Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / RPIdentityRequirement.cs / 1 / RPIdentityRequirement.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.IO; using System.Xml; using Microsoft.InfoCards.Diagnostics; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary // This class contains the RP identity requirement of the card // // Remarks // The parentId index is populated with the URI of the InfoCard associated with this set of PII. // internal class RPIdentityRequirement { const Int32 InvalidRow = 0; Uri m_infoCardId; Int32 m_rowId = InvalidRow; bool m_RPIdentityRequirement = false; // // Summary // Creates an uninitialized instance of the RPIdentityRequirement. // // Remarks // This behavior is not supported and is thus declared as private. // private RPIdentityRequirement() { } // // Summary // Creates a new instance of the RPIdentityRequirement associated with the // InfoCard whose Id is specified. // // Parameters // infoCardId - Id of the owning InfoCard // public RPIdentityRequirement( Uri infoCardId, bool RPIdentityRequirement ) : this() { if( null == infoCardId ) { throw IDT.ThrowHelperArgumentNull( "infoCardId" ); } m_infoCardId = infoCardId; m_RPIdentityRequirement = RPIdentityRequirement; } // // Summary // Retrieves the RPIdentityRequirement from the store. // The InfoCard Id specified in the constructor is used as the parent key. // // Parameters // con - Connection to the store to be used to retrieve the object. // public void Get( StoreConnection con ) { if( null == con ) { throw IDT.ThrowHelperArgumentNull( "con" ); } IDT.TraceDebug( "Retrieving the claims for infocard({0})", m_infoCardId ); // // Find the row for the object from the database, returns null if not exists // DataRow row = TryGetRow( con, QueryDetails.FullRow ); // // A RPIdentityRequirement object does not necessarily exist for this infocard // if( null != row ) { // // Populate the claims from the byte array // Deserialize( new MemoryStream( row.GetDataField() ) ); // // Update the row id from the database // m_rowId = row.LocalId; } } // // Summary // Writes all of the RPIdentityRequirement into a binary stream in an unordered sequence. // // Parameters // stream - Stream that will receive the byte stream of each claim. // public void Serialize( Stream stream ) { // // Setup a BinaryWriter to serialize the bytes of each member to the provided stream // BinaryWriter writer = new BinaryWriter( stream, System.Text.Encoding.Unicode ); writer.Write( m_RPIdentityRequirement ); } // // Summary // Reads a RPIdentityRequirement. // // Parameters // stream - Stream that will provide the byte stream of claims. // public void Deserialize( Stream stream ) { BinaryReader reader = new InfoCardBinaryReader( stream, System.Text.Encoding.Unicode ); m_RPIdentityRequirement = reader.ReadBoolean(); } // // Summary // Writes a serialized RPIdentityRequirement object to the store. // // Remarks // The parentId index field is set to the Id of the associated InfoCard. // // Parameters // con - Connection to the store to be used to store the RPIdentityRequirement. // public void Save( StoreConnection con ) { if( null == con ) { throw IDT.ThrowHelperArgumentNull( "con" ); } // // Write the RPIdentityRequirement to the store associated // this collection with the infocard id specified. // IDT.TraceDebug( "Saving the RPIdentityRequirement..." ); // // Try and get the database header information to // see if this is an insert or update. // // Note: The datafield is not part of the projection // in order to avoid unnecessary decryption. // DataRow row = TryGetRow( con, QueryDetails.FullHeader ); if( null == row ) { row = new DataRow(); row.ObjectType = (Int32)StorableObjectType.RPIdentityRequirement; row.GlobalId = Guid.NewGuid(); } // // Populate the parentId index field // row.SetIndexValue( SecondaryIndexDefinition.ParentIdIndex, GlobalId.DeriveFrom( m_infoCardId.ToString() ) ); // // Populate