Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / PathBox.cs / 1305376 / PathBox.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces. using System; using System.Text; using System.Collections.Generic; using System.Diagnostics; using System.Reflection; using System.Linq; using System.Linq.Expressions; #endregion Namespaces. ////// Holds state (Path, lambda parameter stack, etc) for projection analysis. /// internal class PathBox { #region Private fields. ///This class is used as a marker for an entity projected in its entirety. private const char EntireEntityMarker = UriHelper.ASTERISK; private readonly ListprojectionPaths = new List (); private readonly List expandPaths = new List (); private readonly Stack parameterExpressions = new Stack (); private readonly Dictionary basePaths = new Dictionary (ReferenceEqualityComparer .Instance); #endregion Private fields. /// Initializes a new internal PathBox() { // add a default empty path. projectionPaths.Add(new StringBuilder()); } internal IEnumerableinstance. ProjectionPaths { get { return projectionPaths.Where(s => s.Length > 0).Select(s => s.ToString()).Distinct(); } } internal IEnumerable ExpandPaths { get { return expandPaths.Where(s => s.Length > 0).Select(s => s.ToString()).Distinct(); } } internal void PushParamExpression(ParameterExpression pe) { StringBuilder basePath = projectionPaths.Last(); basePaths.Add(pe, basePath.ToString()); projectionPaths.Remove(basePath); parameterExpressions.Push(pe); } internal void PopParamExpression() { parameterExpressions.Pop(); } internal ParameterExpression ParamExpressionInScope { get { Debug.Assert(parameterExpressions.Count > 0); return parameterExpressions.Peek(); } } /// Starts a new path. internal void StartNewPath() { Debug.Assert(this.ParamExpressionInScope != null, "this.ParamExpressionInScope != null -- should not be starting new path with no lambda parameter in scope."); StringBuilder sb = new StringBuilder(basePaths[this.ParamExpressionInScope]); RemoveEntireEntityMarkerIfPresent(sb); expandPaths.Add(new StringBuilder(sb.ToString())); AddEntireEntityMarker(sb); projectionPaths.Add(sb); } internal void AppendToPath(PropertyInfo pi) { Debug.Assert(pi != null, "pi != null"); StringBuilder sb; Type t = TypeSystem.GetElementType(pi.PropertyType); if (ClientType.CheckElementTypeIsEntity(t)) { // an entity, so need to append to expand path also sb = expandPaths.Last(); Debug.Assert(sb != null); // there should always be an expand path because must call StartNewPath first. if (sb.Length > 0) { sb.Append(UriHelper.FORWARDSLASH); } sb.Append(pi.Name); } sb = projectionPaths.Last(); Debug.Assert(sb != null, "sb != null -- we are always building paths in the context of a parameter"); RemoveEntireEntityMarkerIfPresent(sb); if (sb.Length > 0) { sb.Append(UriHelper.FORWARDSLASH); } sb.Append(pi.Name); if (ClientType.CheckElementTypeIsEntity(t)) { AddEntireEntityMarker(sb); } } private static void RemoveEntireEntityMarkerIfPresent(StringBuilder sb) { if (sb.Length > 0 && sb[sb.Length - 1] == EntireEntityMarker) { sb.Remove(sb.Length - 1, 1); } if (sb.Length > 0 && sb[sb.Length - 1] == UriHelper.FORWARDSLASH) { sb.Remove(sb.Length - 1, 1); } } private static void AddEntireEntityMarker(StringBuilder sb) { if (sb.Length > 0) { sb.Append(UriHelper.FORWARDSLASH); } sb.Append(EntireEntityMarker); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces. using System; using System.Text; using System.Collections.Generic; using System.Diagnostics; using System.Reflection; using System.Linq; using System.Linq.Expressions; #endregion Namespaces. ////// Holds state (Path, lambda parameter stack, etc) for projection analysis. /// internal class PathBox { #region Private fields. ///This class is used as a marker for an entity projected in its entirety. private const char EntireEntityMarker = UriHelper.ASTERISK; private readonly ListprojectionPaths = new List (); private readonly List expandPaths = new List (); private readonly Stack parameterExpressions = new Stack (); private readonly Dictionary basePaths = new Dictionary (ReferenceEqualityComparer .Instance); #endregion Private fields. /// Initializes a new internal PathBox() { // add a default empty path. projectionPaths.Add(new StringBuilder()); } internal IEnumerableinstance. ProjectionPaths { get { return projectionPaths.Where(s => s.Length > 0).Select(s => s.ToString()).Distinct(); } } internal IEnumerable ExpandPaths { get { return expandPaths.Where(s => s.Length > 0).Select(s => s.ToString()).Distinct(); } } internal void PushParamExpression(ParameterExpression pe) { StringBuilder basePath = projectionPaths.Last(); basePaths.Add(pe, basePath.ToString()); projectionPaths.Remove(basePath); parameterExpressions.Push(pe); } internal