Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / IO / Packaging / CompoundFile / FormatVersion.cs / 1305600 / FormatVersion.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implementation of the FormatVersion class, which describes the versioning // of an individual "format feature" within a compound file. // // History: // 06/20/2002: [....]: Created // 05/30/2003: LGolding: Ported to WCP tree. // //----------------------------------------------------------------------------- // Allow use of presharp warning numbers [6506] and [6518] unknown to the compiler #pragma warning disable 1634, 1691 using System; using System.IO; #if PBTCOMPILER using MS.Utility; // For SR.cs #else using System.Windows; using MS.Internal.WindowsBase; // FriendAccessAllowed #endif namespace MS.Internal.IO.Packaging.CompoundFile { ///Class for manipulating version object #if !PBTCOMPILER [FriendAccessAllowed] #endif internal class FormatVersion { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- #region Constructors #if !PBTCOMPILER ////// Constructor for FormatVersion /// private FormatVersion() { } #endif ////// Constructor for FormatVersion with given featureId and version /// /// feature identifier /// version ///reader, updater, and writer versions are set to version public FormatVersion(string featureId, VersionPair version) : this(featureId, version, version, version) { } ////// Constructor for FormatVersion with given featureId and reader, updater, /// and writer version /// /// feature identifier /// Writer Version /// Reader Version /// Updater Version public FormatVersion(String featureId, VersionPair writerVersion, VersionPair readerVersion, VersionPair updaterVersion) { if (featureId == null) throw new ArgumentNullException("featureId"); if (writerVersion == null) throw new ArgumentNullException("writerVersion"); if (readerVersion == null) throw new ArgumentNullException("readerVersion"); if (updaterVersion == null) throw new ArgumentNullException("updaterVersion"); if (featureId.Length == 0) { throw new ArgumentException(SR.Get(SRID.ZeroLengthFeatureID)); } _featureIdentifier = featureId; _reader = readerVersion; _updater = updaterVersion; _writer = writerVersion; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties #if !PBTCOMPILER ////// reader version /// public VersionPair ReaderVersion { get { return _reader; } set { if (value == null) { throw new ArgumentNullException("value"); } _reader = value; } } ////// writer version /// public VersionPair WriterVersion { get { return _writer; } set { if (value == null) { throw new ArgumentNullException("value"); } _writer = value; } } ////// updater version /// public VersionPair UpdaterVersion { get { return _updater; } set { if (value == null) { throw new ArgumentNullException("value"); } _updater = value; } } ////// feature identifier /// public String FeatureIdentifier { get { return _featureIdentifier; } } #endif #endregion Public Properties #region Operators #if false ////// == comparison operator /// /// version to be compared /// version to be compared public static bool operator ==(FormatVersion v1, FormatVersion v2) { // We have to cast v1 and v2 to Object // to ensure that the == operator on Ojbect class is used not the == operator on FormatVersion if ((Object) v1 == null || (Object) v2 == null) { return ((Object) v1 == null && (Object) v2 == null); } // Do comparison only if both v1 and v2 are not null return v1.Equals(v2); } ////// != comparison operator /// /// version to be compared /// version to be compared public static bool operator !=(FormatVersion v1, FormatVersion v2) { return !(v1 == v2); } ////// Eaual comparison operator /// /// Object to compare ///ture if the object is equal to this instance public override bool Equals(Object obj) { if (obj == null) { return false; } if (obj.GetType() != typeof(FormatVersion)) { return false; } FormatVersion v = (FormatVersion) obj; //PRESHARP:Parameter to this public method must be validated: A null-dereference can occur here. // Parameter 'v' to this public method must be validated: A null-dereference can occur here. //This is a false positive as the checks above can gurantee no null dereference will occur #pragma warning disable 6506 if (String.CompareOrdinal(_featureIdentifier.ToUpperInvariant(), v.FeatureIdentifier.ToUpperInvariant()) != 0 || _reader != v.ReaderVersion || _writer != v.WriterVersion || _updater != v.UpdaterVersion) { return false; } #pragma warning restore 6506 return true; } ////// Hash code /// public override int GetHashCode() { int hash = _reader.Major & HashMask; hash <<= 5; hash |= (_reader.Minor & HashMask); hash <<= 5; hash |= (_updater.Major & HashMask); hash <<= 5; hash |= (_updater.Minor & HashMask); hash <<= 5; hash |= (_writer.Major & HashMask); hash <<= 5; hash |= (_writer.Minor & HashMask); return hash; } #endif #endregion Operators //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods #if !PBTCOMPILER ////// Constructor for FormatVersion with information read from the given stream /// /// Stream where version information is read from ///Before this function call the current position of stream should be /// pointing to the begining of the version structure. After this call the current /// poisition will be pointing immediately after the version structure public static FormatVersion LoadFromStream(Stream stream) { int bytesRead; return LoadFromStream(stream, out bytesRead); } #endif ////// Persist format version to the given stream /// /// the stream to be written ////// This operation will change the stream pointer /// stream can be null and will still return the number of bytes to be written /// public int SaveToStream(Stream stream) { checked { // Suppress 56518 Local IDisposable object not disposed: // Reason: The stream is not owned by the BlockManager, therefore we can // close the BinaryWriter as it will Close the stream underneath. #pragma warning disable 6518 int len = 0; BinaryWriter binarywriter = null; #pragma warning restore 6518 if (stream != null) { binarywriter = new BinaryWriter(stream, System.Text.Encoding.Unicode); } // ************ // feature ID // ************ len += ContainerUtilities.WriteByteLengthPrefixedDWordPaddedUnicodeString(binarywriter, _featureIdentifier); // **************** // Reader Version // **************** if (stream != null) { binarywriter.Write(_reader.Major); // Major number binarywriter.Write(_reader.Minor); // Minor number } len += ContainerUtilities.Int16Size; len += ContainerUtilities.Int16Size; // ***************** // Updater Version // ***************** if (stream != null) { binarywriter.Write(_updater.Major); // Major number binarywriter.Write(_updater.Minor); // Minor number } len += ContainerUtilities.Int16Size; len += ContainerUtilities.Int16Size; // **************** // Writer Version // **************** if (stream != null) { binarywriter.Write(_writer.Major); // Major number binarywriter.Write(_writer.Minor); // Minor number } len += ContainerUtilities.Int16Size; len += ContainerUtilities.Int16Size; return len; } } #if !PBTCOMPILER ////// Check if a component with the given version can read this format version safely /// /// version of a component ///true if this format version can be read safely by the component /// with the given version, otherwise false ////// The given version is checked against ReaderVersion /// public bool IsReadableBy(VersionPair version) { if (version == null) { throw new ArgumentNullException("version"); } return (_reader <= version); } ////// Check if a component with the given version can update this format version safely /// /// version of a component ///true if this format version can be updated safely by the component /// with the given version, otherwise false ////// The given version is checked against UpdaterVersion /// public bool IsUpdatableBy(VersionPair version) { if (version == null) { throw new ArgumentNullException("version"); } return (_updater <= version); } #endif #endregion Public Methods //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- // None //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #if !PBTCOMPILER ////// Constructor for FormatVersion with information read from the given BinaryReader /// /// BinaryReader where version information is read from /// number of bytes read including padding ///FormatVersion object ////// This operation will change the stream pointer. This function is preferred over the /// LoadFromStream as it doesn't leave around Undisposed BinaryReader, which /// LoadFromStream will /// private static FormatVersion LoadFromBinaryReader(BinaryReader reader, out Int32 bytesRead) { checked { if (reader == null) { throw new ArgumentNullException("reader"); } FormatVersion ver = new FormatVersion(); bytesRead = 0; // Initialize the number of bytes read // ************** // feature ID // ************** Int32 strBytes; ver._featureIdentifier = ContainerUtilities.ReadByteLengthPrefixedDWordPaddedUnicodeString(reader, out strBytes); bytesRead += strBytes; Int16 major; Int16 minor; // **************** // Reader Version // **************** major = reader.ReadInt16(); // Major