Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripOverflow.cs / 1 / ToolStripOverflow.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Collections; using System.ComponentModel; using System.Drawing; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Layout; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; ////// /// ToolStripOverflow /// [ComVisible(true)] [ClassInterface(ClassInterfaceType.AutoDispatch)] public class ToolStripOverflow : ToolStripDropDown, IArrangedElement { #if DEBUG internal static readonly TraceSwitch PopupLayoutDebug = new TraceSwitch("PopupLayoutDebug", "Debug ToolStripPopup Layout code"); #else internal static readonly TraceSwitch PopupLayoutDebug; #endif private ToolStripOverflowButton ownerItem; ///public ToolStripOverflow (ToolStripItem parentItem) : base(parentItem) { if (parentItem == null) { throw new ArgumentNullException("parentItem"); } ownerItem = parentItem as ToolStripOverflowButton; } /// protected internal override ToolStripItemCollection DisplayedItems { get { if (ParentToolStrip != null) { ToolStripItemCollection items = ParentToolStrip.OverflowItems; return items; } return new ToolStripItemCollection(null, false); } } public override ToolStripItemCollection Items { get { return new ToolStripItemCollection(null, /*ownedCollection=*/false, /*readonly=*/true); } } private ToolStrip ParentToolStrip { get { if (ownerItem != null) { return ownerItem.ParentToolStrip; } return null; } } /// /// ArrangedElementCollection IArrangedElement.Children { get { return DisplayedItems; } } /// /// IArrangedElement IArrangedElement.Container { get { return ParentInternal; } } /// /// bool IArrangedElement.ParticipatesInLayout { get { return GetState(STATE_VISIBLE); } } /// /// PropertyStore IArrangedElement.Properties { get { return Properties; } } /// /// void IArrangedElement.SetBounds(Rectangle bounds, BoundsSpecified specified) { SetBoundsCore(bounds.X, bounds.Y, bounds.Width, bounds.Height, specified); } /// /// /// Summary of CreateLayoutEngine. /// /// public override LayoutEngine LayoutEngine { get { return FlowLayout.Instance; } } protected override AccessibleObject CreateAccessibilityInstance() { return new ToolStripOverflowAccessibleObject(this); } [SuppressMessage("Microsoft.Security", "CA2119:SealMethodsThatSatisfyPrivateInterfaces")] public override Size GetPreferredSize(Size constrainingSize) { constrainingSize.Width = 200; return base.GetPreferredSize(constrainingSize); } protected override void OnLayout(LayoutEventArgs e) { if (ParentToolStrip != null && ParentToolStrip.IsInDesignMode) { if (FlowLayout.GetFlowDirection(this) != FlowDirection.TopDown) { FlowLayout.SetFlowDirection(this, FlowDirection.TopDown); } if (FlowLayout.GetWrapContents(this)) { FlowLayout.SetWrapContents(this, false); } } else { if (FlowLayout.GetFlowDirection(this) != FlowDirection.LeftToRight) { FlowLayout.SetFlowDirection(this, FlowDirection.LeftToRight); } if (!FlowLayout.GetWrapContents(this)) { FlowLayout.SetWrapContents(this, true); } } base.OnLayout(e); } ///protected override void SetDisplayedItems() { // do nothing here.... this is really for the setting the overflow/displayed items on the // main