Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / XamlBuildTask / Microsoft / Build / Tasks / Xaml / WrappingXamlSchemaContext.cs / 1305376 / WrappingXamlSchemaContext.cs
using System; using System.Collections.Generic; using System.Text; using System.Xaml; using System.Reflection; namespace Microsoft.Build.Tasks.Xaml { class XamlNsReplacingContext : XamlSchemaContext { string localAssemblyName; string realAssemblyName; IDictionaryMasterTypeList; public XamlNsReplacingContext(IEnumerable referenceAssemblies, string localAssemblyName, string realAssemblyName) : base(referenceAssemblies) { this.localAssemblyName = localAssemblyName; this.realAssemblyName = realAssemblyName; MasterTypeList = new Dictionary (); } public override XamlType GetXamlType(Type type) { if (type == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("type")); } XamlNsReplacingType xamlType = null; if (!MasterTypeList.TryGetValue(type, out xamlType)) { xamlType = new XamlNsReplacingType(type, this, localAssemblyName, realAssemblyName); MasterTypeList.Add(type, xamlType); } return xamlType; } protected override XamlType GetXamlType(string xamlNamespace, string name, params XamlType[] typeArguments) { XamlType xamlType = base.GetXamlType(xamlNamespace, name, typeArguments); if (xamlType == null || xamlType.IsUnknown) { xamlNamespace = XamlBuildTaskServices.UpdateClrNamespaceUriWithLocalAssembly(xamlNamespace, this.localAssemblyName, this.realAssemblyName); xamlType = base.GetXamlType(xamlNamespace, name, typeArguments); } return xamlType; } } class XamlNsReplacingType : XamlType { string localAssemblyName; string realAssemblyName; List namespaces; public XamlNsReplacingType(Type underlyingType, XamlSchemaContext context, string localAssemblyName, string realAssemblyName) : base(underlyingType, context) { this.localAssemblyName = localAssemblyName; this.realAssemblyName = realAssemblyName; namespaces = null; } public override IList GetXamlNamespaces() { if (namespaces == null) { namespaces = new List (); IList originalNamespaces = base.GetXamlNamespaces(); foreach (var ns in originalNamespaces) { namespaces.Add(XamlBuildTaskServices.UpdateClrNamespaceUriWithLocalAssembly(ns, this.localAssemblyName, this.realAssemblyName)); } } return namespaces; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
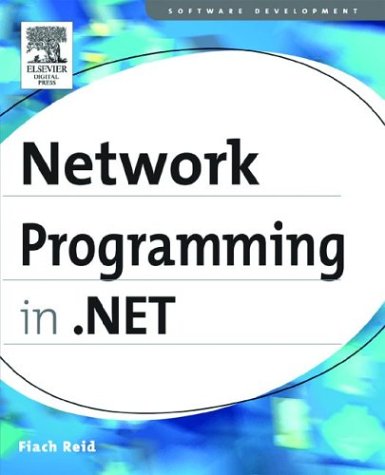
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScriptReferenceBase.cs
- QueryOperator.cs
- PointIndependentAnimationStorage.cs
- MultiView.cs
- SequentialOutput.cs
- ApplicationContext.cs
- SynchronousChannel.cs
- UTF8Encoding.cs
- DeclarationUpdate.cs
- FileInfo.cs
- HttpContextServiceHost.cs
- PageRequestManager.cs
- SimpleHandlerFactory.cs
- FileSystemEnumerable.cs
- ResourceDictionaryCollection.cs
- XmlCountingReader.cs
- EventSourceCreationData.cs
- XmlReaderSettings.cs
- FlowDocumentReaderAutomationPeer.cs
- URI.cs
- ImmutableCollection.cs
- HtmlTableRowCollection.cs
- ToolStripDropTargetManager.cs
- StyleCollection.cs
- XhtmlBasicLiteralTextAdapter.cs
- CancellationHandler.cs
- ModelItemCollectionImpl.cs
- SubMenuStyleCollection.cs
- ClientScriptManager.cs
- SqlRowUpdatingEvent.cs
- IntranetCredentialPolicy.cs
- DataGridTextBox.cs
- PropagatorResult.cs
- UnsupportedPolicyOptionsException.cs
- SqlTriggerAttribute.cs
- ReferenceService.cs
- DBSchemaRow.cs
- XPathBinder.cs
- RelatedImageListAttribute.cs
- XPathDocumentIterator.cs
- SubstitutionDesigner.cs
- TemplateDefinition.cs
- XmlImplementation.cs
- DependencyStoreSurrogate.cs
- FunctionImportElement.cs
- XXXInfos.cs
- SessionParameter.cs
- RadioButtonList.cs
- HeaderUtility.cs
- WebPartUserCapability.cs
- ContextStaticAttribute.cs
- ConfigurationLockCollection.cs
- LiteralControl.cs
- SyndicationElementExtension.cs
- XmlEventCache.cs
- StreamingContext.cs
- XmlIncludeAttribute.cs
- ArraySet.cs
- WizardPanel.cs
- TypeResolver.cs
- ReceiveReply.cs
- UrlPath.cs
- HttpDictionary.cs
- CompositeControl.cs
- MetaForeignKeyColumn.cs
- COM2ExtendedUITypeEditor.cs
- LifetimeServices.cs
- UInt64Converter.cs
- Sql8ConformanceChecker.cs
- ProfileSettingsCollection.cs
- TextRunTypographyProperties.cs
- CompositionAdorner.cs
- ParagraphResult.cs
- DetectRunnableInstancesTask.cs
- ReadOnlyNameValueCollection.cs
- CallbackValidator.cs
- ContextMenuStrip.cs
- ResourcesGenerator.cs
- MatrixKeyFrameCollection.cs
- ProcessInputEventArgs.cs
- TreeNodeSelectionProcessor.cs
- FrameSecurityDescriptor.cs
- SchemaRegistration.cs
- HtmlTableRow.cs
- TreeChangeInfo.cs
- PseudoWebRequest.cs
- WindowsTreeView.cs
- Simplifier.cs
- XmlSchemaImport.cs
- TimeSpanStorage.cs
- FlowDocumentPage.cs
- HierarchicalDataTemplate.cs
- MediaPlayerState.cs
- XamlNamespaceHelper.cs
- Tokenizer.cs
- BufferBuilder.cs
- RowType.cs
- HandlerMappingMemo.cs
- CaseStatement.cs
- DocumentDesigner.cs