Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / textformatting / CharacterHit.cs / 1305600 / CharacterHit.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: The CharacterHit structure represents information about a character hit // within a glyph run - the index of the first character that got hit and the information // about leading or trailing edge. // // See spec at http://team/sites/Avalon/Specs/Glyph%20Run%20hit%20testing%20and%20caret%20placement%20API.htm#CharacterHit // // // History: // 11/30/2004 : mleonov - Created // //--------------------------------------------------------------------------- #region Using directives using System; #endregion namespace System.Windows.Media.TextFormatting { ////// The CharacterHit structure represents information about a character hit within a glyph run /// - the index of the first character that got hit and the information about leading or trailing edge. /// public struct CharacterHit : IEquatable{ /// /// Constructs a new CharacterHit structure. /// /// Index of the first character that got hit. /// In case of leading edge this value is 0. /// In case of trailing edge this value is the number of codepoints until the next valid caret position. public CharacterHit(int firstCharacterIndex, int trailingLength) { _firstCharacterIndex = firstCharacterIndex; _trailingLength = trailingLength; } ////// Index of the first character that got hit. /// public int FirstCharacterIndex { get { return _firstCharacterIndex; } } ////// In case of leading edge this value is 0. /// In case of trailing edge this value is the number of codepoints until the next valid caret position. /// public int TrailingLength { get { return _trailingLength; } } ////// Checks whether two character hit objects are equal. /// /// First object to compare. /// Second object to compare. ///Returns true when the values of FirstCharacterIndex and TrailingLength are equal for both objects, /// and false otherwise. public static bool operator==(CharacterHit left, CharacterHit right) { return left._firstCharacterIndex == right._firstCharacterIndex && left._trailingLength == right._trailingLength; } ////// Checks whether two character hit objects are not equal. /// /// First object to compare. /// Second object to compare. ///Returns false when the values of FirstCharacterIndex and TrailingLength are equal for both objects, /// and true otherwise. public static bool operator!=(CharacterHit left, CharacterHit right) { return !(left == right); } ////// Checks whether an object is equal to another character hit object. /// /// CharacterHit object to compare with. ///Returns true when the object is equal to the input object, /// and false otherwise. public bool Equals(CharacterHit obj) { return this == obj; } ////// Checks whether an object is equal to another character hit object. /// /// CharacterHit object to compare with. ///Returns true when the object is equal to the input object, /// and false otherwise. public override bool Equals(object obj) { if (!(obj is CharacterHit)) return false; return this == (CharacterHit)obj; } ////// Compute hash code for this object. /// ///A 32-bit signed integer hash code. public override int GetHashCode() { return _firstCharacterIndex.GetHashCode() ^ _trailingLength.GetHashCode(); } private int _firstCharacterIndex; private int _trailingLength; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: The CharacterHit structure represents information about a character hit // within a glyph run - the index of the first character that got hit and the information // about leading or trailing edge. // // See spec at http://team/sites/Avalon/Specs/Glyph%20Run%20hit%20testing%20and%20caret%20placement%20API.htm#CharacterHit // // // History: // 11/30/2004 : mleonov - Created // //--------------------------------------------------------------------------- #region Using directives using System; #endregion namespace System.Windows.Media.TextFormatting { ////// The CharacterHit structure represents information about a character hit within a glyph run /// - the index of the first character that got hit and the information about leading or trailing edge. /// public struct CharacterHit : IEquatable{ /// /// Constructs a new CharacterHit structure. /// /// Index of the first character that got hit. /// In case of leading edge this value is 0. /// In case of trailing edge this value is the number of codepoints until the next valid caret position. public CharacterHit(int firstCharacterIndex, int trailingLength) { _firstCharacterIndex = firstCharacterIndex; _trailingLength = trailingLength; } ////// Index of the first character that got hit. /// public int FirstCharacterIndex { get { return _firstCharacterIndex; } } ////// In case of leading edge this value is 0. /// In case of trailing edge this value is the number of codepoints until the next valid caret position. /// public int TrailingLength { get { return _trailingLength; } } ////// Checks whether two character hit objects are equal. /// /// First object to compare. /// Second object to compare. ///Returns true when the values of FirstCharacterIndex and TrailingLength are equal for both objects, /// and false otherwise. public static bool operator==(CharacterHit left, CharacterHit right) { return left._firstCharacterIndex == right._firstCharacterIndex && left._trailingLength == right._trailingLength; } ////// Checks whether two character hit objects are not equal. /// /// First object to compare. /// Second object to compare. ///Returns false when the values of FirstCharacterIndex and TrailingLength are equal for both objects, /// and true otherwise. public static bool operator!=(CharacterHit left, CharacterHit right) { return !(left == right); } ////// Checks whether an object is equal to another character hit object. /// /// CharacterHit object to compare with. ///Returns true when the object is equal to the input object, /// and false otherwise. public bool Equals(CharacterHit obj) { return this == obj; } ////// Checks whether an object is equal to another character hit object. /// /// CharacterHit object to compare with. ///Returns true when the object is equal to the input object, /// and false otherwise. public override bool Equals(object obj) { if (!(obj is CharacterHit)) return false; return this == (CharacterHit)obj; } ////// Compute hash code for this object. /// ///A 32-bit signed integer hash code. public override int GetHashCode() { return _firstCharacterIndex.GetHashCode() ^ _trailingLength.GetHashCode(); } private int _firstCharacterIndex; private int _trailingLength; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
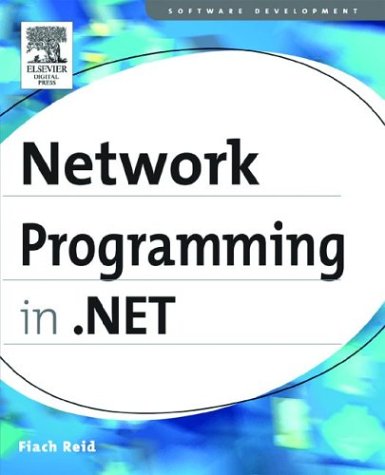
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FunctionQuery.cs
- PrintDialogDesigner.cs
- Light.cs
- MenuAutomationPeer.cs
- GradientStop.cs
- DataKeyCollection.cs
- XmlHierarchyData.cs
- StringDictionary.cs
- StorageFunctionMapping.cs
- ResourceSet.cs
- TextRangeAdaptor.cs
- WebPartChrome.cs
- Transform3DCollection.cs
- TextTreePropertyUndoUnit.cs
- _SSPIWrapper.cs
- WizardSideBarListControlItemEventArgs.cs
- Decorator.cs
- XmlTextWriter.cs
- VSWCFServiceContractGenerator.cs
- StrokeCollection2.cs
- SQLResource.cs
- SByte.cs
- QueryParameter.cs
- FlowDocumentScrollViewer.cs
- UserControlFileEditor.cs
- MenuAdapter.cs
- KeyTime.cs
- KeyEventArgs.cs
- WithStatement.cs
- DocumentReference.cs
- TypeLibConverter.cs
- PropertyDescriptorCollection.cs
- AuthenticationServiceManager.cs
- ConnectionPointConverter.cs
- DocumentXPathNavigator.cs
- ProxyGenerationError.cs
- ActiveXHelper.cs
- SelfIssuedAuthRSAPKCS1SignatureFormatter.cs
- UnsignedPublishLicense.cs
- SafeCloseHandleCritical.cs
- CalendarDateRangeChangingEventArgs.cs
- TimerEventSubscriptionCollection.cs
- ErrorEventArgs.cs
- PersistencePipeline.cs
- ReaderWriterLockSlim.cs
- ControlPersister.cs
- FilterableData.cs
- RuntimeHandles.cs
- MonthChangedEventArgs.cs
- WebBrowser.cs
- CodeDomSerializerBase.cs
- cache.cs
- IndicFontClient.cs
- DLinqDataModelProvider.cs
- Zone.cs
- FontFaceLayoutInfo.cs
- ContentDefinition.cs
- BaseValidator.cs
- SqlUtils.cs
- documentsequencetextpointer.cs
- ActiveXSite.cs
- Int16.cs
- Latin1Encoding.cs
- DataGridViewComboBoxColumnDesigner.cs
- FunctionImportElement.cs
- MatrixCamera.cs
- SByteStorage.cs
- HttpCapabilitiesBase.cs
- _PooledStream.cs
- DbConvert.cs
- InlineCollection.cs
- Material.cs
- RuntimeConfigLKG.cs
- MutableAssemblyCacheEntry.cs
- IteratorFilter.cs
- HotCommands.cs
- SecurityContext.cs
- DesignerActionGlyph.cs
- FilteredDataSetHelper.cs
- JsonQueryStringConverter.cs
- HttpValueCollection.cs
- X509Utils.cs
- BlurBitmapEffect.cs
- sqlinternaltransaction.cs
- ComponentDispatcher.cs
- HtmlHead.cs
- ProtocolViolationException.cs
- DBCSCodePageEncoding.cs
- CollectionViewGroupRoot.cs
- TrailingSpaceComparer.cs
- AssemblyAssociatedContentFileAttribute.cs
- Binding.cs
- ReferentialConstraint.cs
- LayoutInformation.cs
- OutputCacheSettings.cs
- DecoderFallbackWithFailureFlag.cs
- SelectionList.cs
- StructuredType.cs
- SmtpClient.cs
- Annotation.cs