Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Server / System / Data / Services / Serializers / IndentedTextWriter.cs / 1 / IndentedTextWriter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a writer implementation for Json format // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { using System; using System.Diagnostics; using System.Globalization; using System.IO; using System.Text; ///Writes the Json text in indented format. ////// There are many more methods implemented in previous versions /// of this file to handle more type and newline cases. /// internal sealed class IndentedTextWriter : TextWriter { ///writer to which Json text needs to be written private TextWriter writer; ///keeps track of the indentLevel private int indentLevel; ///keeps track of pending tabs private bool tabsPending; ///string representation of tab private string tabString; ////// Creates a new instance of IndentedTextWriter over the given text writer /// /// writer which IndentedTextWriter wraps public IndentedTextWriter(TextWriter writer) : base(CultureInfo.InvariantCulture) { this.writer = writer; this.tabString = " "; } ///Returns the Encoding for the given writer public override Encoding Encoding { get { return this.writer.Encoding; } } ///Returns the new line character public override string NewLine { get { return this.writer.NewLine; } } ///returns the current indent level public int Indent { get { return this.indentLevel; } set { Debug.Assert(value >= 0, "value >= 0"); if (value < 0) { value = 0; } this.indentLevel = value; } } ///Closes the underlying writer public override void Close() { this.writer.Close(); } ///Clears all the buffer of the current writer public override void Flush() { this.writer.Flush(); } ////// Writes the given string value to the underlying writer /// /// string value to be written public override void Write(string s) { this.OutputTabs(); this.writer.Write(s); } ////// Writes the given char value to the underlying writer /// /// char value to be written public override void Write(char value) { this.OutputTabs(); this.writer.Write(value); } ////// Writes the trimmed text if minimizeWhiteSpeace is set to true /// /// string value to be written public void WriteTrimmed(string text) { this.Write(text); } ///Writes the tabs depending on the indent level private void OutputTabs() { if (this.tabsPending) { for (int i = 0; i < this.indentLevel; i++) { this.writer.Write(this.tabString); } this.tabsPending = false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a writer implementation for Json format // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { using System; using System.Diagnostics; using System.Globalization; using System.IO; using System.Text; ///Writes the Json text in indented format. ////// There are many more methods implemented in previous versions /// of this file to handle more type and newline cases. /// internal sealed class IndentedTextWriter : TextWriter { ///writer to which Json text needs to be written private TextWriter writer; ///keeps track of the indentLevel private int indentLevel; ///keeps track of pending tabs private bool tabsPending; ///string representation of tab private string tabString; ////// Creates a new instance of IndentedTextWriter over the given text writer /// /// writer which IndentedTextWriter wraps public IndentedTextWriter(TextWriter writer) : base(CultureInfo.InvariantCulture) { this.writer = writer; this.tabString = " "; } ///Returns the Encoding for the given writer public override Encoding Encoding { get { return this.writer.Encoding; } } ///Returns the new line character public override