Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Common / QueryCache / EntitySqlQueryCacheKey.cs / 1 / EntitySqlQueryCacheKey.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //----------------------------------------------------------------------------- namespace System.Data.Common.QueryCache { using System; using System.Data.Objects; using System.Diagnostics; ////// Represents an Entity-SQL-based ObjectQuery Cache key context /// internal sealed class EntitySqlQueryCacheKey : QueryCacheKey { ////// Aggregate hashcode based the hashcode of the properties of this cache key /// private readonly int _hashCode; ////// The name of the default container in effect when the Entity-SQL text was parsed /// (affects whether or not the text can be successfully parsed) /// private string _defaultContainer; ////// Entity Sql statement /// private readonly string _eSqlStatement; ////// Parameter collection token /// private readonly string _parametersToken; ////// Number of parameters /// private readonly int _parameterCount; ////// Concatenated representation of the Include span paths /// private readonly string _includePathsToken; ////// The merge option in effect /// private readonly MergeOption _mergeOption; ////// Result type affects assembly plan. /// private readonly Type _resultType; ////// Creates a new instance of ObjectQueryCacheKey given a entityCommand instance /// /// The default container name in effect when parsing the query (may be null) /// The Entity-SQL text of the query /// The number of parameters to the query /// A string representation of the parameters to the query (may be null) /// A string representation of the Include span paths in effect (may be null) /// The merge option in effect. Required for result assembly. internal EntitySqlQueryCacheKey(string defaultContainerName, string eSqlStatement, int parameterCount, string parametersToken, string includePathsToken, MergeOption mergeOption, Type resultType) : base() { Debug.Assert(null != eSqlStatement, "eSqlStatement must not be null"); _defaultContainer = defaultContainerName; _eSqlStatement = eSqlStatement; _parameterCount = parameterCount; _parametersToken = parametersToken; _includePathsToken = includePathsToken; _mergeOption = mergeOption; _resultType = resultType; int combinedHash = _eSqlStatement.GetHashCode() ^ _mergeOption.GetHashCode(); if (_parametersToken != null) { combinedHash ^= _parametersToken.GetHashCode(); } if (_includePathsToken != null) { combinedHash ^= _includePathsToken.GetHashCode(); } if (_defaultContainer != null) { combinedHash ^= _defaultContainer.GetHashCode(); } _hashCode = combinedHash; } ////// Determines equality of two cache keys based on cache context values /// /// ///public override bool Equals(object otherObject) { Debug.Assert(null != otherObject, "otherObject must not be null"); if (typeof(EntitySqlQueryCacheKey) != otherObject.GetType()) { return false; } EntitySqlQueryCacheKey otherObjectQueryCacheKey = (EntitySqlQueryCacheKey)otherObject; // also use result type... return (_parameterCount == otherObjectQueryCacheKey._parameterCount) && (_mergeOption == otherObjectQueryCacheKey._mergeOption) && Equals(otherObjectQueryCacheKey._defaultContainer, _defaultContainer) && Equals(otherObjectQueryCacheKey._eSqlStatement, _eSqlStatement) && Equals(otherObjectQueryCacheKey._includePathsToken, _includePathsToken) && Equals(otherObjectQueryCacheKey._parametersToken, _parametersToken) && Equals(otherObjectQueryCacheKey._resultType, _resultType); } /// /// Returns the hashcode for this cache key /// ///public override int GetHashCode() { return _hashCode; } /// /// Returns a string representation of the state of this cache key /// ////// A string representation that includes query text, parameter information, include path information /// and merge option information about this cache key. /// public override string ToString() { return String.Join("|", new string[] { _defaultContainer, _eSqlStatement, _parametersToken, _includePathsToken, Enum.GetName(typeof(MergeOption), _mergeOption) }); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //----------------------------------------------------------------------------- namespace System.Data.Common.QueryCache { using System; using System.Data.Objects; using System.Diagnostics; ////// Represents an Entity-SQL-based ObjectQuery Cache key context /// internal sealed class EntitySqlQueryCacheKey : QueryCacheKey { ////// Aggregate hashcode based the hashcode of the properties of this cache key /// private readonly int _hashCode; ////// The name of the default container in effect when the Entity-SQL text was parsed /// (affects whether or not the text can be successfully parsed) /// private string _defaultContainer; ////// Entity Sql statement /// private readonly string _eSqlStatement; ////// Parameter collection token /// private readonly string _parametersToken; ////// Number of parameters /// private readonly int _parameterCount; ////// Concatenated representation of the Include span paths /// private readonly string _includePathsToken; ////// The merge option in effect /// private readonly MergeOption _mergeOption; ////// Result type affects assembly plan. /// private readonly Type _resultType; ////// Creates