void PopParamExpression() { parameterExpressions.Pop(); } internal ParameterExpression ParamExpressionInScope { get { Debug.Assert(parameterExpressions.Count > 0); return parameterExpressions.Peek(); } } /// Starts a new path. internal void StartNewPath() { Debug.Assert(this.ParamExpressionInScope != null, "this.ParamExpressionInScope != null -- should not be starting new path with no lambda parameter in scope."); StringBuilder sb = new StringBuilder(basePaths[this.ParamExpressionInScope]); RemoveEntireEntityMarkerIfPresent(sb); expandPaths.Add(new StringBuilder(sb.ToString())); AddEntireEntityMarker(sb); projectionPaths.Add(sb); } internal void AppendToPath(PropertyInfo pi) { Debug.Assert(pi != null, "pi != null"); StringBuilder sb; Type t = TypeSystem.GetElementType(pi.PropertyType); if (ClientType.CheckElementTypeIsEntity(t)) { // an entity, so need to append to expand path also sb = expandPaths.Last(); Debug.Assert(sb != null); // there should always be an expand path because must call StartNewPath first. if (sb.Length > 0) { sb.Append(UriHelper.FORWARDSLASH); } sb.Append(pi.Name); } sb = projectionPaths.Last(); Debug.Assert(sb != null, "sb != null -- we are always building paths in the context of a parameter"); RemoveEntireEntityMarkerIfPresent(sb); if (sb.Length > 0) { sb.Append(UriHelper.FORWARDSLASH); } sb.Append(pi.Name); if (ClientType.CheckElementTypeIsEntity(t)) { AddEntireEntityMarker(sb); } } private static void RemoveEntireEntityMarkerIfPresent(StringBuilder sb) { if (sb.Length > 0 && sb[sb.Length - 1] == EntireEntityMarker) { sb.Remove(sb.Length - 1, 1); } if (sb.Length > 0 && sb[sb.Length - 1] == UriHelper.FORWARDSLASH) { sb.Remove(sb.Length - 1, 1); } } private static void AddEntireEntityMarker(StringBuilder sb) { if (sb.Length > 0) { sb.Append(UriHelper.FORWARDSLASH); } sb.Append(EntireEntityMarker); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
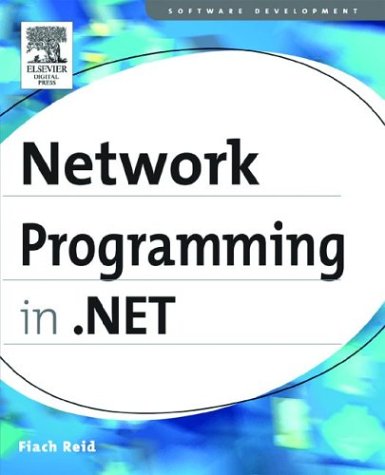
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Utils.cs
- MustUnderstandSoapException.cs
- UtilityExtension.cs
- SequentialUshortCollection.cs
- WorkflowFileItem.cs
- HierarchicalDataTemplate.cs
- ProcessHostMapPath.cs
- TraceHwndHost.cs
- ComponentCommands.cs
- TempFiles.cs
- DataTransferEventArgs.cs
- UnsafeNativeMethods.cs
- SelectionChangedEventArgs.cs
- Expression.cs
- InheritedPropertyChangedEventArgs.cs
- HtmlWindowCollection.cs
- SqlCacheDependency.cs
- DescriptionAttribute.cs
- UIElementParagraph.cs
- TextRunTypographyProperties.cs
- OraclePermissionAttribute.cs
- DetailsViewDeleteEventArgs.cs
- ExtenderControl.cs
- SqlUserDefinedAggregateAttribute.cs
- JsonWriterDelegator.cs
- CompleteWizardStep.cs
- WebDisplayNameAttribute.cs
- GeneratedContractType.cs
- EncodedStreamFactory.cs
- ThaiBuddhistCalendar.cs
- X509CertificateClaimSet.cs
- CompositeDataBoundControl.cs
- ScrollContentPresenter.cs
- FloatMinMaxAggregationOperator.cs
- _UriSyntax.cs
- HttpPostedFile.cs
- WebPartConnection.cs
- OdbcParameter.cs
- HtmlInputPassword.cs
- XmlChildNodes.cs
- ContentHostHelper.cs
- SafeThreadHandle.cs
- Walker.cs
- ValueType.cs
- Panel.cs
- HttpConfigurationContext.cs
- StylusPointPropertyInfoDefaults.cs
- ApplicationSecurityInfo.cs
- VScrollBar.cs
- SkipQueryOptionExpression.cs
- RSAPKCS1KeyExchangeFormatter.cs
- FileRecordSequenceCompletedAsyncResult.cs
- ToolStripPanelRenderEventArgs.cs
- PageContent.cs
- AsymmetricKeyExchangeFormatter.cs
- SuppressMessageAttribute.cs
- CompiledXpathExpr.cs
- AQNBuilder.cs
- HttpProcessUtility.cs
- PropertyValueUIItem.cs
- SettingsPropertyWrongTypeException.cs
- EncryptedXml.cs
- XAMLParseException.cs
- EndOfStreamException.cs
- IisNotInstalledException.cs
- DataServiceOperationContext.cs
- CultureSpecificStringDictionary.cs
- TypeSystemProvider.cs
- ColorTranslator.cs
- EnumValidator.cs
- Menu.cs
- DesignOnlyAttribute.cs
- ChannelManagerHelpers.cs
- XmlSchemaCompilationSettings.cs
- SqlCacheDependencySection.cs
- MetafileHeader.cs
- DefaultDialogButtons.cs
- CodeCatchClauseCollection.cs
- InputMethodStateChangeEventArgs.cs
- DataGridViewEditingControlShowingEventArgs.cs
- DurationConverter.cs
- DllNotFoundException.cs
- OleDbParameterCollection.cs
- EventBindingService.cs
- BinaryMessageFormatter.cs
- ListenerAdapter.cs
- ClassData.cs
- XmlDataSource.cs
- ServiceCredentialsElement.cs
- DataBoundControl.cs
- TypeConverterMarkupExtension.cs
- ClrProviderManifest.cs
- Int32RectValueSerializer.cs
- RuntimeIdentifierPropertyAttribute.cs
- OletxCommittableTransaction.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- WindowsPrincipal.cs
- WindowsComboBox.cs
- Quaternion.cs
- ArrayItemReference.cs