string NewLine { get { return this.writer.NewLine; } } ///returns the current indent level public int Indent { get { return this.indentLevel; } set { Debug.Assert(value >= 0, "value >= 0"); if (value < 0) { value = 0; } this.indentLevel = value; } } ///Closes the underlying writer public override void Close() { this.writer.Close(); } ///Clears all the buffer of the current writer public override void Flush() { this.writer.Flush(); } ////// Writes the given string value to the underlying writer /// /// string value to be written public override void Write(string s) { this.OutputTabs(); this.writer.Write(s); } ////// Writes the given char value to the underlying writer /// /// char value to be written public override void Write(char value) { this.OutputTabs(); this.writer.Write(value); } ////// Writes the trimmed text if minimizeWhiteSpeace is set to true /// /// string value to be written public void WriteTrimmed(string text) { this.Write(text); } ///Writes the tabs depending on the indent level private void OutputTabs() { if (this.tabsPending) { for (int i = 0; i < this.indentLevel; i++) { this.writer.Write(this.tabString); } this.tabsPending = false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
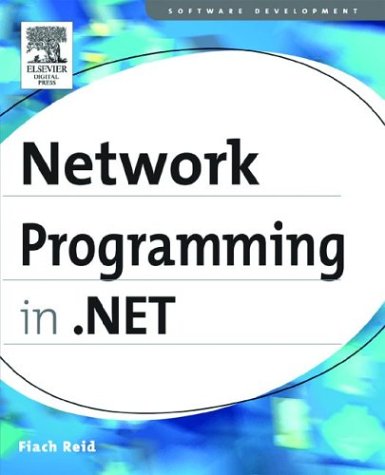
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RichTextBox.cs
- Action.cs
- UrlPath.cs
- CapabilitiesSection.cs
- BindingValueChangedEventArgs.cs
- ListViewGroup.cs
- coordinatorscratchpad.cs
- Int32AnimationBase.cs
- ObjectNotFoundException.cs
- Rotation3DKeyFrameCollection.cs
- CookieParameter.cs
- ParameterBuilder.cs
- RequestResponse.cs
- XmlDocumentFragment.cs
- ValidationException.cs
- FileDialogCustomPlace.cs
- Window.cs
- DataContractSerializer.cs
- StrongNameKeyPair.cs
- PersonalizationProviderHelper.cs
- ObjectQueryProvider.cs
- Walker.cs
- DataGridViewTextBoxCell.cs
- ToolBarPanel.cs
- MimeTypePropertyAttribute.cs
- WebPartDisplayModeEventArgs.cs
- TableLayoutStyle.cs
- ControlPaint.cs
- ParameterModifier.cs
- ListBoxItemAutomationPeer.cs
- VectorCollectionValueSerializer.cs
- BaseCollection.cs
- DodSequenceMerge.cs
- OperationResponse.cs
- XamlPointCollectionSerializer.cs
- _emptywebproxy.cs
- Root.cs
- ItemList.cs
- HttpListenerContext.cs
- ColorBlend.cs
- TypedTableBase.cs
- DelegateSerializationHolder.cs
- InternalBufferOverflowException.cs
- BreadCrumbTextConverter.cs
- AmbientLight.cs
- PermissionSet.cs
- WriteableBitmap.cs
- XmlReaderSettings.cs
- MissingMemberException.cs
- X509InitiatorCertificateServiceElement.cs
- SqlUnionizer.cs
- MeasureItemEvent.cs
- ListViewItem.cs
- JsonCollectionDataContract.cs
- Trace.cs
- EtwTrace.cs
- URLEditor.cs
- HtmlInputButton.cs
- CombinedGeometry.cs
- SimpleBitVector32.cs
- SqlPersonalizationProvider.cs
- AccessibleObject.cs
- GeneralTransform3DTo2D.cs
- BooleanFunctions.cs
- TypeBuilderInstantiation.cs
- MsmqIntegrationMessagePool.cs
- StructuredProperty.cs
- FileUpload.cs
- DocumentViewerHelper.cs
- RelationshipNavigation.cs
- ContextMenuStripActionList.cs
- EntityParameter.cs
- PropertyNames.cs
- RenderData.cs
- MarshalByRefObject.cs
- ColumnProvider.cs
- OrderedHashRepartitionStream.cs
- Property.cs
- RunInstallerAttribute.cs
- DesignBindingEditor.cs
- PreProcessor.cs
- xml.cs
- UIntPtr.cs
- XamlStackWriter.cs
- FormViewInsertEventArgs.cs
- HuffModule.cs
- WmlTextViewAdapter.cs
- SafeHandle.cs
- TypeDescriptor.cs
- StateMachineWorkflow.cs
- DefaultPrintController.cs
- Hyperlink.cs
- RelationshipEndCollection.cs
- AsnEncodedData.cs
- SafeProcessHandle.cs
- AnnotationResource.cs
- CachedFontFamily.cs
- NumberSubstitution.cs
- HttpEncoder.cs
- DataServiceQueryException.cs