Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Activities / Rules / Exceptions.cs / 1305376 / Exceptions.cs
// ---------------------------------------------------------------------------- // Copyright (C) 2005 Microsoft Corporation - All Rights Reserved // --------------------------------------------------------------------------- using System; using System.CodeDom; using System.Security.Permissions; using System.Runtime.Serialization; using System.Workflow.ComponentModel.Compiler; namespace System.Workflow.Activities.Rules { #region RuleException ////// Represents the base class for all rule engine exception classes /// [Serializable] public class RuleException : Exception, ISerializable { ////// Initializes a new instance of the RuleException class /// public RuleException() : base() { } ////// Initializes a new instance of the RuleException class /// /// The error message that explains the reason for the exception public RuleException(string message) : base(message) { } ////// Initializes a new instance of the RuleException class /// /// The error message that explains the reason for the exception /// The inner exception public RuleException(string message, Exception ex) : base(message, ex) { } ////// Constructor required by for Serialization - initialize a new instance from serialized data /// /// Reference to the object that holds the data needed to deserialize the exception /// Provides the means for deserializing the exception data protected RuleException(SerializationInfo serializeInfo, StreamingContext context) : base(serializeInfo, context) { } } #endregion #region RuleEvaluationException ////// Represents the the exception thrown when an error is encountered during evaluation /// [Serializable] public class RuleEvaluationException : RuleException, ISerializable { ////// Initializes a new instance of the RuleRuntimeException class /// public RuleEvaluationException() : base() { } ////// Initializes a new instance of the RuleRuntimeException class /// /// The error message that explains the reason for the exception public RuleEvaluationException(string message) : base(message) { } ////// Initializes a new instance of the RuleRuntimeException class /// /// The error message that explains the reason for the exception /// The inner exception public RuleEvaluationException(string message, Exception ex) : base(message, ex) { } ////// Constructor required by for Serialization - initialize a new instance from serialized data /// /// Reference to the object that holds the data needed to deserialize the exception /// Provides the means for deserializing the exception data protected RuleEvaluationException(SerializationInfo serializeInfo, StreamingContext context) : base(serializeInfo, context) { } } #endregion #region RuleEvaluationIncompatibleTypesException ////// Represents the exception thrown when types are incompatible /// [Serializable] public class RuleEvaluationIncompatibleTypesException : RuleException, ISerializable { private Type m_leftType; private CodeBinaryOperatorType m_op; private Type m_rightType; ////// Type on the left of the operator /// public Type Left { get { return m_leftType; } set { m_leftType = value; } } ////// Arithmetic operation that failed /// public CodeBinaryOperatorType Operator { get { return m_op; } set { m_op = value; } } ////// Type on the right of the operator /// public Type Right { get { return m_rightType; } set { m_rightType = value; } } ////// Initializes a new instance of the RuleEvaluationIncompatibleTypesException class /// public RuleEvaluationIncompatibleTypesException() : base() { } ////// Initializes a new instance of the RuleEvaluationIncompatibleTypesException class /// /// public RuleEvaluationIncompatibleTypesException(string message) : base(message) { } ////// Initializes a new instance of the RuleEvaluationIncompatibleTypesException class /// /// /// public RuleEvaluationIncompatibleTypesException(string message, Exception ex) : base(message, ex) { } ////// Initializes a new instance of the RuleEvaluationIncompatibleTypesException class /// /// /// /// /// public RuleEvaluationIncompatibleTypesException( string message, Type left, CodeBinaryOperatorType op, Type right) : base(message) { m_leftType = left; m_op = op; m_rightType = right; } ////// Initializes a new instance of the RuleEvaluationIncompatibleTypesException class /// /// /// /// /// /// public RuleEvaluationIncompatibleTypesException( string message, Type left, CodeBinaryOperatorType op, Type right, Exception ex) : base(message, ex) { m_leftType = left; m_op = op; m_rightType = right; } ////// Constructor required by for Serialization - initialize a