Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / System / Windows / AttachedPropertyBrowsableForTypeAttribute.cs / 1 / AttachedPropertyBrowsableForTypeAttribute.cs
namespace System.Windows { using System; ////// This class declares that an attached property is browsable only /// for dependency objects that derive from the given type. If more /// than one type is specified, the property is browsable if any type /// matches (logical or). The type may also be an interface. /// [AttributeUsage(AttributeTargets.Method, AllowMultiple = true)] public sealed class AttachedPropertyBrowsableForTypeAttribute : AttachedPropertyBrowsableAttribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// Creates a new AttachedPropertyBrowsableForTypeAttribute. Provide the type /// you want the attached property to be browsable for. Multiple /// attributes may be used to provide support for more than one /// type. /// public AttachedPropertyBrowsableForTypeAttribute(Type targetType) { if (targetType == null) throw new ArgumentNullException("targetType"); _targetType = targetType; } //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// Returns the type passed into the constructor. /// public Type TargetType { get { return _targetType; } } ////// For AllowMultiple attributes, TypeId must be unique for /// each unique instance. The default returns the type, which /// is only correct for AllowMultiple == false. /// public override object TypeId { get { return this; } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// Overrides Object.Equals to implement correct equality semantics for this /// attribute. /// public override bool Equals(object obj) { AttachedPropertyBrowsableForTypeAttribute other = obj as AttachedPropertyBrowsableForTypeAttribute; if (other == null) return false; return _targetType == other._targetType; } ////// Overrides Object.GetHashCode to implement correct hashing semantics. /// public override int GetHashCode() { return _targetType.GetHashCode(); } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ ////// Returns true if the dependency object passed to the method is a type, /// subtype or implememts the interface of any of the the types contained /// in this object. /// internal override bool IsBrowsable(DependencyObject d, DependencyProperty dp) { if (d == null) throw new ArgumentNullException("d"); if (dp == null) throw new ArgumentNullException("dp"); // Get the dependency object type for our target type. // We cannot assume the user didn't do something wrong and // feed us a type that is not a dependency object, but that is // rare enough that it is worth the try/catch here rather than // a double IsAssignableFrom (one here, and one in DependencyObjectType). // We still use a flag here rather than checking for a null // _dTargetType so that a bad property that throws won't consistently // slow the system down with ArgumentExceptions. if (!_dTargetTypeChecked) { try { _dTargetType = DependencyObjectType.FromSystemType(_targetType); } catch(ArgumentException) { } _dTargetTypeChecked = true; } if (_dTargetType != null && _dTargetType.IsInstanceOfType(d)) { return true; } return false; } ////// Returns true if a browsable match is true if any one of multiple /// instances of the same type return true for IsBrowsable. We override /// this to return true because any one of a successfull match for /// IsBrowsable is accepted. /// internal override bool UnionResults { get { return true; } } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private Type _targetType; private DependencyObjectType _dTargetType; private bool _dTargetTypeChecked; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Windows { using System; ////// This class declares that an attached property is browsable only /// for dependency objects that derive from the given type. If more /// than one type is specified, the property is browsable if any type /// matches (logical or). The type may also be an interface. /// [AttributeUsage(AttributeTargets.Method, AllowMultiple = true)] public sealed class AttachedPropertyBrowsableForTypeAttribute : AttachedPropertyBrowsableAttribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// Creates a new AttachedPropertyBrowsableForTypeAttribute. Provide the type /// you want the attached property to be browsable for. Multiple /// attributes may be used to provide support for more than one /// type. /// public AttachedPropertyBrowsableForTypeAttribute(Type targetType) { if (targetType == null) throw new ArgumentNullException("targetType"); _targetType = targetType; } //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// Returns the type passed into the constructor. /// public Type TargetType { get { return _targetType; } } ////// For AllowMultiple attributes, TypeId must be unique for /// each unique instance. The