the data object // MemoryStream ms = new MemoryStream(); Serialize( ms ); row.SetDataField( ms.ToArray() ); // // Save the row to the database // con.Save( row ); // // Update the row id in the object in case // this was an insert. // m_rowId = row.LocalId; } public bool StrongIdentityRequired { get { return m_RPIdentityRequirement; } set { m_RPIdentityRequirement = value; } } // // Summary // Queries the store for the RPIdentityRequirement object associated with the InfoCard Id // currently in m_infoCardId. // // Remarks // This function will return null if no object is found in the store. // // Parameters // con - Connection to the store to be used to find the RPIdentityRequirement object. // details - The projection of the store information to be returned. // protected DataRow TryGetRow( StoreConnection con, QueryDetails details ) { IDT.Assert( null != m_infoCardId, "null infocard id" ); // // Retrieve a single object from the database // DataRow row = con.GetSingleRow( details, new QueryParameter( SecondaryIndexDefinition.ObjectTypeIndex, (Int32)StorableObjectType.RPIdentityRequirement ), new QueryParameter( SecondaryIndexDefinition.ParentIdIndex, GlobalId.DeriveFrom( m_infoCardId.ToString() ) ) ); return row; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
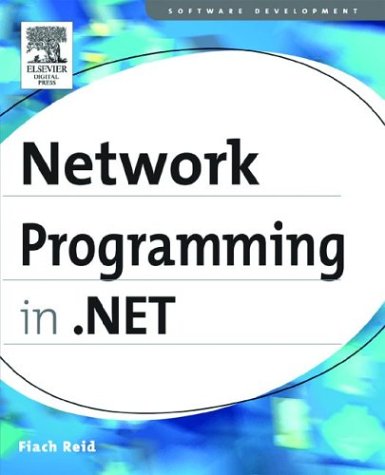
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewRowDividerDoubleClickEventArgs.cs
- DirectionalLight.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- OdbcConnectionHandle.cs
- Classification.cs
- ItemContainerPattern.cs
- DayRenderEvent.cs
- RegexMatch.cs
- WindowAutomationPeer.cs
- TreeViewCancelEvent.cs
- SelectionItemProviderWrapper.cs
- Main.cs
- PrinterResolution.cs
- DocumentPageViewAutomationPeer.cs
- PrintDialog.cs
- RawMouseInputReport.cs
- Camera.cs
- Timer.cs
- ISAPIWorkerRequest.cs
- SqlCharStream.cs
- DataGridViewControlCollection.cs
- ThreadNeutralSemaphore.cs
- Util.cs
- PartialCachingControl.cs
- TableParaClient.cs
- Dispatcher.cs
- RawStylusActions.cs
- TableCellAutomationPeer.cs
- LiteralControl.cs
- Transactions.cs
- JsonObjectDataContract.cs
- BasicViewGenerator.cs
- Splitter.cs
- ReadOnlyObservableCollection.cs
- RenderData.cs
- WindowsGrip.cs
- XmlException.cs
- OdbcException.cs
- DecoderExceptionFallback.cs
- NopReturnReader.cs
- CodeTypeReferenceCollection.cs
- WebPartConnectionsConfigureVerb.cs
- RegexGroupCollection.cs
- XPathNavigatorReader.cs
- XpsS0ValidatingLoader.cs
- HttpCachePolicy.cs
- SystemInformation.cs
- Substitution.cs
- IgnorePropertiesAttribute.cs
- BufferedWebEventProvider.cs
- HttpErrorTraceRecord.cs
- RoleProviderPrincipal.cs
- codemethodreferenceexpression.cs
- CapabilitiesState.cs
- DesignerActionUIStateChangeEventArgs.cs
- FilterException.cs
- NotConverter.cs
- StrongName.cs
- DataListDesigner.cs
- SerialStream.cs
- ToolStripGrip.cs
- LambdaCompiler.Binary.cs
- AssociationTypeEmitter.cs
- SingleTagSectionHandler.cs
- WebPartDisplayMode.cs
- TypeDescriptionProvider.cs
- CustomWebEventKey.cs
- SiteMap.cs
- ConnectionStringSettings.cs
- HttpModuleAction.cs
- SQLInt64.cs
- Error.cs
- WebScriptMetadataInstanceContextProvider.cs
- IdentityManager.cs
- ChangeInterceptorAttribute.cs
- CommandHelper.cs
- httpserverutility.cs
- ByteAnimation.cs
- UnauthorizedWebPart.cs
- RightsManagementPermission.cs
- MenuAdapter.cs
- ToolStripItemClickedEventArgs.cs
- GCHandleCookieTable.cs
- SafeProcessHandle.cs
- ToolStripItemRenderEventArgs.cs
- ResourceReferenceExpression.cs
- mediaeventargs.cs
- ApplicationHost.cs
- SafePEFileHandle.cs
- DebugView.cs
- ObservableCollectionDefaultValueFactory.cs
- XamlPathDataSerializer.cs
- ContainerUIElement3D.cs
- Signature.cs
- PropertyValueUIItem.cs
- SoapAttributeAttribute.cs
- ChannelServices.cs
- PersonalizableTypeEntry.cs
- IndentedWriter.cs
- _Rfc2616CacheValidators.cs