Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripStatusLabel.cs / 1305376 / ToolStripStatusLabel.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.ComponentModel; using System.Drawing; using System.Drawing.Design; using System.Diagnostics; using System.Windows.Forms.ButtonInternal; using System.Security.Permissions; using System.Security; using System.Windows.Forms.Layout; using System.Windows.Forms.Design; ////// /// A non selectable winbar item /// [ToolStripItemDesignerAvailability(ToolStripItemDesignerAvailability.StatusStrip)] public class ToolStripStatusLabel : ToolStripLabel { private Border3DStyle borderStyle = Border3DStyle.Flat; private ToolStripStatusLabelBorderSides borderSides = ToolStripStatusLabelBorderSides.None; private bool spring = false; ////// /// A non selectable winbar item /// public ToolStripStatusLabel() { } public ToolStripStatusLabel(string text):base(text,null,false,null) { } public ToolStripStatusLabel(Image image):base(null,image,false,null) { } public ToolStripStatusLabel(string text, Image image):base(text,image,false,null) { } public ToolStripStatusLabel(string text, Image image, EventHandler onClick):base(text,image,/*isLink=*/false,onClick,null) { } public ToolStripStatusLabel(string text, Image image, EventHandler onClick, string name) :base(text,image,/*isLink=*/false,onClick, name) { } ////// Creates an instance of the object that defines how image and text /// gets laid out in the ToolStripItem /// internal override ToolStripItemInternalLayout CreateInternalLayout() { return new ToolStripStatusLabelLayout(this); } [Browsable(false)] [EditorBrowsable(EditorBrowsableState.Advanced)] public new ToolStripItemAlignment Alignment { get { return base.Alignment; } set { base.Alignment = value; } } ///[ DefaultValue(Border3DStyle.Flat), SRDescription(SR.ToolStripStatusLabelBorderStyleDescr), SRCategory(SR.CatAppearance) ] public Border3DStyle BorderStyle { get { return borderStyle; } set { if (!ClientUtils.IsEnumValid_NotSequential(value, (int)value, (int)Border3DStyle.Adjust, (int)Border3DStyle.Bump, (int)Border3DStyle.Etched, (int)Border3DStyle.Flat, (int)Border3DStyle.Raised, (int)Border3DStyle.RaisedInner, (int)Border3DStyle.RaisedOuter, (int)Border3DStyle.Sunken, (int)Border3DStyle.SunkenInner, (int)Border3DStyle.SunkenOuter )) { throw new InvalidEnumArgumentException("value", (int)value, typeof(Border3DStyle)); } if (borderStyle != value) { borderStyle = value; Invalidate(); } } } /// [ DefaultValue(ToolStripStatusLabelBorderSides.None), SRDescription(SR.ToolStripStatusLabelBorderSidesDescr), SRCategory(SR.CatAppearance) ] public ToolStripStatusLabelBorderSides BorderSides { get { return borderSides; } set { // no Enum.IsDefined as this is a flags enum. if (borderSides != value) { borderSides = value; LayoutTransaction.DoLayout(Owner,this, PropertyNames.BorderStyle); Invalidate(); } } } protected internal override Padding DefaultMargin { get { return new Padding(0, 3, 0, 2); } } [ DefaultValue(false), SRDescription(SR.ToolStripStatusLabelSpringDescr), SRCategory(SR.CatAppearance) ] public bool Spring { get { return spring; } set { if (spring != value) { spring = value; if (ParentInternal != null) { LayoutTransaction.DoLayout(ParentInternal, this, PropertyNames.Spring); } } } } public override System.Drawing.Size GetPreferredSize(System.Drawing.Size constrainingSize) { if (BorderSides != ToolStripStatusLabelBorderSides.None) { return base.GetPreferredSize(constrainingSize) + new Size(4, 4); } else { return base.GetPreferredSize(constrainingSize); } } /// /// /// Inheriting classes should override this method to handle this event. /// protected override void OnPaint(System.Windows.Forms.PaintEventArgs e) { if (this.Owner != null) { ToolStripRenderer renderer = this.Renderer; renderer.DrawToolStripStatusLabelBackground(new ToolStripItemRenderEventArgs(e.Graphics, this)); if ((DisplayStyle & ToolStripItemDisplayStyle.Image) == ToolStripItemDisplayStyle.Image) { renderer.DrawItemImage(new