winbar. Our working item collection is our displayed item collection... calling // base would clear it out. Size biggestItemSize = Size.Empty; for (int j = 0; j < DisplayedItems.Count; j++) { ToolStripItem item = DisplayedItems[j]; if (((IArrangedElement)item).ParticipatesInLayout) { HasVisibleItems = true; biggestItemSize = LayoutUtils.UnionSizes(biggestItemSize, item.Bounds.Size); } } SetLargestItemSize(biggestItemSize); } private class ToolStripOverflowAccessibleObject : ToolStripAccessibleObject { public ToolStripOverflowAccessibleObject(ToolStripOverflow owner) : base(owner) { } public override AccessibleObject GetChild(int index) { return ((ToolStripOverflow)Owner).DisplayedItems[index].AccessibilityObject; } public override int GetChildCount() { return ((ToolStripOverflow)Owner).DisplayedItems.Count; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
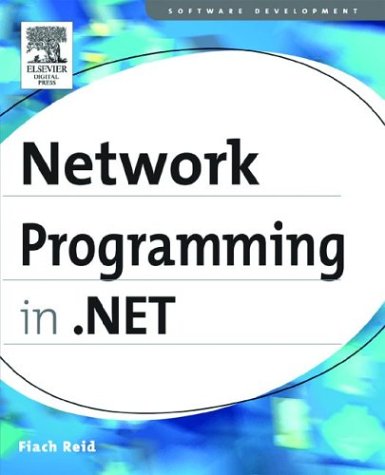
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QilFactory.cs
- SecurityRuntime.cs
- OdbcFactory.cs
- NodeInfo.cs
- SimpleRecyclingCache.cs
- Itemizer.cs
- XslAst.cs
- PriorityItem.cs
- TypeLoadException.cs
- FontSizeConverter.cs
- Container.cs
- BezierSegment.cs
- AgileSafeNativeMemoryHandle.cs
- XPathNodePointer.cs
- AssemblyBuilder.cs
- ZipPackagePart.cs
- Activator.cs
- BitmapDecoder.cs
- Literal.cs
- ImageSourceValueSerializer.cs
- IsolatedStorageFile.cs
- TableLayoutRowStyleCollection.cs
- PresentationSource.cs
- HostProtectionPermission.cs
- SerializationSectionGroup.cs
- CategoryEditor.cs
- TransactionContext.cs
- FlowDocumentFormatter.cs
- __ComObject.cs
- Documentation.cs
- MatrixCamera.cs
- FileDataSourceCache.cs
- RoutedEvent.cs
- ListBindingHelper.cs
- DynamicHyperLink.cs
- ResizeGrip.cs
- DataGridViewColumnHeaderCell.cs
- DBProviderConfigurationHandler.cs
- EventLogInternal.cs
- ApplicationTrust.cs
- ListView.cs
- MultiTrigger.cs
- DesignerVerb.cs
- EntityDataSourceContextCreatedEventArgs.cs
- KnownTypesHelper.cs
- ActivityStateQuery.cs
- NumberSubstitution.cs
- BindingMemberInfo.cs
- ResizeGrip.cs
- ImageBrush.cs
- ArraySubsetEnumerator.cs
- UrlPath.cs
- DataContractSet.cs
- PersonalizationStateQuery.cs
- Canvas.cs
- ClientSideQueueItem.cs
- DelegatingConfigHost.cs
- Table.cs
- DynamicPropertyHolder.cs
- InputScopeManager.cs
- MembershipValidatePasswordEventArgs.cs
- RepeatButton.cs
- ThemeInfoAttribute.cs
- DataTrigger.cs
- ChameleonKey.cs
- PaperSource.cs
- CompiledELinqQueryState.cs
- SevenBitStream.cs
- ErrorStyle.cs
- XmlLanguageConverter.cs
- ContentWrapperAttribute.cs
- CheckableControlBaseAdapter.cs
- TerminatorSinks.cs
- XmlUtil.cs
- ConfigurationManagerHelperFactory.cs
- SequenceFullException.cs
- GuidTagList.cs
- ScrollChrome.cs
- EtwTrackingBehaviorElement.cs
- TraceLevelStore.cs
- SymbolMethod.cs
- XmlCountingReader.cs
- HttpResponseHeader.cs
- TraceHandlerErrorFormatter.cs
- MissingMethodException.cs
- XmlIlGenerator.cs
- SystemResourceKey.cs
- HwndProxyElementProvider.cs
- Tracking.cs
- FileStream.cs
- XsltCompileContext.cs
- Int32.cs
- CLSCompliantAttribute.cs
- PersianCalendar.cs
- Switch.cs
- DataChangedEventManager.cs
- Pointer.cs
- TypeConstant.cs
- PageAsyncTaskManager.cs
- CommittableTransaction.cs