Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Annotations / Anchoring / TreeNodeSelectionProcessor.cs / 1305600 / TreeNodeSelectionProcessor.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // TreeNodeSelectionProcessor is a selection processor // that can handle Logical Tree Nodes as selections. // Spec: http://team/sites/ag/Specifications/Anchoring%20Namespace%20Spec.doc // // History: // 8/8/2003: magedz: Created // 08/18/2003: rruiz: Updated to Anchoring Namespace Spec. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Globalization; using System.Windows; using System.Windows.Annotations; using System.Windows.Annotations.Storage; using System.Windows.Documents; using System.Windows.Media; using System.Xml; using MS.Utility; namespace MS.Internal.Annotations.Anchoring { ////// TreeNodeSelectionProcessor is a selection processor /// that can handle Logical Tree Nodes as selections. /// internal sealed class TreeNodeSelectionProcessor : SelectionProcessor { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of TreeNodeSelectionProcessor. /// public TreeNodeSelectionProcessor() { } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Merges the two selections into one, if possible. /// /// selection to merge /// other selection to merge /// new selection that contains the data from both /// selection1 and selection2 ///always returns false, Logical Tree Nodes cannot be merged /// ///selection1 or selection2 are /// null public override bool MergeSelections(Object selection1, Object selection2, out Object newSelection) { if (selection1 == null) throw new ArgumentNullException("selection1"); if (selection2 == null) throw new ArgumentNullException("selection2"); newSelection = null; return false; } ////// Gets the tree elements spanned by the selection. /// /// the selection to examine ///a list of elements spanned by the selection; never returns /// null ///selection is null ///selection is of wrong type public override IListGetSelectedNodes(Object selection) { return new DependencyObject[] { GetParent(selection) }; } /// /// Gets the parent element of this selection. /// /// the selection to examine ///the parent element of the selection; can be null ///selection is null ///selection is of wrong type public override UIElement GetParent(Object selection) { if (selection == null) throw new ArgumentNullException("selection"); UIElement element = selection as UIElement; if (element == null) { throw new ArgumentException(SR.Get(SRID.WrongSelectionType), "selection"); } return element; } ////// Gets the anchor point for the selection /// /// the selection to examine ///the anchor point of the selection; can be null ///selection is null ///selection is of wrong type public override Point GetAnchorPoint(Object selection) { if (selection == null) throw new ArgumentNullException("selection"); Visual element = selection as Visual; if (element == null) throw new ArgumentException(SR.Get(SRID.WrongSelectionType), "selection"); // get the Visual's bounding rectangle's let, top and store them in a point Rect rect = element.VisualContentBounds; return new Point(rect.Left, rect.Top); } ////// Creates one or more locator parts representing the portion /// of 'startNode' spanned by 'selection'. /// /// the selection that is being processed /// the node the locator parts should be in the /// context of ///one or more locator parts representing the portion of 'startNode' spanned /// by 'selection' ///startNode or selection is null ///startNode is not a DependencyObject or /// selection is of the wrong type public override IListGenerateLocatorParts(Object selection, DependencyObject startNode) { if (startNode == null) throw new ArgumentNullException("startNode"); if (selection == null) throw new