ToolStripItemImageRenderEventArgs(e.Graphics, this, InternalLayout.ImageRectangle)); } PaintText(e.Graphics); } } ////// This class performs internal layout for the "split button button" portion of a split button. /// Its main job is to make sure the inner button has the same parent as the split button, so /// that layout can be performed using the correct graphics context. /// private class ToolStripStatusLabelLayout : ToolStripItemInternalLayout { ToolStripStatusLabel owner; public ToolStripStatusLabelLayout(ToolStripStatusLabel owner) : base(owner) { this.owner = owner; } protected override ToolStripItemLayoutOptions CommonLayoutOptions() { ToolStripItemLayoutOptions layoutOptions = base.CommonLayoutOptions(); layoutOptions.borderSize = 0; return layoutOptions; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.ComponentModel; using System.Drawing; using System.Drawing.Design; using System.Diagnostics; using System.Windows.Forms.ButtonInternal; using System.Security.Permissions; using System.Security; using System.Windows.Forms.Layout; using System.Windows.Forms.Design; ////// /// A non selectable winbar item /// [ToolStripItemDesignerAvailability(ToolStripItemDesignerAvailability.StatusStrip)] public class ToolStripStatusLabel : ToolStripLabel { private Border3DStyle borderStyle = Border3DStyle.Flat; private ToolStripStatusLabelBorderSides borderSides = ToolStripStatusLabelBorderSides.None; private bool spring = false; ////// /// A non selectable winbar item /// public ToolStripStatusLabel() { } public ToolStripStatusLabel(string text):base(text,null,false,null) { } public ToolStripStatusLabel(Image image):base(null,image,false,null) { } public ToolStripStatusLabel(string text, Image image):base(text,image,false,null) { } public ToolStripStatusLabel(string text, Image image, EventHandler onClick):base(text,image,/*isLink=*/false,onClick,null) { } public ToolStripStatusLabel(string text, Image image, EventHandler onClick, string name) :base(text,image,/*isLink=*/false,onClick, name) { } ////// Creates an instance of the object that defines how image and text /// gets laid out in the ToolStripItem /// internal override ToolStripItemInternalLayout CreateInternalLayout() { return new ToolStripStatusLabelLayout(this); } [Browsable(false)] [EditorBrowsable(EditorBrowsableState.Advanced)] public new ToolStripItemAlignment Alignment { get { return base.Alignment; } set { base.Alignment = value; } } ///[ DefaultValue(Border3DStyle.Flat), SRDescription(SR.ToolStripStatusLabelBorderStyleDescr), SRCategory(SR.CatAppearance) ] public Border3DStyle BorderStyle { get { return borderStyle; } set { if (!ClientUtils.IsEnumValid_NotSequential(value, (int)value, (int)Border3DStyle.Adjust, (int)Border3DStyle.Bump, (int)Border3DStyle.Etched, (int)Border3DStyle.Flat, (int)Border3DStyle.Raised, (int)Border3DStyle.RaisedInner, (int)Border3DStyle.RaisedOuter, (int)Border3DStyle.Sunken, (int)Border3DStyle.SunkenInner, (int)Border3DStyle.SunkenOuter )) { throw new InvalidEnumArgumentException("value", (int)value, typeof(Border3DStyle)); } if (borderStyle != value) { borderStyle = value; Invalidate(); } } } /// [ DefaultValue(ToolStripStatusLabelBorderSides.None), SRDescription(SR.ToolStripStatusLabelBorderSidesDescr), SRCategory(SR.CatAppearance) ] public ToolStripStatusLabelBorderSides BorderSides { get { return borderSides; } set { // no Enum.IsDefined as this is a flags enum. if (borderSides != value) { borderSides = value; LayoutTransaction.DoLayout(Owner,this, PropertyNames.BorderStyle); Invalidate(); } } } protected internal override Padding DefaultMargin { get { return new Padding(0, 3, 0, 2); } } [ DefaultValue(false), SRDescription(SR.ToolStripStatusLabelSpringDescr), SRCategory(SR.CatAppearance) ] public bool Spring { get { return spring; } set { if (spring != value) { spring = value; if (ParentInternal != null) { LayoutTransaction.DoLayout(ParentInternal, this, PropertyNames.Spring); } } } } public override