Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Internal / Ink / Quad.cs / 1 / Quad.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink { ////// A helper structure used in StrokeNode and StrokeNodeOperation implementations /// to store endpoints of the quad connecting two nodes of a stroke. /// The vertices of a quad are supposed to be clockwise with points A and D located /// on the begin node and B and C on the end one. /// internal struct Quad { #region Statics private static Quad s_empty = new Quad(new Point(0, 0), new Point(0, 0), new Point(0, 0), new Point(0, 0)); #endregion #region API ///Returns the static object representing an empty (unitialized) quad internal static Quad Empty { get { return s_empty; } } ///Constructor internal Quad(Point a, Point b, Point c, Point d) { _A = a; _B = b; _C = c; _D = d; } ///The A vertex of the quad internal Point A { get { return _A; } set { _A = value; } } ///The B vertex of the quad internal Point B { get { return _B; } set { _B = value; } } ///The C vertex of the quad internal Point C { get { return _C; } set { _C = value; } } ///The D vertex of the quad internal Point D { get { return _D; } set { _D = value; } } // Returns quad's vertex by index where A is of the index 0, B - is 1, etc internal Point this[int index] { get { switch (index) { case 0: return _A; case 1: return _B; case 2: return _C; case 3: return _D; default: throw new IndexOutOfRangeException("index"); } } } ///Tells whether the quad is invalid (empty) internal bool IsEmpty { get { return (_A == _B) && (_C == _D); } } internal void GetPoints(ListpointBuffer) { pointBuffer.Add(_A); pointBuffer.Add(_B); pointBuffer.Add(_C); pointBuffer.Add(_D); } /// Returns the bounds of the quad internal Rect Bounds { get { return IsEmpty ? Rect.Empty : Rect.Union(new Rect(_A, _B), new Rect(_C, _D)); } } #endregion #region Fields private Point _A; private Point _B; private Point _C; private Point _D; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink { ////// A helper structure used in StrokeNode and StrokeNodeOperation implementations /// to store endpoints of the quad connecting two nodes of a stroke. /// The vertices of a quad are supposed to be clockwise with points A and D located /// on the begin node and B and C on the end one. /// internal struct Quad { #region Statics private static Quad s_empty = new Quad(new Point(0, 0), new Point(0, 0), new Point(0, 0), new Point(0, 0)); #endregion #region API ///Returns the static object representing an empty (unitialized) quad internal static Quad Empty { get { return s_empty; } } ///Constructor internal Quad(Point a, Point b, Point c, Point d) { _A = a; _B = b; _C = c; _D = d; } ///The A vertex of the quad internal Point A { get { return _A; } set { _A = value; } } ///The B vertex of the quad internal Point B { get { return _B; } set { _B = value; } } ///The C vertex of the quad internal Point C { get { return _C; } set { _C = value; } } ///The D vertex of the quad internal Point D { get { return _D; } set { _D = value; } } // Returns quad's vertex by index where A is of the index 0, B - is 1, etc internal Point this[int index] { get { switch (index) { case 0: return _A; case 1: return _B; case 2: return _C; case 3: return _D; default: throw new IndexOutOfRangeException("index"); } } } ///Tells whether the quad is invalid (empty) internal bool IsEmpty { get { return (_A == _B) && (_C == _D); } } internal void GetPoints(ListpointBuffer) { pointBuffer.Add(_A); pointBuffer.Add(_B); pointBuffer.Add(_C); pointBuffer.Add(_D); } /// Returns the bounds of the quad internal Rect Bounds { get { return IsEmpty ? Rect.Empty : Rect.Union(new Rect(_A, _B), new Rect(_C, _D)); } } #endregion #region Fields private Point _A; private Point _B; private Point _C; private Point _D; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
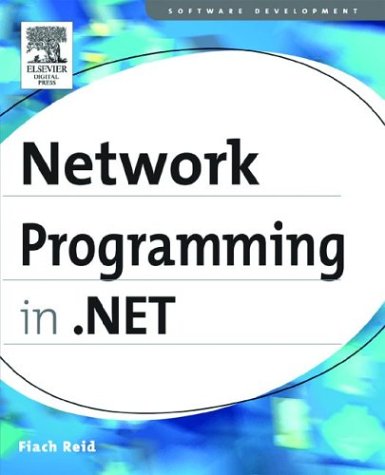
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BasicHttpMessageSecurityElement.cs
- VisualTreeHelper.cs
- PersonalizationEntry.cs
- CopyAttributesAction.cs
- Peer.cs
- ItemPager.cs
- ColorConvertedBitmap.cs
- AuthenticateEventArgs.cs
- BoundField.cs
- TextElement.cs
- IFlowDocumentViewer.cs
- altserialization.cs
- SafeEventLogWriteHandle.cs
- DataSysAttribute.cs
- XmlNodeReader.cs
- ObjectNavigationPropertyMapping.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- ResolvePPIDRequest.cs
- InitializationEventAttribute.cs
- TargetPerspective.cs
- MappingSource.cs
- ProfileGroupSettings.cs
- StringConverter.cs
- DesignerTextWriter.cs
- NestedContainer.cs
- Image.cs
- D3DImage.cs
- SortDescription.cs
- CounterSet.cs
- MarkupExtensionSerializer.cs
- UrlAuthFailedErrorFormatter.cs
- GridViewRow.cs
- PipelineComponent.cs
- RewritingProcessor.cs
- XamlTemplateSerializer.cs
- Msmq4PoisonHandler.cs
- Regex.cs
- basecomparevalidator.cs
- LoginUtil.cs
- PrintPageEvent.cs
- TextHidden.cs
- XPathNodePointer.cs
- PolyBezierSegment.cs
- OrderedDictionary.cs
- SupportsEventValidationAttribute.cs
- RelationshipEndCollection.cs
- WindowsPrincipal.cs
- TypedDataSourceCodeGenerator.cs
- MarshalByValueComponent.cs
- ReachFixedPageSerializer.cs
- ValueChangedEventManager.cs
- XmlStreamedByteStreamReader.cs
- TiffBitmapEncoder.cs
- MaterialCollection.cs
- Switch.cs
- Color.cs
- HttpRequestCacheValidator.cs
- SimpleTextLine.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- SafeWaitHandle.cs
- ListControl.cs
- TypeTypeConverter.cs
- ReferenceEqualityComparer.cs
- CollectionContainer.cs
- RequestCacheManager.cs
- FontStyles.cs
- RegexNode.cs
- DiscoveryServerProtocol.cs
- ServiceBehaviorAttribute.cs
- _DisconnectOverlappedAsyncResult.cs
- TextBoxLine.cs
- XmlName.cs
- CalculatedColumn.cs
- CodeSpit.cs
- AnnotationStore.cs
- CacheMemory.cs
- TdsParserStateObject.cs
- DataPagerFieldCommandEventArgs.cs
- ServerValidateEventArgs.cs
- ScriptMethodAttribute.cs
- TextContainerHelper.cs
- SurrogateSelector.cs
- RuntimeHelpers.cs
- UIPermission.cs
- WhitespaceRuleLookup.cs
- VisualStyleRenderer.cs
- DrawingContextWalker.cs
- RelatedView.cs
- ExecutionContext.cs
- SizeIndependentAnimationStorage.cs
- _NetworkingPerfCounters.cs
- Cursors.cs
- QuaternionAnimation.cs
- PkcsMisc.cs
- OutputBuffer.cs
- PeerService.cs
- EntityDataSourceContainerNameConverter.cs
- ToolStripOverflow.cs
- HttpBrowserCapabilitiesWrapper.cs
- InputScopeAttribute.cs