ArgumentNullException("selection"); return new List (0); } /// /// Creates a selection object spanning the portion of 'startNode' /// specified by 'locatorPart'. /// /// locator part specifying data to be spanned /// the node to be spanned by the created /// selection /// always set to AttachmentLevel.Full ///a selection spanning the portion of 'startNode' specified by /// 'locatorPart' ///locatorPart or startNode are /// null ///locatorPart is of the incorrect type public override Object ResolveLocatorPart(ContentLocatorPart locatorPart, DependencyObject startNode, out AttachmentLevel attachmentLevel) { if (startNode == null) throw new ArgumentNullException("startNode"); if (locatorPart == null) throw new ArgumentNullException("locatorPart"); attachmentLevel = AttachmentLevel.Full; return startNode; } ////// Returns a list of XmlQualifiedNames representing the /// the locator parts this processor can resolve/generate. /// public override XmlQualifiedName[] GetLocatorPartTypes() { return (XmlQualifiedName[])LocatorPartTypeNames.Clone(); } #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //----------------------------------------------------- //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // ContentLocatorPart types understood by this processor private static readonly XmlQualifiedName[] LocatorPartTypeNames = new XmlQualifiedName[0]; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
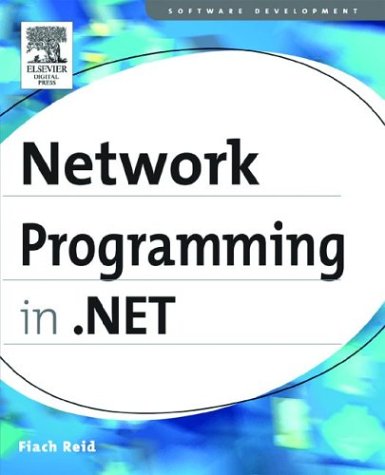
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PersistChildrenAttribute.cs
- ImpersonateTokenRef.cs
- OutputScope.cs
- StringArrayEditor.cs
- ActivityDesignerResources.cs
- XmlSchemaType.cs
- UncommonField.cs
- ExternalFile.cs
- NotCondition.cs
- AnimationStorage.cs
- TextBox.cs
- Gdiplus.cs
- HtmlMeta.cs
- DependencyPropertyAttribute.cs
- GPRECTF.cs
- KeyInterop.cs
- ListViewGroupConverter.cs
- SecurityDocument.cs
- PrintControllerWithStatusDialog.cs
- GridViewColumnHeader.cs
- DataGridViewComboBoxEditingControl.cs
- StylusTip.cs
- HttpHandlerAction.cs
- HitTestWithPointDrawingContextWalker.cs
- SecurityManager.cs
- VarRemapper.cs
- __Error.cs
- DbConnectionHelper.cs
- BindingsCollection.cs
- XPathParser.cs
- QilStrConcatenator.cs
- MultiAsyncResult.cs
- TabControlEvent.cs
- BoundsDrawingContextWalker.cs
- ExceptionRoutedEventArgs.cs
- Point3D.cs
- DrawingVisual.cs
- SafeEventLogWriteHandle.cs
- COM2FontConverter.cs
- Error.cs
- JapaneseLunisolarCalendar.cs
- ExtensionFile.cs
- WhitespaceRuleReader.cs
- TreeIterators.cs
- Variant.cs
- AsymmetricCryptoHandle.cs
- ThreadStateException.cs
- TranslateTransform.cs
- QilChoice.cs
- SecurityResources.cs
- PropertyFilterAttribute.cs
- Internal.cs
- ToolStripGripRenderEventArgs.cs
- ServiceHost.cs
- ChildDocumentBlock.cs
- AsmxEndpointPickerExtension.cs
- PreviewControlDesigner.cs
- XmlArrayAttribute.cs
- DecimalConstantAttribute.cs
- BitmapDecoder.cs
- SatelliteContractVersionAttribute.cs
- SplineKeyFrames.cs
- FormatControl.cs
- FullTextBreakpoint.cs
- DataBindingCollection.cs
- WebPartTransformerCollection.cs
- ToolTipAutomationPeer.cs
- PageParserFilter.cs
- ProxyWebPart.cs
- OracleBFile.cs
- FontFamilyConverter.cs
- WorkerRequest.cs
- ApplicationGesture.cs
- SqlDataReaderSmi.cs
- XsdBuilder.cs
- SmiEventStream.cs
- CalendarAutomationPeer.cs
- OleDbConnectionInternal.cs
- ByteStreamGeometryContext.cs
- InputScope.cs
- AccessDataSource.cs
- columnmapfactory.cs
- CodeSnippetTypeMember.cs
- TextDecoration.cs
- DefaultProfileManager.cs
- TokenBasedSetEnumerator.cs
- PageThemeParser.cs
- PkcsMisc.cs
- NamespaceDecl.cs
- BinaryWriter.cs
- SHA1.cs
- RsaSecurityKey.cs
- NamedElement.cs
- ChannelSinkStacks.cs
- PartialTrustHelpers.cs
- FrameworkPropertyMetadata.cs
- Mouse.cs
- EntitySetDataBindingList.cs
- HtmlControl.cs
- UpdatableWrapper.cs