Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Toolbox / ToolboxItemLoader.cs / 1305376 / ToolboxItemLoader.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.Activities.Presentation.Toolbox { using System; using System.Collections.Generic; using System.IO; using System.Globalization; // This is helper class which tries to load and create tool items from flat text file. // The content of a file must contain following, colon separated values // 1.) fully qualified class name, which is flagged with ToolboxItemAttribute // 2.) name of the assembly (with extension) where the tool class is located // 3.) an optional bitmap file (in case when activity is not marked with toolboxitem attribute) // 4.) an optional display name // // Positions 3 & 4 can be in mixed order. the loader checks for known graphic extension // // The category information is defined by string content placed within square brackets [ ]. // All items under category definition fall into that category unless new category is defined. // If no category is defined, default one is created // // All lines starting with ';' or '#' char are treaded as comments and skipped sealed class ToolboxItemLoader { static readonly string FormatExceptionText = "Tool has to be defined either as 'name, assembly', or 'name, assembly, bitmap'"; static readonly string DefaultCategory = "default"; private ToolboxItemLoader() { } public static ToolboxItemLoader GetInstance() { return new ToolboxItemLoader(); } public void LoadToolboxItems(string fileName, ToolboxCategoryItems container, bool resetContainer) { if (string.IsNullOrEmpty(fileName)) { throw FxTrace.Exception.AsError(new ArgumentNullException("fileName")); } if (null == container) { throw FxTrace.Exception.AsError(new ArgumentNullException("container")); } if (resetContainer) { container.Clear(); } using (StreamReader reader = File.OpenText(fileName)) { string entry = null; ToolboxCategory category = null; while (null != (entry = reader.ReadLine())) { entry = entry.Trim(); if (entry.Length > 1 && (entry[0] != ';' && entry[0] != '#')) { if (entry.StartsWith("[", StringComparison.CurrentCulture) && entry.EndsWith("]", StringComparison.CurrentCulture)) { string categoryName = entry.Substring(1, entry.Length - 2); category = GetCategoryItem(container, categoryName); } else { if (null == category) { category = GetCategoryItem(container, DefaultCategory); } string[] toolDefinition = entry.Split(';'); string toolName = null; string assembly = null; string displayName = null; string bitmap = null; if (GetToolAssemblyAndName(toolDefinition, ref toolName, ref assembly)) { GetBitmap(toolDefinition, ref bitmap); GetDisplayName(toolDefinition, ref displayName); category.Add(new ToolboxItemWrapper(toolName, assembly, bitmap, displayName)); } else { throw FxTrace.Exception.AsError(new ArgumentOutOfRangeException(FormatExceptionText)); } } } } } } bool GetToolAssemblyAndName(string[] toolDefinition, ref string toolName, ref string assembly) { if (toolDefinition.Length >= 2) { toolName = toolDefinition[0].Trim(); assembly = toolDefinition[1].Trim(); return true; } return false; } bool GetBitmap(string[] toolDefinition, ref string bitmap) { for (int i = 2; i < toolDefinition.Length; ++i) { string current = toolDefinition[i].Trim(); if (current.EndsWith(".bmp", StringComparison.OrdinalIgnoreCase) || current.EndsWith(".jpg", StringComparison.OrdinalIgnoreCase) || current.EndsWith(".gif", StringComparison.OrdinalIgnoreCase) || current.EndsWith(".png", StringComparison.OrdinalIgnoreCase) || current.EndsWith(".tiff", StringComparison.OrdinalIgnoreCase) || current.EndsWith(".jpeg", StringComparison.OrdinalIgnoreCase) || current.EndsWith(".exig", StringComparison.OrdinalIgnoreCase)) { bitmap = current; return true; } } return false; } bool GetDisplayName(string[] toolDefinition, ref string displayName) { for (int i = 2; i < toolDefinition.Length; ++i) { string current = toolDefinition[i].Trim(); if (!current.EndsWith(".bmp", StringComparison.OrdinalIgnoreCase) && !current.EndsWith(".jpg", StringComparison.OrdinalIgnoreCase) && !current.EndsWith(".gif", StringComparison.OrdinalIgnoreCase) && !current.EndsWith(".png", StringComparison.OrdinalIgnoreCase) && !current.EndsWith(".tiff", StringComparison.OrdinalIgnoreCase) && !current.EndsWith(".jpeg", StringComparison.OrdinalIgnoreCase) && !current.EndsWith(".exig", StringComparison.OrdinalIgnoreCase)) { displayName = current; return true; } } return false; } ToolboxCategory GetCategoryItem(ToolboxCategoryItems container, string categoryName) { foreach (ToolboxCategory category in container) { if (0 == string.Compare(category.CategoryName, categoryName, true, CultureInfo.CurrentUICulture)) { return category; } } ToolboxCategory newCategory = new ToolboxCategory(categoryName); container.Add(newCategory); return newCategory; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
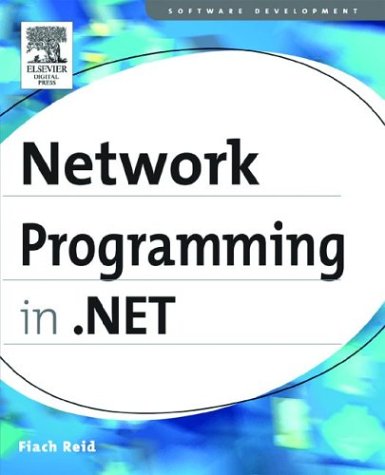
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HasCopySemanticsAttribute.cs
- CanonicalXml.cs
- VectorCollection.cs
- Activation.cs
- OutputWindow.cs
- NameService.cs
- AspCompat.cs
- EventLogger.cs
- TreeWalker.cs
- GCHandleCookieTable.cs
- MetabaseServerConfig.cs
- AsymmetricKeyExchangeFormatter.cs
- WhitespaceSignificantCollectionAttribute.cs
- MediaElementAutomationPeer.cs
- SourceItem.cs
- ScheduleChanges.cs
- AlphabetConverter.cs
- FixedPageAutomationPeer.cs
- SearchForVirtualItemEventArgs.cs
- XsdBuilder.cs
- ExpressionStringBuilder.cs
- KeyValuePair.cs
- HelpProvider.cs
- SystemResourceHost.cs
- HtmlInputRadioButton.cs
- SiteMapDataSourceView.cs
- ShimAsPublicXamlType.cs
- TextContainer.cs
- TimeStampChecker.cs
- GridViewColumnHeaderAutomationPeer.cs
- SecurityKeyIdentifierClause.cs
- AvTraceDetails.cs
- DataServiceEntityAttribute.cs
- TraceRecord.cs
- WeakReferenceEnumerator.cs
- Closure.cs
- SqlVisitor.cs
- GlobalEventManager.cs
- RoutedEventArgs.cs
- ProtocolsConfiguration.cs
- SchemaConstraints.cs
- ProxyAttribute.cs
- Utils.cs
- ConnectionPoint.cs
- COM2Properties.cs
- Adorner.cs
- LineGeometry.cs
- RuleSetDialog.cs
- DesignerTransactionCloseEvent.cs
- XmlChoiceIdentifierAttribute.cs
- WsdlInspector.cs
- XmlChoiceIdentifierAttribute.cs
- WmlListAdapter.cs
- AsyncPostBackErrorEventArgs.cs
- HostingEnvironment.cs
- MSAAEventDispatcher.cs
- ComplexObject.cs
- MailMessageEventArgs.cs
- CodeDelegateInvokeExpression.cs
- SystemIcmpV6Statistics.cs
- DynamicResourceExtension.cs
- ParameterCollectionEditor.cs
- SizeFConverter.cs
- JumpTask.cs
- FieldAccessException.cs
- DetailsViewPagerRow.cs
- ContentFileHelper.cs
- ControlBindingsCollection.cs
- MatrixAnimationUsingKeyFrames.cs
- CustomTypeDescriptor.cs
- CSharpCodeProvider.cs
- PropertyDescriptorGridEntry.cs
- Encoder.cs
- ProxyGenerationError.cs
- DataContractSet.cs
- CustomBindingCollectionElement.cs
- Trace.cs
- ModelService.cs
- RuleDefinitions.cs
- ItemsControl.cs
- ErrorProvider.cs
- XmlArrayItemAttribute.cs
- ItemsPresenter.cs
- ArraySegment.cs
- Point.cs
- TextReader.cs
- WebPartConnectionsCloseVerb.cs
- SqlCachedBuffer.cs
- PathFigure.cs
- serverconfig.cs
- ClientTargetSection.cs
- AsyncCompletedEventArgs.cs
- FocusTracker.cs
- ConvertersCollection.cs
- AssemblyCollection.cs
- DataGridViewCellStateChangedEventArgs.cs
- ReadOnlyCollectionBase.cs
- JapaneseCalendar.cs
- LinqDataView.cs
- PeerEndPoint.cs