default returns the type, which /// is only correct for AllowMultiple == false. /// public override object TypeId { get { return this; } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// Overrides Object.Equals to implement correct equality semantics for this /// attribute. /// public override bool Equals(object obj) { AttachedPropertyBrowsableForTypeAttribute other = obj as AttachedPropertyBrowsableForTypeAttribute; if (other == null) return false; return _targetType == other._targetType; } ////// Overrides Object.GetHashCode to implement correct hashing semantics. /// public override int GetHashCode() { return _targetType.GetHashCode(); } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ ////// Returns true if the dependency object passed to the method is a type, /// subtype or implememts the interface of any of the the types contained /// in this object. /// internal override bool IsBrowsable(DependencyObject d, DependencyProperty dp) { if (d == null) throw new ArgumentNullException("d"); if (dp == null) throw new ArgumentNullException("dp"); // Get the dependency object type for our target type. // We cannot assume the user didn't do something wrong and // feed us a type that is not a dependency object, but that is // rare enough that it is worth the try/catch here rather than // a double IsAssignableFrom (one here, and one in DependencyObjectType). // We still use a flag here rather than checking for a null // _dTargetType so that a bad property that throws won't consistently // slow the system down with ArgumentExceptions. if (!_dTargetTypeChecked) { try { _dTargetType = DependencyObjectType.FromSystemType(_targetType); } catch(ArgumentException) { } _dTargetTypeChecked = true; } if (_dTargetType != null && _dTargetType.IsInstanceOfType(d)) { return true; } return false; } ////// Returns true if a browsable match is true if any one of multiple /// instances of the same type return true for IsBrowsable. We override /// this to return true because any one of a successfull match for /// IsBrowsable is accepted. /// internal override bool UnionResults { get { return true; } } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private Type _targetType; private DependencyObjectType _dTargetType; private bool _dTargetTypeChecked; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
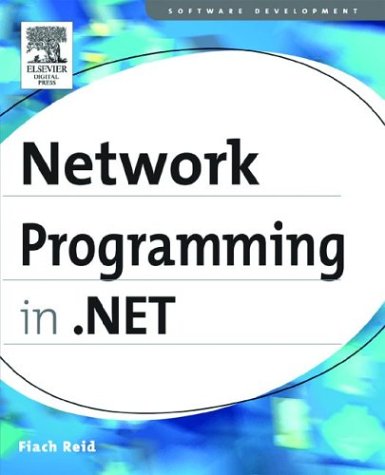
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridViewRowPresenter.cs
- UserMapPath.cs
- CommandEventArgs.cs
- SqlDependencyUtils.cs
- DelegatingTypeDescriptionProvider.cs
- ActivityPreviewDesigner.cs
- ApplicationCommands.cs
- DoubleAnimationClockResource.cs
- UpdatePanelTrigger.cs
- HtmlString.cs
- SecureConversationVersion.cs
- TcpHostedTransportConfiguration.cs
- ToolboxItemFilterAttribute.cs
- DescendantBaseQuery.cs
- EventProviderWriter.cs
- FormViewUpdateEventArgs.cs
- DataGridViewSortCompareEventArgs.cs
- RPIdentityRequirement.cs
- ConvertTextFrag.cs
- Utils.cs
- FormViewDeleteEventArgs.cs
- ISAPIRuntime.cs
- XmlCodeExporter.cs
- ItemsChangedEventArgs.cs
- WorkflowRuntimeServiceElement.cs
- ThreadExceptionEvent.cs
- ThreadAttributes.cs
- LinearKeyFrames.cs
- EventWaitHandle.cs
- BamlResourceSerializer.cs
- WindowsScrollBar.cs
- AppDomainUnloadedException.cs
- DocumentApplicationJournalEntry.cs
- TimeoutValidationAttribute.cs
- MemoryMappedViewAccessor.cs
- HttpDebugHandler.cs
- HostingEnvironment.cs
- FileUtil.cs
- TypeElementCollection.cs
- HeaderedItemsControl.cs
- EventDescriptorCollection.cs
- SystemKeyConverter.cs
- XmlSchemaComplexContent.cs
- StdValidatorsAndConverters.cs
- formatter.cs
- WebBrowserHelper.cs
- ButtonColumn.cs
- ErrorWebPart.cs
- LogicalExpressionEditor.cs
- Regex.cs
- SafeNativeMethods.cs
- EventMappingSettingsCollection.cs
- XPathNodeInfoAtom.cs
- ContentPlaceHolder.cs
- OleDbConnectionInternal.cs
- SelectionItemPatternIdentifiers.cs
- WinFormsUtils.cs
- ViewRendering.cs
- BitmapVisualManager.cs
- FixedSOMTableRow.cs
- StrokeNodeEnumerator.cs
- MetadataItemEmitter.cs
- OracleString.cs
- BlurEffect.cs
- ListViewContainer.cs
- SystemInformation.cs
- MouseActionConverter.cs
- RoutedEvent.cs
- ViewPort3D.cs
- SQLDecimal.cs
- FragmentQuery.cs
- XmlChildNodes.cs
- DesignerForm.cs
- XNodeValidator.cs
- KeySpline.cs
- FixedTextContainer.cs
- AspNetCompatibilityRequirementsAttribute.cs
- Helpers.cs
- FastEncoderWindow.cs
- FastEncoderWindow.cs
- SHA512Managed.cs
- PrintControllerWithStatusDialog.cs
- XsltInput.cs
- CodeConstructor.cs
- CompilerResults.cs
- SolidBrush.cs
- StaticSiteMapProvider.cs
- TagMapCollection.cs
- MethodCallConverter.cs
- EventlogProvider.cs
- ProjectionNode.cs
- UrlPath.cs
- ControlAdapter.cs
- BindingOperations.cs
- EndpointAddressProcessor.cs
- AssemblyGen.cs
- AccessibleObject.cs
- AvtEvent.cs
- AttachedPropertyInfo.cs
- WebAdminConfigurationHelper.cs