Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / RelationshipEndCollection.cs / 1 / RelationshipEndCollection.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Data; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// A collection of RelationshipEnds /// internal sealed class RelationshipEndCollection : IList{ private Dictionary _endLookup = null; private List _keysInDefOrder = null; /// /// construct a RelationshipEndCollection /// public RelationshipEndCollection() { } ////// How many RelationshipEnds are in the collection /// public int Count { get { return KeysInDefOrder.Count; } } ////// Add a relationship end /// /// the end to add public void Add(IRelationshipEnd end) { Debug.Assert(end != null, "end parameter is null"); SchemaElement endElement = end as SchemaElement; Debug.Assert(endElement != null, "end is not a SchemaElement"); // this should have been caught before this, just ignore it if ( !IsEndValid(end) ) return; if ( !ValidateUniqueName(endElement, end.Name)) return; EndLookup.Add(end.Name,end); KeysInDefOrder.Add(end.Name); } ////// See if an end can be added to the collection /// /// the end to add ///true if the end is valid, false otherwise private static bool IsEndValid(IRelationshipEnd end) { return !string.IsNullOrEmpty(end.Name); } ////// /// /// /// ///private bool ValidateUniqueName(SchemaElement end, string name) { if ( EndLookup.ContainsKey(name) ) { end.AddError( ErrorCode.AlreadyDefined, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.EndNameAlreadyDefinedDuplicate(name)); return false; } return true; } /// /// Remove a relationship end /// /// the end to remove ///true if item was in list public bool Remove(IRelationshipEnd end) { Debug.Assert(end != null, "end parameter is null"); if ( !IsEndValid(end) ) return false; KeysInDefOrder.Remove(end.Name); bool wasInList = EndLookup.Remove(end.Name); return wasInList; } ////// See if a relationship end is in the collection /// /// the name of the end ///true if the end name is in the collection public bool Contains(string name) { return EndLookup.ContainsKey(name); } ////// See if a relationship end is in the collection /// /// the name of the end ///true if the end is in the collection public bool Contains(IRelationshipEnd end) { Debug.Assert(end != null, "end parameter is null"); return Contains(end.Name); } public IRelationshipEnd this[int index] { get { return EndLookup[KeysInDefOrder[index]]; } set { throw EntityUtil.NotSupported(); } } ////// get a typed enumerator for the collection /// ///the enumerator public IEnumeratorGetEnumerator() { return new Enumerator(EndLookup,KeysInDefOrder); } public bool TryGetEnd( string name, out IRelationshipEnd end ) { return EndLookup.TryGetValue( name, out end ); } /// /// get an un-typed enumerator for the collection /// ///the enumerator IEnumerator System.Collections.IEnumerable.GetEnumerator() { return new Enumerator(EndLookup,KeysInDefOrder); } ////// The data for the collection /// private DictionaryEndLookup { get { if ( _endLookup == null ) _endLookup = new Dictionary (StringComparer.Ordinal); return _endLookup; } } /// /// the definition order collection /// private ListKeysInDefOrder { get { if ( _keysInDefOrder == null ) _keysInDefOrder = new List (); return _keysInDefOrder; } } /// /// remove all elements from the collection /// public void Clear() { EndLookup.Clear(); KeysInDefOrder.Clear(); } ////// can the collection be modified /// public bool IsReadOnly { get { return false; } } ////// Not supported /// /// the end ///nothing int IList.IndexOf(IRelationshipEnd end) { throw EntityUtil.NotSupported(); } /// /// Not supported /// /// the index /// the end void IList.Insert(int index, IRelationshipEnd end) { throw EntityUtil.NotSupported(); } /// /// Not supported /// /// the index void IList.RemoveAt(int index) { throw EntityUtil.NotSupported(); } /// /// copy all elements to an array /// /// array to copy to /// The zero-based index in array at which copying begins. public void CopyTo(IRelationshipEnd[] ends, int index ) { Debug.Assert(ends.Length-index >= Count); foreach ( IRelationshipEnd end in this ) ends[index++] = end; } ////// enumerator for the RelationshipEnd collection /// the ends as traversed in the order in which they were added /// private sealed class Enumerator : IEnumerator{ private List .Enumerator _Enumerator; private Dictionary _Data = null; /// /// construct