Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewSelectedColumnCollection.cs / 1 / DataGridViewSelectedColumnCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Collections; using System.Windows.Forms; using System.ComponentModel; using System.Globalization; using System.Diagnostics.CodeAnalysis; ///[ ListBindable(false), SuppressMessage("Microsoft.Design", "CA1010:CollectionsShouldImplementGenericInterface") // Consider adding an IList implementation ] public class DataGridViewSelectedColumnCollection : BaseCollection, IList { ArrayList items = new ArrayList(); /// /// int IList.Add(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.Contains(object value) { return this.items.Contains(value); } /// /// int IList.IndexOf(object value) { return this.items.IndexOf(value); } /// /// void IList.Insert(int index, object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Remove(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.RemoveAt(int index) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.IsFixedSize { get { return true; } } /// /// bool IList.IsReadOnly { get { return true; } } /// /// object IList.this[int index] { get { return this.items[index]; } set { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } /// /// void ICollection.CopyTo(Array array, int index) { this.items.CopyTo(array, index); } /// /// int ICollection.Count { get { return this.items.Count; } } /// /// bool ICollection.IsSynchronized { get { return false; } } /// /// object ICollection.SyncRoot { get { return this; } } /// /// IEnumerator IEnumerable.GetEnumerator() { return this.items.GetEnumerator(); } internal DataGridViewSelectedColumnCollection() { } /// protected override ArrayList List { get { return this.items; } } /// public DataGridViewColumn this[int index] { get { return (DataGridViewColumn) this.items[index]; } } /// /// /// internal int Add(DataGridViewColumn dataGridViewColumn) { return this.items.Add(dataGridViewColumn); } /* Unused at this point internal void AddRange(DataGridViewColumn[] dataGridViewColumns) { Debug.Assert(dataGridViewColumns != null); foreach(DataGridViewColumn dataGridViewColumn in dataGridViewColumns) { this.items.Add(dataGridViewColumn); } } */ /* Unused at this point internal void AddColumnCollection(DataGridViewColumnCollection dataGridViewColumns) { Debug.Assert(dataGridViewColumns != null); foreach(DataGridViewColumn dataGridViewColumn in dataGridViewColumns) { this.items.Add(dataGridViewColumn); } } */ ///Adds a ///to this collection. [ EditorBrowsable(EditorBrowsableState.Never) ] public void Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// /// Checks to see if a DataGridViewCell is contained in this collection. /// public bool Contains(DataGridViewColumn dataGridViewColumn) { return this.items.IndexOf(dataGridViewColumn) != -1; } ///public void CopyTo(DataGridViewColumn[] array, int index) { this.items.CopyTo(array, index); } /// [ EditorBrowsable(EditorBrowsableState.Never), SuppressMessage("Microsoft.Performance", "CA1801:AvoidUnusedParameters") ] public void Insert(int index, DataGridViewColumn dataGridViewColumn) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Collections; using System.Windows.Forms; using System.ComponentModel; using System.Globalization; using System.Diagnostics.CodeAnalysis; ///[ ListBindable(false), SuppressMessage("Microsoft.Design", "CA1010:CollectionsShouldImplementGenericInterface") // Consider adding an IList implementation ] public class DataGridViewSelectedColumnCollection : BaseCollection, IList { ArrayList items = new ArrayList(); /// /// int IList.Add(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.Contains(object value) { return this.items.Contains(value); } /// /// int IList.IndexOf(object value) { return this.items.IndexOf(value); } /// /// void IList.Insert(int index, object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Remove(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.RemoveAt(int index) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.IsFixedSize { get { return true; } } /// /// bool IList.IsReadOnly { get { return true; } } /// /// object IList.this[int index] { get { return this.items[index]; } set { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } /// /// void ICollection.CopyTo(Array array, int index) { this.items.CopyTo(array, index); } /// /// int