Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / UIAutomation / Win32Providers / MS / Internal / AutomationProxies / WindowsGrip.cs / 1 / WindowsGrip.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Windows Button Proxy // // History: // 07/01/2003 : preid Created //--------------------------------------------------------------------------- using System; using System.Collections; using System.Text; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.ComponentModel; using MS.Win32; namespace MS.Internal.AutomationProxies { class WindowsGrip: ProxyFragment { // ----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors public WindowsGrip (IntPtr hwnd, ProxyHwnd parent, int item) : base( hwnd, parent, item) { _sType = ST.Get(STID.LocalizedControlTypeGrip); _sAutomationId = "Window.Grip"; // This string is a non-localizable string } #endregion //------------------------------------------------------ // // Patterns Implementation // //----------------------------------------------------- #region ProxySimple Interface ////// Gets the bounding rectangle for this element /// internal override Rect BoundingRectangle { get { if (IsGripPresent(_hwnd, false)) { NativeMethods.Win32Rect client = new NativeMethods.Win32Rect(); if (Misc.GetClientRectInScreenCoordinates(_hwnd, ref client)) { NativeMethods.SIZE sizeGrip = GetGripSize(_hwnd, false); if (Misc.IsLayoutRTL(_hwnd)) { return new Rect(client.left - sizeGrip.cx, client.bottom, sizeGrip.cx, sizeGrip.cy); } else { return new Rect(client.right, client.bottom, sizeGrip.cx, sizeGrip.cy); } } } return Rect.Empty; } } #endregion //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods static internal bool IsGripPresent(IntPtr hwnd, bool onStatusBar) { NativeMethods.Win32Rect client = new NativeMethods.Win32Rect(); if (!Misc.GetClientRectInScreenCoordinates(hwnd, ref client)) { return false; } // According to the documentation of GetClientRect, the left and top members are zero. So if // they are negitive the control must be minimized, therefore the grip is not present. if (client.left < 0 && client.top < 0 ) { return false; } NativeMethods.SIZE sizeGrip = GetGripSize(hwnd, onStatusBar); if (!onStatusBar) { // When not on a status bar the grip should be out side of the client area. sizeGrip.cx *= -1; sizeGrip.cy *= -1; } if (Misc.IsLayoutRTL(hwnd)) { int x = client.left + (int)(sizeGrip.cx / 2); int y = client.bottom - (int)(sizeGrip.cy / 2); int hit = Misc.ProxySendMessageInt(hwnd, NativeMethods.WM_NCHITTEST, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); return hit == NativeMethods.HTBOTTOMLEFT; } else { int x = client.right - (int)(sizeGrip.cx / 2); int y = client.bottom - (int)(sizeGrip.cy / 2); int hit = Misc.ProxySendMessageInt(hwnd, NativeMethods.WM_NCHITTEST, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); return hit == NativeMethods.HTBOTTOMRIGHT; } } internal static NativeMethods.SIZE GetGripSize(IntPtr hwnd, bool onStatusBar) { using (ThemePart themePart = new ThemePart(hwnd, onStatusBar ? "STATUS" : "SCROLLBAR")) { return themePart.Size(onStatusBar ? (int)ThemePart.STATUSPARTS.SP_GRIPPER : (int)ThemePart.SCROLLBARPARTS.SBP_SIZEBOX, 0); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Windows Button Proxy // // History: // 07/01/2003 : preid Created //--------------------------------------------------------------------------- using System; using System.Collections; using System.Text; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.ComponentModel; using MS.Win32; namespace MS.Internal.AutomationProxies { class WindowsGrip: ProxyFragment { // ----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors public WindowsGrip (IntPtr hwnd, ProxyHwnd parent, int item) : base( hwnd, parent, item) { _sType = ST.Get(STID.LocalizedControlTypeGrip); _sAutomationId = "Window.Grip"; // This string is a non-localizable string } #endregion //------------------------------------------------------ // // Patterns Implementation // //----------------------------------------------------- #region ProxySimple Interface ////// Gets the bounding rectangle for this element /// internal override Rect BoundingRectangle { get { if (IsGripPresent(_hwnd, false)) { NativeMethods.Win32Rect client = new NativeMethods.Win32Rect(); if (Misc.GetClientRectInScreenCoordinates(_hwnd, ref client)) { NativeMethods.SIZE sizeGrip = GetGripSize(_hwnd, false); if (Misc.IsLayoutRTL(_hwnd)) { return new Rect(client.left - sizeGrip.cx, client.bottom, sizeGrip.cx, sizeGrip.cy); } else { return new Rect(client.right, client.bottom, sizeGrip.cx, sizeGrip.cy); } } } return Rect.Empty; } } #endregion //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods static internal bool IsGripPresent(IntPtr hwnd, bool onStatusBar) { NativeMethods.Win32Rect client = new NativeMethods.Win32Rect(); if (!Misc.GetClientRectInScreenCoordinates(hwnd, ref client)) { return false; } // According to the documentation of GetClientRect, the left and top members are zero. So if // they are negitive the control must be minimized, therefore the grip is not present. if (client.left < 0 && client.top < 0 ) { return false; } NativeMethods.SIZE sizeGrip = GetGripSize(hwnd, onStatusBar); if (!onStatusBar) { // When not on a status bar the grip should be out side of the client area. sizeGrip.cx *= -1; sizeGrip.cy *= -1; } if (Misc.IsLayoutRTL(hwnd)) { int x = client.left + (int)(sizeGrip.cx / 2); int y = client.bottom - (int)(sizeGrip.cy / 2); int hit = Misc.ProxySendMessageInt(hwnd, NativeMethods.WM_NCHITTEST, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); return hit == NativeMethods.HTBOTTOMLEFT; } else { int x = client.right - (int)(sizeGrip.cx / 2); int y = client.bottom - (int)(sizeGrip.cy / 2); int hit = Misc.ProxySendMessageInt(hwnd, NativeMethods.WM_NCHITTEST, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); return hit == NativeMethods.HTBOTTOMRIGHT; } } internal static NativeMethods.SIZE GetGripSize(IntPtr hwnd, bool onStatusBar) { using (ThemePart themePart = new ThemePart(hwnd, onStatusBar ? "STATUS" : "SCROLLBAR")) { return themePart.Size(onStatusBar ? (int)ThemePart.STATUSPARTS.SP_GRIPPER : (int)ThemePart.SCROLLBARPARTS.SBP_SIZEBOX, 0); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
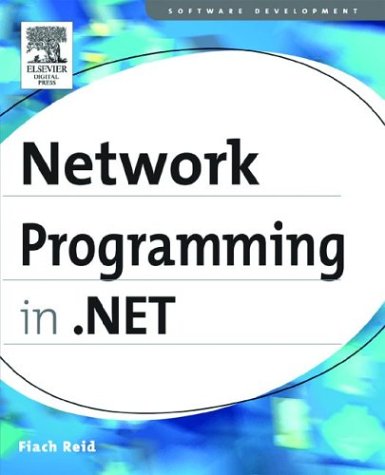
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Model3DGroup.cs
- PartialCachingControl.cs
- MetadataArtifactLoaderResource.cs
- DataGridViewColumnConverter.cs
- SqlInfoMessageEvent.cs
- EntityCollectionChangedParams.cs
- Emitter.cs
- RuntimeWrappedException.cs
- WmlObjectListAdapter.cs
- AppDomainFactory.cs
- XmlTextReader.cs
- _IPv4Address.cs
- AuthStoreRoleProvider.cs
- ReachDocumentPageSerializer.cs
- XmlILConstructAnalyzer.cs
- RsaSecurityKey.cs
- _TimerThread.cs
- Int32CAMarshaler.cs
- Axis.cs
- MethodImplAttribute.cs
- PersistChildrenAttribute.cs
- SignerInfo.cs
- ClientProxyGenerator.cs
- SafeNativeMethods.cs
- OracleMonthSpan.cs
- MimeTypeMapper.cs
- BinaryCommonClasses.cs
- HttpListenerContext.cs
- smtpconnection.cs
- Input.cs
- TableFieldsEditor.cs
- XmlSchemaObjectCollection.cs
- XmlCodeExporter.cs
- ToolStripItem.cs
- Pick.cs
- WindowsScroll.cs
- BindableTemplateBuilder.cs
- InvalidPrinterException.cs
- WindowsBrush.cs
- PtsPage.cs
- GridSplitter.cs
- CodeSubDirectory.cs
- VScrollBar.cs
- SoundPlayer.cs
- OdbcErrorCollection.cs
- XMLUtil.cs
- SliderAutomationPeer.cs
- FilterElement.cs
- XhtmlBasicValidatorAdapter.cs
- AvTraceFormat.cs
- _UriSyntax.cs
- UDPClient.cs
- CriticalHandle.cs
- IpcChannel.cs
- DefaultObjectSerializer.cs
- _SslState.cs
- SqlTrackingWorkflowInstance.cs
- RegistrySecurity.cs
- OdbcTransaction.cs
- DataServiceQueryException.cs
- RightsManagementEncryptionTransform.cs
- ITextView.cs
- ToolStripPanelRow.cs
- ImageDrawing.cs
- SrgsSemanticInterpretationTag.cs
- Geometry3D.cs
- StringAnimationUsingKeyFrames.cs
- MyContact.cs
- DesignerObjectListAdapter.cs
- ScriptReferenceEventArgs.cs
- DataReceivedEventArgs.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- RelationshipConverter.cs
- XmlSchemaAnnotated.cs
- LocalFileSettingsProvider.cs
- RequestStatusBarUpdateEventArgs.cs
- DefaultBinder.cs
- Point3DCollectionConverter.cs
- SiteMapProvider.cs
- XPathAncestorQuery.cs
- FontStyle.cs
- XamlSerializationHelper.cs
- SettingsAttributeDictionary.cs
- CodeExpressionCollection.cs
- PerformanceCounter.cs
- login.cs
- localization.cs
- List.cs
- ValidatingPropertiesEventArgs.cs
- TextDocumentView.cs
- PointF.cs
- ReadOnlyDictionary.cs
- CleanUpVirtualizedItemEventArgs.cs
- TreeWalker.cs
- WindowsButton.cs
- PersonalizationAdministration.cs
- StorageModelBuildProvider.cs
- VariableQuery.cs
- EventLogEntryCollection.cs
- TypeConverterAttribute.cs