Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CompMod / System / Diagnostics / Trace.cs / 1 / Trace.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ #define TRACE namespace System.Diagnostics { using System; using System.Collections; using System.Security.Permissions; using System.Threading; ////// public sealed class Trace { private static CorrelationManager correlationManager = null; // not creatble... // private Trace() { } ///Provides a set of properties and methods to trace the execution of your code. ////// public static TraceListenerCollection Listeners { [HostProtection(SharedState=true)] get { // Do a full damand new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Demand(); return TraceInternal.Listeners; } } ///Gets the collection of listeners that is monitoring the trace output. ////// public static bool AutoFlush { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { return TraceInternal.AutoFlush; } [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] set { TraceInternal.AutoFlush = value; } } public static bool UseGlobalLock { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { return TraceInternal.UseGlobalLock; } [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] set { TraceInternal.UseGlobalLock = value; } } public static CorrelationManager CorrelationManager { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { if (correlationManager == null) correlationManager = new CorrelationManager(); return correlationManager; } } ////// Gets or sets whether ///should be called on the after every write. /// /// public static int IndentLevel { get { return TraceInternal.IndentLevel; } set { TraceInternal.IndentLevel = value; } } ///Gets or sets the indent level. ////// public static int IndentSize { get { return TraceInternal.IndentSize; } set { TraceInternal.IndentSize = value; } } ////// Gets or sets the number of spaces in an indent. /// ////// [System.Diagnostics.Conditional("TRACE")] public static void Flush() { TraceInternal.Flush(); } ///Clears the output buffer, and causes buffered data to /// be written to the ///. /// [System.Diagnostics.Conditional("TRACE")] public static void Close() { // Do a full damand new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Demand(); TraceInternal.Close(); } ///Clears the output buffer, and then closes the ///so that they no /// longer receive debugging output. /// [System.Diagnostics.Conditional("TRACE")] public static void Assert(bool condition) { TraceInternal.Assert(condition); } ///Checks for a condition, and outputs the callstack if the /// condition /// is ///. /// [System.Diagnostics.Conditional("TRACE")] public static void Assert(bool condition, string message) { TraceInternal.Assert(condition, message); } ///Checks for a condition, and displays a message if the condition is /// ///. /// [System.Diagnostics.Conditional("TRACE")] public static void Assert(bool condition, string message, string detailMessage) { TraceInternal.Assert(condition, message, detailMessage); } ///Checks for a condition, and displays both messages if the condition /// is ///. /// [System.Diagnostics.Conditional("TRACE")] public static void Fail(string message) { TraceInternal.Fail(message); } ///Emits or displays a message for an assertion that always fails. ////// [System.Diagnostics.Conditional("TRACE")] public static void Fail(string message, string detailMessage) { TraceInternal.Fail(message, detailMessage); } public static void Refresh() { DiagnosticsConfiguration.Refresh(); Switch.RefreshAll(); TraceSource.RefreshAll(); } [System.Diagnostics.Conditional("TRACE")] public static void TraceInformation(string message) { TraceInternal.TraceEvent(TraceEventType.Information, 0, message, null); } [System.Diagnostics.Conditional("TRACE")] public static void TraceInformation(string format, params object[] args) { TraceInternal.TraceEvent(TraceEventType.Information, 0, format, args); } [System.Diagnostics.Conditional("TRACE")] public static void TraceWarning(string message) { TraceInternal.TraceEvent(TraceEventType.Warning, 0, message, null); } [System.Diagnostics.Conditional("TRACE")] public static void TraceWarning(string format, params object[] args) { TraceInternal.TraceEvent(TraceEventType.Warning, 0, format, args); } [System.Diagnostics.Conditional("TRACE")] public static void TraceError(string message) { TraceInternal.TraceEvent(TraceEventType.Error, 0, message, null); } [System.Diagnostics.Conditional("TRACE")] public static void TraceError(string format, params object[] args) { TraceInternal.TraceEvent(TraceEventType.Error, 0, format, args); } ///Emits or displays both messages for an assertion that always fails. ////// [System.Diagnostics.Conditional("TRACE")] public static void Write(string message) { TraceInternal.Write(message); } ///Writes a message to the trace listeners in the ////// collection. /// [System.Diagnostics.Conditional("TRACE")] public static void Write(object value) { TraceInternal.Write(value); } ///Writes the name of the ////// parameter to the trace listeners in the collection. /// [System.Diagnostics.Conditional("TRACE")] public static void Write(string message, string category) { TraceInternal.Write(message, category); } ///Writes a category name and message to the trace listeners /// in the ///collection. /// [System.Diagnostics.Conditional("TRACE")] public static void Write(object value, string category) { TraceInternal.Write(value, category); } ///Writes a category name and the name of the value parameter to the trace listeners /// in the ///collection. /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLine(string message) { TraceInternal.WriteLine(message); } ///Writes a message followed by a line terminator to the /// trace listeners in the ///collection. /// The default line terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLine(object value) { TraceInternal.WriteLine(value); } ///Writes the name of the ///parameter followed by a line terminator to the trace listeners in the collection. The default line /// terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLine(string message, string category) { TraceInternal.WriteLine(message, category); } ///Writes a category name and message followed by a line terminator to the trace /// listeners in the ////// collection. The default line terminator is a carriage return followed by a line /// feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLine(object value, string category) { TraceInternal.WriteLine(value, category); } ///Writes a ///name and the name of the parameter followed by a line /// terminator to the trace listeners in the collection. The default line /// terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteIf(bool condition, string message) { TraceInternal.WriteIf(condition, message); } ///Writes a message to the trace listeners in the ///collection /// if a condition is . /// [System.Diagnostics.Conditional("TRACE")] public static void WriteIf(bool condition, object value) { TraceInternal.WriteIf(condition, value); } ///Writes the name of the ////// parameter to the trace listeners in the collection if a condition is /// . /// [System.Diagnostics.Conditional("TRACE")] public static void WriteIf(bool condition, string message, string category) { TraceInternal.WriteIf(condition, message, category); } ///Writes a category name and message to the trace listeners in the ////// collection if a condition is . /// [System.Diagnostics.Conditional("TRACE")] public static void WriteIf(bool condition, object value, string category) { TraceInternal.WriteIf(condition, value, category); } ///Writes a category name and the name of the ///parameter to the trace /// listeners in the collection /// if a condition is . /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLineIf(bool condition, string message) { TraceInternal.WriteLineIf(condition, message); } ///Writes a message followed by a line terminator to the trace listeners in the /// ///collection if a condition is /// . The default line terminator is a carriage return followed /// by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLineIf(bool condition, object value) { TraceInternal.WriteLineIf(condition, value); } ///Writes the name of the ///parameter followed by a line terminator to the /// trace listeners in the collection /// if a condition is /// . The default line /// terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLineIf(bool condition, string message, string category) { TraceInternal.WriteLineIf(condition, message, category); } ///Writes a category name and message followed by a line terminator to the trace /// listeners in the ///collection if a condition is /// . The default line terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLineIf(bool condition, object value, string category) { TraceInternal.WriteLineIf(condition, value, category); } ///Writes a category name and the name of the ///parameter followed by a line /// terminator to the trace listeners in the collection /// if a is . The /// default line terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void Indent() { TraceInternal.Indent(); } ///[To be supplied.] ////// [System.Diagnostics.Conditional("TRACE")] public static void Unindent() { TraceInternal.Unindent(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ #define TRACE namespace System.Diagnostics { using System; using System.Collections; using System.Security.Permissions; using System.Threading; ////// public sealed class Trace { private static CorrelationManager correlationManager = null; // not creatble... // private Trace() { } ///Provides a set of properties and methods to trace the execution of your code. ////// public static TraceListenerCollection Listeners { [HostProtection(SharedState=true)] get { // Do a full damand new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Demand(); return TraceInternal.Listeners; } } ///Gets the collection of listeners that is monitoring the trace output. ////// public static bool AutoFlush { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { return TraceInternal.AutoFlush; } [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] set { TraceInternal.AutoFlush = value; } } public static bool UseGlobalLock { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { return TraceInternal.UseGlobalLock; } [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] set { TraceInternal.UseGlobalLock = value; } } public static CorrelationManager CorrelationManager { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { if (correlationManager == null) correlationManager = new CorrelationManager(); return correlationManager; } } ////// Gets or sets whether ///should be called on the after every write. /// /// public static int IndentLevel { get { return TraceInternal.IndentLevel; } set { TraceInternal.IndentLevel = value; } } ///Gets or sets the indent level. ////// public static int IndentSize { get { return TraceInternal.IndentSize; } set { TraceInternal.IndentSize = value; } } ////// Gets or sets the number of spaces in an indent. /// ////// [System.Diagnostics.Conditional("TRACE")] public static void Flush() { TraceInternal.Flush(); } ///Clears the output buffer, and causes buffered data to /// be written to the ///. /// [System.Diagnostics.Conditional("TRACE")] public static void Close() { // Do a full damand new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Demand(); TraceInternal.Close(); } ///Clears the output buffer, and then closes the ///so that they no /// longer receive debugging output. /// [System.Diagnostics.Conditional("TRACE")] public static void Assert(bool condition) { TraceInternal.Assert(condition); } ///Checks for a condition, and outputs the callstack if the /// condition /// is ///. /// [System.Diagnostics.Conditional("TRACE")] public static void Assert(bool condition, string message) { TraceInternal.Assert(condition, message); } ///Checks for a condition, and displays a message if the condition is /// ///. /// [System.Diagnostics.Conditional("TRACE")] public static void Assert(bool condition, string message, string detailMessage) { TraceInternal.Assert(condition, message, detailMessage); } ///Checks for a condition, and displays both messages if the condition /// is ///. /// [System.Diagnostics.Conditional("TRACE")] public static void Fail(string message) { TraceInternal.Fail(message); } ///Emits or displays a message for an assertion that always fails. ////// [System.Diagnostics.Conditional("TRACE")] public static void Fail(string message, string detailMessage) { TraceInternal.Fail(message, detailMessage); } public static void Refresh() { DiagnosticsConfiguration.Refresh(); Switch.RefreshAll(); TraceSource.RefreshAll(); } [System.Diagnostics.Conditional("TRACE")] public static void TraceInformation(string message) { TraceInternal.TraceEvent(TraceEventType.Information, 0, message, null); } [System.Diagnostics.Conditional("TRACE")] public static void TraceInformation(string format, params object[] args) { TraceInternal.TraceEvent(TraceEventType.Information, 0, format, args); } [System.Diagnostics.Conditional("TRACE")] public static void TraceWarning(string message) { TraceInternal.TraceEvent(TraceEventType.Warning, 0, message, null); } [System.Diagnostics.Conditional("TRACE")] public static void TraceWarning(string format, params object[] args) { TraceInternal.TraceEvent(TraceEventType.Warning, 0, format, args); } [System.Diagnostics.Conditional("TRACE")] public static void TraceError(string message) { TraceInternal.TraceEvent(TraceEventType.Error, 0, message, null); } [System.Diagnostics.Conditional("TRACE")] public static void TraceError(string format, params object[] args) { TraceInternal.TraceEvent(TraceEventType.Error, 0, format, args); } ///Emits or displays both messages for an assertion that always fails. ////// [System.Diagnostics.Conditional("TRACE")] public static void Write(string message) { TraceInternal.Write(message); } ///Writes a message to the trace listeners in the ////// collection. /// [System.Diagnostics.Conditional("TRACE")] public static void Write(object value) { TraceInternal.Write(value); } ///Writes the name of the ////// parameter to the trace listeners in the collection. /// [System.Diagnostics.Conditional("TRACE")] public static void Write(string message, string category) { TraceInternal.Write(message, category); } ///Writes a category name and message to the trace listeners /// in the ///collection. /// [System.Diagnostics.Conditional("TRACE")] public static void Write(object value, string category) { TraceInternal.Write(value, category); } ///Writes a category name and the name of the value parameter to the trace listeners /// in the ///collection. /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLine(string message) { TraceInternal.WriteLine(message); } ///Writes a message followed by a line terminator to the /// trace listeners in the ///collection. /// The default line terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLine(object value) { TraceInternal.WriteLine(value); } ///Writes the name of the ///parameter followed by a line terminator to the trace listeners in the collection. The default line /// terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLine(string message, string category) { TraceInternal.WriteLine(message, category); } ///Writes a category name and message followed by a line terminator to the trace /// listeners in the ////// collection. The default line terminator is a carriage return followed by a line /// feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLine(object value, string category) { TraceInternal.WriteLine(value, category); } ///Writes a ///name and the name of the parameter followed by a line /// terminator to the trace listeners in the collection. The default line /// terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteIf(bool condition, string message) { TraceInternal.WriteIf(condition, message); } ///Writes a message to the trace listeners in the ///collection /// if a condition is . /// [System.Diagnostics.Conditional("TRACE")] public static void WriteIf(bool condition, object value) { TraceInternal.WriteIf(condition, value); } ///Writes the name of the ////// parameter to the trace listeners in the collection if a condition is /// . /// [System.Diagnostics.Conditional("TRACE")] public static void WriteIf(bool condition, string message, string category) { TraceInternal.WriteIf(condition, message, category); } ///Writes a category name and message to the trace listeners in the ////// collection if a condition is . /// [System.Diagnostics.Conditional("TRACE")] public static void WriteIf(bool condition, object value, string category) { TraceInternal.WriteIf(condition, value, category); } ///Writes a category name and the name of the ///parameter to the trace /// listeners in the collection /// if a condition is . /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLineIf(bool condition, string message) { TraceInternal.WriteLineIf(condition, message); } ///Writes a message followed by a line terminator to the trace listeners in the /// ///collection if a condition is /// . The default line terminator is a carriage return followed /// by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLineIf(bool condition, object value) { TraceInternal.WriteLineIf(condition, value); } ///Writes the name of the ///parameter followed by a line terminator to the /// trace listeners in the collection /// if a condition is /// . The default line /// terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLineIf(bool condition, string message, string category) { TraceInternal.WriteLineIf(condition, message, category); } ///Writes a category name and message followed by a line terminator to the trace /// listeners in the ///collection if a condition is /// . The default line terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void WriteLineIf(bool condition, object value, string category) { TraceInternal.WriteLineIf(condition, value, category); } ///Writes a category name and the name of the ///parameter followed by a line /// terminator to the trace listeners in the collection /// if a is . The /// default line terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("TRACE")] public static void Indent() { TraceInternal.Indent(); } ///[To be supplied.] ////// [System.Diagnostics.Conditional("TRACE")] public static void Unindent() { TraceInternal.Unindent(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
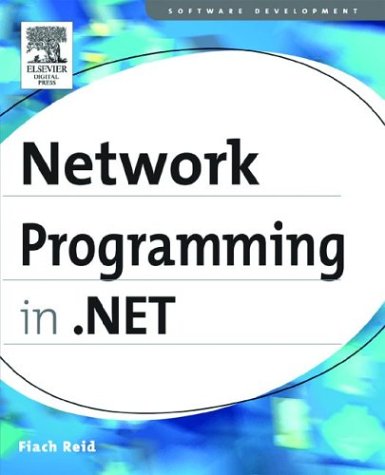
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Certificate.cs
- ValidationSummary.cs
- WindowsPen.cs
- SspiSafeHandles.cs
- IdentityManager.cs
- XmlCharacterData.cs
- OleDbEnumerator.cs
- WebPartCatalogAddVerb.cs
- AppSettingsExpressionBuilder.cs
- PackagePartCollection.cs
- PrivilegeNotHeldException.cs
- OpenTypeCommon.cs
- XmlUnspecifiedAttribute.cs
- KeyboardNavigation.cs
- EnumValAlphaComparer.cs
- WebPartEditorApplyVerb.cs
- FormsIdentity.cs
- GridViewRowCollection.cs
- SelectManyQueryOperator.cs
- ArgumentValidation.cs
- GenericTypeParameterBuilder.cs
- BitmapCodecInfoInternal.cs
- DuplexChannelBinder.cs
- X509CertificateClaimSet.cs
- ComplexTypeEmitter.cs
- StreamUpgradeProvider.cs
- MultiSelectRootGridEntry.cs
- RunWorkerCompletedEventArgs.cs
- sqlpipe.cs
- DataObjectSettingDataEventArgs.cs
- SwitchLevelAttribute.cs
- ObjectHandle.cs
- DesignerCommandAdapter.cs
- EditorPart.cs
- Int64Storage.cs
- OleDbPropertySetGuid.cs
- SQLSingleStorage.cs
- HttpAsyncResult.cs
- FreeFormPanel.cs
- documentsequencetextpointer.cs
- FileLogRecord.cs
- EntityKeyElement.cs
- DateTimePicker.cs
- Point3DAnimationUsingKeyFrames.cs
- RootDesignerSerializerAttribute.cs
- ReadWriteObjectLock.cs
- Binding.cs
- CuspData.cs
- TreeViewDataItemAutomationPeer.cs
- DataFieldConverter.cs
- NetworkInformationException.cs
- MatrixValueSerializer.cs
- HostDesigntimeLicenseContext.cs
- LambdaCompiler.Expressions.cs
- DynamicResourceExtension.cs
- GroupDescription.cs
- TabPage.cs
- DesignBindingPicker.cs
- SynchronizedDispatch.cs
- ConnectionsZone.cs
- SamlConstants.cs
- HttpCookie.cs
- XmlDictionaryString.cs
- ChannelServices.cs
- ExcCanonicalXml.cs
- BindingValueChangedEventArgs.cs
- RuntimeEnvironment.cs
- ClonableStack.cs
- PointIndependentAnimationStorage.cs
- GridSplitter.cs
- Style.cs
- ExtensibleSyndicationObject.cs
- DbConnectionOptions.cs
- ColorContextHelper.cs
- BasicBrowserDialog.designer.cs
- SourceItem.cs
- LoginUtil.cs
- ConfigXmlText.cs
- NonPrimarySelectionGlyph.cs
- TransformGroup.cs
- ToolStripDropDownItemDesigner.cs
- ConfigXmlWhitespace.cs
- TimerExtension.cs
- KeyFrames.cs
- DateTimeFormatInfoScanner.cs
- SchemaElementLookUpTable.cs
- SqlProviderManifest.cs
- RecipientServiceModelSecurityTokenRequirement.cs
- EventLogSession.cs
- DataGridViewCheckBoxColumn.cs
- HotCommands.cs
- RegexParser.cs
- StorageConditionPropertyMapping.cs
- SafeRightsManagementPubHandle.cs
- TableAutomationPeer.cs
- ControlOperationInvoker.cs
- CollectionBuilder.cs
- MsdtcClusterUtils.cs
- ControlValuePropertyAttribute.cs
- filewebrequest.cs