number bytesRead += ContainerUtilities.Int16Size; minor = reader.ReadInt16(); // Minor number bytesRead += ContainerUtilities.Int16Size; ver.ReaderVersion = new VersionPair(major, minor); // ***************** // Updater Version // ***************** major = reader.ReadInt16(); // Major number bytesRead += ContainerUtilities.Int16Size; minor = reader.ReadInt16(); // Minor number bytesRead += ContainerUtilities.Int16Size; ver.UpdaterVersion = new VersionPair(major, minor); // **************** // Writer Version // **************** major = reader.ReadInt16(); // Major number bytesRead += ContainerUtilities.Int16Size; minor = reader.ReadInt16(); // Minor number bytesRead += ContainerUtilities.Int16Size; ver.WriterVersion = new VersionPair(major, minor); return ver; } } ////// Create FormatVersion object and read version information from the given stream /// /// the stream to read version information from /// number of bytes read including padding ///FormatVersion object ////// This operation will change the stream pointer. This function shouldn't be /// used in the scenarios when LoadFromBinaryReader can do the job. /// LoadFromBinaryReader will not leave around any undisposed objects, /// and LoadFromStream will. /// internal static FormatVersion LoadFromStream(Stream stream, out Int32 bytesRead) { if (stream == null) { throw new ArgumentNullException("stream"); } // Suppress 56518 Local IDisposable object not disposed: // Reason: The stream is not owned by the BlockManager, therefore we can // close the BinaryWriter as it will Close the stream underneath. #pragma warning disable 6518 BinaryReader streamReader = new BinaryReader(stream, System.Text.Encoding.Unicode); #pragma warning restore 6518 return LoadFromBinaryReader(streamReader, out bytesRead); } #endif //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- // None //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Member Variables private VersionPair _reader; private VersionPair _updater; private VersionPair _writer; private String _featureIdentifier; #if false static private readonly int HashMask = 0x1f; #endif #endregion Member Variables } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
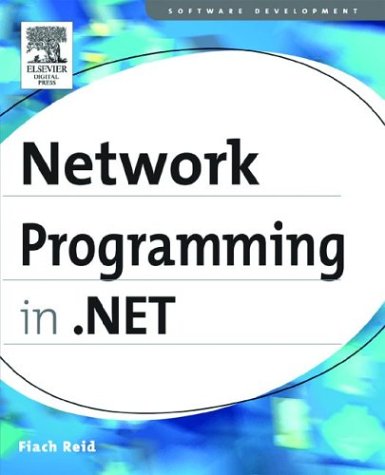
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CleanUpVirtualizedItemEventArgs.cs
- ObjectDataSourceSelectingEventArgs.cs
- XMLDiffLoader.cs
- InternalPolicyElement.cs
- CookielessHelper.cs
- FontFamilyConverter.cs
- SystemTcpConnection.cs
- XmlComplianceUtil.cs
- ImageConverter.cs
- TimeSpanFormat.cs
- JournalEntryListConverter.cs
- X509CertificateStore.cs
- EDesignUtil.cs
- ManagementDateTime.cs
- MSG.cs
- RemotingConfiguration.cs
- BooleanExpr.cs
- TemplateParser.cs
- Variant.cs
- Asn1IntegerConverter.cs
- infer.cs
- BinHexDecoder.cs
- SingleConverter.cs
- DispatcherExceptionFilterEventArgs.cs
- ProjectionCamera.cs
- ContainerUtilities.cs
- Types.cs
- StateItem.cs
- MailSettingsSection.cs
- XmlQualifiedName.cs
- DataGridTableCollection.cs
- BitmapPalettes.cs
- OptimalBreakSession.cs
- DiscoveryExceptionDictionary.cs
- InternalConfigHost.cs
- loginstatus.cs
- DriveInfo.cs
- BeginStoryboard.cs
- TextEditorContextMenu.cs
- MethodImplAttribute.cs
- EntitySet.cs
- HMACSHA1.cs
- CodeGeneratorOptions.cs
- TCPListener.cs
- ScriptManager.cs
- TableLayoutSettingsTypeConverter.cs
- StdValidatorsAndConverters.cs
- X509Extension.cs
- AVElementHelper.cs
- input.cs
- Vector3D.cs
- ObjectStateEntryDbDataRecord.cs
- util.cs
- UIElementPropertyUndoUnit.cs
- DateTimeConstantAttribute.cs
- CdpEqualityComparer.cs
- TreeWalkHelper.cs
- XmlSchemaParticle.cs
- TaiwanCalendar.cs
- ParseNumbers.cs
- RowParagraph.cs
- TdsValueSetter.cs
- PropertyMetadata.cs
- SqlTriggerContext.cs
- SimpleWebHandlerParser.cs
- WSHttpBindingCollectionElement.cs
- SchemaAttDef.cs
- HelloOperation11AsyncResult.cs
- RtfToken.cs
- DragDrop.cs
- Exception.cs
- TextReader.cs
- InitializingNewItemEventArgs.cs
- QueueProcessor.cs
- SplitterEvent.cs
- WpfMemberInvoker.cs
- CacheEntry.cs
- PackageDigitalSignature.cs
- ResourceExpressionBuilder.cs
- metadatamappinghashervisitor.cs
- XmlSchema.cs
- MsmqMessageProperty.cs
- ImageCollectionEditor.cs
- PropertyInformationCollection.cs
- Content.cs
- BrowserTree.cs
- DefaultValueConverter.cs
- StorageTypeMapping.cs
- ModelUIElement3D.cs
- SyntaxCheck.cs
- CheckBoxRenderer.cs
- Convert.cs
- PageCache.cs
- ArraySegment.cs
- AppDomainShutdownMonitor.cs
- ReachDocumentReferenceCollectionSerializerAsync.cs
- InkSerializer.cs
- TextElementCollection.cs
- AutomationIdentifier.cs
- Button.cs