Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / Security / Policy / Zone.cs / 1 / Zone.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Zone.cs // // Zone is an IIdentity representing Internet/Intranet/MyComputer etc. // namespace System.Security.Policy { using System.Security.Util; using ZoneIdentityPermission = System.Security.Permissions.ZoneIdentityPermission; using System.Runtime.CompilerServices; using System.Runtime.Serialization; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class Zone : IIdentityPermissionFactory, IBuiltInEvidence { [OptionalField(VersionAdded = 2)] private String m_url; private SecurityZone m_zone; private static readonly String[] s_names = {"MyComputer", "Intranet", "Trusted", "Internet", "Untrusted", "NoZone"}; internal Zone() { m_url = null; m_zone = SecurityZone.NoZone; } public Zone(SecurityZone zone) { if (zone < SecurityZone.NoZone || zone > SecurityZone.Untrusted) throw new ArgumentException( Environment.GetResourceString( "Argument_IllegalZone" ) ); m_url = null; m_zone = zone; } private Zone(String url) { m_url = url; m_zone = SecurityZone.NoZone; } public static Zone CreateFromUrl( String url ) { if (url == null) throw new ArgumentNullException( "url" ); return new Zone( url ); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern static SecurityZone _CreateFromUrl( String url ); public IPermission CreateIdentityPermission( Evidence evidence ) { return new ZoneIdentityPermission( SecurityZone ); } public SecurityZone SecurityZone { get { if (m_url != null) m_zone = _CreateFromUrl( m_url ); return m_zone; } } public override bool Equals(Object o) { if (o is Zone) { Zone z = (Zone) o; return SecurityZone == z.SecurityZone; } return false; } public override int GetHashCode() { return (int)SecurityZone; } public Object Copy() { Zone z = new Zone(); z.m_zone = m_zone; z.m_url = m_url; return z; } internal SecurityElement ToXml() { SecurityElement elem = new SecurityElement( "System.Security.Policy.Zone" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. BCLDebug.Assert( this.GetType().FullName.Equals( "System.Security.Policy.Zone" ), "Class name changed!" ); elem.AddAttribute( "version", "1" ); if (SecurityZone != SecurityZone.NoZone) elem.AddChild( new SecurityElement( "Zone", s_names[(int)SecurityZone] ) ); else elem.AddChild( new SecurityElement( "Zone", s_names[s_names.Length-1] ) ); return elem; } ///int IBuiltInEvidence.OutputToBuffer( char[] buffer, int position, bool verbose ) { buffer[position] = BuiltInEvidenceHelper.idZone; BuiltInEvidenceHelper.CopyIntToCharArray( (int)SecurityZone, buffer, position + 1 ); return position + 3; } /// int IBuiltInEvidence.GetRequiredSize(bool verbose) { return 3; } /// int IBuiltInEvidence.InitFromBuffer( char[] buffer, int position ) { m_url = null; m_zone = (SecurityZone)BuiltInEvidenceHelper.GetIntFromCharArray(buffer, position); return position + 2; } public override String ToString() { return ToXml().ToString(); } // INormalizeForIsolatedStorage is not implemented for startup perf // equivalent to INormalizeForIsolatedStorage.Normalize() internal Object Normalize() { return s_names[(int)SecurityZone]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Zone.cs // // Zone is an IIdentity representing Internet/Intranet/MyComputer etc. // namespace System.Security.Policy { using System.Security.Util; using ZoneIdentityPermission = System.Security.Permissions.ZoneIdentityPermission; using System.Runtime.CompilerServices; using System.Runtime.Serialization; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class Zone : IIdentityPermissionFactory, IBuiltInEvidence { [OptionalField(VersionAdded = 2)] private String m_url; private SecurityZone m_zone; private static readonly String[] s_names = {"MyComputer", "Intranet", "Trusted", "Internet", "Untrusted", "NoZone"}; internal Zone() { m_url = null; m_zone = SecurityZone.NoZone; } public Zone(SecurityZone zone) { if (zone < SecurityZone.NoZone || zone > SecurityZone.Untrusted) throw new ArgumentException( Environment.GetResourceString( "Argument_IllegalZone" ) ); m_url = null; m_zone = zone; } private Zone(String url) { m_url = url; m_zone = SecurityZone.NoZone; } public static Zone CreateFromUrl( String url ) { if (url == null) throw new ArgumentNullException( "url" ); return new Zone( url ); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern static