Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / XmlUtils / System / Xml / Xsl / Runtime / DodSequenceMerge.cs / 1 / DodSequenceMerge.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Xml.XPath; using System.Diagnostics; using System.Globalization; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// Merges several doc-order-distinct sequences into a single doc-order-distinct sequence. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct DodSequenceMerge { private IListfirstSequence; private List > sequencesToMerge; private int nodeCount; private XmlQueryRuntime runtime; /// /// Initialize this instance of DodSequenceMerge. /// public void Create(XmlQueryRuntime runtime) { this.firstSequence = null; this.sequencesToMerge = null; this.nodeCount = 0; this.runtime = runtime; } ////// Add a new sequence to the list of sequences to merge. /// public void AddSequence(IListsequence) { // Ignore empty sequences if (sequence.Count == 0) return; if (this.firstSequence == null) { this.firstSequence = sequence; } else { if (this.sequencesToMerge == null) { this.sequencesToMerge = new List >(); MoveAndInsertSequence(this.firstSequence.GetEnumerator()); this.nodeCount = this.firstSequence.Count; } MoveAndInsertSequence(sequence.GetEnumerator()); this.nodeCount += sequence.Count; } } /// /// Return the fully merged sequence. /// public IListMergeSequences() { XmlQueryNodeSequence newSequence; // Zero sequences to merge if (this.firstSequence == null) return XmlQueryNodeSequence.Empty; // One sequence to merge if (this.sequencesToMerge == null || this.sequencesToMerge.Count <= 1) return this.firstSequence; // Two or more sequences to merge newSequence = new XmlQueryNodeSequence(this.nodeCount); while (this.sequencesToMerge.Count != 1) { // Save last item in list in temp variable, and remove it from list IEnumerator sequence = this.sequencesToMerge[this.sequencesToMerge.Count - 1]; this.sequencesToMerge.RemoveAt(this.sequencesToMerge.Count - 1); // Add current node to merged sequence newSequence.Add(sequence.Current); // Now move to the next node, and re-insert it into the list in reverse document order MoveAndInsertSequence(sequence); } // Add nodes in remaining sequence to end of list Debug.Assert(this.sequencesToMerge.Count == 1, "While loop should terminate when count == 1"); do { newSequence.Add(this.sequencesToMerge[0].Current); } while (this.sequencesToMerge[0].MoveNext()); return newSequence; } /// /// Move to the next item in the sequence. If there is no next item, then do not /// insert the sequence. Otherwise, call InsertSequence. /// private void MoveAndInsertSequence(IEnumeratorsequence) { if (sequence.MoveNext()) InsertSequence(sequence); } /// /// Insert the specified sequence into the list of sequences to be merged. /// Insert it in reverse document order with respect to the current nodes in other sequences. /// private void InsertSequence(IEnumeratorsequence) { for (int i = this.sequencesToMerge.Count - 1; i >= 0; i--) { int cmp = this.runtime.ComparePosition(sequence.Current, this.sequencesToMerge[i].Current); if (cmp == -1) { // Insert after current item this.sequencesToMerge.Insert(i + 1, sequence); return; } else if (cmp == 0) { // Found duplicate, so skip the duplicate if (!sequence.MoveNext()) { // No more nodes, so don't insert anything return; } // Next node must be after current node in document order, so don't need to reset loop } } // Insert at beginning of list this.sequencesToMerge.Insert(0, sequence); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Xml.XPath; using System.Diagnostics; using System.Globalization; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// Merges several doc-order-distinct sequences into a single doc-order-distinct sequence. /// [EditorBrowsable(EditorBrowsableState.Never)] public struct DodSequenceMerge { private IListfirstSequence; private List > sequencesToMerge; private int nodeCount; private XmlQueryRuntime runtime; /// /// Initialize this instance of DodSequenceMerge. /// public void Create(XmlQueryRuntime runtime) { this.firstSequence = null; this.sequencesToMerge = null; this.nodeCount = 0; this.runtime = runtime; } ////// Add a new sequence to the list of sequences to merge. /// public void AddSequence(IListsequence) { // Ignore empty sequences if (sequence.Count == 0) return; if (this.firstSequence == null) { this.firstSequence = sequence; } else { if (this.sequencesToMerge == null) { this.sequencesToMerge = new List >(); MoveAndInsertSequence(this.firstSequence.GetEnumerator()); this.nodeCount = this.firstSequence.Count; } MoveAndInsertSequence(sequence.GetEnumerator()); this.nodeCount += sequence.Count; } } /// /// Return the fully merged