Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / ComponentModel / DefaultValueAttribute.cs / 1305376 / DefaultValueAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; #if !SILVERLIGHT using System.Runtime.Serialization.Formatters; #endif using System.Security.Permissions; ////// #if !SILVERLIGHT [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1019:DefineAccessorsForAttributeArguments")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1813:AvoidUnsealedAttributes")] #endif [AttributeUsage(AttributeTargets.All)] public class DefaultValueAttribute : Attribute { ///Specifies the default value for a property. ////// This is the default value. /// private object value; ////// public DefaultValueAttribute(Type type, string value) { // The try/catch here is because attributes should never throw exceptions. We would fail to // load an otherwise normal class. try { #if !SILVERLIGHT this.value = TypeDescriptor.GetConverter(type).ConvertFromInvariantString(value); #else if (type.IsEnum) { this.value = Enum.Parse(type, value, true); } else if (type == typeof(TimeSpan)) { this.value = TimeSpan.Parse(value); } else if (type.Module == typeof(string).Module) { this.value = Convert.ChangeType(value, type, CultureInfo.InvariantCulture); } #endif } catch { #if !SILVERLIGHT Debug.Fail("Default value attribute of type " + type.FullName + " threw converting from the string '" + value + "'."); #else Debug.WriteLine("Default value attribute of type " + type.FullName + " threw converting from the string '" + value + "'."); #endif } } ///Initializes a new instance of the ///class, converting the /// specified value to the /// specified type, and using the U.S. English culture as the /// translation /// context. /// public DefaultValueAttribute(char value) { this.value = value; } ///Initializes a new instance of the ///class using a Unicode /// character. /// public DefaultValueAttribute(byte value) { this.value = value; } ///Initializes a new instance of the ///class using an 8-bit unsigned /// integer. /// public DefaultValueAttribute(short value) { this.value = value; } ///Initializes a new instance of the ///class using a 16-bit signed /// integer. /// public DefaultValueAttribute(int value) { this.value = value; } ///Initializes a new instance of the ///class using a 32-bit signed /// integer. /// public DefaultValueAttribute(long value) { this.value = value; } ///Initializes a new instance of the ///class using a 64-bit signed /// integer. /// public DefaultValueAttribute(float value) { this.value = value; } ///Initializes a new instance of the ///class using a /// single-precision floating point /// number. /// public DefaultValueAttribute(double value) { this.value = value; } ///Initializes a new instance of the ///class using a /// double-precision floating point /// number. /// public DefaultValueAttribute(bool value) { this.value = value; } ///Initializes a new instance of the ///class using a /// value. /// public DefaultValueAttribute(string value) { this.value = value; } ///Initializes a new instance of the ///class using a . /// public DefaultValueAttribute(object value) { this.value = value; } ///Initializes a new instance of the ////// class. /// public virtual object Value { get { return value; } } public override bool Equals(object obj) { if (obj == this) { return true; } DefaultValueAttribute other = obj as DefaultValueAttribute; if (other != null) { if (Value != null) { return Value.Equals(other.Value); } else { return (other.Value == null); } } return false; } public override int GetHashCode() { return base.GetHashCode(); } protected void SetValue(object value) { this.value = value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Gets the default value of the property this /// attribute is /// bound to. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; #if !SILVERLIGHT using System.Runtime.Serialization.Formatters; #endif using System.Security.Permissions; ////// #if !SILVERLIGHT [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1019:DefineAccessorsForAttributeArguments")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1813:AvoidUnsealedAttributes")] #endif [AttributeUsage(AttributeTargets.All)] public class DefaultValueAttribute : Attribute { ///Specifies the default value for a property. ////// This is the default value. /// private object value; ////// public DefaultValueAttribute(Type type, string value) { // The try/catch here is because attributes should never throw exceptions. We would fail to // load an otherwise normal class. try { #if !SILVERLIGHT this.value = TypeDescriptor.GetConverter(type).ConvertFromInvariantString(value); #else if (type.IsEnum) { this.value = Enum.Parse(type, value, true); } else if (type == typeof(TimeSpan)) { this.value = TimeSpan.Parse(value); } else if (type.Module == typeof(string).Module) { this.value = Convert.ChangeType(value, type, CultureInfo.InvariantCulture); } #endif } catch { #if !SILVERLIGHT Debug.Fail("Default value attribute of type " + type.FullName + " threw converting from the string '" + value + "'."); #else Debug.WriteLine("Default value attribute of type " + type.FullName + " threw converting from the string '" + value + "'."); #endif } } ///Initializes a new instance of the ///class, converting the /// specified value to the /// specified type, and using the U.S. English culture as the /// translation /// context. /// public DefaultValueAttribute(char value) { this.value = value; } ///Initializes a new instance of the ///class using a Unicode /// character. /// public DefaultValueAttribute(byte value) { this.value = value; } ///Initializes a new instance of the ///class using an 8-bit unsigned /// integer. /// public DefaultValueAttribute(short value) { this.value = value; } ///Initializes a new instance of the ///class using a 16-bit signed /// integer. /// public DefaultValueAttribute(int value) { this.value = value; } ///Initializes a new instance of the ///class using a 32-bit signed /// integer. /// public DefaultValueAttribute(long value) { this.value = value; } ///Initializes a new instance of the ///class using a 64-bit signed /// integer. /// public DefaultValueAttribute(float value) { this.value = value; } ///Initializes a new instance of the ///class using a /// single-precision floating point /// number. /// public DefaultValueAttribute(double value) { this.value = value; } ///Initializes a new instance of the ///class using a /// double-precision floating point /// number. /// public DefaultValueAttribute(bool value) { this.value = value; } ///Initializes a new instance of the ///class using a /// value. /// public DefaultValueAttribute(string value) { this.value = value; } ///Initializes a new instance of the ///class using a . /// public DefaultValueAttribute(object value) { this.value = value; } ///Initializes a new instance of the ////// class. /// public virtual object Value { get { return value; } } public override bool Equals(object obj) { if (obj == this) { return true; } DefaultValueAttribute other = obj as DefaultValueAttribute; if (other != null) { if (Value != null) { return Value.Equals(other.Value); } else { return (other.Value == null); } } return false; } public override int GetHashCode() { return base.GetHashCode(); } protected void SetValue(object value) { this.value = value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Gets the default value of the property this /// attribute is /// bound to. /// ///
Link Menu
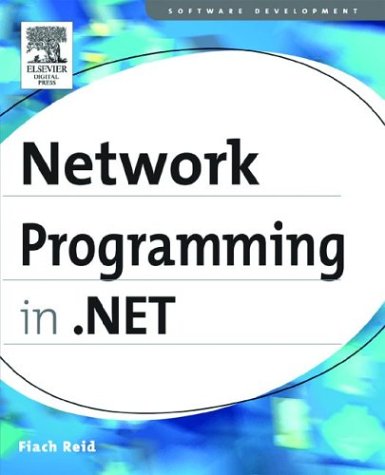
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegionData.cs
- MergeExecutor.cs
- CatalogZoneBase.cs
- IndexingContentUnit.cs
- MethodRental.cs
- LoopExpression.cs
- KnownTypesProvider.cs
- StateInitializationDesigner.cs
- ScriptingAuthenticationServiceSection.cs
- MergablePropertyAttribute.cs
- Symbol.cs
- AppSettingsExpressionBuilder.cs
- DataGridViewCellCancelEventArgs.cs
- ReflectionServiceProvider.cs
- Html32TextWriter.cs
- DefaultTextStoreTextComposition.cs
- WindowsFormsHostAutomationPeer.cs
- DocumentStream.cs
- EventLogEntryCollection.cs
- XmlTypeMapping.cs
- ObjectAnimationUsingKeyFrames.cs
- PaintValueEventArgs.cs
- TemplateColumn.cs
- LinkUtilities.cs
- EntityClassGenerator.cs
- ObjectMemberMapping.cs
- PropertyCondition.cs
- StatusBarPanel.cs
- UMPAttributes.cs
- MaskedTextProvider.cs
- PropertyBuilder.cs
- StylusPlugin.cs
- PointLightBase.cs
- BooleanConverter.cs
- RegexParser.cs
- ToolboxSnapDragDropEventArgs.cs
- BrowserTree.cs
- TemplateControlBuildProvider.cs
- ConditionChanges.cs
- AuthenticationSection.cs
- DataObjectSettingDataEventArgs.cs
- CorrelationManager.cs
- Stroke2.cs
- Int32EqualityComparer.cs
- PagePropertiesChangingEventArgs.cs
- EntityClassGenerator.cs
- PhysicalAddress.cs
- Fonts.cs
- xmlfixedPageInfo.cs
- Literal.cs
- CodeTypeMember.cs
- IndicFontClient.cs
- Gdiplus.cs
- TableStyle.cs
- TableCellCollection.cs
- Primitive.cs
- TrackingServices.cs
- ResourceReader.cs
- SQLMembershipProvider.cs
- EntityStoreSchemaFilterEntry.cs
- SqlConnectionHelper.cs
- FigureHelper.cs
- ColorComboBox.cs
- TabItemAutomationPeer.cs
- Lease.cs
- Style.cs
- CallbackHandler.cs
- NetworkInterface.cs
- ProcessModuleCollection.cs
- OdbcPermission.cs
- Profiler.cs
- DoubleLink.cs
- TypeUsageBuilder.cs
- EndEvent.cs
- XPathSingletonIterator.cs
- UserControlBuildProvider.cs
- InfoCardCryptoHelper.cs
- Maps.cs
- XmlQueryTypeFactory.cs
- SQLDecimal.cs
- FloaterParagraph.cs
- DecoderFallback.cs
- PointLight.cs
- XPathParser.cs
- EdmItemError.cs
- SafeLibraryHandle.cs
- XmlBinaryReaderSession.cs
- ReadWriteSpinLock.cs
- DataGridViewControlCollection.cs
- SecurityKeyUsage.cs
- ListViewAutomationPeer.cs
- Tag.cs
- ImageButton.cs
- _ListenerResponseStream.cs
- ValueChangedEventManager.cs
- UserControlCodeDomTreeGenerator.cs
- tooltip.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- InitializationEventAttribute.cs
- SR.Designer.cs