Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Base / System / Windows / LocalValueEnumerator.cs / 1 / LocalValueEnumerator.cs
using System; using System.Collections; using System.Diagnostics; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows { ////// Local value enumeration object /// ////// Modifying local values (via SetValue or ClearValue) during enumeration /// is unsupported /// public struct LocalValueEnumerator : IEnumerator { ////// Overrides Object.GetHashCode /// ///An integer that represents the hashcode for this object public override int GetHashCode() { return base.GetHashCode(); } ////// Determine equality /// public override bool Equals(object obj) { LocalValueEnumerator other = (LocalValueEnumerator) obj; return (_count == other._count && _index == other._index && _snapshot == other._snapshot); } ////// Determine equality /// public static bool operator ==(LocalValueEnumerator obj1, LocalValueEnumerator obj2) { return obj1.Equals(obj2); } ////// Determine inequality /// public static bool operator !=(LocalValueEnumerator obj1, LocalValueEnumerator obj2) { return !(obj1 == obj2); } ////// Get current entry /// public LocalValueEntry Current { get { if(_index == -1 ) { #pragma warning suppress 6503 // IEnumerator.Current is documented to throw this exception throw new InvalidOperationException(SR.Get(SRID.LocalValueEnumerationReset)); } if(_index >= Count ) { #pragma warning suppress 6503 // IEnumerator.Current is documented to throw this exception throw new InvalidOperationException(SR.Get(SRID.LocalValueEnumerationOutOfBounds)); } return _snapshot[_index]; } } ////// Get current entry (object reference based) /// object IEnumerator.Current { get { return Current; } } ////// Move to the next item in the enumerator /// ///Success of the method public bool MoveNext() { _index++; return _index < Count; } ////// Reset enumeration /// public void Reset() { _index = -1; } ////// Return number of items represented in the collection /// public int Count { get { return _count; } } internal LocalValueEnumerator(LocalValueEntry[] snapshot, int count) { _index = -1; _count = count; _snapshot = snapshot; } private int _index; private LocalValueEntry[] _snapshot; private int _count; } ////// Represents a Property-Value pair for local value enumeration /// public struct LocalValueEntry { ////// Overrides Object.GetHashCode /// ///An integer that represents the hashcode for this object public override int GetHashCode() { return base.GetHashCode(); } ////// Determine equality /// public override bool Equals(object obj) { LocalValueEntry other = (LocalValueEntry) obj; return (_dp == other._dp && _value == other._value); } ////// Determine equality /// public static bool operator ==(LocalValueEntry obj1, LocalValueEntry obj2) { return obj1.Equals(obj2); } ////// Determine inequality /// public static bool operator !=(LocalValueEntry obj1, LocalValueEntry obj2) { return !(obj1 == obj2); } ////// Dependency property /// public DependencyProperty Property { get { return _dp; } } ////// Value of the property /// public object Value { get { return _value; } } internal LocalValueEntry(DependencyProperty dp, object value) { _dp = dp; _value = value; } // Internal here because we need to change these around when building // the snapshot for the LocalValueEnumerator, and we can't make internal // setters when we have public getters. internal DependencyProperty _dp; internal object _value; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
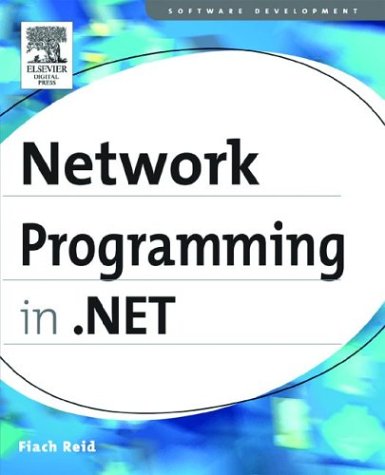
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentSchemaValidator.cs
- DtrList.cs
- SafeEventHandle.cs
- ItemAutomationPeer.cs
- SoapEnumAttribute.cs
- SimpleWorkerRequest.cs
- XAMLParseException.cs
- _OverlappedAsyncResult.cs
- Page.cs
- ComponentResourceManager.cs
- MutexSecurity.cs
- RegistryPermission.cs
- PiiTraceSource.cs
- DataFieldConverter.cs
- ColumnMapProcessor.cs
- BidOverLoads.cs
- SmtpReplyReader.cs
- TextTrailingWordEllipsis.cs
- MulticastIPAddressInformationCollection.cs
- TextStore.cs
- ObjectDataSourceFilteringEventArgs.cs
- EventSetter.cs
- StorageFunctionMapping.cs
- CompositionAdorner.cs
- Int16AnimationUsingKeyFrames.cs
- FilterQueryOptionExpression.cs
- ElementHostPropertyMap.cs
- HttpApplicationStateWrapper.cs
- Currency.cs
- WindowVisualStateTracker.cs
- GlyphManager.cs
- InstanceDataCollectionCollection.cs
- StreamInfo.cs
- ApplicationId.cs
- Point3DCollection.cs
- HyperLink.cs
- BinaryNode.cs
- CodeBlockBuilder.cs
- Model3D.cs
- ValidationErrorEventArgs.cs
- ValidationSummary.cs
- ActiveXHelper.cs
- PrinterUnitConvert.cs
- MimeBasePart.cs
- ToolStripCodeDomSerializer.cs
- CompoundFileStorageReference.cs
- BitmapImage.cs
- ChtmlCommandAdapter.cs
- XamlUtilities.cs
- SignatureToken.cs
- HttpValueCollection.cs
- ReadOnlyMetadataCollection.cs
- ModuleElement.cs
- XmlSignificantWhitespace.cs
- DataGridViewLayoutData.cs
- OleDbInfoMessageEvent.cs
- CacheAxisQuery.cs
- XsdBuildProvider.cs
- GuidConverter.cs
- StringTraceRecord.cs
- Component.cs
- BitmapImage.cs
- OrthographicCamera.cs
- EndpointNameMessageFilter.cs
- HwndTarget.cs
- ReadOnlyMetadataCollection.cs
- DbModificationCommandTree.cs
- SubtreeProcessor.cs
- HostSecurityManager.cs
- Atom10FormatterFactory.cs
- Double.cs
- FileInfo.cs
- UnsafeNativeMethods.cs
- ExpressionCopier.cs
- RegionData.cs
- BindingNavigatorDesigner.cs
- SecurityResources.cs
- IItemProperties.cs
- ConfigurationSectionGroup.cs
- UICuesEvent.cs
- DataControlField.cs
- EntityCommand.cs
- XamlParser.cs
- FixedSOMGroup.cs
- Addressing.cs
- SafeNativeMethods.cs
- PtsCache.cs
- XmlLinkedNode.cs
- ToolStripSeparatorRenderEventArgs.cs
- WmiEventSink.cs
- OrderingQueryOperator.cs
- QueueProcessor.cs
- TextElement.cs
- OracleDataReader.cs
- InProcStateClientManager.cs
- ObjectDataSourceSelectingEventArgs.cs
- ToolBarButtonClickEvent.cs
- SoapSchemaImporter.cs
- SHA512.cs
- MultiTargetingUtil.cs