Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Shapes / Polygon.cs / 1 / Polygon.cs
//---------------------------------------------------------------------------- // File: Polygon.cs // // Description: // Implementation of Polygon shape element. // // History: // 05/30/02 - AdSmith - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System; namespace System.Windows.Shapes { ////// The polygon shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// Since a Polygon is really a polyline which closes its path /// public sealed class Polygon : Shape { #region Constructors ////// Instantiates a new instance of a polygon. /// public Polygon() { } #endregion Constructors #region Dynamic Properties ////// Points property /// public static readonly DependencyProperty PointsProperty = DependencyProperty.Register( "Points", typeof(PointCollection), typeof(Polygon), new FrameworkPropertyMetadata(new FreezableDefaultValueFactory(PointCollection.Empty), FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender)); ////// Points property /// public PointCollection Points { get { return (PointCollection)GetValue(PointsProperty); } set { SetValue(PointsProperty, value); } } ////// FillRule property /// public static readonly DependencyProperty FillRuleProperty = DependencyProperty.Register( "FillRule", typeof(FillRule), typeof(Polygon), new FrameworkPropertyMetadata( FillRule.EvenOdd, FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsFillRuleValid) ); ////// FillRule property /// public FillRule FillRule { get { return (FillRule)GetValue(FillRuleProperty); } set { SetValue(FillRuleProperty, value); } } #endregion Dynamic Properties #region Protected Methods and properties ////// Get the polygon that defines this shape /// protected override Geometry DefiningGeometry { get { return _polygonGeometry; } } #endregion #region Internal Methods internal override void CacheDefiningGeometry() { PointCollection pointCollection = Points; PathFigure pathFigure = new PathFigure(); // Are we degenerate? // Yes, if we don't have data if (pointCollection == null) { _polygonGeometry = Geometry.Empty; return; } // Create the polygon PathGeometry // ISSUE-[....]-07/11/2003 - Bug 859068 // The constructor for PathFigure that takes a PointCollection is internal in the Core // so the below causes an A/V. Consider making it public. if (pointCollection.Count > 0) { pathFigure.StartPoint = pointCollection[0]; if (pointCollection.Count > 1) { Point[] array = new Point[pointCollection.Count - 1]; for (int i = 1; i < pointCollection.Count; i++) { array[i - 1] = pointCollection[i]; } pathFigure.Segments.Add(new PolyLineSegment(array, true)); } pathFigure.IsClosed = true; } PathGeometry polygonGeometry = new PathGeometry(); polygonGeometry.Figures.Add(pathFigure); // Set FillRule polygonGeometry.FillRule = FillRule; _polygonGeometry = polygonGeometry; } #endregion Internal Methods #region Private Methods and Members private Geometry _polygonGeometry; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // File: Polygon.cs // // Description: // Implementation of Polygon shape element. // // History: // 05/30/02 - AdSmith - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System; namespace System.Windows.Shapes { ////// The polygon shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// Since a Polygon is really a polyline which closes its path /// public sealed class Polygon : Shape { #region Constructors ////// Instantiates a new instance of a polygon. /// public Polygon() { } #endregion Constructors #region Dynamic Properties ////// Points property /// public static readonly DependencyProperty PointsProperty = DependencyProperty.Register( "Points", typeof(PointCollection), typeof(Polygon), new FrameworkPropertyMetadata(new FreezableDefaultValueFactory(PointCollection.Empty), FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender)); ////// Points property /// public PointCollection Points { get { return (PointCollection)GetValue(PointsProperty); } set { SetValue(PointsProperty, value); } } ////// FillRule property /// public static readonly DependencyProperty