new instance from serialized data /// /// Reference to the object that holds the data needed to deserialize the exception /// Provides the means for deserializing the exception data protected RuleEvaluationIncompatibleTypesException(SerializationInfo serializeInfo, StreamingContext context) : base(serializeInfo, context) { if (serializeInfo == null) throw new ArgumentNullException("serializeInfo"); string qualifiedTypeString = serializeInfo.GetString("left"); if (qualifiedTypeString != "null") m_leftType = Type.GetType(qualifiedTypeString); m_op = (CodeBinaryOperatorType)serializeInfo.GetValue("op", typeof(CodeBinaryOperatorType)); qualifiedTypeString = serializeInfo.GetString("right"); if (qualifiedTypeString != "null") m_rightType = Type.GetType(qualifiedTypeString); } ////// Implements the ISerializable interface /// /// Reference to the object that holds the data needed to serialize/deserialize the exception /// Provides the means for serialiing the exception data [SecurityPermissionAttribute(SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info == null) throw new ArgumentNullException("info"); base.GetObjectData(info, context); info.AddValue("left", (m_leftType != null) ? m_leftType.AssemblyQualifiedName : "null"); info.AddValue("op", m_op); info.AddValue("right", (m_rightType != null) ? m_rightType.AssemblyQualifiedName : "null"); } } #endregion #region RuleSetValidationException ////// Represents the exception thrown when a ruleset can not be validated /// [Serializable] public class RuleSetValidationException : RuleException, ISerializable { private ValidationErrorCollection m_errors; ////// Collection of validation errors that occurred while validating the RuleSet /// public ValidationErrorCollection Errors { get { return m_errors; } } ////// Initializes a new instance of the RuleSetValidationException class /// public RuleSetValidationException() : base() { } ////// Initializes a new instance of the RuleSetValidationException class /// /// public RuleSetValidationException(string message) : base(message) { } ////// Initializes a new instance of the RuleSetValidationException class /// /// /// public RuleSetValidationException(string message, Exception ex) : base(message, ex) { } ////// Initializes a new instance of the RuleSetValidationException class /// /// /// public RuleSetValidationException( string message, ValidationErrorCollection errors) : base(message) { m_errors = errors; } ////// Constructor required by for Serialization - initialize a new instance from serialized data /// /// Reference to the object that holds the data needed to deserialize the exception /// Provides the means for deserializing the exception data protected RuleSetValidationException(SerializationInfo serializeInfo, StreamingContext context) : base(serializeInfo, context) { if (serializeInfo == null) throw new ArgumentNullException("serializeInfo"); m_errors = (ValidationErrorCollection)serializeInfo.GetValue("errors", typeof(ValidationErrorCollection)); } ////// Implements the ISerializable interface /// /// Reference to the object that holds the data needed to serialize/deserialize the exception /// Provides the means for serialiing the exception data [SecurityPermissionAttribute(SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info == null) throw new ArgumentNullException("info"); base.GetObjectData(info, context); info.AddValue("errors", m_errors); } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. // ---------------------------------------------------------------------------- // Copyright (C) 2005 Microsoft Corporation - All Rights Reserved // --------------------------------------------------------------------------- using System; using System.CodeDom; using System.Security.Permissions; using System.Runtime.Serialization; using System.Workflow.ComponentModel.Compiler; namespace System.Workflow.Activities.Rules { #region RuleException ////// Represents the base class for all rule engine exception classes /// [Serializable] public class RuleException : Exception, ISerializable { ////// Initializes a new instance of the RuleException class /// public RuleException() : base() { } ////// Initializes a new instance of the RuleException class /// /// The error message that explains the reason for the exception public RuleException(string message) : base(message) { } ////// Initializes a new instance of the RuleException class /// /// The error message that explains the reason for the exception /// The inner exception public RuleException(string message, Exception ex) : base(message, ex) { } ////// Constructor required by for Serialization - initialize a new instance from