ICollection.Count { get { return this.items.Count; } } /// /// bool ICollection.IsSynchronized { get { return false; } } /// /// object ICollection.SyncRoot { get { return this; } } /// /// IEnumerator IEnumerable.GetEnumerator() { return this.items.GetEnumerator(); } internal DataGridViewSelectedColumnCollection() { } /// protected override ArrayList List { get { return this.items; } } /// public DataGridViewColumn this[int index] { get { return (DataGridViewColumn) this.items[index]; } } /// /// /// internal int Add(DataGridViewColumn dataGridViewColumn) { return this.items.Add(dataGridViewColumn); } /* Unused at this point internal void AddRange(DataGridViewColumn[] dataGridViewColumns) { Debug.Assert(dataGridViewColumns != null); foreach(DataGridViewColumn dataGridViewColumn in dataGridViewColumns) { this.items.Add(dataGridViewColumn); } } */ /* Unused at this point internal void AddColumnCollection(DataGridViewColumnCollection dataGridViewColumns) { Debug.Assert(dataGridViewColumns != null); foreach(DataGridViewColumn dataGridViewColumn in dataGridViewColumns) { this.items.Add(dataGridViewColumn); } } */ ///Adds a ///to this collection. [ EditorBrowsable(EditorBrowsableState.Never) ] public void Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// /// Checks to see if a DataGridViewCell is contained in this collection. /// public bool Contains(DataGridViewColumn dataGridViewColumn) { return this.items.IndexOf(dataGridViewColumn) != -1; } ///public void CopyTo(DataGridViewColumn[] array, int index) { this.items.CopyTo(array, index); } /// [ EditorBrowsable(EditorBrowsableState.Never), SuppressMessage("Microsoft.Performance", "CA1801:AvoidUnusedParameters") ] public void Insert(int index, DataGridViewColumn dataGridViewColumn) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
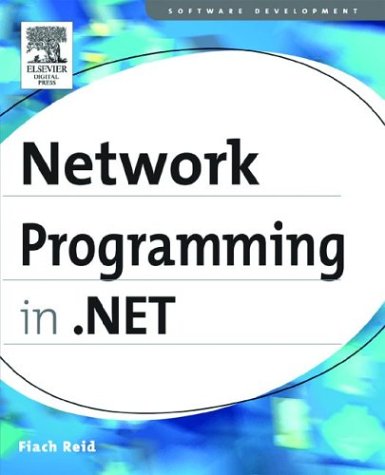
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmptyControlCollection.cs
- ContainerParaClient.cs
- ClientBuildManager.cs
- QueryStringParameter.cs
- OleStrCAMarshaler.cs
- EmptyStringExpandableObjectConverter.cs
- PresentationTraceSources.cs
- DbMetaDataColumnNames.cs
- ChangeInterceptorAttribute.cs
- PageCatalogPart.cs
- PlatformCulture.cs
- UIElementCollection.cs
- mda.cs
- SemanticBasicElement.cs
- ZipIORawDataFileBlock.cs
- AttributeConverter.cs
- SoapExtensionImporter.cs
- ThicknessAnimationUsingKeyFrames.cs
- ApplyTemplatesAction.cs
- SchemaCollectionCompiler.cs
- ApplicationSettingsBase.cs
- TypeReference.cs
- ReadOnlyCollectionBase.cs
- CustomAttributeFormatException.cs
- XmlBoundElement.cs
- StreamGeometry.cs
- SystemIcmpV6Statistics.cs
- Utils.cs
- SmtpFailedRecipientsException.cs
- DataGridRelationshipRow.cs
- GroupStyle.cs
- EntityConnection.cs
- HttpCachePolicyWrapper.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- FocusTracker.cs
- FixedSOMPageElement.cs
- CardSpaceException.cs
- CollectionBuilder.cs
- HwndTarget.cs
- HtmlDocument.cs
- SynchronizingStream.cs
- ComboBoxRenderer.cs
- XmlSerializationWriter.cs
- GPStream.cs
- VsPropertyGrid.cs
- SmtpMail.cs
- EmptyReadOnlyDictionaryInternal.cs
- EdmToObjectNamespaceMap.cs
- ScrollBarRenderer.cs
- PenLineJoinValidation.cs
- UserPersonalizationStateInfo.cs
- Single.cs
- ValidationUtility.cs
- SelectedGridItemChangedEvent.cs
- ArraySortHelper.cs
- DataGridCaption.cs
- AxHost.cs
- InvalidateEvent.cs
- CollectionCodeDomSerializer.cs
- DrawListViewColumnHeaderEventArgs.cs
- SqlFlattener.cs
- VariableDesigner.xaml.cs
- PrivilegedConfigurationManager.cs
- DataAdapter.cs
- XmlIlTypeHelper.cs
- LiteralText.cs
- SqlGenericUtil.cs
- ReturnEventArgs.cs
- ImageCreator.cs
- CurrentChangingEventArgs.cs
- Completion.cs
- CustomCategoryAttribute.cs
- EntityRecordInfo.cs
- ParserStreamGeometryContext.cs
- Validator.cs
- TextEndOfLine.cs
- TraceUtils.cs
- CommentAction.cs
- ChangeDirector.cs
- SqlDataSourceConfigureFilterForm.cs
- XPathConvert.cs
- Set.cs
- ObjectContext.cs
- XmlWriterDelegator.cs
- PauseStoryboard.cs
- RadioButtonAutomationPeer.cs
- ToolStripSplitStackLayout.cs
- TreeViewBindingsEditorForm.cs
- OleDbParameter.cs
- WeakEventManager.cs
- RegistryDataKey.cs
- OutgoingWebRequestContext.cs
- CharStorage.cs
- KeyGestureConverter.cs
- PersistenceTypeAttribute.cs
- PropertyInfoSet.cs
- CustomExpressionEventArgs.cs
- ServiceDesigner.cs
- _HeaderInfoTable.cs
- XmlWriter.cs