FillRuleProperty = DependencyProperty.Register( "FillRule", typeof(FillRule), typeof(Polygon), new FrameworkPropertyMetadata( FillRule.EvenOdd, FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsFillRuleValid) ); ////// FillRule property /// public FillRule FillRule { get { return (FillRule)GetValue(FillRuleProperty); } set { SetValue(FillRuleProperty, value); } } #endregion Dynamic Properties #region Protected Methods and properties ////// Get the polygon that defines this shape /// protected override Geometry DefiningGeometry { get { return _polygonGeometry; } } #endregion #region Internal Methods internal override void CacheDefiningGeometry() { PointCollection pointCollection = Points; PathFigure pathFigure = new PathFigure(); // Are we degenerate? // Yes, if we don't have data if (pointCollection == null) { _polygonGeometry = Geometry.Empty; return; } // Create the polygon PathGeometry // ISSUE-[....]-07/11/2003 - Bug 859068 // The constructor for PathFigure that takes a PointCollection is internal in the Core // so the below causes an A/V. Consider making it public. if (pointCollection.Count > 0) { pathFigure.StartPoint = pointCollection[0]; if (pointCollection.Count > 1) { Point[] array = new Point[pointCollection.Count - 1]; for (int i = 1; i < pointCollection.Count; i++) { array[i - 1] = pointCollection[i]; } pathFigure.Segments.Add(new PolyLineSegment(array, true)); } pathFigure.IsClosed = true; } PathGeometry polygonGeometry = new PathGeometry(); polygonGeometry.Figures.Add(pathFigure); // Set FillRule polygonGeometry.FillRule = FillRule; _polygonGeometry = polygonGeometry; } #endregion Internal Methods #region Private Methods and Members private Geometry _polygonGeometry; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
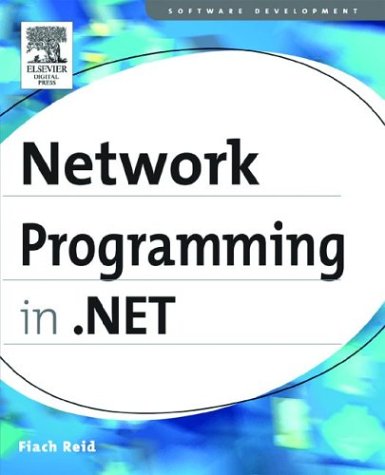
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ParseNumbers.cs
- HideDisabledControlAdapter.cs
- ListMarkerSourceInfo.cs
- AnnotationResourceCollection.cs
- InvariantComparer.cs
- ConfigurationFileMap.cs
- ErrorFormatter.cs
- ClientViaElement.cs
- RuleSetDialog.Designer.cs
- SizeLimitedCache.cs
- ILGen.cs
- RawStylusInput.cs
- WebHostScriptMappingsInstallComponent.cs
- Debugger.cs
- PeerApplication.cs
- BigInt.cs
- Math.cs
- Grant.cs
- Context.cs
- ContentTextAutomationPeer.cs
- XmlDataSourceNodeDescriptor.cs
- HttpListenerPrefixCollection.cs
- NavigationFailedEventArgs.cs
- _SslState.cs
- IntSecurity.cs
- MetadataPropertyCollection.cs
- PersonalizationDictionary.cs
- ProcessModuleCollection.cs
- updatecommandorderer.cs
- SQLInt16.cs
- BindingMAnagerBase.cs
- ObjectParameterCollection.cs
- PropertyReferenceExtension.cs
- DependencySource.cs
- SqlDataSourceStatusEventArgs.cs
- ObjectDataSourceMethodEditor.cs
- DeploymentExceptionMapper.cs
- ServiceReference.cs
- OdbcConnectionOpen.cs
- Section.cs
- XmlAttributes.cs
- MasterPage.cs
- SqlDependency.cs
- CodeEntryPointMethod.cs
- SaveFileDialog.cs
- SystemIPAddressInformation.cs
- ScaleTransform.cs
- FormConverter.cs
- ActivityTrace.cs
- SqlHelper.cs
- BadImageFormatException.cs
- FileChangeNotifier.cs
- Msec.cs
- ConfigXmlElement.cs
- ConfigUtil.cs
- Switch.cs
- Scripts.cs
- ToolStripArrowRenderEventArgs.cs
- WebCategoryAttribute.cs
- VectorConverter.cs
- SafeCancelMibChangeNotify.cs
- BounceEase.cs
- CreatingCookieEventArgs.cs
- SessionPageStatePersister.cs
- EmptyReadOnlyDictionaryInternal.cs
- QilFunction.cs
- IChannel.cs
- BufferedStream.cs
- DateTimeConstantAttribute.cs
- PointIndependentAnimationStorage.cs
- RuleAttributes.cs
- SelectionRange.cs
- SpeakInfo.cs
- DocumentOrderComparer.cs
- ResourcesChangeInfo.cs
- QueryContinueDragEventArgs.cs
- Quad.cs
- PrintSchema.cs
- Emitter.cs
- SmiGettersStream.cs
- DataSourceHelper.cs
- XmlIncludeAttribute.cs
- CatalogZoneBase.cs
- PreservationFileReader.cs
- PtsCache.cs
- HtmlDocument.cs
- DataFormat.cs
- PropertyInformation.cs
- PackagingUtilities.cs
- GlyphCollection.cs
- ScalarOps.cs
- SafeFileMappingHandle.cs
- XappLauncher.cs
- FlowDocumentView.cs
- CodeDirectoryCompiler.cs
- SqlStream.cs
- NumberSubstitution.cs
- IDQuery.cs
- MetaTableHelper.cs
- Page.cs