serialized data /// /// Reference to the object that holds the data needed to deserialize the exception /// Provides the means for deserializing the exception data protected RuleException(SerializationInfo serializeInfo, StreamingContext context) : base(serializeInfo, context) { } } #endregion #region RuleEvaluationException ////// Represents the the exception thrown when an error is encountered during evaluation /// [Serializable] public class RuleEvaluationException : RuleException, ISerializable { ////// Initializes a new instance of the RuleRuntimeException class /// public RuleEvaluationException() : base() { } ////// Initializes a new instance of the RuleRuntimeException class /// /// The error message that explains the reason for the exception public RuleEvaluationException(string message) : base(message) { } ////// Initializes a new instance of the RuleRuntimeException class /// /// The error message that explains the reason for the exception /// The inner exception public RuleEvaluationException(string message, Exception ex) : base(message, ex) { } ////// Constructor required by for Serialization - initialize a new instance from serialized data /// /// Reference to the object that holds the data needed to deserialize the exception /// Provides the means for deserializing the exception data protected RuleEvaluationException(SerializationInfo serializeInfo, StreamingContext context) : base(serializeInfo, context) { } } #endregion #region RuleEvaluationIncompatibleTypesException ////// Represents the exception thrown when types are incompatible /// [Serializable] public class RuleEvaluationIncompatibleTypesException : RuleException, ISerializable { private Type m_leftType; private CodeBinaryOperatorType m_op; private Type m_rightType; ////// Type on the left of the operator /// public Type Left { get { return m_leftType; } set { m_leftType = value; } } ////// Arithmetic operation that failed /// public CodeBinaryOperatorType Operator { get { return m_op; } set { m_op = value; } } ////// Type on the right of the operator /// public Type Right { get { return m_rightType; } set { m_rightType = value; } } ////// Initializes a new instance of the RuleEvaluationIncompatibleTypesException class /// public RuleEvaluationIncompatibleTypesException() : base() { } ////// Initializes a new instance of the RuleEvaluationIncompatibleTypesException class /// /// public RuleEvaluationIncompatibleTypesException(string message) : base(message) { } ////// Initializes a new instance of the RuleEvaluationIncompatibleTypesException class /// /// /// public RuleEvaluationIncompatibleTypesException(string message, Exception ex) : base(message, ex) { } ////// Initializes a new instance of the RuleEvaluationIncompatibleTypesException class /// /// /// /// /// public RuleEvaluationIncompatibleTypesException( string message, Type left, CodeBinaryOperatorType op, Type right) : base(message) { m_leftType = left; m_op = op; m_rightType = right; } ////// Initializes a new instance of the RuleEvaluationIncompatibleTypesException class /// /// /// /// /// /// public RuleEvaluationIncompatibleTypesException( string message, Type left, CodeBinaryOperatorType op, Type right, Exception ex) : base(message, ex) { m_leftType = left; m_op = op; m_rightType = right; } ////// Constructor required by for Serialization - initialize a new instance from serialized data /// /// Reference to the object that holds the data needed to deserialize the exception /// Provides the means for deserializing the exception data protected RuleEvaluationIncompatibleTypesException(SerializationInfo serializeInfo, StreamingContext context) : base(serializeInfo, context) { if (serializeInfo == null) throw new ArgumentNullException("serializeInfo"); string qualifiedTypeString = serializeInfo.GetString("left"); if (qualifiedTypeString != "null") m_leftType = Type.GetType(qualifiedTypeString); m_op = (CodeBinaryOperatorType)serializeInfo.GetValue("op", typeof(CodeBinaryOperatorType)); qualifiedTypeString = serializeInfo.GetString("right"); if (qualifiedTypeString != "null") m_rightType = Type.GetType(qualifiedTypeString); } ////// Implements the ISerializable interface /// /// Reference to the object that holds the data needed to serialize/deserialize the exception /// Provides the means for serialiing the exception data [SecurityPermissionAttribute(SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info == null) throw new ArgumentNullException("info"); base.GetObjectData(info, context); info.AddValue("left", (m_leftType != null) ? m_leftType.AssemblyQualifiedName : "null"); info.AddValue("op", m_op); info.AddValue("right", (m_rightType != null) ? m_rightType.AssemblyQualifiedName : "null"); } } #endregion #region RuleSetValidationException ////// Represents the exception thrown when a ruleset can not be validated /// [Serializable] public class RuleSetValidationException : RuleException, ISerializable { private ValidationErrorCollection m_errors; ////// Collection of validation errors that occurred while validating the RuleSet /// public ValidationErrorCollection Errors { get { return m_errors; } } ////// Initializes a new instance of the RuleSetValidationException class /// public RuleSetValidationException() : base() { } ////// Initializes a new instance of the RuleSetValidationException class /// /// public RuleSetValidationException(string message) : base(message) { } ////// Initializes a new instance of the RuleSetValidationException class /// /// /// public RuleSetValidationException(string message, Exception ex) : base(message, ex) { } ////// Initializes a new instance of the RuleSetValidationException class /// /// /// public RuleSetValidationException( string message, ValidationErrorCollection errors) : base(message) { m_errors = errors; } ////// Constructor required by for Serialization - initialize a new instance from serialized data /// /// Reference to the object that holds the data needed to deserialize the exception /// Provides the means for deserializing the exception data protected RuleSetValidationException(SerializationInfo serializeInfo, StreamingContext context) : base(serializeInfo, context) { if (serializeInfo == null) throw new ArgumentNullException("serializeInfo"); m_errors = (ValidationErrorCollection)serializeInfo.GetValue("errors", typeof(ValidationErrorCollection)); } ////// Implements the ISerializable interface /// /// Reference to the object that holds the data needed to serialize/deserialize the exception /// Provides the means for serialiing the exception data [SecurityPermissionAttribute(SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info == null) throw new ArgumentNullException("info"); base.GetObjectData(info, context); info.AddValue("errors", m_errors); } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
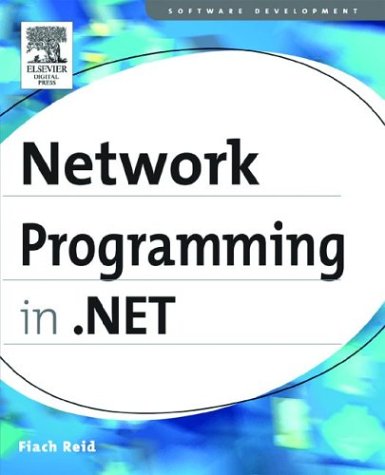
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StreamGeometry.cs
- FolderLevelBuildProvider.cs
- PriorityRange.cs
- entityreference_tresulttype.cs
- ObjectParameterCollection.cs
- oledbmetadatacolumnnames.cs
- StringFreezingAttribute.cs
- ComAdminWrapper.cs
- PrintDialogException.cs
- CanExecuteRoutedEventArgs.cs
- ValidationSummaryDesigner.cs
- PathBox.cs
- SymbolDocumentInfo.cs
- XmlSchemaInferenceException.cs
- SortExpressionBuilder.cs
- FixedTextBuilder.cs
- WindowAutomationPeer.cs
- RestHandlerFactory.cs
- WinFormsUtils.cs
- WindowsToolbar.cs
- WebPartDisplayModeCancelEventArgs.cs
- processwaithandle.cs
- ProcessHostFactoryHelper.cs
- Win32.cs
- NegotiationTokenAuthenticator.cs
- DisableDpiAwarenessAttribute.cs
- Int32RectValueSerializer.cs
- BinaryExpression.cs
- ToolboxItem.cs
- XPathAncestorIterator.cs
- CriticalFinalizerObject.cs
- Parsers.cs
- WSHttpSecurityElement.cs
- RequestCachePolicy.cs
- _Semaphore.cs
- PropertyCollection.cs
- RequestChannel.cs
- PerformanceCounterPermissionEntryCollection.cs
- FilterEventArgs.cs
- DbParameterCollectionHelper.cs
- TextBox.cs
- IpcManager.cs
- StyleReferenceConverter.cs
- XmlSchemaSimpleType.cs
- HttpContext.cs
- CommonObjectSecurity.cs
- SQLDateTimeStorage.cs
- SQLResource.cs
- xsdvalidator.cs
- RangeBase.cs
- initElementDictionary.cs
- PeerNearMe.cs
- NavigateEvent.cs
- Validator.cs
- HTTPNotFoundHandler.cs
- PointCollection.cs
- SmiSettersStream.cs
- CultureInfoConverter.cs
- ImageAnimator.cs
- UriParserTemplates.cs
- NativeMethods.cs
- WinFormsSecurity.cs
- HtmlTernaryTree.cs
- HttpResponseHeader.cs
- DesignerDeviceConfig.cs
- SrgsSemanticInterpretationTag.cs
- CoTaskMemSafeHandle.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- TypeNameConverter.cs
- Decorator.cs
- PathSegmentCollection.cs
- TypeForwardedToAttribute.cs
- RsaSecurityTokenParameters.cs
- CanonicalizationDriver.cs
- FixUpCollection.cs
- BamlRecordWriter.cs
- DeclaredTypeValidator.cs
- QilName.cs
- CodeLabeledStatement.cs
- SafeNativeMethodsMilCoreApi.cs
- IsolatedStorage.cs
- ProxyAttribute.cs
- ComplexTypeEmitter.cs
- LinearGradientBrush.cs
- AssemblyCollection.cs
- ContainerParagraph.cs
- QuinticEase.cs
- Stack.cs
- BitmapDecoder.cs
- DataProtection.cs
- IIS7UserPrincipal.cs
- HttpRuntimeSection.cs
- StatusStrip.cs
- TextRangeBase.cs
- WrappingXamlSchemaContext.cs
- ExtensionElementCollection.cs
- compensatingcollection.cs
- FileCodeGroup.cs
- XPathParser.cs
- StatusBarItemAutomationPeer.cs