Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Configuration / System / Configuration / elementinformation.cs / 1 / elementinformation.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Configuration; using System.Collections.Specialized; using System.ComponentModel; using System.Collections; using System.Runtime.Serialization; namespace System.Configuration { // ElementInformation // // Expose information on Configuration Elements, and the // properties that they contain // public sealed class ElementInformation { private ConfigurationElement _thisElement; private PropertyInformationCollection _internalProperties; private ConfigurationException[] _errors; internal ElementInformation(ConfigurationElement thisElement) { _thisElement = thisElement; } // Properties // // Retrieve Collection of properties within this element // public PropertyInformationCollection Properties { get { if (_internalProperties == null) { _internalProperties = new PropertyInformationCollection(_thisElement); } return _internalProperties; } } // IsInherited // // Was this element inheritted, or was the property actually // set here // public bool IsPresent { get { return _thisElement.ElementPresent; } } // IsLocked // // Is this property locked? // public bool IsLocked { get { return (((_thisElement.ItemLocked & ConfigurationValueFlags.Locked) != 0) && ((_thisElement.ItemLocked & ConfigurationValueFlags.Inherited) != 0)); } } // IsCollection // // Is this element a collection? // public bool IsCollection { get { ConfigurationElementCollection collection = _thisElement as ConfigurationElementCollection; if (collection == null) { // Try the default collection if (_thisElement.Properties.DefaultCollectionProperty != null) { // this is not a collection but it may contain a default collection collection = _thisElement[_thisElement.Properties.DefaultCollectionProperty] as ConfigurationElementCollection; } } return (collection != null); } } // Internal method to fix SetRawXML defect... internal PropertySourceInfo PropertyInfoInternal() { return _thisElement.PropertyInfoInternal(_thisElement.ElementTagName); } internal void ChangeSourceAndLineNumber(PropertySourceInfo sourceInformation) { _thisElement.Values.ChangeSourceInfo(_thisElement.ElementTagName, sourceInformation); } // Source // // What is the source file where this data came from // public string Source { get { PropertySourceInfo psi = _thisElement.Values.GetSourceInfo(_thisElement.ElementTagName); if (psi == null) { return null; } return psi.FileName; } } // LineNumber // // What is the line number associated with the source // // Note: // 1 is the first line in the file. 0 is returned when there is no // source // public int LineNumber { get { PropertySourceInfo psi = _thisElement.Values.GetSourceInfo(_thisElement.ElementTagName); if (psi == null) { return 0; } return psi.LineNumber; } } // Type // // What is the type for the element // public Type Type { get { return _thisElement.GetType(); } } // Validator // // What is the validator to validate the element? // public ConfigurationValidatorBase Validator { get { return _thisElement.ElementProperty.Validator; } } // GetReadOnlyErrorsList // // Get a Read Only list of the exceptions for this // element // private ConfigurationException[] GetReadOnlyErrorsList() { ArrayList arrayList; int count; ConfigurationException[] exceptionList; arrayList = _thisElement.GetErrorsList(); count = arrayList.Count; // Create readonly array exceptionList = new ConfigurationException[arrayList.Count]; if (count != 0) { arrayList.CopyTo(exceptionList, 0); } return exceptionList; } // Errors // // Retrieve the _errors for this element and sub elements // public ICollection Errors { get { if (_errors == null) { _errors = GetReadOnlyErrorsList(); } return _errors; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Configuration; using System.Collections.Specialized; using System.ComponentModel; using System.Collections; using System.Runtime.Serialization; namespace System.Configuration { // ElementInformation // // Expose information on Configuration Elements, and the // properties that they contain // public sealed class ElementInformation { private ConfigurationElement _thisElement; private PropertyInformationCollection _internalProperties; private ConfigurationException[] _errors; internal ElementInformation(ConfigurationElement thisElement) { _thisElement = thisElement; } // Properties // // Retrieve Collection of properties within this element // public PropertyInformationCollection Properties { get { if (_internalProperties == null) { _internalProperties = new PropertyInformationCollection(_thisElement); } return _internalProperties; } } // IsInherited // // Was this element inheritted, or was the property actually // set here // public bool IsPresent { get { return _thisElement.ElementPresent; } } // IsLocked // // Is this property locked? // public bool IsLocked { get { return (((_thisElement.ItemLocked & ConfigurationValueFlags.Locked) != 0) && ((_thisElement.ItemLocked & ConfigurationValueFlags.Inherited) != 0)); } } // IsCollection // // Is this element a collection? // public bool IsCollection { get { ConfigurationElementCollection collection = _thisElement as ConfigurationElementCollection; if (collection == null) { // Try the default collection if (_thisElement.Properties.DefaultCollectionProperty != null) { // this is not a collection but it may contain a default collection collection = _thisElement[_thisElement.Properties.DefaultCollectionProperty] as ConfigurationElementCollection; } } return (collection != null); } } // Internal method to fix SetRawXML defect... internal PropertySourceInfo PropertyInfoInternal() { return _thisElement.PropertyInfoInternal(_thisElement.ElementTagName); } internal void ChangeSourceAndLineNumber(PropertySourceInfo sourceInformation) { _thisElement.Values.ChangeSourceInfo(_thisElement.ElementTagName, sourceInformation); } // Source // // What is the source file where this data came from // public string Source { get { PropertySourceInfo psi = _thisElement.Values.GetSourceInfo(_thisElement.ElementTagName); if (psi == null) { return null; } return psi.FileName; } } // LineNumber // // What is the line number associated with the source // // Note: // 1 is the first line in the file. 0 is returned when there is no // source // public int LineNumber { get { PropertySourceInfo psi = _thisElement.Values.GetSourceInfo(_thisElement.ElementTagName); if (psi == null) { return 0; } return psi.LineNumber; } } // Type // // What is the type for the element // public Type Type { get { return _thisElement.GetType(); } } // Validator // // What is the validator to validate the element? // public ConfigurationValidatorBase Validator { get { return _thisElement.ElementProperty.Validator; } } // GetReadOnlyErrorsList // // Get a Read Only list of the exceptions for this // element // private ConfigurationException[] GetReadOnlyErrorsList() { ArrayList arrayList; int count; ConfigurationException[] exceptionList; arrayList = _thisElement.GetErrorsList(); count = arrayList.Count; // Create readonly array exceptionList = new ConfigurationException[arrayList.Count]; if (count != 0) { arrayList.CopyTo(exceptionList, 0); } return exceptionList; } // Errors // // Retrieve the _errors for this element and sub elements // public ICollection Errors { get { if (_errors == null) { _errors = GetReadOnlyErrorsList(); } return _errors; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
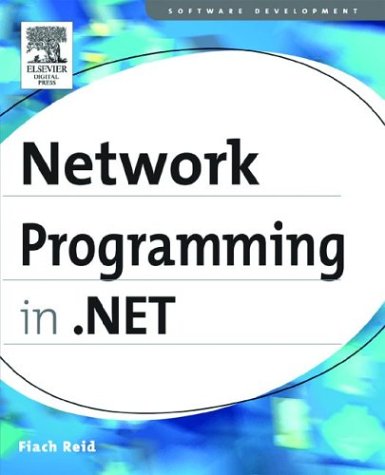
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HitTestWithGeometryDrawingContextWalker.cs
- BinaryMethodMessage.cs
- WebPartTransformerCollection.cs
- ConnectorRouter.cs
- GridItemProviderWrapper.cs
- mactripleDES.cs
- ProtocolReflector.cs
- UIElementCollection.cs
- OciHandle.cs
- ClaimComparer.cs
- PatternMatcher.cs
- StackOverflowException.cs
- SafeLocalMemHandle.cs
- DataContractSerializerFaultFormatter.cs
- ApplyTemplatesAction.cs
- CultureTableRecord.cs
- FloaterBaseParagraph.cs
- PointIndependentAnimationStorage.cs
- DependencyPropertyChangedEventArgs.cs
- SetterBaseCollection.cs
- ImageSource.cs
- DataGridColumn.cs
- SafeSecurityHandles.cs
- XmlCDATASection.cs
- StyleTypedPropertyAttribute.cs
- UncommonField.cs
- WorkflowCompensationBehavior.cs
- Speller.cs
- SessionStateSection.cs
- DrawingContextDrawingContextWalker.cs
- EventProviderClassic.cs
- ObsoleteAttribute.cs
- MsmqIntegrationChannelListener.cs
- ExceptionWrapper.cs
- DisplayInformation.cs
- ItemCollection.cs
- Label.cs
- SchemaTableOptionalColumn.cs
- CryptoApi.cs
- RectAnimationBase.cs
- DebugViewWriter.cs
- TempEnvironment.cs
- TrustManager.cs
- Knowncolors.cs
- ToolStripHighContrastRenderer.cs
- DbMetaDataColumnNames.cs
- NavigationProperty.cs
- DrawingContext.cs
- SerialPort.cs
- XPathDocumentBuilder.cs
- ViewgenGatekeeper.cs
- RuntimeVariablesExpression.cs
- CommonDialog.cs
- ThaiBuddhistCalendar.cs
- webeventbuffer.cs
- IntegrationExceptionEventArgs.cs
- GridViewColumn.cs
- RuntimeResourceSet.cs
- BindingElementExtensionElement.cs
- ObjectQuery.cs
- ThreadStartException.cs
- TextRangeEditLists.cs
- ColumnTypeConverter.cs
- VisualTreeHelper.cs
- __ComObject.cs
- XPathMultyIterator.cs
- SponsorHelper.cs
- DirectoryNotFoundException.cs
- KnownBoxes.cs
- PaperSource.cs
- ChangeDirector.cs
- ListArgumentProvider.cs
- AssemblyAttributes.cs
- DesignTimeParseData.cs
- ToolStripContentPanelDesigner.cs
- ScriptManager.cs
- ResumeStoryboard.cs
- PolyQuadraticBezierSegment.cs
- SendMailErrorEventArgs.cs
- StandardCommandToolStripMenuItem.cs
- DataGridColumnReorderingEventArgs.cs
- OleStrCAMarshaler.cs
- RepeaterItemEventArgs.cs
- MonikerUtility.cs
- XmlAttributeAttribute.cs
- EditorPartCollection.cs
- ConstrainedDataObject.cs
- FolderNameEditor.cs
- QuarticEase.cs
- NameSpaceExtractor.cs
- JsonGlobals.cs
- FlowThrottle.cs
- KoreanCalendar.cs
- MailFileEditor.cs
- ProvideValueServiceProvider.cs
- DataServiceQueryProvider.cs
- RegistrySecurity.cs
- WebRequestModuleElement.cs
- LinqDataSourceStatusEventArgs.cs
- DynamicPropertyHolder.cs