the enumerator /// /// the real data /// the keys to the real data in inserted order public Enumerator(Dictionarydata, List keysInDefOrder) { Debug.Assert(data != null); Debug.Assert(keysInDefOrder != null); _Enumerator = keysInDefOrder.GetEnumerator(); _Data = data; } /// /// reset the enumerator /// public void Reset() { // reset is implemented explicitly ((IEnumerator)_Enumerator).Reset(); } ////// get current relationship end from the enumerator /// public IRelationshipEnd Current { get { return _Data[_Enumerator.Current]; } } ////// get current relationship end from the enumerator /// object System.Collections.IEnumerator.Current { get { return _Data[_Enumerator.Current]; } } ////// move to the next element in the collection /// ///true if there is a next, false if not public bool MoveNext() { return _Enumerator.MoveNext(); } ////// dispose of the enumerator /// public void Dispose() { } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Data; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// A collection of RelationshipEnds /// internal sealed class RelationshipEndCollection : IList{ private Dictionary _endLookup = null; private List _keysInDefOrder = null; /// /// construct a RelationshipEndCollection /// public RelationshipEndCollection() { } ////// How many RelationshipEnds are in the collection /// public int Count { get { return KeysInDefOrder.Count; } } ////// Add a relationship end /// /// the end to add public void Add(IRelationshipEnd end) { Debug.Assert(end != null, "end parameter is null"); SchemaElement endElement = end as SchemaElement; Debug.Assert(endElement != null, "end is not a SchemaElement"); // this should have been caught before this, just ignore it if ( !IsEndValid(end) ) return; if ( !ValidateUniqueName(endElement, end.Name)) return; EndLookup.Add(end.Name,end); KeysInDefOrder.Add(end.Name); } ////// See if an end can be added to the collection /// /// the end to add ///true if the end is valid, false otherwise private static bool IsEndValid(IRelationshipEnd end) { return !string.IsNullOrEmpty(end.Name); } ////// /// /// /// ///private bool ValidateUniqueName(SchemaElement end, string name) { if ( EndLookup.ContainsKey(name) ) { end.AddError( ErrorCode.AlreadyDefined, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.EndNameAlreadyDefinedDuplicate(name)); return false; } return true; } /// /// Remove a relationship end /// /// the end to remove ///true if item was in list public bool Remove(IRelationshipEnd end) { Debug.Assert(end != null, "end parameter is null"); if ( !IsEndValid(end) ) return false; KeysInDefOrder.Remove(end.Name); bool wasInList = EndLookup.Remove(end.Name); return wasInList; } ////// See if a relationship end is in the collection /// /// the name of the end ///true if the end name is in the collection public bool Contains(string name) { return EndLookup.ContainsKey(name); } ////// See if a relationship end is in the collection /// /// the name of the end ///true if the end is in the collection public bool Contains(IRelationshipEnd end) { Debug.Assert(end != null, "end parameter is null"); return Contains(end.Name); } public IRelationshipEnd this[int index] { get { return EndLookup[KeysInDefOrder[index]]; } set { throw EntityUtil.NotSupported(); } } ////// get a typed enumerator for the collection /// ///the enumerator public IEnumeratorGetEnumerator() { return new Enumerator(EndLookup,KeysInDefOrder); } public bool TryGetEnd( string name, out IRelationshipEnd end ) { return EndLookup.TryGetValue( name, out end ); } /// /// get an un-typed enumerator for the collection /// ///the enumerator IEnumerator System.Collections.IEnumerable.GetEnumerator() { return new Enumerator(EndLookup,KeysInDefOrder); } ////// The data for the collection /// private DictionaryEndLookup { get { if ( _endLookup == null ) _endLookup = new Dictionary (StringComparer.Ordinal); return _endLookup; } } /// /// the definition order collection /// private ListKeysInDefOrder { get { if ( _keysInDefOrder == null ) _keysInDefOrder = new List (); return _keysInDefOrder; } } /// /// remove all elements from the collection /// public void Clear() { EndLookup.Clear(); KeysInDefOrder.Clear(); } ////// can the collection be modified /// public bool IsReadOnly { get { return false; } } ////// Not supported /// /// the end ///nothing int IList.IndexOf(IRelationshipEnd end) { throw