sequence. /// public IListMergeSequences() { XmlQueryNodeSequence newSequence; // Zero sequences to merge if (this.firstSequence == null) return XmlQueryNodeSequence.Empty; // One sequence to merge if (this.sequencesToMerge == null || this.sequencesToMerge.Count <= 1) return this.firstSequence; // Two or more sequences to merge newSequence = new XmlQueryNodeSequence(this.nodeCount); while (this.sequencesToMerge.Count != 1) { // Save last item in list in temp variable, and remove it from list IEnumerator sequence = this.sequencesToMerge[this.sequencesToMerge.Count - 1]; this.sequencesToMerge.RemoveAt(this.sequencesToMerge.Count - 1); // Add current node to merged sequence newSequence.Add(sequence.Current); // Now move to the next node, and re-insert it into the list in reverse document order MoveAndInsertSequence(sequence); } // Add nodes in remaining sequence to end of list Debug.Assert(this.sequencesToMerge.Count == 1, "While loop should terminate when count == 1"); do { newSequence.Add(this.sequencesToMerge[0].Current); } while (this.sequencesToMerge[0].MoveNext()); return newSequence; } /// /// Move to the next item in the sequence. If there is no next item, then do not /// insert the sequence. Otherwise, call InsertSequence. /// private void MoveAndInsertSequence(IEnumeratorsequence) { if (sequence.MoveNext()) InsertSequence(sequence); } /// /// Insert the specified sequence into the list of sequences to be merged. /// Insert it in reverse document order with respect to the current nodes in other sequences. /// private void InsertSequence(IEnumeratorsequence) { for (int i = this.sequencesToMerge.Count - 1; i >= 0; i--) { int cmp = this.runtime.ComparePosition(sequence.Current, this.sequencesToMerge[i].Current); if (cmp == -1) { // Insert after current item this.sequencesToMerge.Insert(i + 1, sequence); return; } else if (cmp == 0) { // Found duplicate, so skip the duplicate if (!sequence.MoveNext()) { // No more nodes, so don't insert anything return; } // Next node must be after current node in document order, so don't need to reset loop } } // Insert at beginning of list this.sequencesToMerge.Insert(0, sequence); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
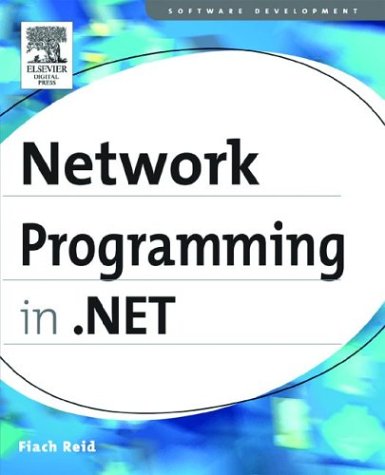
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InputChannelAcceptor.cs
- HierarchicalDataBoundControl.cs
- App.cs
- SelectionChangedEventArgs.cs
- Vector3DAnimationBase.cs
- RootProfilePropertySettingsCollection.cs
- SessionEndingCancelEventArgs.cs
- LambdaCompiler.Lambda.cs
- CompressEmulationStream.cs
- InstanceDataCollectionCollection.cs
- DecoderFallback.cs
- CopyNodeSetAction.cs
- ErrorRuntimeConfig.cs
- KnownTypesHelper.cs
- securitycriticaldata.cs
- DiscoveryReferences.cs
- ObjectViewListener.cs
- BitmapCodecInfoInternal.cs
- Table.cs
- IsolationInterop.cs
- InputScope.cs
- OperandQuery.cs
- NameValuePair.cs
- ChannelSinkStacks.cs
- CodeDefaultValueExpression.cs
- CurrentTimeZone.cs
- IListConverters.cs
- SendMailErrorEventArgs.cs
- XmlBinaryWriterSession.cs
- ReflectionHelper.cs
- cookie.cs
- CodeStatementCollection.cs
- StateChangeEvent.cs
- TemplateContainer.cs
- XmlSchemaSimpleTypeUnion.cs
- GenerateHelper.cs
- ConfigXmlSignificantWhitespace.cs
- AuthorizationRuleCollection.cs
- RelationshipWrapper.cs
- MultiBinding.cs
- UICuesEvent.cs
- ResetableIterator.cs
- TemplateBamlRecordReader.cs
- AuthenticationServiceManager.cs
- WindowsToolbar.cs
- FusionWrap.cs
- ResourceProviderFactory.cs
- CompatibleIComparer.cs
- OptimizedTemplateContentHelper.cs
- StringBuilder.cs
- PathGradientBrush.cs
- DisableDpiAwarenessAttribute.cs
- TextModifierScope.cs
- ScriptResourceInfo.cs
- WindowProviderWrapper.cs
- TableChangeProcessor.cs
- CaseDesigner.xaml.cs
- FlowDocumentView.cs
- IndexingContentUnit.cs
- DrawListViewItemEventArgs.cs
- HyperLinkColumn.cs
- WinFormsSpinner.cs
- ServiceManager.cs
- GuidelineSet.cs
- PolicyValidationException.cs
- brushes.cs
- MatchNoneMessageFilter.cs
- KnownTypesProvider.cs
- WizardForm.cs
- ConnectivityStatus.cs
- ExpressionBuilder.cs
- CommandBindingCollection.cs
- StrokeIntersection.cs
- UriTemplateQueryValue.cs
- IndentedWriter.cs
- ReaderWriterLock.cs
- StringStorage.cs
- SqlDeflator.cs
- Queue.cs
- SurrogateSelector.cs
- ConfigurationLockCollection.cs
- ScopelessEnumAttribute.cs
- ToolStripItemTextRenderEventArgs.cs
- DataGridCell.cs
- AccessorTable.cs
- ScriptControlDescriptor.cs
- HtmlLiteralTextAdapter.cs
- MultipleViewPattern.cs
- SoapCommonClasses.cs
- ClientApiGenerator.cs
- ChannelSinkStacks.cs
- NestedContainer.cs
- UInt32Storage.cs
- MouseEvent.cs
- TransactedReceiveData.cs
- OptimalTextSource.cs
- MaterializeFromAtom.cs
- XamlTypeMapper.cs
- Token.cs
- SecurityUtils.cs