
- OpenTypeCommon.cs
- DeclarativeCatalogPart.cs
- DrawingDrawingContext.cs
- SuspendDesigner.cs
- IisTraceWebEventProvider.cs
- WmlSelectionListAdapter.cs
- LineServicesRun.cs
- TraceInternal.cs
- RuntimeConfigLKG.cs
- DataTableNewRowEvent.cs
- ColorKeyFrameCollection.cs
- XslNumber.cs
- WpfSharedBamlSchemaContext.cs
- ExpressionQuoter.cs
- ApplicationActivator.cs
- Duration.cs
- CustomError.cs
- EmptyStringExpandableObjectConverter.cs
- ServiceDebugElement.cs
- SemaphoreSlim.cs
- XmlLoader.cs
- InputDevice.cs
- ISFClipboardData.cs
- TagPrefixInfo.cs
- OpenFileDialog.cs
- FragmentQueryKB.cs
- HMACSHA1.cs
- isolationinterop.cs
- ObjectPersistData.cs
- XamlHostingSection.cs
- Pointer.cs
- TextSelectionHelper.cs
- CacheHelper.cs
- ComboBoxRenderer.cs
- WebEventCodes.cs
- WriteStateInfoBase.cs
- CurrentTimeZone.cs
- DataPagerFieldCollection.cs
- SafeRightsManagementEnvironmentHandle.cs
- BindingListCollectionView.cs
- EntityTemplateFactory.cs
- TailPinnedEventArgs.cs
- EntityDesignerDataSourceView.cs
- UInt64.cs
- ContentDesigner.cs
- Knowncolors.cs
- DataServiceQueryProvider.cs
- Attribute.cs
- DataGridColumnFloatingHeader.cs
- MetadataArtifactLoaderCompositeFile.cs
- OptimizerPatterns.cs
- PropertyReferenceSerializer.cs
- _LocalDataStore.cs
- ZipIOExtraFieldZip64Element.cs
- BamlStream.cs
- BaseAsyncResult.cs
- CultureInfo.cs
- XmlJsonWriter.cs
- StorageAssociationTypeMapping.cs
- IRCollection.cs