EntityUtil.NotSupported(); } /// /// Not supported /// /// the index /// the end void IList.Insert(int index, IRelationshipEnd end) { throw EntityUtil.NotSupported(); } /// /// Not supported /// /// the index void IList.RemoveAt(int index) { throw EntityUtil.NotSupported(); } /// /// copy all elements to an array /// /// array to copy to /// The zero-based index in array at which copying begins. public void CopyTo(IRelationshipEnd[] ends, int index ) { Debug.Assert(ends.Length-index >= Count); foreach ( IRelationshipEnd end in this ) ends[index++] = end; } ////// enumerator for the RelationshipEnd collection /// the ends as traversed in the order in which they were added /// private sealed class Enumerator : IEnumerator{ private List .Enumerator _Enumerator; private Dictionary _Data = null; /// /// construct the enumerator /// /// the real data /// the keys to the real data in inserted order public Enumerator(Dictionarydata, List keysInDefOrder) { Debug.Assert(data != null); Debug.Assert(keysInDefOrder != null); _Enumerator = keysInDefOrder.GetEnumerator(); _Data = data; } /// /// reset the enumerator /// public void Reset() { // reset is implemented explicitly ((IEnumerator)_Enumerator).Reset(); } ////// get current relationship end from the enumerator /// public IRelationshipEnd Current { get { return _Data[_Enumerator.Current]; } } ////// get current relationship end from the enumerator /// object System.Collections.IEnumerator.Current { get { return _Data[_Enumerator.Current]; } } ////// move to the next element in the collection /// ///true if there is a next, false if not public bool MoveNext() { return _Enumerator.MoveNext(); } ////// dispose of the enumerator /// public void Dispose() { } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
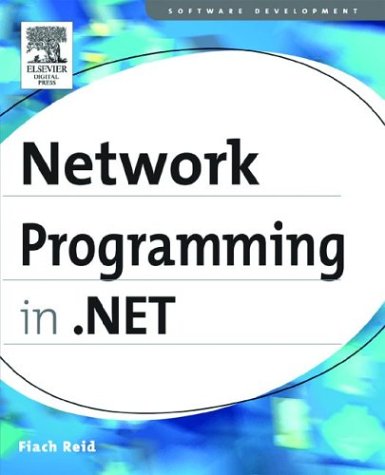
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SchemaNotation.cs
- TypefaceMetricsCache.cs
- ResourcesGenerator.cs
- HttpCookiesSection.cs
- FontNameEditor.cs
- Light.cs
- oledbmetadatacollectionnames.cs
- followingsibling.cs
- AttachedPropertyInfo.cs
- DataRelationCollection.cs
- JumpTask.cs
- ToolboxBitmapAttribute.cs
- DetailsViewCommandEventArgs.cs
- TextEditorLists.cs
- FileLogRecordHeader.cs
- DecoratedNameAttribute.cs
- FixedFindEngine.cs
- RenderContext.cs
- ClockGroup.cs
- _NetworkingPerfCounters.cs
- WindowShowOrOpenTracker.cs
- DataGridViewRowEventArgs.cs
- XmlObjectSerializer.cs
- CookielessHelper.cs
- DbReferenceCollection.cs
- InternalsVisibleToAttribute.cs
- ZoomingMessageFilter.cs
- UrlMappingsModule.cs
- ProtocolsConfigurationHandler.cs
- DummyDataSource.cs
- QilPatternFactory.cs
- TagPrefixInfo.cs
- GridViewRowCollection.cs
- MatrixAnimationUsingKeyFrames.cs
- Button.cs
- CanonicalFontFamilyReference.cs
- XmlIncludeAttribute.cs
- ChtmlPhoneCallAdapter.cs
- FileDialog_Vista.cs
- _FtpDataStream.cs
- InputGestureCollection.cs
- ProfessionalColorTable.cs
- CryptoConfig.cs
- OrderedDictionaryStateHelper.cs
- basevalidator.cs
- ChangeDirector.cs
- DataGridViewToolTip.cs
- SelectionHighlightInfo.cs
- SchemaTypeEmitter.cs
- XmlElementAttribute.cs
- MailDefinitionBodyFileNameEditor.cs
- SymbolMethod.cs
- ArrayListCollectionBase.cs
- CompiledXpathExpr.cs
- UIElement3D.cs
- ItemContainerGenerator.cs
- ObservableDictionary.cs
- DataGridViewDataConnection.cs
- UrlAuthorizationModule.cs
- Int16AnimationUsingKeyFrames.cs
- TextChangedEventArgs.cs
- AdPostCacheSubstitution.cs
- TemplateColumn.cs
- SessionEndingEventArgs.cs
- WinCategoryAttribute.cs
- __Filters.cs
- GAC.cs
- XamlGridLengthSerializer.cs
- ContextItemManager.cs
- PageThemeBuildProvider.cs
- InstanceKeyCollisionException.cs
- DebuggerAttributes.cs
- UnsafeNativeMethods.cs
- Formatter.cs
- CompilerGlobalScopeAttribute.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- HttpCapabilitiesEvaluator.cs
- _SecureChannel.cs
- StringReader.cs
- BamlLocalizableResourceKey.cs
- XmlReflectionMember.cs
- ArrayElementGridEntry.cs
- KeyGestureConverter.cs
- TypeUtils.cs
- TextTreeFixupNode.cs
- Closure.cs
- SafeFileMappingHandle.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- DataBindingHandlerAttribute.cs
- PointHitTestResult.cs
- DesignTimeSiteMapProvider.cs
- OleDbMetaDataFactory.cs
- WhitespaceRuleLookup.cs
- ProtectedConfiguration.cs
- TextEffectCollection.cs
- CopyAttributesAction.cs
- TypeConverterAttribute.cs
- CalendarDay.cs
- StatusBarItem.cs
- CalendarDesigner.cs