System.Drawing.Size GetPreferredSize(System.Drawing.Size constrainingSize) { if (BorderSides != ToolStripStatusLabelBorderSides.None) { return base.GetPreferredSize(constrainingSize) + new Size(4, 4); } else { return base.GetPreferredSize(constrainingSize); } } /// /// /// Inheriting classes should override this method to handle this event. /// protected override void OnPaint(System.Windows.Forms.PaintEventArgs e) { if (this.Owner != null) { ToolStripRenderer renderer = this.Renderer; renderer.DrawToolStripStatusLabelBackground(new ToolStripItemRenderEventArgs(e.Graphics, this)); if ((DisplayStyle & ToolStripItemDisplayStyle.Image) == ToolStripItemDisplayStyle.Image) { renderer.DrawItemImage(new ToolStripItemImageRenderEventArgs(e.Graphics, this, InternalLayout.ImageRectangle)); } PaintText(e.Graphics); } } ////// This class performs internal layout for the "split button button" portion of a split button. /// Its main job is to make sure the inner button has the same parent as the split button, so /// that layout can be performed using the correct graphics context. /// private class ToolStripStatusLabelLayout : ToolStripItemInternalLayout { ToolStripStatusLabel owner; public ToolStripStatusLabelLayout(ToolStripStatusLabel owner) : base(owner) { this.owner = owner; } protected override ToolStripItemLayoutOptions CommonLayoutOptions() { ToolStripItemLayoutOptions layoutOptions = base.CommonLayoutOptions(); layoutOptions.borderSize = 0; return layoutOptions; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
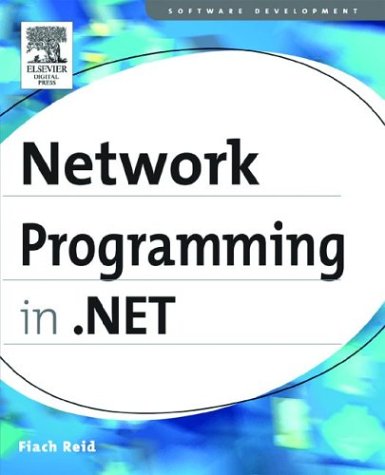
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MediaContext.cs
- DictionaryBase.cs
- PrincipalPermission.cs
- PageThemeParser.cs
- DefaultPrintController.cs
- CodeDirectiveCollection.cs
- SystemEvents.cs
- TextTreeRootNode.cs
- ArrayList.cs
- ListParaClient.cs
- ListChangedEventArgs.cs
- UserValidatedEventArgs.cs
- ReachDocumentReferenceCollectionSerializer.cs
- MeshGeometry3D.cs
- ControlCommandSet.cs
- NonParentingControl.cs
- DataGridRowClipboardEventArgs.cs
- TrustLevel.cs
- XmlQueryCardinality.cs
- ClipboardProcessor.cs
- HitTestFilterBehavior.cs
- ADMembershipProvider.cs
- XmlDocumentSerializer.cs
- DrawingContextWalker.cs
- ParserHooks.cs
- SerializationSectionGroup.cs
- TrackingProfileManager.cs
- Error.cs
- FileLogRecord.cs
- PersistenceIOParticipant.cs
- WebControlsSection.cs
- CellNormalizer.cs
- RemoteWebConfigurationHost.cs
- MdImport.cs
- SecurityKeyType.cs
- TextTrailingWordEllipsis.cs
- MenuItemCollectionEditor.cs
- ConvertEvent.cs
- XPathSelfQuery.cs
- XmlSchemaValidator.cs
- TableLayoutStyleCollection.cs
- WindowsBrush.cs
- BitConverter.cs
- EndpointAddress.cs
- SupportingTokenChannel.cs
- ProfilePropertyMetadata.cs
- WebPartZoneBase.cs
- QilStrConcatenator.cs
- MetabaseReader.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- XmlWellformedWriter.cs
- UInt16Converter.cs
- XmlSchemaValidationException.cs
- HybridObjectCache.cs
- ItemMap.cs
- HttpProfileBase.cs
- XmlnsCompatibleWithAttribute.cs
- BamlRecords.cs
- Size.cs
- PerspectiveCamera.cs
- BinaryKeyIdentifierClause.cs
- DataGridViewCellStyleConverter.cs
- ObjectView.cs
- SupportingTokenParameters.cs
- WeakHashtable.cs
- CacheEntry.cs
- CodeMemberMethod.cs
- CodeSubDirectory.cs
- AspNetSynchronizationContext.cs
- Hashtable.cs
- SystemFonts.cs
- UnauthorizedAccessException.cs
- ArgumentOutOfRangeException.cs
- WebPartActionVerb.cs
- Error.cs
- IProvider.cs
- KeyInfo.cs
- Closure.cs
- XmlArrayAttribute.cs
- XmlSchemaSimpleType.cs
- SqlResolver.cs
- ZipArchive.cs
- HebrewNumber.cs
- BuildDependencySet.cs
- WebServiceResponse.cs
- CodeLabeledStatement.cs
- FixedPage.cs
- ContainsRowNumberChecker.cs
- UriTemplateDispatchFormatter.cs
- Timer.cs
- peernodeimplementation.cs
- OdbcReferenceCollection.cs
- WorkflowStateRollbackService.cs
- CodeMethodReturnStatement.cs
- IdentityHolder.cs
- JulianCalendar.cs
- HeaderElement.cs
- InplaceBitmapMetadataWriter.cs
- UserControl.cs
- _LoggingObject.cs