a new instance of ObjectQueryCacheKey given a entityCommand instance /// /// The default container name in effect when parsing the query (may be null) /// The Entity-SQL text of the query /// The number of parameters to the query /// A string representation of the parameters to the query (may be null) /// A string representation of the Include span paths in effect (may be null) /// The merge option in effect. Required for result assembly. internal EntitySqlQueryCacheKey(string defaultContainerName, string eSqlStatement, int parameterCount, string parametersToken, string includePathsToken, MergeOption mergeOption, Type resultType) : base() { Debug.Assert(null != eSqlStatement, "eSqlStatement must not be null"); _defaultContainer = defaultContainerName; _eSqlStatement = eSqlStatement; _parameterCount = parameterCount; _parametersToken = parametersToken; _includePathsToken = includePathsToken; _mergeOption = mergeOption; _resultType = resultType; int combinedHash = _eSqlStatement.GetHashCode() ^ _mergeOption.GetHashCode(); if (_parametersToken != null) { combinedHash ^= _parametersToken.GetHashCode(); } if (_includePathsToken != null) { combinedHash ^= _includePathsToken.GetHashCode(); } if (_defaultContainer != null) { combinedHash ^= _defaultContainer.GetHashCode(); } _hashCode = combinedHash; } ////// Determines equality of two cache keys based on cache context values /// /// ///public override bool Equals(object otherObject) { Debug.Assert(null != otherObject, "otherObject must not be null"); if (typeof(EntitySqlQueryCacheKey) != otherObject.GetType()) { return false; } EntitySqlQueryCacheKey otherObjectQueryCacheKey = (EntitySqlQueryCacheKey)otherObject; // also use result type... return (_parameterCount == otherObjectQueryCacheKey._parameterCount) && (_mergeOption == otherObjectQueryCacheKey._mergeOption) && Equals(otherObjectQueryCacheKey._defaultContainer, _defaultContainer) && Equals(otherObjectQueryCacheKey._eSqlStatement, _eSqlStatement) && Equals(otherObjectQueryCacheKey._includePathsToken, _includePathsToken) && Equals(otherObjectQueryCacheKey._parametersToken, _parametersToken) && Equals(otherObjectQueryCacheKey._resultType, _resultType); } /// /// Returns the hashcode for this cache key /// ///public override int GetHashCode() { return _hashCode; } /// /// Returns a string representation of the state of this cache key /// ////// A string representation that includes query text, parameter information, include path information /// and merge option information about this cache key. /// public override string ToString() { return String.Join("|", new string[] { _defaultContainer, _eSqlStatement, _parametersToken, _includePathsToken, Enum.GetName(typeof(MergeOption), _mergeOption) }); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
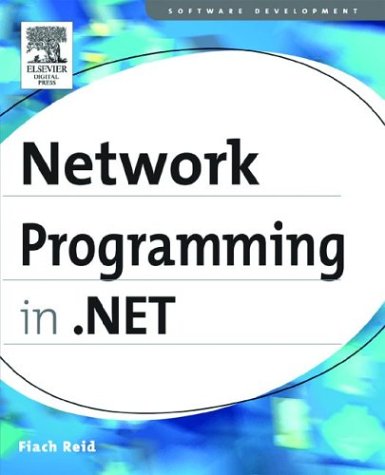
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PositiveTimeSpanValidatorAttribute.cs
- SchemaContext.cs
- ConversionContext.cs
- ValidatorCollection.cs
- PrintDialog.cs
- ImagingCache.cs
- CalendarDesigner.cs
- UIElement.cs
- RootProjectionNode.cs
- UserControlDocumentDesigner.cs
- SmiMetaDataProperty.cs
- UITypeEditor.cs
- IISUnsafeMethods.cs
- ExtractorMetadata.cs
- SlotInfo.cs
- BitmapPalette.cs
- Win32Exception.cs
- ToolStripHighContrastRenderer.cs
- ServiceInstallComponent.cs
- DataControlFieldsEditor.cs
- DataGridLinkButton.cs
- SchemaImporterExtensionsSection.cs
- EventEntry.cs
- GatewayDefinition.cs
- Propagator.Evaluator.cs
- FunctionImportMapping.cs
- IndentedWriter.cs
- DifferencingCollection.cs
- NameValueConfigurationElement.cs
- SubqueryRules.cs
- ValidationHelpers.cs
- StyleTypedPropertyAttribute.cs
- SessionState.cs
- BaseTreeIterator.cs
- PrintDocument.cs
- ParserExtension.cs
- DateTimeStorage.cs
- CaseInsensitiveComparer.cs
- TabControlAutomationPeer.cs
- EntityDataSourceContextCreatingEventArgs.cs
- Model3D.cs
- ISFClipboardData.cs
- ToolStripGripRenderEventArgs.cs
- WorkflowPersistenceService.cs
- Run.cs
- FlatButtonAppearance.cs
- RuleSetReference.cs
- CompilerTypeWithParams.cs
- GridViewRowEventArgs.cs
- MappingItemCollection.cs
- SharedPersonalizationStateInfo.cs
- XXXInfos.cs
- DependencyObjectCodeDomSerializer.cs
- AttachedPropertyDescriptor.cs
- XmlSignificantWhitespace.cs
- ReaderWriterLock.cs
- SecurityAttributeGenerationHelper.cs
- ThreadInterruptedException.cs
- StdValidatorsAndConverters.cs
- CheckBoxStandardAdapter.cs
- DropDownList.cs
- ObjectDataSource.cs
- EventHandlers.cs
- CatalogZone.cs
- ClickablePoint.cs
- BasicSecurityProfileVersion.cs
- ToolbarAUtomationPeer.cs
- TypeListConverter.cs
- BasicViewGenerator.cs
- QilBinary.cs
- SmiSettersStream.cs
- DbDataAdapter.cs
- Exception.cs
- PanelStyle.cs
- Pair.cs
- IArgumentProvider.cs
- ProcessHostFactoryHelper.cs
- ClientData.cs
- TableCellAutomationPeer.cs
- State.cs
- ModulesEntry.cs
- BaseTypeViewSchema.cs
- DragDeltaEventArgs.cs
- MaskedTextProvider.cs
- AnnotationHighlightLayer.cs
- WebPageTraceListener.cs
- translator.cs
- ContextMarshalException.cs
- Message.cs
- WebBrowsableAttribute.cs
- Translator.cs
- GridViewHeaderRowPresenter.cs
- Image.cs
- SharedUtils.cs
- MatrixCamera.cs
- PanelDesigner.cs
- XmlCodeExporter.cs
- LineInfo.cs
- TextEditorCopyPaste.cs
- ShapeTypeface.cs