SecurityZone _CreateFromUrl( String url ); public IPermission CreateIdentityPermission( Evidence evidence ) { return new ZoneIdentityPermission( SecurityZone ); } public SecurityZone SecurityZone { get { if (m_url != null) m_zone = _CreateFromUrl( m_url ); return m_zone; } } public override bool Equals(Object o) { if (o is Zone) { Zone z = (Zone) o; return SecurityZone == z.SecurityZone; } return false; } public override int GetHashCode() { return (int)SecurityZone; } public Object Copy() { Zone z = new Zone(); z.m_zone = m_zone; z.m_url = m_url; return z; } internal SecurityElement ToXml() { SecurityElement elem = new SecurityElement( "System.Security.Policy.Zone" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. BCLDebug.Assert( this.GetType().FullName.Equals( "System.Security.Policy.Zone" ), "Class name changed!" ); elem.AddAttribute( "version", "1" ); if (SecurityZone != SecurityZone.NoZone) elem.AddChild( new SecurityElement( "Zone", s_names[(int)SecurityZone] ) ); else elem.AddChild( new SecurityElement( "Zone", s_names[s_names.Length-1] ) ); return elem; } /// int IBuiltInEvidence.OutputToBuffer( char[] buffer, int position, bool verbose ) { buffer[position] = BuiltInEvidenceHelper.idZone; BuiltInEvidenceHelper.CopyIntToCharArray( (int)SecurityZone, buffer, position + 1 ); return position + 3; } /// int IBuiltInEvidence.GetRequiredSize(bool verbose) { return 3; } /// int IBuiltInEvidence.InitFromBuffer( char[] buffer, int position ) { m_url = null; m_zone = (SecurityZone)BuiltInEvidenceHelper.GetIntFromCharArray(buffer, position); return position + 2; } public override String ToString() { return ToXml().ToString(); } // INormalizeForIsolatedStorage is not implemented for startup perf // equivalent to INormalizeForIsolatedStorage.Normalize() internal Object Normalize() { return s_names[(int)SecurityZone]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
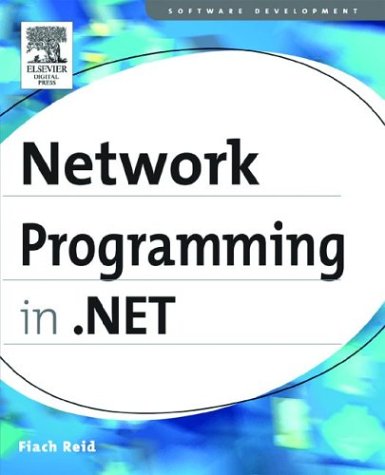
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RecordsAffectedEventArgs.cs
- Object.cs
- Vector3DIndependentAnimationStorage.cs
- WebBaseEventKeyComparer.cs
- EntityStoreSchemaGenerator.cs
- Tag.cs
- BitmapMetadata.cs
- Point3DKeyFrameCollection.cs
- DoubleLinkListEnumerator.cs
- _ConnectOverlappedAsyncResult.cs
- SqlReferenceCollection.cs
- BufferedOutputAsyncStream.cs
- Model3DCollection.cs
- SiteMapSection.cs
- SystemColorTracker.cs
- ProfilePropertySettings.cs
- TypedDataSetSchemaImporterExtension.cs
- OrderToken.cs
- StrongNameMembershipCondition.cs
- IntegerValidatorAttribute.cs
- TimeoutException.cs
- CardSpacePolicyElement.cs
- LinearKeyFrames.cs
- TextInfo.cs
- SQLBytesStorage.cs
- SqlComparer.cs
- Fault.cs
- BoolExpr.cs
- Block.cs
- StrokeNode.cs
- XmlDocument.cs
- ComponentGlyph.cs
- ValidationHelpers.cs
- BaseValidator.cs
- Utils.cs
- ExternalCalls.cs
- ProxyHwnd.cs
- HostingEnvironmentSection.cs
- XmlDocumentFragment.cs
- FunctionCommandText.cs
- FreezableOperations.cs
- BamlStream.cs
- EntitySqlQueryCacheEntry.cs
- HideDisabledControlAdapter.cs
- ConcurrentStack.cs
- SmiEventStream.cs
- XmlIlVisitor.cs
- TemplateParser.cs
- datacache.cs
- RenderDataDrawingContext.cs
- WindowsAltTab.cs
- EnumValidator.cs
- BaseTemplateCodeDomTreeGenerator.cs
- DataGridViewCellCollection.cs
- SystemResourceHost.cs
- ReaderWriterLockSlim.cs
- PropertyGeneratedEventArgs.cs
- HttpGetProtocolReflector.cs
- FirewallWrapper.cs
- SchemaTypeEmitter.cs
- SqlClientPermission.cs
- PreProcessor.cs
- SqlWebEventProvider.cs
- SoapRpcMethodAttribute.cs
- SoapReflectionImporter.cs
- RectAnimationBase.cs
- xmlsaver.cs
- SqlCachedBuffer.cs
- SqlServices.cs
- XmlSerializationReader.cs
- CompareInfo.cs
- SectionInput.cs
- DataGridViewLayoutData.cs
- ResourcePermissionBase.cs
- SourceElementsCollection.cs
- HostExecutionContextManager.cs
- SplayTreeNode.cs
- QilPatternFactory.cs
- ErrorTableItemStyle.cs
- Parameter.cs
- RelationshipEndCollection.cs
- LambdaCompiler.Unary.cs
- SuppressMergeCheckAttribute.cs
- WebReferencesBuildProvider.cs
- FastEncoder.cs
- ResourceSetExpression.cs
- StreamWithDictionary.cs
- MetadataItemCollectionFactory.cs
- DispatcherHookEventArgs.cs
- CollectionAdapters.cs
- GridViewUpdateEventArgs.cs
- TrackingMemoryStreamFactory.cs
- SerialErrors.cs
- Literal.cs
- XmlUtil.cs
- SessionStateSection.cs
- LiteralControl.cs
- ProcessProtocolHandler.cs
- DataGridViewRowEventArgs.cs
- AppDomainEvidenceFactory.cs